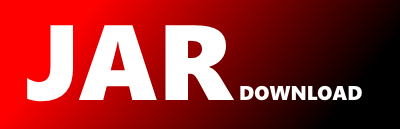
com.yuxuan66.core.proxy.ServiceProxy Maven / Gradle / Ivy
package com.yuxuan66.core.proxy;
import java.lang.reflect.Method;
import com.yuxuan66.core.annotaion.Aop;
import com.yuxuan66.core.annotaion.AopFilter;
import com.yuxuan66.core.annotaion.enums.AopType;
import com.yuxuan66.core.utils.Null;
import net.sf.cglib.proxy.Enhancer;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
public class ServiceProxy implements MethodInterceptor {
private Object target;
@SuppressWarnings({ "unchecked" })
public T getInstance(Object target) {
this.target = target; // 给业务对象赋值
Enhancer enhancer = new Enhancer(); // 创建加强器,用来创建动态代理类
enhancer.setSuperclass(this.target.getClass()); // 为加强器指定要代理的业务类(即:为下面生成的代理类指定父类)
// 设置回调:对于代理类上所有方法的调用,都会调用CallBack,而Callback则需要实现intercept()方法进行拦
enhancer.setCallback(this);
// 创建动态代理类对象并返回
return (T) enhancer.create();
}
@Override
public Object intercept(Object object, Method method, Object[] objects, MethodProxy proxy) throws Throwable {
// 获取AOP注解
Aop aopMethod = method.getAnnotation(Aop.class);
Aop aopClass = object.getClass().getAnnotation(Aop.class);
if (aopMethod == null && aopClass == null) {
return proxy.invokeSuper(object, objects);
}
if (aopMethod == null) {
aopMethod = aopClass;
}
Object run = null;
if (aopMethod.runClass() == Null.class) {
run = object;
} else {
run = aopClass.runClass().newInstance();
}
AopFilter aopFilter = aopMethod.aopFilter();
if (aopMethod.aopType() == AopType.before || aopMethod.aopType() == AopType.both) {
if (inArr(aopFilter.value(), method.getName())) {
// 此方法被过滤
return proxy.invokeSuper(object, objects);
}
run.getClass().getMethod(aopMethod.runMethod()).invoke(object);
}
return proxy.invokeSuper(object, objects);
}
private boolean inArr(String[] arr, String key) {
for (String item : arr) {
if (item.equals(key)) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy