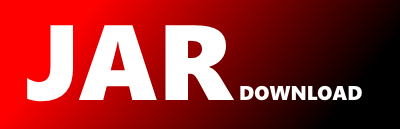
vertx-redis.redis_sentinel.rb Maven / Gradle / Ivy
The newest version!
require 'vertx/vertx'
require 'vertx/util/utils.rb'
# Generated from io.vertx.redis.sentinel.RedisSentinel
module VertxRedis
# Interface for sentinel commands
class RedisSentinel
# @private
# @param j_del [::VertxRedis::RedisSentinel] the java delegate
def initialize(j_del)
@j_del = j_del
end
# @private
# @return [::VertxRedis::RedisSentinel] the underlying java delegate
def j_del
@j_del
end
@@j_api_type = Object.new
def @@j_api_type.accept?(obj)
obj.class == RedisSentinel
end
def @@j_api_type.wrap(obj)
RedisSentinel.new(obj)
end
def @@j_api_type.unwrap(obj)
obj.j_del
end
def self.j_api_type
@@j_api_type
end
def self.j_class
Java::IoVertxRedisSentinel::RedisSentinel.java_class
end
# @param [::Vertx::Vertx] vertx
# @param [Hash] config
# @return [::VertxRedis::RedisSentinel]
def self.create(vertx=nil,config=nil)
if vertx.class.method_defined?(:j_del) && config.class == Hash && !block_given?
return ::Vertx::Util::Utils.safe_create(Java::IoVertxRedisSentinel::RedisSentinel.java_method(:create, [Java::IoVertxCore::Vertx.java_class,Java::IoVertxRedis::RedisOptions.java_class]).call(vertx.j_del,Java::IoVertxRedis::RedisOptions.new(::Vertx::Util::Utils.to_json_object(config))),::VertxRedis::RedisSentinel)
end
raise ArgumentError, "Invalid arguments when calling create(#{vertx},#{config})"
end
# Close the client - when it is fully closed the handler will be called.
# @yield
# @return [void]
def close
if block_given?
return @j_del.java_method(:close, [Java::IoVertxCore::Handler.java_class]).call((Proc.new { |ar| yield(ar.failed ? ar.cause : nil) }))
end
raise ArgumentError, "Invalid arguments when calling close()"
end
# Show a list of monitored masters and their state
# @yield Handler for the result of this call
# @return [self]
def masters
if block_given?
@j_del.java_method(:masters, [Java::IoVertxCore::Handler.java_class]).call((Proc.new { |ar| yield(ar.failed ? ar.cause : nil, ar.succeeded ? ar.result != nil ? JSON.parse(ar.result.encode) : nil : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling masters()"
end
# Show the state and info of the specified master
# @param [String] name master name
# @yield Handler for the result of this call
# @return [self]
def master(name=nil)
if name.class == String && block_given?
@j_del.java_method(:master, [Java::java.lang.String.java_class,Java::IoVertxCore::Handler.java_class]).call(name,(Proc.new { |ar| yield(ar.failed ? ar.cause : nil, ar.succeeded ? ar.result != nil ? JSON.parse(ar.result.encode) : nil : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling master(#{name})"
end
# Show a list of slaves for this master, and their state
# @param [String] name master name
# @yield Handler for the result of this call
# @return [self]
def slaves(name=nil)
if name.class == String && block_given?
@j_del.java_method(:slaves, [Java::java.lang.String.java_class,Java::IoVertxCore::Handler.java_class]).call(name,(Proc.new { |ar| yield(ar.failed ? ar.cause : nil, ar.succeeded ? ar.result != nil ? JSON.parse(ar.result.encode) : nil : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling slaves(#{name})"
end
# Show a list of sentinel instances for this master, and their state
# @param [String] name master name
# @yield Handler for the result of this call
# @return [self]
def sentinels(name=nil)
if name.class == String && block_given?
@j_del.java_method(:sentinels, [Java::java.lang.String.java_class,Java::IoVertxCore::Handler.java_class]).call(name,(Proc.new { |ar| yield(ar.failed ? ar.cause : nil, ar.succeeded ? ar.result != nil ? JSON.parse(ar.result.encode) : nil : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling sentinels(#{name})"
end
# Return the ip and port number of the master with that name.
# If a failover is in progress or terminated successfully for this master
# it returns the address and port of the promoted slave
# @param [String] name master name
# @yield Handler for the result of this call
# @return [self]
def get_master_addr_by_name(name=nil)
if name.class == String && block_given?
@j_del.java_method(:getMasterAddrByName, [Java::java.lang.String.java_class,Java::IoVertxCore::Handler.java_class]).call(name,(Proc.new { |ar| yield(ar.failed ? ar.cause : nil, ar.succeeded ? ar.result != nil ? JSON.parse(ar.result.encode) : nil : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling get_master_addr_by_name(#{name})"
end
# Reset all the masters with matching name.
# The pattern argument is a glob-style pattern.
# The reset process clears any previous state in a master (including a failover in pro
# @param [String] pattern pattern String
# @yield Handler for the result of this call
# @return [self]
def reset(pattern=nil)
if pattern.class == String && block_given?
@j_del.java_method(:reset, [Java::java.lang.String.java_class,Java::IoVertxCore::Handler.java_class]).call(pattern,(Proc.new { |ar| yield(ar.failed ? ar.cause : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling reset(#{pattern})"
end
# Force a failover as if the master was not reachable, and without asking for agreement to other Sentinels
# (however a new version of the configuration will be published so that the other Sentinels
# will update their configurations)
# @param [String] name master name
# @yield Handler for the result of this call
# @return [self]
def failover(name=nil)
if name.class == String && block_given?
@j_del.java_method(:failover, [Java::java.lang.String.java_class,Java::IoVertxCore::Handler.java_class]).call(name,(Proc.new { |ar| yield(ar.failed ? ar.cause : nil, ar.succeeded ? ar.result : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling failover(#{name})"
end
# Check if the current Sentinel configuration is able to reach the quorum needed to failover a master,
# and the majority needed to authorize the failover. This command should be used in monitoring systems
# to check if a Sentinel deployment is ok.
# @param [String] name master name
# @yield Handler for the result of this call
# @return [self]
def ckquorum(name=nil)
if name.class == String && block_given?
@j_del.java_method(:ckquorum, [Java::java.lang.String.java_class,Java::IoVertxCore::Handler.java_class]).call(name,(Proc.new { |ar| yield(ar.failed ? ar.cause : nil, ar.succeeded ? ar.result : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling ckquorum(#{name})"
end
# Force Sentinel to rewrite its configuration on disk, including the current Sentinel state.
# Normally Sentinel rewrites the configuration every time something changes in its state
# (in the context of the subset of the state which is persisted on disk across restart).
# However sometimes it is possible that the configuration file is lost because of operation errors,
# disk failures, package upgrade scripts or configuration managers. In those cases a way to to force Sentinel to
# rewrite the configuration file is handy. This command works even if the previous configuration file
# is completely missing.
# @yield Handler for the result of this call
# @return [self]
def flush_config
if block_given?
@j_del.java_method(:flushConfig, [Java::IoVertxCore::Handler.java_class]).call((Proc.new { |ar| yield(ar.failed ? ar.cause : nil) }))
return self
end
raise ArgumentError, "Invalid arguments when calling flush_config()"
end
end
end
© 2015 - 2025 Weber Informatics LLC | Privacy Policy