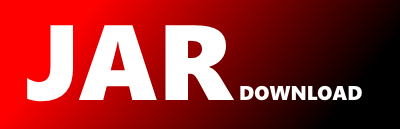
com.zeroc.Ice.BlobjectAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ice Show documentation
Show all versions of ice Show documentation
Ice is a comprehensive RPC framework that helps you build distributed applications with minimal effort using familiar object-oriented idioms
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
package com.zeroc.Ice;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.CompletableFuture;
/**
* BlobjectAsync
is the base class for asynchronous dynamic
* dispatch servants. A server application derives a concrete servant
* class that implements the {@link BlobjectAsync#ice_invokeAsync} method,
* which is called by the Ice run time to deliver every request on this
* object.
**/
public interface BlobjectAsync extends com.zeroc.Ice.Object
{
/**
* Dispatch an incoming request.
*
* @param inEncaps The encoded input parameters.
* @param current The Current object, which provides important information
* about the request, such as the identity of the target object and the
* name of the operation.
* @return A completion stage that eventually completes with the result of
* the invocation, an instance of Ice_invokeResult
.
* If the operation completed successfully, set the returnValue
* member to true
and the outParams
member to
* the encoded results. If the operation raises a user exception, you can
* throw it directly from ice_invokeAsync
, or complete the
* future by setting the returnValue
member to
* false
and the outParams
member to the encoded
* user exception.
* @throws UserException A user exception raised by this method will be marshaled
* as the result of the invocation.
**/
CompletionStage ice_invokeAsync(byte[] inEncaps, Current current)
throws UserException;
/** @hidden */
@Override
default CompletionStage _iceDispatch(com.zeroc.IceInternal.Incoming in, Current current)
throws UserException
{
byte[] inEncaps = in.readParamEncaps();
CompletableFuture f = new CompletableFuture<>();
CompletionStage s = ice_invokeAsync(inEncaps, current);
final OutputStream cached = in.getAndClearCachedOutputStream(); // If an output stream is cached, re-use it
s.whenComplete((result, ex) ->
{
if(ex != null)
{
f.completeExceptionally(ex);
}
else
{
f.complete(in.writeParamEncaps(cached, result.outParams, result.returnValue));
}
});
return f;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy