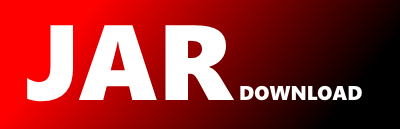
com.zeroc.IceMX.MetricsAdmin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ice Show documentation
Show all versions of ice Show documentation
Ice is a comprehensive RPC framework that helps you build distributed applications with minimal effort using familiar object-oriented idioms
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
//
// Ice version 3.7.8
//
//
//
// Generated from file `Metrics.ice'
//
// Warning: do not edit this file.
//
//
//
package com.zeroc.IceMX;
/**
* The metrics administrative facet interface. This interface allows
* remote administrative clients to access metrics of an application
* that enabled the Ice administrative facility and configured some
* metrics views.
**/
public interface MetricsAdmin extends com.zeroc.Ice.Object
{
/**
* Holds the result of operation getMetricsViewNames.
**/
public static class GetMetricsViewNamesResult
{
/**
* Default constructor.
**/
public GetMetricsViewNamesResult()
{
}
/**
* This constructor makes shallow copies of the results for operation GetMetricsViewNames.
* @param returnValue The name of the enabled views.
* @param disabledViews The names of the disabled views.
**/
public GetMetricsViewNamesResult(String[] returnValue, String[] disabledViews)
{
this.returnValue = returnValue;
this.disabledViews = disabledViews;
}
/**
* The name of the enabled views.
**/
public String[] returnValue;
/**
* The names of the disabled views.
**/
public String[] disabledViews;
public void write(com.zeroc.Ice.OutputStream ostr)
{
ostr.writeStringSeq(this.disabledViews);
ostr.writeStringSeq(returnValue);
}
public void read(com.zeroc.Ice.InputStream istr)
{
this.disabledViews = istr.readStringSeq();
returnValue = istr.readStringSeq();
}
}
/**
* Holds the result of operation getMetricsView.
**/
public static class GetMetricsViewResult
{
/**
* Default constructor.
**/
public GetMetricsViewResult()
{
}
/**
* This constructor makes shallow copies of the results for operation GetMetricsView.
* @param returnValue The metrics view data.
* @param timestamp The local time of the process when the metrics
* object were retrieved.
**/
public GetMetricsViewResult(java.util.Map returnValue, long timestamp)
{
this.returnValue = returnValue;
this.timestamp = timestamp;
}
/**
* The metrics view data.
**/
public java.util.Map returnValue;
/**
* The local time of the process when the metrics
* object were retrieved.
**/
public long timestamp;
public void write(com.zeroc.Ice.OutputStream ostr)
{
ostr.writeLong(this.timestamp);
MetricsViewHelper.write(ostr, returnValue);
}
public void read(com.zeroc.Ice.InputStream istr)
{
this.timestamp = istr.readLong();
returnValue = MetricsViewHelper.read(istr);
}
}
/**
* Get the names of enabled and disabled metrics.
* @param current The Current object for the invocation.
* @return An instance of MetricsAdmin.GetMetricsViewNamesResult.
**/
MetricsAdmin.GetMetricsViewNamesResult getMetricsViewNames(com.zeroc.Ice.Current current);
/**
* Enables a metrics view.
* @param name The metrics view name.
* @param current The Current object for the invocation.
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
**/
void enableMetricsView(String name, com.zeroc.Ice.Current current)
throws UnknownMetricsView;
/**
* Disable a metrics view.
* @param name The metrics view name.
* @param current The Current object for the invocation.
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
**/
void disableMetricsView(String name, com.zeroc.Ice.Current current)
throws UnknownMetricsView;
/**
* Get the metrics objects for the given metrics view. This
* returns a dictionnary of metric maps for each metrics class
* configured with the view. The timestamp allows the client to
* compute averages which are not dependent of the invocation
* latency for this operation.
* @param view The name of the metrics view.
* @param current The Current object for the invocation.
* @return An instance of MetricsAdmin.GetMetricsViewResult.
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
**/
MetricsAdmin.GetMetricsViewResult getMetricsView(String view, com.zeroc.Ice.Current current)
throws UnknownMetricsView;
/**
* Get the metrics failures associated with the given view and map.
* @param view The name of the metrics view.
* @param map The name of the metrics map.
* @param current The Current object for the invocation.
* @return The metrics failures associated with the map.
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
**/
MetricsFailures[] getMapMetricsFailures(String view, String map, com.zeroc.Ice.Current current)
throws UnknownMetricsView;
/**
* Get the metrics failure associated for the given metrics.
* @param view The name of the metrics view.
* @param map The name of the metrics map.
* @param id The ID of the metrics.
* @param current The Current object for the invocation.
* @return The metrics failures associated with the metrics.
* @throws UnknownMetricsView Raised if the metrics view cannot be
* found.
**/
MetricsFailures getMetricsFailures(String view, String map, String id, com.zeroc.Ice.Current current)
throws UnknownMetricsView;
/** @hidden */
static final String[] _iceIds =
{
"::Ice::Object",
"::IceMX::MetricsAdmin"
};
@Override
default String[] ice_ids(com.zeroc.Ice.Current current)
{
return _iceIds;
}
@Override
default String ice_id(com.zeroc.Ice.Current current)
{
return ice_staticId();
}
static String ice_staticId()
{
return "::IceMX::MetricsAdmin";
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
**/
static java.util.concurrent.CompletionStage _iceD_getMetricsViewNames(MetricsAdmin obj, final com.zeroc.IceInternal.Incoming inS, com.zeroc.Ice.Current current)
{
com.zeroc.Ice.Object._iceCheckMode(null, current.mode);
inS.readEmptyParams();
inS.setFormat(com.zeroc.Ice.FormatType.SlicedFormat);
MetricsAdmin.GetMetricsViewNamesResult ret = obj.getMetricsViewNames(current);
com.zeroc.Ice.OutputStream ostr = inS.startWriteParams();
ret.write(ostr);
inS.endWriteParams(ostr);
return inS.setResult(ostr);
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
* @throws com.zeroc.Ice.UserException -
**/
static java.util.concurrent.CompletionStage _iceD_enableMetricsView(MetricsAdmin obj, final com.zeroc.IceInternal.Incoming inS, com.zeroc.Ice.Current current)
throws com.zeroc.Ice.UserException
{
com.zeroc.Ice.Object._iceCheckMode(null, current.mode);
com.zeroc.Ice.InputStream istr = inS.startReadParams();
String iceP_name;
iceP_name = istr.readString();
inS.endReadParams();
inS.setFormat(com.zeroc.Ice.FormatType.SlicedFormat);
obj.enableMetricsView(iceP_name, current);
return inS.setResult(inS.writeEmptyParams());
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
* @throws com.zeroc.Ice.UserException -
**/
static java.util.concurrent.CompletionStage _iceD_disableMetricsView(MetricsAdmin obj, final com.zeroc.IceInternal.Incoming inS, com.zeroc.Ice.Current current)
throws com.zeroc.Ice.UserException
{
com.zeroc.Ice.Object._iceCheckMode(null, current.mode);
com.zeroc.Ice.InputStream istr = inS.startReadParams();
String iceP_name;
iceP_name = istr.readString();
inS.endReadParams();
inS.setFormat(com.zeroc.Ice.FormatType.SlicedFormat);
obj.disableMetricsView(iceP_name, current);
return inS.setResult(inS.writeEmptyParams());
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
* @throws com.zeroc.Ice.UserException -
**/
static java.util.concurrent.CompletionStage _iceD_getMetricsView(MetricsAdmin obj, final com.zeroc.IceInternal.Incoming inS, com.zeroc.Ice.Current current)
throws com.zeroc.Ice.UserException
{
com.zeroc.Ice.Object._iceCheckMode(null, current.mode);
com.zeroc.Ice.InputStream istr = inS.startReadParams();
String iceP_view;
iceP_view = istr.readString();
inS.endReadParams();
inS.setFormat(com.zeroc.Ice.FormatType.SlicedFormat);
MetricsAdmin.GetMetricsViewResult ret = obj.getMetricsView(iceP_view, current);
com.zeroc.Ice.OutputStream ostr = inS.startWriteParams();
ret.write(ostr);
ostr.writePendingValues();
inS.endWriteParams(ostr);
return inS.setResult(ostr);
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
* @throws com.zeroc.Ice.UserException -
**/
static java.util.concurrent.CompletionStage _iceD_getMapMetricsFailures(MetricsAdmin obj, final com.zeroc.IceInternal.Incoming inS, com.zeroc.Ice.Current current)
throws com.zeroc.Ice.UserException
{
com.zeroc.Ice.Object._iceCheckMode(null, current.mode);
com.zeroc.Ice.InputStream istr = inS.startReadParams();
String iceP_view;
String iceP_map;
iceP_view = istr.readString();
iceP_map = istr.readString();
inS.endReadParams();
inS.setFormat(com.zeroc.Ice.FormatType.SlicedFormat);
MetricsFailures[] ret = obj.getMapMetricsFailures(iceP_view, iceP_map, current);
com.zeroc.Ice.OutputStream ostr = inS.startWriteParams();
MetricsFailuresSeqHelper.write(ostr, ret);
inS.endWriteParams(ostr);
return inS.setResult(ostr);
}
/**
* @hidden
* @param obj -
* @param inS -
* @param current -
* @return -
* @throws com.zeroc.Ice.UserException -
**/
static java.util.concurrent.CompletionStage _iceD_getMetricsFailures(MetricsAdmin obj, final com.zeroc.IceInternal.Incoming inS, com.zeroc.Ice.Current current)
throws com.zeroc.Ice.UserException
{
com.zeroc.Ice.Object._iceCheckMode(null, current.mode);
com.zeroc.Ice.InputStream istr = inS.startReadParams();
String iceP_view;
String iceP_map;
String iceP_id;
iceP_view = istr.readString();
iceP_map = istr.readString();
iceP_id = istr.readString();
inS.endReadParams();
inS.setFormat(com.zeroc.Ice.FormatType.SlicedFormat);
MetricsFailures ret = obj.getMetricsFailures(iceP_view, iceP_map, iceP_id, current);
com.zeroc.Ice.OutputStream ostr = inS.startWriteParams();
MetricsFailures.ice_write(ostr, ret);
inS.endWriteParams(ostr);
return inS.setResult(ostr);
}
/** @hidden */
final static String[] _iceOps =
{
"disableMetricsView",
"enableMetricsView",
"getMapMetricsFailures",
"getMetricsFailures",
"getMetricsView",
"getMetricsViewNames",
"ice_id",
"ice_ids",
"ice_isA",
"ice_ping"
};
/** @hidden */
@Override
default java.util.concurrent.CompletionStage _iceDispatch(com.zeroc.IceInternal.Incoming in, com.zeroc.Ice.Current current)
throws com.zeroc.Ice.UserException
{
int pos = java.util.Arrays.binarySearch(_iceOps, current.operation);
if(pos < 0)
{
throw new com.zeroc.Ice.OperationNotExistException(current.id, current.facet, current.operation);
}
switch(pos)
{
case 0:
{
return _iceD_disableMetricsView(this, in, current);
}
case 1:
{
return _iceD_enableMetricsView(this, in, current);
}
case 2:
{
return _iceD_getMapMetricsFailures(this, in, current);
}
case 3:
{
return _iceD_getMetricsFailures(this, in, current);
}
case 4:
{
return _iceD_getMetricsView(this, in, current);
}
case 5:
{
return _iceD_getMetricsViewNames(this, in, current);
}
case 6:
{
return com.zeroc.Ice.Object._iceD_ice_id(this, in, current);
}
case 7:
{
return com.zeroc.Ice.Object._iceD_ice_ids(this, in, current);
}
case 8:
{
return com.zeroc.Ice.Object._iceD_ice_isA(this, in, current);
}
case 9:
{
return com.zeroc.Ice.Object._iceD_ice_ping(this, in, current);
}
}
assert(false);
throw new com.zeroc.Ice.OperationNotExistException(current.id, current.facet, current.operation);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy