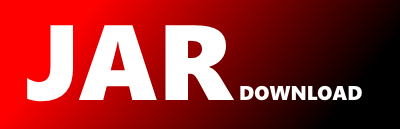
com.zaxxer.hikari.util.ConcurrentBag Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of HikariCP Show documentation
Show all versions of HikariCP Show documentation
Ultimate JDBC Connection Pool
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy