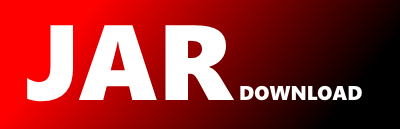
Glacier2.SessionControlPrx Maven / Gradle / Ivy
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
//
// Ice version 3.7.7
//
//
//
// Generated from file `Session.ice'
//
// Warning: do not edit this file.
//
//
//
package Glacier2;
/**
* An administrative session control object, which is tied to the
* lifecycle of a {@link Session}.
*
* @see Session
*
**/
public interface SessionControlPrx extends Ice.ObjectPrx
{
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @return A StringSet object.
*
**/
public StringSetPrx categories();
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param context The Context map to send with the invocation.
* @return A StringSet object.
*
**/
public StringSetPrx categories(java.util.Map context);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories();
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(java.util.Map context);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(Ice.Callback cb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(java.util.Map context, Ice.Callback cb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(Callback_SessionControl_categories cb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(java.util.Map context, Callback_SessionControl_categories cb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_categories(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Access the object that manages the allowable categories
* for object identities for this session.
*
* @param result The asynchronous result object.
* @return A StringSet object.
*
**/
public StringSetPrx end_categories(Ice.AsyncResult result);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @return A StringSet object.
*
**/
public StringSetPrx adapterIds();
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param context The Context map to send with the invocation.
* @return A StringSet object.
*
**/
public StringSetPrx adapterIds(java.util.Map context);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds();
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(java.util.Map context);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(Ice.Callback cb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(java.util.Map context, Ice.Callback cb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(Callback_SessionControl_adapterIds cb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(java.util.Map context, Callback_SessionControl_adapterIds cb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_adapterIds(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Access the object that manages the allowable adapter identities
* for objects for this session.
*
* @param result The asynchronous result object.
* @return A StringSet object.
*
**/
public StringSetPrx end_adapterIds(Ice.AsyncResult result);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @return An IdentitySet object.
*
**/
public IdentitySetPrx identities();
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param context The Context map to send with the invocation.
* @return An IdentitySet object.
*
**/
public IdentitySetPrx identities(java.util.Map context);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities();
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(java.util.Map context);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(Ice.Callback cb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(java.util.Map context, Ice.Callback cb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(Callback_SessionControl_identities cb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(java.util.Map context, Callback_SessionControl_identities cb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_identities(java.util.Map context,
IceInternal.Functional_GenericCallback1 responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Access the object that manages the allowable object identities
* for this session.
*
* @param result The asynchronous result object.
* @return An IdentitySet object.
*
**/
public IdentitySetPrx end_identities(Ice.AsyncResult result);
/**
* Get the session timeout.
*
* @return The timeout.
*
**/
public int getSessionTimeout();
/**
* Get the session timeout.
*
* @param context The Context map to send with the invocation.
* @return The timeout.
*
**/
public int getSessionTimeout(java.util.Map context);
/**
* Get the session timeout.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout();
/**
* Get the session timeout.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context);
/**
* Get the session timeout.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(Ice.Callback cb);
/**
* Get the session timeout.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context, Ice.Callback cb);
/**
* Get the session timeout.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(Callback_SessionControl_getSessionTimeout cb);
/**
* Get the session timeout.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context, Callback_SessionControl_getSessionTimeout cb);
/**
* Get the session timeout.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Get the session timeout.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Get the session timeout.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context,
IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Get the session timeout.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getSessionTimeout(java.util.Map context,
IceInternal.Functional_IntCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Get the session timeout.
*
* @param result The asynchronous result object.
* @return The timeout.
*
**/
public int end_getSessionTimeout(Ice.AsyncResult result);
/**
* Destroy the associated session.
*
**/
public void destroy();
/**
* Destroy the associated session.
*
* @param context The Context map to send with the invocation.
**/
public void destroy(java.util.Map context);
/**
* Destroy the associated session.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy();
/**
* Destroy the associated session.
*
* @param context The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(java.util.Map context);
/**
* Destroy the associated session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(Ice.Callback cb);
/**
* Destroy the associated session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(java.util.Map context, Ice.Callback cb);
/**
* Destroy the associated session.
*
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(Callback_SessionControl_destroy cb);
/**
* Destroy the associated session.
*
* @param context The Context map to send with the invocation.
* @param cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(java.util.Map context, Callback_SessionControl_destroy cb);
/**
* Destroy the associated session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Destroy the associated session.
*
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Destroy the associated session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(java.util.Map context,
IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb);
/**
* Destroy the associated session.
*
* @param context The Context map to send with the invocation.
* @param responseCb The lambda response callback.
* @param exceptionCb The lambda exception callback.
* @param sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_destroy(java.util.Map context,
IceInternal.Functional_VoidCallback responseCb,
IceInternal.Functional_GenericCallback1 exceptionCb,
IceInternal.Functional_BoolCallback sentCb);
/**
* Destroy the associated session.
*
* @param result The asynchronous result object.
**/
public void end_destroy(Ice.AsyncResult result);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy