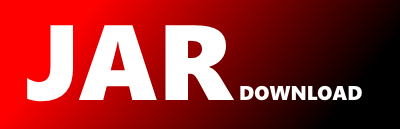
com.zaradai.gluon.kafka.KafkaProducerConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gluon-core Show documentation
Show all versions of gluon-core Show documentation
Gluon - Core micro services implementation.
The newest version!
/**
* Copyright 2017 Zaradai
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.zaradai.gluon.kafka;
import org.apache.kafka.clients.CommonClientConfigs;
import org.apache.kafka.clients.producer.ProducerConfig;
import java.util.Properties;
public class KafkaProducerConfig {
static final String DEFAULT_KEY_SERIALIZER = org.apache.kafka.common.serialization.StringSerializer.class.getName();
static final String DEFAULT_VALUE_SERIALIZER = org.apache.kafka.common.serialization.ByteArraySerializer.class.getName();
static final String DEFAULT_ACKS = "1";
static final int DEFAULT_RETRIES = 0;
static final int DEFAULT_BATCH_SIZE = 8196;
static final long DEFAULT_LINGER = 1;
static final long DEFAULT_BUFFER_MEMORY = 67108864;
static final int DEFAULT_MAX_REQUEST_SIZE = 1024 * 1024;
static final int DEFAULT_REQUEST_TIMEOUT = 60 * 1000;
private String servers;
private String keySerializer;
private String valueSerializer;
private String acks;
private int retries;
private int batchSize;
private long linger;
private long bufferMemory;
private int maxMessageSize;
public KafkaProducerConfig() {
this(KafkaConfig.DEFAULT_SERVERS);
}
public KafkaProducerConfig(String servers) {
setServers(servers).setAcks(DEFAULT_ACKS).setRetries(DEFAULT_RETRIES).setBatchSize(DEFAULT_BATCH_SIZE).setLinger(DEFAULT_LINGER)
.setBufferMemory(DEFAULT_BUFFER_MEMORY).setKeySerializer(DEFAULT_KEY_SERIALIZER).setValueSerializer(DEFAULT_VALUE_SERIALIZER)
.setMaxMessageSize(DEFAULT_MAX_REQUEST_SIZE);
}
public String getServers() {
return servers;
}
public KafkaProducerConfig setServers(String servers) {
this.servers = servers;
return this;
}
public String getKeySerializer() {
return keySerializer;
}
public KafkaProducerConfig setKeySerializer(String keySerializer) {
this.keySerializer = keySerializer;
return this;
}
public String getValueSerializer() {
return valueSerializer;
}
public KafkaProducerConfig setValueSerializer(String valueSerializer) {
this.valueSerializer = valueSerializer;
return this;
}
public String getAcks() {
return acks;
}
public KafkaProducerConfig setAcks(String acks) {
this.acks = acks;
return this;
}
public int getRetries() {
return retries;
}
public KafkaProducerConfig setRetries(int retries) {
this.retries = retries;
return this;
}
public int getBatchSize() {
return batchSize;
}
public KafkaProducerConfig setBatchSize(int batchSize) {
this.batchSize = batchSize;
return this;
}
public long getLinger() {
return linger;
}
public KafkaProducerConfig setLinger(long linger) {
this.linger = linger;
return this;
}
public long getBufferMemory() {
return bufferMemory;
}
public KafkaProducerConfig setBufferMemory(long bufferMemory) {
this.bufferMemory = bufferMemory;
return this;
}
public int getMaxMessageSize() {
return maxMessageSize;
}
public KafkaProducerConfig setMaxMessageSize(int maxMessageSize) {
this.maxMessageSize = maxMessageSize;
return this;
}
public Properties getProperties() {
Properties properties = new Properties();
properties.put(CommonClientConfigs.BOOTSTRAP_SERVERS_CONFIG, getServers());
properties.put(ProducerConfig.ACKS_CONFIG, getAcks());
properties.put(ProducerConfig.RETRIES_CONFIG, getRetries());
properties.put(ProducerConfig.BATCH_SIZE_CONFIG, getBatchSize());
properties.put(ProducerConfig.LINGER_MS_CONFIG, getLinger());
properties.put(ProducerConfig.BUFFER_MEMORY_CONFIG, getBufferMemory());
properties.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, getKeySerializer());
properties.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, getValueSerializer());
properties.put(ProducerConfig.MAX_REQUEST_SIZE_CONFIG, getMaxMessageSize());
properties.put(ProducerConfig.REQUEST_TIMEOUT_MS_CONFIG, DEFAULT_REQUEST_TIMEOUT);
return properties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy