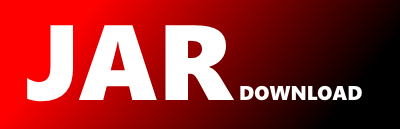
com.zeroc.Ice.ProcessPrx Maven / Gradle / Ivy
Show all versions of ice Show documentation
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
//
// Ice version 3.7.8
//
//
//
// Generated from file `Process.ice'
//
// Warning: do not edit this file.
//
//
//
package com.zeroc.Ice;
/**
* An administrative interface for process management. Managed servers must
* implement this interface.
*
* A servant implementing this interface is a potential target
* for denial-of-service attacks, therefore proper security precautions
* should be taken. For example, the servant can use a UUID to make its
* identity harder to guess, and be registered in an object adapter with
* a secured endpoint.
**/
public interface ProcessPrx extends ObjectPrx
{
/**
* Initiate a graceful shut-down.
*
* @see Communicator#shutdown
**/
default void shutdown()
{
shutdown(com.zeroc.Ice.ObjectPrx.noExplicitContext);
}
/**
* Initiate a graceful shut-down.
* @param context The Context map to send with the invocation.
*
* @see Communicator#shutdown
**/
default void shutdown(java.util.Map context)
{
_iceI_shutdownAsync(context, true).waitForResponse();
}
/**
* Initiate a graceful shut-down.
* @return A future that will be completed when the invocation completes.
*
* @see Communicator#shutdown
**/
default java.util.concurrent.CompletableFuture shutdownAsync()
{
return _iceI_shutdownAsync(com.zeroc.Ice.ObjectPrx.noExplicitContext, false);
}
/**
* Initiate a graceful shut-down.
* @param context The Context map to send with the invocation.
* @return A future that will be completed when the invocation completes.
*
* @see Communicator#shutdown
**/
default java.util.concurrent.CompletableFuture shutdownAsync(java.util.Map context)
{
return _iceI_shutdownAsync(context, false);
}
/**
* @hidden
* @param context -
* @param sync -
* @return -
**/
default com.zeroc.IceInternal.OutgoingAsync _iceI_shutdownAsync(java.util.Map context, boolean sync)
{
com.zeroc.IceInternal.OutgoingAsync f = new com.zeroc.IceInternal.OutgoingAsync<>(this, "shutdown", null, sync, null);
f.invoke(false, context, null, null, null);
return f;
}
/**
* Write a message on the process' stdout or stderr.
* @param message The message.
* @param fd 1 for stdout, 2 for stderr.
**/
default void writeMessage(String message, int fd)
{
writeMessage(message, fd, com.zeroc.Ice.ObjectPrx.noExplicitContext);
}
/**
* Write a message on the process' stdout or stderr.
* @param message The message.
* @param fd 1 for stdout, 2 for stderr.
* @param context The Context map to send with the invocation.
**/
default void writeMessage(String message, int fd, java.util.Map context)
{
_iceI_writeMessageAsync(message, fd, context, true).waitForResponse();
}
/**
* Write a message on the process' stdout or stderr.
* @param message The message.
* @param fd 1 for stdout, 2 for stderr.
* @return A future that will be completed when the invocation completes.
**/
default java.util.concurrent.CompletableFuture writeMessageAsync(String message, int fd)
{
return _iceI_writeMessageAsync(message, fd, com.zeroc.Ice.ObjectPrx.noExplicitContext, false);
}
/**
* Write a message on the process' stdout or stderr.
* @param message The message.
* @param fd 1 for stdout, 2 for stderr.
* @param context The Context map to send with the invocation.
* @return A future that will be completed when the invocation completes.
**/
default java.util.concurrent.CompletableFuture writeMessageAsync(String message, int fd, java.util.Map context)
{
return _iceI_writeMessageAsync(message, fd, context, false);
}
/**
* @hidden
* @param iceP_message -
* @param iceP_fd -
* @param context -
* @param sync -
* @return -
**/
default com.zeroc.IceInternal.OutgoingAsync _iceI_writeMessageAsync(String iceP_message, int iceP_fd, java.util.Map context, boolean sync)
{
com.zeroc.IceInternal.OutgoingAsync f = new com.zeroc.IceInternal.OutgoingAsync<>(this, "writeMessage", null, sync, null);
f.invoke(false, context, null, ostr -> {
ostr.writeString(iceP_message);
ostr.writeInt(iceP_fd);
}, null);
return f;
}
/**
* Contacts the remote server to verify that the object implements this type.
* Raises a local exception if a communication error occurs.
* @param obj The untyped proxy.
* @return A proxy for this type, or null if the object does not support this type.
**/
static ProcessPrx checkedCast(ObjectPrx obj)
{
return ObjectPrx._checkedCast(obj, ice_staticId(), ProcessPrx.class, _ProcessPrxI.class);
}
/**
* Contacts the remote server to verify that the object implements this type.
* Raises a local exception if a communication error occurs.
* @param obj The untyped proxy.
* @param context The Context map to send with the invocation.
* @return A proxy for this type, or null if the object does not support this type.
**/
static ProcessPrx checkedCast(ObjectPrx obj, java.util.Map context)
{
return ObjectPrx._checkedCast(obj, context, ice_staticId(), ProcessPrx.class, _ProcessPrxI.class);
}
/**
* Contacts the remote server to verify that a facet of the object implements this type.
* Raises a local exception if a communication error occurs.
* @param obj The untyped proxy.
* @param facet The name of the desired facet.
* @return A proxy for this type, or null if the object does not support this type.
**/
static ProcessPrx checkedCast(ObjectPrx obj, String facet)
{
return ObjectPrx._checkedCast(obj, facet, ice_staticId(), ProcessPrx.class, _ProcessPrxI.class);
}
/**
* Contacts the remote server to verify that a facet of the object implements this type.
* Raises a local exception if a communication error occurs.
* @param obj The untyped proxy.
* @param facet The name of the desired facet.
* @param context The Context map to send with the invocation.
* @return A proxy for this type, or null if the object does not support this type.
**/
static ProcessPrx checkedCast(ObjectPrx obj, String facet, java.util.Map context)
{
return ObjectPrx._checkedCast(obj, facet, context, ice_staticId(), ProcessPrx.class, _ProcessPrxI.class);
}
/**
* Downcasts the given proxy to this type without contacting the remote server.
* @param obj The untyped proxy.
* @return A proxy for this type.
**/
static ProcessPrx uncheckedCast(ObjectPrx obj)
{
return ObjectPrx._uncheckedCast(obj, ProcessPrx.class, _ProcessPrxI.class);
}
/**
* Downcasts the given proxy to this type without contacting the remote server.
* @param obj The untyped proxy.
* @param facet The name of the desired facet.
* @return A proxy for this type.
**/
static ProcessPrx uncheckedCast(ObjectPrx obj, String facet)
{
return ObjectPrx._uncheckedCast(obj, facet, ProcessPrx.class, _ProcessPrxI.class);
}
/**
* Returns a proxy that is identical to this proxy, except for the per-proxy context.
* @param newContext The context for the new proxy.
* @return A proxy with the specified per-proxy context.
**/
@Override
default ProcessPrx ice_context(java.util.Map newContext)
{
return (ProcessPrx)_ice_context(newContext);
}
/**
* Returns a proxy that is identical to this proxy, except for the adapter ID.
* @param newAdapterId The adapter ID for the new proxy.
* @return A proxy with the specified adapter ID.
**/
@Override
default ProcessPrx ice_adapterId(String newAdapterId)
{
return (ProcessPrx)_ice_adapterId(newAdapterId);
}
/**
* Returns a proxy that is identical to this proxy, except for the endpoints.
* @param newEndpoints The endpoints for the new proxy.
* @return A proxy with the specified endpoints.
**/
@Override
default ProcessPrx ice_endpoints(Endpoint[] newEndpoints)
{
return (ProcessPrx)_ice_endpoints(newEndpoints);
}
/**
* Returns a proxy that is identical to this proxy, except for the locator cache timeout.
* @param newTimeout The new locator cache timeout (in seconds).
* @return A proxy with the specified locator cache timeout.
**/
@Override
default ProcessPrx ice_locatorCacheTimeout(int newTimeout)
{
return (ProcessPrx)_ice_locatorCacheTimeout(newTimeout);
}
/**
* Returns a proxy that is identical to this proxy, except for the invocation timeout.
* @param newTimeout The new invocation timeout (in seconds).
* @return A proxy with the specified invocation timeout.
**/
@Override
default ProcessPrx ice_invocationTimeout(int newTimeout)
{
return (ProcessPrx)_ice_invocationTimeout(newTimeout);
}
/**
* Returns a proxy that is identical to this proxy, except for connection caching.
* @param newCache true
if the new proxy should cache connections; false
otherwise.
* @return A proxy with the specified caching policy.
**/
@Override
default ProcessPrx ice_connectionCached(boolean newCache)
{
return (ProcessPrx)_ice_connectionCached(newCache);
}
/**
* Returns a proxy that is identical to this proxy, except for the endpoint selection policy.
* @param newType The new endpoint selection policy.
* @return A proxy with the specified endpoint selection policy.
**/
@Override
default ProcessPrx ice_endpointSelection(EndpointSelectionType newType)
{
return (ProcessPrx)_ice_endpointSelection(newType);
}
/**
* Returns a proxy that is identical to this proxy, except for how it selects endpoints.
* @param b If b
is true
, only endpoints that use a secure transport are
* used by the new proxy. If b
is false, the returned proxy uses both secure and
* insecure endpoints.
* @return A proxy with the specified selection policy.
**/
@Override
default ProcessPrx ice_secure(boolean b)
{
return (ProcessPrx)_ice_secure(b);
}
/**
* Returns a proxy that is identical to this proxy, except for the encoding used to marshal parameters.
* @param e The encoding version to use to marshal request parameters.
* @return A proxy with the specified encoding version.
**/
@Override
default ProcessPrx ice_encodingVersion(EncodingVersion e)
{
return (ProcessPrx)_ice_encodingVersion(e);
}
/**
* Returns a proxy that is identical to this proxy, except for its endpoint selection policy.
* @param b If b
is true
, the new proxy will use secure endpoints for invocations
* and only use insecure endpoints if an invocation cannot be made via secure endpoints. If b
is
* false
, the proxy prefers insecure endpoints to secure ones.
* @return A proxy with the specified selection policy.
**/
@Override
default ProcessPrx ice_preferSecure(boolean b)
{
return (ProcessPrx)_ice_preferSecure(b);
}
/**
* Returns a proxy that is identical to this proxy, except for the router.
* @param router The router for the new proxy.
* @return A proxy with the specified router.
**/
@Override
default ProcessPrx ice_router(RouterPrx router)
{
return (ProcessPrx)_ice_router(router);
}
/**
* Returns a proxy that is identical to this proxy, except for the locator.
* @param locator The locator for the new proxy.
* @return A proxy with the specified locator.
**/
@Override
default ProcessPrx ice_locator(LocatorPrx locator)
{
return (ProcessPrx)_ice_locator(locator);
}
/**
* Returns a proxy that is identical to this proxy, except for collocation optimization.
* @param b true
if the new proxy enables collocation optimization; false
otherwise.
* @return A proxy with the specified collocation optimization.
**/
@Override
default ProcessPrx ice_collocationOptimized(boolean b)
{
return (ProcessPrx)_ice_collocationOptimized(b);
}
/**
* Returns a proxy that is identical to this proxy, but uses twoway invocations.
* @return A proxy that uses twoway invocations.
**/
@Override
default ProcessPrx ice_twoway()
{
return (ProcessPrx)_ice_twoway();
}
/**
* Returns a proxy that is identical to this proxy, but uses oneway invocations.
* @return A proxy that uses oneway invocations.
**/
@Override
default ProcessPrx ice_oneway()
{
return (ProcessPrx)_ice_oneway();
}
/**
* Returns a proxy that is identical to this proxy, but uses batch oneway invocations.
* @return A proxy that uses batch oneway invocations.
**/
@Override
default ProcessPrx ice_batchOneway()
{
return (ProcessPrx)_ice_batchOneway();
}
/**
* Returns a proxy that is identical to this proxy, but uses datagram invocations.
* @return A proxy that uses datagram invocations.
**/
@Override
default ProcessPrx ice_datagram()
{
return (ProcessPrx)_ice_datagram();
}
/**
* Returns a proxy that is identical to this proxy, but uses batch datagram invocations.
* @return A proxy that uses batch datagram invocations.
**/
@Override
default ProcessPrx ice_batchDatagram()
{
return (ProcessPrx)_ice_batchDatagram();
}
/**
* Returns a proxy that is identical to this proxy, except for compression.
* @param co true
enables compression for the new proxy; false
disables compression.
* @return A proxy with the specified compression setting.
**/
@Override
default ProcessPrx ice_compress(boolean co)
{
return (ProcessPrx)_ice_compress(co);
}
/**
* Returns a proxy that is identical to this proxy, except for its connection timeout setting.
* @param t The connection timeout for the proxy in milliseconds.
* @return A proxy with the specified timeout.
**/
@Override
default ProcessPrx ice_timeout(int t)
{
return (ProcessPrx)_ice_timeout(t);
}
/**
* Returns a proxy that is identical to this proxy, except for its connection ID.
* @param connectionId The connection ID for the new proxy. An empty string removes the connection ID.
* @return A proxy with the specified connection ID.
**/
@Override
default ProcessPrx ice_connectionId(String connectionId)
{
return (ProcessPrx)_ice_connectionId(connectionId);
}
/**
* Returns a proxy that is identical to this proxy, except it's a fixed proxy bound
* the given connection.@param connection The fixed proxy connection.
* @return A fixed proxy bound to the given connection.
**/
@Override
default ProcessPrx ice_fixed(com.zeroc.Ice.Connection connection)
{
return (ProcessPrx)_ice_fixed(connection);
}
static String ice_staticId()
{
return "::Ice::Process";
}
}