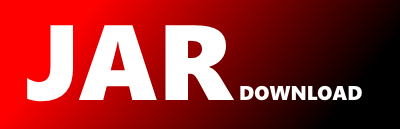
com.zepben.vertxutils.json.LazyJsonArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-utils Show documentation
Show all versions of vertx-utils Show documentation
Helpers and utils for working with Vert.x in Zepben projects.
/*
* Copyright 2020 Zeppelin Bend Pty Ltd
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at https://mozilla.org/MPL/2.0/.
*/
package com.zepben.vertxutils.json;
import com.zepben.annotations.EverythingIsNonnullByDefault;
import io.vertx.core.buffer.Buffer;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import java.time.Instant;
import java.util.Iterator;
import java.util.List;
import java.util.Spliterator;
import java.util.function.Consumer;
import java.util.function.Supplier;
import java.util.stream.Stream;
@EverythingIsNonnullByDefault
public class LazyJsonArray extends io.vertx.core.json.JsonArray {
private final Supplier supplier;
private boolean materialised = false;
public LazyJsonArray(Supplier supplier) {
this.supplier = supplier;
}
@Override
public String getString(int pos) {
checkLoad();
return super.getString(pos);
}
@Override
public Integer getInteger(int pos) {
checkLoad();
return super.getInteger(pos);
}
@Override
public Long getLong(int pos) {
checkLoad();
return super.getLong(pos);
}
@Override
public Double getDouble(int pos) {
checkLoad();
return super.getDouble(pos);
}
@Override
public Float getFloat(int pos) {
checkLoad();
return super.getFloat(pos);
}
@Override
public Boolean getBoolean(int pos) {
checkLoad();
return super.getBoolean(pos);
}
@Override
public JsonObject getJsonObject(int pos) {
checkLoad();
return super.getJsonObject(pos);
}
@Override
public JsonArray getJsonArray(int pos) {
checkLoad();
return super.getJsonArray(pos);
}
@Override
public byte[] getBinary(int pos) {
checkLoad();
return super.getBinary(pos);
}
@Override
public Instant getInstant(int pos) {
checkLoad();
return super.getInstant(pos);
}
@Override
public Object getValue(int pos) {
checkLoad();
return super.getValue(pos);
}
@Override
public boolean hasNull(int pos) {
checkLoad();
return super.hasNull(pos);
}
@Override
public boolean contains(Object value) {
checkLoad();
return super.contains(value);
}
@Override
public boolean remove(Object value) {
checkLoad();
return super.remove(value);
}
@Override
public Object remove(int pos) {
checkLoad();
return super.remove(pos);
}
@Override
public int size() {
checkLoad();
return super.size();
}
@Override
public boolean isEmpty() {
checkLoad();
return super.isEmpty();
}
@Override
public List> getList() {
checkLoad();
return super.getList();
}
@Override
public Iterator
© 2015 - 2024 Weber Informatics LLC | Privacy Policy