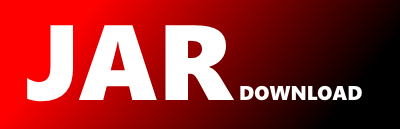
IceMX.MetricsAdminPrx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ice Show documentation
Show all versions of ice Show documentation
Ice is a comprehensive RPC framework that helps you build distributed applications with minimal effort using familiar object-oriented idioms
// **********************************************************************
//
// Copyright (c) 2003-2017 ZeroC, Inc. All rights reserved.
//
// This copy of Ice is licensed to you under the terms described in the
// ICE_LICENSE file included in this distribution.
//
// **********************************************************************
//
// Ice version 3.6.4
//
//
//
// Generated from file `Metrics.ice'
//
// Warning: do not edit this file.
//
//
//
package IceMX;
/**
* The metrics administrative facet interface. This interface allows
* remote administrative clients to access metrics of an application
* that enabled the Ice administrative facility and configured some
* metrics views.
*
**/
public interface MetricsAdminPrx extends Ice.ObjectPrx
{
/**
* Get the names of enabled and disabled metrics.
*
* @param disabledViews The names of the disabled views.
*
* @return The name of the enabled views.
*
**/
public String[] getMetricsViewNames(Ice.StringSeqHolder disabledViews);
/**
* Get the names of enabled and disabled metrics.
*
* @param disabledViews The names of the disabled views.
*
* @param __ctx The Context map to send with the invocation.
* @return The name of the enabled views.
*
**/
public String[] getMetricsViewNames(Ice.StringSeqHolder disabledViews, java.util.Map __ctx);
/**
* Get the names of enabled and disabled metrics.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames();
/**
* Get the names of enabled and disabled metrics.
*
* @param __ctx The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(java.util.Map __ctx);
/**
* Get the names of enabled and disabled metrics.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(Ice.Callback __cb);
/**
* Get the names of enabled and disabled metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(java.util.Map __ctx, Ice.Callback __cb);
/**
* Get the names of enabled and disabled metrics.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(Callback_MetricsAdmin_getMetricsViewNames __cb);
/**
* Get the names of enabled and disabled metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(java.util.Map __ctx, Callback_MetricsAdmin_getMetricsViewNames __cb);
public interface FunctionalCallback_MetricsAdmin_getMetricsViewNames_Response
{
void apply(String[] __ret, String[] disabledViews);
}
/**
* Get the names of enabled and disabled metrics.
*
* @param __responseCb The lambda response callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(FunctionalCallback_MetricsAdmin_getMetricsViewNames_Response __responseCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the names of enabled and disabled metrics.
*
* @param __responseCb The lambda response callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(FunctionalCallback_MetricsAdmin_getMetricsViewNames_Response __responseCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the names of enabled and disabled metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(java.util.Map __ctx,
FunctionalCallback_MetricsAdmin_getMetricsViewNames_Response __responseCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the names of enabled and disabled metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsViewNames(java.util.Map __ctx,
FunctionalCallback_MetricsAdmin_getMetricsViewNames_Response __responseCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the names of enabled and disabled metrics.
*
* @param disabledViews The names of the disabled views.
*
* @param __result The asynchronous result object.
* @return The name of the enabled views.
*
**/
public String[] end_getMetricsViewNames(Ice.StringSeqHolder disabledViews, Ice.AsyncResult __result);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public void enableMetricsView(String name)
throws UnknownMetricsView;
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
* @param __ctx The Context map to send with the invocation.
**/
public void enableMetricsView(String name, java.util.Map __ctx)
throws UnknownMetricsView;
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name, java.util.Map __ctx);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name, Ice.Callback __cb);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name, java.util.Map __ctx, Ice.Callback __cb);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name, Callback_MetricsAdmin_enableMetricsView __cb);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name, java.util.Map __ctx, Callback_MetricsAdmin_enableMetricsView __cb);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name,
java.util.Map __ctx,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Enables a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_enableMetricsView(String name,
java.util.Map __ctx,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Enables a metrics view.
*
* @param __result The asynchronous result object.
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public void end_enableMetricsView(Ice.AsyncResult __result)
throws UnknownMetricsView;
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public void disableMetricsView(String name)
throws UnknownMetricsView;
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
* @param __ctx The Context map to send with the invocation.
**/
public void disableMetricsView(String name, java.util.Map __ctx)
throws UnknownMetricsView;
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name, java.util.Map __ctx);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name, Ice.Callback __cb);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name, java.util.Map __ctx, Ice.Callback __cb);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name, Callback_MetricsAdmin_disableMetricsView __cb);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name, java.util.Map __ctx, Callback_MetricsAdmin_disableMetricsView __cb);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name,
java.util.Map __ctx,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Disable a metrics view.
*
* @param name The metrics view name.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_disableMetricsView(String name,
java.util.Map __ctx,
IceInternal.Functional_VoidCallback __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Disable a metrics view.
*
* @param __result The asynchronous result object.
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public void end_disableMetricsView(Ice.AsyncResult __result)
throws UnknownMetricsView;
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param timestamp The local time of the process when the metrics
* object were retrieved.
*
* @return The metrics view data.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public java.util.Map getMetricsView(String view, Ice.LongHolder timestamp)
throws UnknownMetricsView;
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param timestamp The local time of the process when the metrics
* object were retrieved.
*
* @param __ctx The Context map to send with the invocation.
* @return The metrics view data.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public java.util.Map getMetricsView(String view, Ice.LongHolder timestamp, java.util.Map __ctx)
throws UnknownMetricsView;
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __ctx The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view, java.util.Map __ctx);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view, Ice.Callback __cb);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view, java.util.Map __ctx, Ice.Callback __cb);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view, Callback_MetricsAdmin_getMetricsView __cb);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view, java.util.Map __ctx, Callback_MetricsAdmin_getMetricsView __cb);
public interface FunctionalCallback_MetricsAdmin_getMetricsView_Response
{
void apply(java.util.Map __ret, long timestamp);
}
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view,
FunctionalCallback_MetricsAdmin_getMetricsView_Response __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view,
FunctionalCallback_MetricsAdmin_getMetricsView_Response __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view,
java.util.Map __ctx,
FunctionalCallback_MetricsAdmin_getMetricsView_Response __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param view The name of the metrics view.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsView(String view,
java.util.Map __ctx,
FunctionalCallback_MetricsAdmin_getMetricsView_Response __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
*
* @param timestamp The local time of the process when the metrics
* object were retrieved.
*
* @param __result The asynchronous result object.
* @return The metrics view data.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public java.util.Map end_getMetricsView(Ice.LongHolder timestamp, Ice.AsyncResult __result)
throws UnknownMetricsView;
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @return The metrics failures associated with the map.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public MetricsFailures[] getMapMetricsFailures(String view, String map)
throws UnknownMetricsView;
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __ctx The Context map to send with the invocation.
* @return The metrics failures associated with the map.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public MetricsFailures[] getMapMetricsFailures(String view, String map, java.util.Map __ctx)
throws UnknownMetricsView;
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view, String map);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __ctx The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view, String map, java.util.Map __ctx);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view, String map, Ice.Callback __cb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view, String map, java.util.Map __ctx, Ice.Callback __cb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view, String map, Callback_MetricsAdmin_getMapMetricsFailures __cb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view, String map, java.util.Map __ctx, Callback_MetricsAdmin_getMapMetricsFailures __cb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view,
String map,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view,
String map,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view,
String map,
java.util.Map __ctx,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMapMetricsFailures(String view,
String map,
java.util.Map __ctx,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the metrics failures associated with the given view and map.
*
* @param __result The asynchronous result object.
* @return The metrics failures associated with the map.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public MetricsFailures[] end_getMapMetricsFailures(Ice.AsyncResult __result)
throws UnknownMetricsView;
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @return The metrics failures associated with the metrics.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public MetricsFailures getMetricsFailures(String view, String map, String id)
throws UnknownMetricsView;
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __ctx The Context map to send with the invocation.
* @return The metrics failures associated with the metrics.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public MetricsFailures getMetricsFailures(String view, String map, String id, java.util.Map __ctx)
throws UnknownMetricsView;
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view, String map, String id);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __ctx The Context map to send with the invocation.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view, String map, String id, java.util.Map __ctx);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view, String map, String id, Ice.Callback __cb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view, String map, String id, java.util.Map __ctx, Ice.Callback __cb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view, String map, String id, Callback_MetricsAdmin_getMetricsFailures __cb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __cb The asynchronous callback object.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view, String map, String id, java.util.Map __ctx, Callback_MetricsAdmin_getMetricsFailures __cb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view,
String map,
String id,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view,
String map,
String id,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view,
String map,
String id,
java.util.Map __ctx,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param view The name of the metrics view.
*
* @param map The name of the metrics map.
*
* @param id The ID of the metrics.
*
* @param __ctx The Context map to send with the invocation.
* @param __responseCb The lambda response callback.
* @param __userExceptionCb The lambda user exception callback.
* @param __exceptionCb The lambda exception callback.
* @param __sentCb The lambda sent callback.
* @return The asynchronous result object.
**/
public Ice.AsyncResult begin_getMetricsFailures(String view,
String map,
String id,
java.util.Map __ctx,
IceInternal.Functional_GenericCallback1 __responseCb,
IceInternal.Functional_GenericCallback1 __userExceptionCb,
IceInternal.Functional_GenericCallback1 __exceptionCb,
IceInternal.Functional_BoolCallback __sentCb);
/**
* Get the metrics failure associated for the given metrics.
*
* @param __result The asynchronous result object.
* @return The metrics failures associated with the metrics.
*
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
*
**/
public MetricsFailures end_getMetricsFailures(Ice.AsyncResult __result)
throws UnknownMetricsView;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy