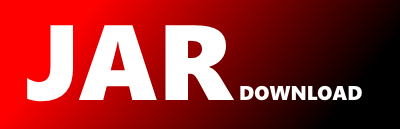
com.zepben.vertxutils.routing.handlers.params.QueryParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-utils Show documentation
Show all versions of vertx-utils Show documentation
Helpers and utils for working with Vert.x in Zepben projects.
/*
* Copyright 2020 Zeppelin Bend Pty Ltd
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at https://mozilla.org/MPL/2.0/.
*/
package com.zepben.vertxutils.routing.handlers.params;
import com.zepben.annotations.EverythingIsNonnullByDefault;
import org.jetbrains.annotations.Contract;
import javax.annotation.Nullable;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
@SuppressWarnings("WeakerAccess")
@EverythingIsNonnullByDefault
public class QueryParams {
private final Set> validRules;
private final Map> params;
public QueryParams(Set> validRules, Map> params) {
this.validRules = validRules;
this.params = params;
}
/**
* Get the first values passed via the query string for the given rule or the default value if no values were passed.
*
* @param rule The {@link QueryParamRule} to get the value for.
* @param The value type defined by the {@code rule}.
* @return The first values passed via the query string or the rules default value if no values were passed.
*/
public T get(QueryParamRule rule) {
return getAll(rule).get(0);
}
/**
* Get the first values passed via the query string for the given rule or the specified value if no values were passed.
*
* @param rule The {@link QueryParamRule} to get the value for.
* @param other The value to use if no values were passed via the query string.
* @param The value type defined by the {@code rule}.
* @return The first values passed via the query string or {@code other} if no values were passed.
*/
@Nullable
@Contract("_, !null, -> !null")
public T getOrElse(QueryParamRule rule, @Nullable T other) {
return getAllOrElse(rule, other).get(0);
}
/**
* Get all of the values passed via the query string for the given rule or the default value if no values were passed.
*
* @param rule The {@link QueryParamRule} to get the value for.
* @param The value type defined by the {@code rule}.
* @return The list of values passed via the query string or the rules default value if {@code other} is an empty list.
*/
public List getAll(QueryParamRule rule) {
List values = getAllValues(rule);
if (values == null || values.isEmpty()) {
T defaultValue = rule.defaultValue();
if (defaultValue == null)
throw new IllegalArgumentException(String.format("INTERNAL ERROR: Param %s has no values and no default. Either mark the param as required, provide a default or use with getOrElse or getAllOrElse.", rule.name()));
else
return Collections.singletonList(defaultValue);
}
return values;
}
/**
* Get all of the values passed via the query string for the given rule or the specified value if no values were passed.
*
* @param rule The {@link QueryParamRule} to get the value for.
* @param other The value to return if no values were passed via the query string.
* @param The value type defined by the {@code rule}.
* @return The list of values passed via the query string or a list containing {@code other} if no values were found.
*/
public List getAllOrElse(QueryParamRule rule, @Nullable T other) {
return getAllOrElse(rule, Collections.singletonList(other));
}
/**
* Get all of the values passed via the query string for the given rule or the specified value if no values were passed.
*
* @param rule The {@link QueryParamRule} to get the value for.
* @param other The list of values to return if no values were passed via the query string.
* @param The value type defined by the {@code rule}.
* @return The list of values passed via the query string or {@code other} if no values were found.
*/
public List getAllOrElse(QueryParamRule rule, List other) {
List values = getAllValues(rule);
if (values == null || values.isEmpty())
return other;
else
return values;
}
/**
* @param rule The {@link QueryParamRule} to get the value for.
* @param The value type defined by the {@code rule}.
* @return True if at least one value was passed for the {@code rule} via the query string.
*/
public boolean exists(QueryParamRule rule) {
return params.containsKey(rule.name());
}
@SuppressWarnings("unchecked")
@Nullable
private List getAllValues(QueryParamRule rule) {
if (!validRules.contains(rule))
throw new IllegalArgumentException(String.format("INTERNAL ERROR: Query param %s was not registered with this route. Did you forget to register it?", rule.name()));
return (List) params.get(rule.name());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy