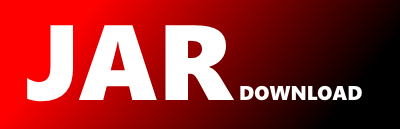
Glacier2.RouterPrx Maven / Gradle / Ivy
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
//
// Ice version 3.7.7
//
//
//
// Generated from file `Router.ice'
//
// Warning: do not edit this file.
//
//
//
package Glacier2;
/**
* The Glacier2 specialization of the Ice::Router
interface.
*
**/
public interface RouterPrx extends Ice.RouterPrx
{
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @return The category.
*
**/
public String getCategoryForClient();
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param context The Context map to send with the invocation.
* @return The category.
*
**/
public String getCategoryForClient(java.util.Map context);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient();
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(java.util.Map context);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(Ice.Callback cb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(java.util.Map context, Ice.Callback cb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(Callback_Router_getCategoryForClient cb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(java.util.Map context, Callback_Router_getCategoryForClient cb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getCategoryForClient(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* This category must be used in the identities of all of the client's
* callback objects. This is necessary in order for the router to
* forward callback requests to the intended client. If the Glacier2
* server endpoints are not set, the returned category is an empty
* string.
*
* @param result The asynchronous result object.
* @return The category.
*
**/
public String end_getCategoryForClient(Ice.AsyncResult result);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @see Session
* @see SessionManager
* @see PermissionsVerifier
*
* @return A proxy for the newly created session, or null if no
* {@link SessionManager} has been installed.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @throws PermissionDeniedException Raised if the password for
* the given user id is not correct, or if the user is not allowed
* access.
*
* @throws CannotCreateSessionException Raised if the session
* cannot be created.
*
**/
public SessionPrx createSession(String userId, String password)
throws CannotCreateSessionException,
PermissionDeniedException;
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @see Session
* @see SessionManager
* @see PermissionsVerifier
*
* @param context The Context map to send with the invocation.
* @return A proxy for the newly created session, or null if no
* {@link SessionManager} has been installed.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @throws PermissionDeniedException Raised if the password for
* the given user id is not correct, or if the user is not allowed
* access.
*
* @throws CannotCreateSessionException Raised if the session
* cannot be created.
*
**/
public SessionPrx createSession(String userId, String password, java.util.Map context)
throws CannotCreateSessionException,
PermissionDeniedException;
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId, String password);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId, String password, java.util.Map context);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId, String password, Ice.Callback cb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId, String password, java.util.Map context, Ice.Callback cb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId, String password, Callback_Router_createSession cb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId, String password, java.util.Map context, Callback_Router_createSession cb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId,
String password,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId,
String password,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId,
String password,
java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param userId The user id for which to check the password.
*
* @param password The password for the given user id.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSession(String userId,
String password,
java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Create a per-client session with the router. If a
* {@link SessionManager} has been installed, a proxy to a {@link Session}
* object is returned to the client. Otherwise, null is returned
* and only an internal session (i.e., not visible to the client)
* is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param result The asynchronous result object.
* @see Session
* @see SessionManager
* @see PermissionsVerifier
*
* @return A proxy for the newly created session, or null if no
* {@link SessionManager} has been installed.
*
* @throws PermissionDeniedException Raised if the password for
* the given user id is not correct, or if the user is not allowed
* access.
*
* @throws CannotCreateSessionException Raised if the session
* cannot be created.
*
**/
public SessionPrx end_createSession(Ice.AsyncResult result)
throws CannotCreateSessionException,
PermissionDeniedException;
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @see Session
* @see SessionManager
* @see PermissionsVerifier
*
* @return A proxy for the newly created session, or null if no
* {@link SessionManager} has been installed.
*
* @throws PermissionDeniedException Raised if the user cannot be
* authenticated or if the user is not allowed access.
*
* @throws CannotCreateSessionException Raised if the session
* cannot be created.
*
**/
public SessionPrx createSessionFromSecureConnection()
throws CannotCreateSessionException,
PermissionDeniedException;
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @see Session
* @see SessionManager
* @see PermissionsVerifier
*
* @param context The Context map to send with the invocation.
* @return A proxy for the newly created session, or null if no
* {@link SessionManager} has been installed.
*
* @throws PermissionDeniedException Raised if the user cannot be
* authenticated or if the user is not allowed access.
*
* @throws CannotCreateSessionException Raised if the session
* cannot be created.
*
**/
public SessionPrx createSessionFromSecureConnection(java.util.Map context)
throws CannotCreateSessionException,
PermissionDeniedException;
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection();
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(java.util.Map context);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(Ice.Callback cb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(java.util.Map context, Ice.Callback cb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(Callback_Router_createSessionFromSecureConnection cb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(java.util.Map context, Callback_Router_createSessionFromSecureConnection cb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_createSessionFromSecureConnection(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Create a per-client session with the router. The user is
* authenticated through the SSL certificates that have been
* associated with the connection. If a {@link SessionManager} has been
* installed, a proxy to a {@link Session} object is returned to the
* client. Otherwise, null is returned and only an internal
* session (i.e., not visible to the client) is created.
*
* If a session proxy is returned, it must be configured to route
* through the router that created it. This will happen automatically
* if the router is configured as the client's default router at the
* time the session proxy is created in the client process, otherwise
* the client must configure the session proxy explicitly.
*
* @param result The asynchronous result object.
* @see Session
* @see SessionManager
* @see PermissionsVerifier
*
* @return A proxy for the newly created session, or null if no
* {@link SessionManager} has been installed.
*
* @throws PermissionDeniedException Raised if the user cannot be
* authenticated or if the user is not allowed access.
*
* @throws CannotCreateSessionException Raised if the session
* cannot be created.
*
**/
public SessionPrx end_createSessionFromSecureConnection(Ice.AsyncResult result)
throws CannotCreateSessionException,
PermissionDeniedException;
/**
* Keep the calling client's session with this router alive.
*
* @throws SessionNotExistException Raised if no session exists
* for the calling client.
*
**/
public void refreshSession()
throws SessionNotExistException;
/**
* Keep the calling client's session with this router alive.
*
* @throws SessionNotExistException Raised if no session exists
* for the calling client.
*
* @param context The Context map to send with the invocation.
**/
public void refreshSession(java.util.Map context)
throws SessionNotExistException;
/**
* Keep the calling client's session with this router alive.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession();
/**
* Keep the calling client's session with this router alive.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(java.util.Map context);
/**
* Keep the calling client's session with this router alive.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(Ice.Callback cb);
/**
* Keep the calling client's session with this router alive.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(java.util.Map context, Ice.Callback cb);
/**
* Keep the calling client's session with this router alive.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(Callback_Router_refreshSession cb);
/**
* Keep the calling client's session with this router alive.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(java.util.Map context, Callback_Router_refreshSession cb);
/**
* Keep the calling client's session with this router alive.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Keep the calling client's session with this router alive.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Keep the calling client's session with this router alive.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(java.util.Map context,
IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Keep the calling client's session with this router alive.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_refreshSession(java.util.Map context,
IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Keep the calling client's session with this router alive.
*
* @param result The asynchronous result object.
* @throws SessionNotExistException Raised if no session exists
* for the calling client.
*
**/
public void end_refreshSession(Ice.AsyncResult result)
throws SessionNotExistException;
/**
* Destroy the calling client's session with this router.
*
* @throws SessionNotExistException Raised if no session exists
* for the calling client.
*
**/
public void destroySession()
throws SessionNotExistException;
/**
* Destroy the calling client's session with this router.
*
* @throws SessionNotExistException Raised if no session exists
* for the calling client.
*
* @param context The Context map to send with the invocation.
**/
public void destroySession(java.util.Map context)
throws SessionNotExistException;
/**
* Destroy the calling client's session with this router.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession();
/**
* Destroy the calling client's session with this router.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(java.util.Map context);
/**
* Destroy the calling client's session with this router.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(Ice.Callback cb);
/**
* Destroy the calling client's session with this router.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(java.util.Map context, Ice.Callback cb);
/**
* Destroy the calling client's session with this router.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(Callback_Router_destroySession cb);
/**
* Destroy the calling client's session with this router.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(java.util.Map context, Callback_Router_destroySession cb);
/**
* Destroy the calling client's session with this router.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Destroy the calling client's session with this router.
*
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Destroy the calling client's session with this router.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(java.util.Map context,
IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Destroy the calling client's session with this router.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param userExceptionCb The lambda user exception callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroySession(java.util.Map context,
IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 userExceptionCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Destroy the calling client's session with this router.
*
* @param result The asynchronous result object.
* @throws SessionNotExistException Raised if no session exists
* for the calling client.
*
**/
public void end_destroySession(Ice.AsyncResult result)
throws SessionNotExistException;
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @return The timeout (in seconds).
*
**/
public long getSessionTimeout();
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param context The Context map to send with the invocation.
* @return The timeout (in seconds).
*
**/
public long getSessionTimeout(java.util.Map context);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout();
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(Ice.Callback cb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context, Ice.Callback cb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(Callback_Router_getSessionTimeout cb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context, Callback_Router_getSessionTimeout cb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(IceInternal.Functional_LongCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(IceInternal.Functional_LongCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context,
IceInternal.Functional_LongCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context,
IceInternal.Functional_LongCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Get the value of the session timeout. Sessions are destroyed
* if they see no activity for this period of time.
*
* @param result The asynchronous result object.
* @return The timeout (in seconds).
*
**/
public long end_getSessionTimeout(Ice.AsyncResult result);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @return The timeout (in seconds).
*
**/
public int getACMTimeout();
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param context The Context map to send with the invocation.
* @return The timeout (in seconds).
*
**/
public int getACMTimeout(java.util.Map context);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout();
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(java.util.Map context);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(Ice.Callback cb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(java.util.Map context, Ice.Callback cb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(Callback_Router_getACMTimeout cb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(java.util.Map context, Callback_Router_getACMTimeout cb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(java.util.Map context,
IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getACMTimeout(java.util.Map context,
IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Get the value of the ACM timeout. Clients supporting connection
* heartbeats can enable them instead of explicitly sending keep
* alives requests.
*
* NOTE: This method is only available since Ice 3.6.
*
* @param result The asynchronous result object.
* @return The timeout (in seconds).
*
**/
public int end_getACMTimeout(Ice.AsyncResult result);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy