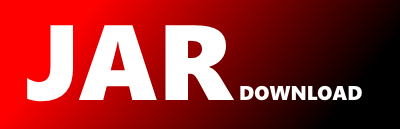
com.zeroc.IceMX.Observer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ice Show documentation
Show all versions of ice Show documentation
Ice is a comprehensive RPC framework that helps you build distributed applications with minimal effort using familiar object-oriented idioms
//
// Copyright (c) ZeroC, Inc. All rights reserved.
//
package com.zeroc.IceMX;
import com.zeroc.IceInternal.MetricsMap;
public class Observer extends com.zeroc.IceUtilInternal.StopWatch
implements com.zeroc.Ice.Instrumentation.Observer
{
public interface MetricsUpdate
{
void update(T m);
}
@Override
public void
attach()
{
if(!isStarted())
{
start();
}
}
@Override
public void
detach()
{
long lifetime = _previousDelay + stop();
for(MetricsMap.Entry e : _objects)
{
e.detach(lifetime);
}
}
@Override
public void
failed(String exceptionName)
{
for(MetricsMap.Entry e : _objects)
{
e.failed(exceptionName);
}
}
public void
forEach(MetricsUpdate u)
{
for(MetricsMap.Entry e : _objects)
{
e.execute(u);
}
}
public void
init(MetricsHelper helper, java.util.List.Entry> objects, Observer previous)
{
_objects = objects;
if(previous == null)
{
return;
}
_previousDelay = previous._previousDelay + previous.delay();
//
// Detach entries from previous observer which are no longer
// attached to this new observer.
//
for(MetricsMap.Entry p : previous._objects)
{
if(!_objects.contains(p))
{
p.detach(_previousDelay);
}
}
}
public > ObserverImpl
getObserver(String mapName, MetricsHelper helper, Class mcl, Class ocl)
{
java.util.List.Entry> metricsObjects = null;
for(MetricsMap.Entry entry : _objects)
{
MetricsMap.Entry e = entry.getMatching(mapName, helper, mcl);
if(e != null)
{
if(metricsObjects == null)
{
metricsObjects = new java.util.ArrayList<>(_objects.size());
}
metricsObjects.add(e);
}
}
if(metricsObjects == null)
{
return null;
}
try
{
ObserverImpl obsv = ocl.getDeclaredConstructor().newInstance();
obsv.init(helper, metricsObjects, null);
return obsv;
}
catch(Exception ex)
{
assert(false);
return null;
}
}
public MetricsMap.Entry
getEntry(MetricsMap> map)
{
for(MetricsMap.Entry e : _objects)
{
if(e.getMap() == map)
{
return e;
}
}
return null;
}
private java.util.List.Entry> _objects;
private long _previousDelay = 0;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy