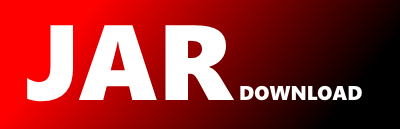
com.yuxuan66.core.db.make.MakeUtils Maven / Gradle / Ivy
package com.yuxuan66.core.db.make;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.List;
import com.yuxuan66.core.db.anno.Column;
import com.yuxuan66.core.db.anno.Id;
import com.yuxuan66.core.db.anno.Table;
import com.yuxuan66.core.db.anno.enums.PrimaryKeyType;
import com.yuxuan66.core.utils.CacheManager;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class MakeUtils {
private static String CachePre = "makesql__";
public static String getTableName(Object obj) {
if (obj == null)
return "";
Table table = obj.getClass().getAnnotation(Table.class);
if (table == null || "".equals(table.value())) {
return obj.getClass().getSimpleName().toLowerCase();
} else {
return table.value();
}
}
public static List getColumns(Object obj) {
List columns = new ArrayList<>();
CacheManager.setData(CachePre + "IDType" + obj, PrimaryKeyType.INCREMENT, 0);
for (Field field : obj.getClass().getDeclaredFields()) {
Column column = field.getAnnotation(Column.class);
Id id = field.getAnnotation(Id.class);
if (column == null && id == null) {
columns.add(field.getName().toLowerCase());
continue;
}
if (column != null && column.notDB()) {
continue;
}
if (id == null) {
columns.add("".equals(column.columnName()) ? field.getName().toLowerCase() : column.columnName());
continue;
}
PrimaryKeyType keyType = id.primaryKeyType();
CacheManager.setData(CachePre + "isGeneratedKey" + obj, id.isGeneratedKey(), 0);
if (keyType != null)
CacheManager.setData(CachePre + "IDType" + obj, keyType, 0);
if (keyType == PrimaryKeyType.INCREMENT || keyType == PrimaryKeyType.UUID) {
continue;
}
String idName = "".equals(column.columnName()) ? field.getName().toLowerCase() : column.columnName();
CacheManager.setData(CachePre + "IDName" + obj, idName, 0);
columns.add(idName);
}
if ("".equals(getIdName(obj))) {
if (!columns.isEmpty()) {
CacheManager.setData(CachePre + "IDName" + obj, columns.get(0), 0);
} else {
log.warn("There is no field and no ID in entity class.");
}
}
return columns;
}
public static String getIdName(Object obj) {
return CacheManager.getData(CachePre + "IDName" + obj);
}
public static Object getFieidVal(Object obj, String fieldName) {
Field field;
try {
field = obj.getClass().getDeclaredField(fieldName);
field.setAccessible(true);
return field.get(obj);
} catch (Exception e) {
e.printStackTrace();
}
return "";
}
public static PrimaryKeyType getIdType(Object obj) {
return CacheManager.getData(CachePre + "IDType" + obj);
}
public static boolean iskey(Object obj) {
return CacheManager.getData(CachePre + "isGeneratedKey" + obj);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy