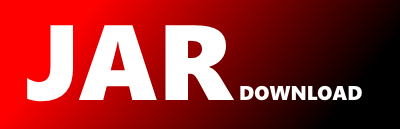
com.zipwhip.api.signals.SignalConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zipwhip-api Show documentation
Show all versions of zipwhip-api Show documentation
Java client to support applications powered by the Zipwhip Cloud
The newest version!
package com.zipwhip.api.signals;
import com.zipwhip.api.signals.commands.Command;
import com.zipwhip.api.signals.commands.SerializingCommand;
import com.zipwhip.api.signals.reconnect.ReconnectStrategy;
import com.zipwhip.api.signals.sockets.ConnectionHandle;
import com.zipwhip.api.signals.sockets.ConnectionState;
import com.zipwhip.concurrent.ObservableFuture;
import com.zipwhip.events.Observable;
import com.zipwhip.lifecycle.Destroyable;
import java.net.SocketAddress;
/**
* Created by IntelliJ IDEA. User: Michael Date: 8/2/11 Time: 11:48 AM
*
* Encapsulates a connection to the signal server. This is a very LOW LEVEL
* interface for talking with the SignalServer.
*
* It's not really intended for the callers to interact with this API directly.
*/
public interface SignalConnection extends Destroyable {
ConnectionHandle getConnectionHandle();
ConnectionState getConnectionState();
/**
* Initiate a raw TCP connection to the signal server ASYNCHRONOUSLY. This
* is just a raw connection, not an authenticated/initialized one.
*
* @return The future will tell you when the connection is complete.
* @throws Exception if there is is an error connecting
*/
ObservableFuture connect();
/**
* Kills the current connection and reconnects to a new connection within one safe operation/transaction. Will not allow other
* operations to occur between those two actions.
*
* @return
*/
ObservableFuture reconnect();
/**
* Kill the TCP connection to the SignalServer ASYNCHRONOUSLY
*
* @return The future will tell you when the connection is terminated, (Connection that was disconnected)
* @throws Exception if there is is an error disconnecting
*/
ObservableFuture disconnect();
/**
* Kill the TCP connection to the SignalServer ASYNCHRONOUSLY. Pass true if the disconnect
* is being ordered as a result of a network problem such as a stale connection or a channel
* disconnect.
*
* @param network True if the disconnect results from a problem on the network.
* @return The future will tell you when the connection is terminated, (Connection that was disconnected)
* @throws Exception if there is is an error disconnecting
*/
ObservableFuture disconnect(boolean network);
/**
* Cancel any pending network keepalives and fire one immediately.
*/
ObservableFuture ping();
/**
* Send something to the SignalServer
*
* @param command the Command to send
* @return a {@code Future} of type boolean where true is a successful send.
*/
ObservableFuture send(SerializingCommand command);
/**
* Allows you to observe the connection trying to connect.
* The observer will return True if the connection was successful, False otherwise.
*/
Observable getConnectEvent();
/**
* Allows you to observe the connection disconnecting.
* The observer will return True if a reconnect is requested, False otherwise.
*
* @return observer An observer to receive callbacks on when this event fires
*/
Observable getDisconnectEvent();
/**
* Allows you to listen for things that are received by the API.
*/
Observable getCommandReceivedEvent();
/**
* Observe an inactive ping event.
*/
Observable getPingEventReceivedEvent();
/**
* Observe a caught exception in the connection.
*/
Observable getExceptionEvent();
/**
* The address of the server to connect to.
*
* @param address
*/
void setAddress(SocketAddress address);
SocketAddress getAddress();
/**
* Get the current reconnection strategy for the connection.
*
* @return the current reconnection strategy for the connection.
*/
ReconnectStrategy getReconnectStrategy();
/**
* Set the reconnection strategy for the connection.
*
* @param strategy the strategy to use when reconnecting
*/
void setReconnectStrategy(ReconnectStrategy strategy);
/**
* Set the current setting for a connection time out in seconds.
*
* @param connectTimeoutSeconds The setting for a connection time out in seconds.
*/
void setConnectTimeoutSeconds(int connectTimeoutSeconds);
/**
* Get the current setting for a connection time out in seconds.
*
* @return The current setting for a connection time out in seconds.
*/
int getConnectTimeoutSeconds();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy