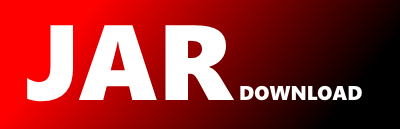
simkit.stat.PercentageInStateStat Maven / Gradle / Ivy
package simkit.stat;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.TreeMap;
import simkit.Schedule;
/**
* Listens for states of a given name and computes the percentage of time
* spent in each state. The assumption is that the name of the property
* can be in one, and only one, of a number of discrete states at a time.
* The actual states are determined on-the-fly, but an initial state is
* required to be given.
*
* @version $Id$
* @author ahbuss
*/
public class PercentageInStateStat implements PropertyChangeListener {
private NumberFormat numberFormat;
private Object initialState;
private String propertyName;
protected Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy