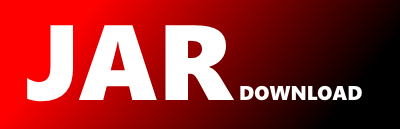
commonMain.com.zegreatrob.testmints.async.TestTemplate.kt Maven / Gradle / Ivy
package com.zegreatrob.testmints.async
import com.zegreatrob.testmints.report.ReporterProvider
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.CoroutineStart
import kotlinx.coroutines.async
class TestTemplate(
private val reporterProvider: ReporterProvider,
private val templateScope: CoroutineScope = mintScope(),
val wrapper: suspend (TestFunc) -> Unit,
) {
fun extend(wrapper: suspend (SC, TestFunc) -> Unit) = TestTemplate(reporterProvider) { test ->
this.wrapper { sc1 -> wrapper(sc1, test) }
}
fun extend(sharedSetup: suspend (SC) -> SC2, sharedTeardown: suspend (SC2) -> Unit = {}) = extend { sc1, test ->
val sc2 = sharedSetup(sc1)
test(sc2)
sharedTeardown(sc2)
}
fun extend(sharedSetup: suspend () -> Unit = {}, sharedTeardown: suspend () -> Unit = {}) = TestTemplate(reporterProvider) { test ->
wrapper {
sharedSetup()
test(it)
sharedTeardown()
}
}
fun extend(beforeAll: suspend () -> BAC): TestTemplate = extend(
beforeAll = beforeAll,
mergeContext = { _, bac -> bac },
)
fun extend(
beforeAll: suspend () -> BAC,
mergeContext: suspend (SC, BAC) -> SC2,
): TestTemplate {
val deferred = templateScope.async(start = CoroutineStart.LAZY) { beforeAll() }
return extend(sharedSetup = { sharedContext ->
mergeContext(sharedContext, deferred.await())
})
}
operator fun invoke(context: C, timeoutMs: Long = 60_000L, additionalActions: suspend C.() -> Unit = {}) = Setup(
{ context },
context.chooseTestScope(),
additionalActions,
reporterProvider.reporter,
timeoutMs,
wrapper,
)
operator fun invoke(timeoutMs: Long = 60_000L, additionalActions: suspend SC.() -> Unit = {}) = Setup(
{ it },
mintScope(),
additionalActions,
reporterProvider.reporter,
timeoutMs,
wrapper,
)
fun with(
contextProvider: suspend (SC) -> C,
timeoutMs: Long = 60_000L,
additionalActions: suspend C.() -> Unit = {},
) = Setup(contextProvider, mintScope(), additionalActions, reporterProvider.reporter, timeoutMs, wrapper)
}
fun TestTemplate.with(
contextProvider: suspend () -> C,
timeoutMs: Long = 60_000L,
additionalAction: suspend C.() -> Unit = {},
): Setup {
val unitSharedContextAdapter: suspend (Unit) -> C = { contextProvider() }
return with(unitSharedContextAdapter, timeoutMs, additionalAction)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy