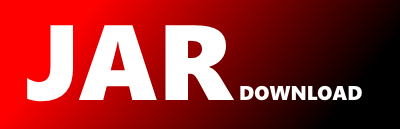
com.zaradai.gluon.server.MicroServiceFactory Maven / Gradle / Ivy
Show all versions of gluon-core Show documentation
/**
* Copyright 2017 Zaradai
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.zaradai.gluon.server;
import com.zaradai.gluon.MicroService;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Executor;
import java.util.concurrent.ForkJoinPool;
import java.util.function.Function;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.zaradai.gluon.util.Validations.checkString;
public final class MicroServiceFactory {
public static MicroService fixed(String serviceName, Rep reply) {
return new MicroServiceFactory.FixedMicroService<>(serviceName, CompletableFuture.completedFuture(reply));
}
public static MicroService failing(String serviceName, Throwable throwable) {
CompletableFuture completableFuture = new CompletableFuture<>();
completableFuture.completeExceptionally(throwable);
return new MicroServiceFactory.FixedMicroService<>(serviceName, completableFuture);
}
public static MicroService create(String serviceName, Function function) {
return new MicroServiceFactory.MicroServiceImpl<>(serviceName, function, false);
}
public static MicroService createAsync(String serviceName, Function function) {
return new MicroServiceFactory.MicroServiceImpl<>(serviceName, function, true);
}
public static MicroService createAsync(String serviceName, Function function, Executor executor) {
return new MicroServiceFactory.MicroServiceImpl<>(serviceName, function, true, executor);
}
private static abstract class AbstractMicroService implements MicroService {
private final String name;
protected AbstractMicroService(String name) {
this.name = checkString(name, "Service name cannot be null or empty");
}
@Override
public Status getStatus() {
return Status.OPEN;
}
@Override
public String name() {
return name;
}
}
private static class FixedMicroService extends MicroServiceFactory.AbstractMicroService {
private final CompletableFuture rep;
public FixedMicroService(String name, CompletableFuture rep) {
super(name);
this.rep = rep;
}
@Override
public CompletableFuture apply(Req req) {
return rep;
}
}
private static class MicroServiceImpl extends MicroServiceFactory.AbstractMicroService {
private final Function function;
private final Executor executor;
private final boolean async;
private MicroServiceImpl(String name, Function function, boolean async) {
this(name, function, async, ForkJoinPool.commonPool());
}
private MicroServiceImpl(String name, Function function, boolean async, Executor executor) {
super(name);
this.function = checkNotNull(function, "Function cannot be null");
this.executor = checkNotNull(executor, "Supplied executor is invalid.");
this.async = async;
}
@Override
public CompletableFuture apply(Req req) {
if (async) {
return CompletableFuture.supplyAsync(() -> function.apply(req), executor);
} else {
return CompletableFuture.completedFuture(function.apply(req));
}
}
}
}