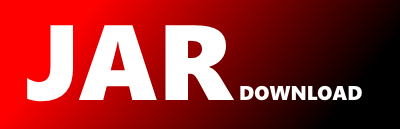
Glacier2._RouterDisp Maven / Gradle / Ivy
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
//
// Ice version 3.7.7
//
//
//
// Generated from file `Router.ice'
//
// Warning: do not edit this file.
//
//
//
package Glacier2;
public abstract class _RouterDisp extends Ice.ObjectImpl implements Router
{
private static final String[] _ids =
{
"::Glacier2::Router",
"::Ice::Object",
"::Ice::Router"
};
public boolean ice_isA(String s)
{
return java.util.Arrays.binarySearch(_ids, s) >= 0;
}
public boolean ice_isA(String s, Ice.Current current)
{
return java.util.Arrays.binarySearch(_ids, s) >= 0;
}
public String[] ice_ids()
{
return _ids;
}
public String[] ice_ids(Ice.Current current)
{
return _ids;
}
public String ice_id()
{
return _ids[0];
}
public String ice_id(Ice.Current current)
{
return _ids[0];
}
public static String ice_staticId()
{
return _ids[0];
}
public final Ice.ObjectPrx getClientProxy(Ice.BooleanHolder hasRoutingTable)
{
return getClientProxy(hasRoutingTable, null);
}
public final Ice.ObjectPrx getServerProxy()
{
return getServerProxy(null);
}
public final Ice.ObjectPrx[] addProxies(Ice.ObjectPrx[] proxies)
{
return addProxies(proxies, null);
}
public final String getCategoryForClient()
{
return getCategoryForClient(null);
}
public final void createSession_async(AMD_Router_createSession cb, String userId, String password)
throws CannotCreateSessionException,
PermissionDeniedException
{
createSession_async(cb, userId, password, null);
}
public final void createSessionFromSecureConnection_async(AMD_Router_createSessionFromSecureConnection cb)
throws CannotCreateSessionException,
PermissionDeniedException
{
createSessionFromSecureConnection_async(cb, null);
}
public final void refreshSession_async(AMD_Router_refreshSession cb)
throws SessionNotExistException
{
refreshSession_async(cb, null);
}
public final void destroySession()
throws SessionNotExistException
{
destroySession(null);
}
public final long getSessionTimeout()
{
return getSessionTimeout(null);
}
public final int getACMTimeout()
{
return getACMTimeout(null);
}
public static boolean _iceD_getCategoryForClient(Router obj, IceInternal.Incoming inS, Ice.Current current)
throws Ice.UserException
{
_iceCheckMode(Ice.OperationMode.Idempotent, current.mode);
inS.readEmptyParams();
String ret = obj.getCategoryForClient(current);
Ice.OutputStream ostr = inS.startWriteParams();
ostr.writeString(ret);
inS.endWriteParams();
return true;
}
public static boolean _iceD_createSession(Router obj, IceInternal.Incoming inS, Ice.Current current)
throws Ice.UserException
{
_iceCheckMode(Ice.OperationMode.Normal, current.mode);
Ice.InputStream istr = inS.startReadParams();
String iceP_userId;
String iceP_password;
iceP_userId = istr.readString();
iceP_password = istr.readString();
inS.endReadParams();
inS.setFormat(Ice.FormatType.SlicedFormat);
AMD_Router_createSession cb = new _AMD_Router_createSession(inS);
obj.createSession_async(cb, iceP_userId, iceP_password, current);
return false;
}
public static boolean _iceD_createSessionFromSecureConnection(Router obj, IceInternal.Incoming inS, Ice.Current current)
throws Ice.UserException
{
_iceCheckMode(Ice.OperationMode.Normal, current.mode);
inS.readEmptyParams();
inS.setFormat(Ice.FormatType.SlicedFormat);
AMD_Router_createSessionFromSecureConnection cb = new _AMD_Router_createSessionFromSecureConnection(inS);
obj.createSessionFromSecureConnection_async(cb, current);
return false;
}
public static boolean _iceD_refreshSession(Router obj, IceInternal.Incoming inS, Ice.Current current)
throws Ice.UserException
{
_iceCheckMode(Ice.OperationMode.Normal, current.mode);
inS.readEmptyParams();
AMD_Router_refreshSession cb = new _AMD_Router_refreshSession(inS);
obj.refreshSession_async(cb, current);
return false;
}
public static boolean _iceD_destroySession(Router obj, IceInternal.Incoming inS, Ice.Current current)
throws Ice.UserException
{
_iceCheckMode(Ice.OperationMode.Normal, current.mode);
inS.readEmptyParams();
obj.destroySession(current);
inS.writeEmptyParams();
return true;
}
public static boolean _iceD_getSessionTimeout(Router obj, IceInternal.Incoming inS, Ice.Current current)
throws Ice.UserException
{
_iceCheckMode(Ice.OperationMode.Idempotent, current.mode);
inS.readEmptyParams();
long ret = obj.getSessionTimeout(current);
Ice.OutputStream ostr = inS.startWriteParams();
ostr.writeLong(ret);
inS.endWriteParams();
return true;
}
public static boolean _iceD_getACMTimeout(Router obj, IceInternal.Incoming inS, Ice.Current current)
throws Ice.UserException
{
_iceCheckMode(Ice.OperationMode.Idempotent, current.mode);
inS.readEmptyParams();
int ret = obj.getACMTimeout(current);
Ice.OutputStream ostr = inS.startWriteParams();
ostr.writeInt(ret);
inS.endWriteParams();
return true;
}
private final static String[] _all =
{
"addProxies",
"createSession",
"createSessionFromSecureConnection",
"destroySession",
"getACMTimeout",
"getCategoryForClient",
"getClientProxy",
"getServerProxy",
"getSessionTimeout",
"ice_id",
"ice_ids",
"ice_isA",
"ice_ping",
"refreshSession"
};
public boolean _iceDispatch(IceInternal.Incoming in, Ice.Current current)
throws Ice.UserException
{
int pos = java.util.Arrays.binarySearch(_all, current.operation);
if(pos < 0)
{
throw new Ice.OperationNotExistException(current.id, current.facet, current.operation);
}
switch(pos)
{
case 0:
{
return Ice._RouterDisp._iceD_addProxies(this, in, current);
}
case 1:
{
return _iceD_createSession(this, in, current);
}
case 2:
{
return _iceD_createSessionFromSecureConnection(this, in, current);
}
case 3:
{
return _iceD_destroySession(this, in, current);
}
case 4:
{
return _iceD_getACMTimeout(this, in, current);
}
case 5:
{
return _iceD_getCategoryForClient(this, in, current);
}
case 6:
{
return Ice._RouterDisp._iceD_getClientProxy(this, in, current);
}
case 7:
{
return Ice._RouterDisp._iceD_getServerProxy(this, in, current);
}
case 8:
{
return _iceD_getSessionTimeout(this, in, current);
}
case 9:
{
return _iceD_ice_id(this, in, current);
}
case 10:
{
return _iceD_ice_ids(this, in, current);
}
case 11:
{
return _iceD_ice_isA(this, in, current);
}
case 12:
{
return _iceD_ice_ping(this, in, current);
}
case 13:
{
return _iceD_refreshSession(this, in, current);
}
}
assert(false);
throw new Ice.OperationNotExistException(current.id, current.facet, current.operation);
}
protected void _iceWriteImpl(Ice.OutputStream ostr_)
{
ostr_.startSlice(ice_staticId(), -1, true);
ostr_.endSlice();
}
protected void _iceReadImpl(Ice.InputStream istr_)
{
istr_.startSlice();
istr_.endSlice();
}
public static final long serialVersionUID = 0L;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy