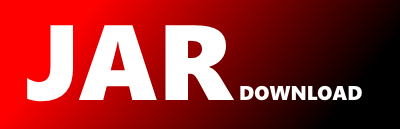
com.zeroc.Ice.LoggerAdmin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ice Show documentation
Show all versions of ice Show documentation
Ice is a comprehensive RPC framework that helps you build distributed applications with minimal effort using familiar object-oriented idioms
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
//
// Ice version 3.7.8
//
//
//
// Generated from file `RemoteLogger.ice'
//
// Warning: do not edit this file.
//
//
//
package com.zeroc.Ice;
/**
* The interface of the admin object that allows an Ice application the attach its
* {@link RemoteLogger} to the {@link Logger} of this admin object's Ice communicator.
**/
public interface LoggerAdmin extends Object
{
/**
* Holds the result of operation getLog.
**/
public static class GetLogResult
{
/**
* Default constructor.
**/
public GetLogResult()
{
}
/**
* This constructor makes shallow copies of the results for operation GetLog.
* @param returnValue The Log messages.
* @param prefix The prefix of the associated local logger.
**/
public GetLogResult(LogMessage[] returnValue, String prefix)
{
this.returnValue = returnValue;
this.prefix = prefix;
}
/**
* The Log messages.
**/
public LogMessage[] returnValue;
/**
* The prefix of the associated local logger.
**/
public String prefix;
public void write(OutputStream ostr)
{
ostr.writeString(this.prefix);
LogMessageSeqHelper.write(ostr, returnValue);
}
public void read(InputStream istr)
{
this.prefix = istr.readString();
returnValue = LogMessageSeqHelper.read(istr);
}
}
/**
* Attaches a RemoteLogger object to the local logger.
* attachRemoteLogger calls init on the provided RemoteLogger proxy.
* @param prx A proxy to the remote logger.
* @param messageTypes The list of message types that the remote logger wishes to receive.
* An empty list means no filtering (send all message types).
* @param traceCategories The categories of traces that the remote logger wishes to receive.
* This parameter is ignored if messageTypes is not empty and does not include trace.
* An empty list means no filtering (send all trace categories).
* @param messageMax The maximum number of log messages (of all types) to be provided
* to init. A negative value requests all messages available.
* @param current The Current object for the invocation.
* @throws RemoteLoggerAlreadyAttachedException Raised if this remote logger is already
* attached to this admin object.
**/
void attachRemoteLogger(RemoteLoggerPrx prx, LogMessageType[] messageTypes, String[] traceCategories, int messageMax, Current current)
throws RemoteLoggerAlreadyAttachedException;
/**
* Detaches a RemoteLogger object from the local logger.
* @param prx A proxy to the remote logger.
* @param current The Current object for the invocation.
* @return True if the provided remote logger proxy was detached, and false otherwise.
**/
boolean detachRemoteLogger(RemoteLoggerPrx prx, Current current);
/**
* Retrieves log messages recently logged.
* @param messageTypes The list of message types that the caller wishes to receive.
* An empty list means no filtering (send all message types).
* @param traceCategories The categories of traces that caller wish to receive.
* This parameter is ignored if messageTypes is not empty and does not include trace.
* An empty list means no filtering (send all trace categories).
* @param messageMax The maximum number of log messages (of all types) to be returned.
* A negative value requests all messages available.
* @param current The Current object for the invocation.
* @return An instance of LoggerAdmin.GetLogResult.
**/
LoggerAdmin.GetLogResult getLog(LogMessageType[] messageTypes, String[] traceCategories, int messageMax, Current current);
/** @hidden */
static final String[] _iceIds =
{
"::Ice::LoggerAdmin",
"::Ice::Object"
};
@Override
default String[] ice_ids(Current current)
{
return _iceIds;
}
@Override
default String ice_id(Current current)
{
return ice_staticId();
}
static String ice_staticId()
{
return "::Ice::LoggerAdmin";
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
* @throws UserException -
**/
static java.util.concurrent.CompletionStage _iceD_attachRemoteLogger(LoggerAdmin obj, final com.zeroc.IceInternal.Incoming inS, Current current)
throws UserException
{
Object._iceCheckMode(null, current.mode);
InputStream istr = inS.startReadParams();
RemoteLoggerPrx iceP_prx;
LogMessageType[] iceP_messageTypes;
String[] iceP_traceCategories;
int iceP_messageMax;
iceP_prx = RemoteLoggerPrx.uncheckedCast(istr.readProxy());
iceP_messageTypes = LogMessageTypeSeqHelper.read(istr);
iceP_traceCategories = istr.readStringSeq();
iceP_messageMax = istr.readInt();
inS.endReadParams();
obj.attachRemoteLogger(iceP_prx, iceP_messageTypes, iceP_traceCategories, iceP_messageMax, current);
return inS.setResult(inS.writeEmptyParams());
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
**/
static java.util.concurrent.CompletionStage _iceD_detachRemoteLogger(LoggerAdmin obj, final com.zeroc.IceInternal.Incoming inS, Current current)
{
Object._iceCheckMode(null, current.mode);
InputStream istr = inS.startReadParams();
RemoteLoggerPrx iceP_prx;
iceP_prx = RemoteLoggerPrx.uncheckedCast(istr.readProxy());
inS.endReadParams();
boolean ret = obj.detachRemoteLogger(iceP_prx, current);
OutputStream ostr = inS.startWriteParams();
ostr.writeBool(ret);
inS.endWriteParams(ostr);
return inS.setResult(ostr);
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
**/
static java.util.concurrent.CompletionStage _iceD_getLog(LoggerAdmin obj, final com.zeroc.IceInternal.Incoming inS, Current current)
{
Object._iceCheckMode(null, current.mode);
InputStream istr = inS.startReadParams();
LogMessageType[] iceP_messageTypes;
String[] iceP_traceCategories;
int iceP_messageMax;
iceP_messageTypes = LogMessageTypeSeqHelper.read(istr);
iceP_traceCategories = istr.readStringSeq();
iceP_messageMax = istr.readInt();
inS.endReadParams();
LoggerAdmin.GetLogResult ret = obj.getLog(iceP_messageTypes, iceP_traceCategories, iceP_messageMax, current);
OutputStream ostr = inS.startWriteParams();
ret.write(ostr);
inS.endWriteParams(ostr);
return inS.setResult(ostr);
}
/** @hidden */
final static String[] _iceOps =
{
"attachRemoteLogger",
"detachRemoteLogger",
"getLog",
"ice_id",
"ice_ids",
"ice_isA",
"ice_ping"
};
/** @hidden */
@Override
default java.util.concurrent.CompletionStage _iceDispatch(com.zeroc.IceInternal.Incoming in, Current current)
throws UserException
{
int pos = java.util.Arrays.binarySearch(_iceOps, current.operation);
if(pos < 0)
{
throw new OperationNotExistException(current.id, current.facet, current.operation);
}
switch(pos)
{
case 0:
{
return _iceD_attachRemoteLogger(this, in, current);
}
case 1:
{
return _iceD_detachRemoteLogger(this, in, current);
}
case 2:
{
return _iceD_getLog(this, in, current);
}
case 3:
{
return Object._iceD_ice_id(this, in, current);
}
case 4:
{
return Object._iceD_ice_ids(this, in, current);
}
case 5:
{
return Object._iceD_ice_isA(this, in, current);
}
case 6:
{
return Object._iceD_ice_ping(this, in, current);
}
}
assert(false);
throw new OperationNotExistException(current.id, current.facet, current.operation);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy