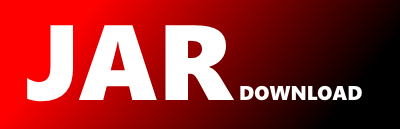
com.zipwhip.api.signals.SignalObserverAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zipwhip-api Show documentation
Show all versions of zipwhip-api Show documentation
Java client to support applications powered by the Zipwhip Cloud
The newest version!
package com.zipwhip.api.signals;
import com.zipwhip.api.dto.*;
import com.zipwhip.events.Observer;
import com.zipwhip.util.StringUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.List;
/**
* Created by IntelliJ IDEA.
* User: jed
* Date: 9/6/11
* Time: 5:22 PM
*
* This is a convenience class for translating a generic Signal into a Zipwhip DTO.
*
* Use this when calling ZipwhipClient.addSignalObserver()
*/
public abstract class SignalObserverAdapter implements Observer> {
private static Logger logger = LoggerFactory.getLogger(SignalObserverAdapter.class);
public enum SignalType {
CONTACT,
CONVERSATION,
DEVICE,
MESSAGE,
CARBON,
NOVALUE,
UNKNOWN;
public static SignalType toSignalType(String typeString) {
if (StringUtil.isNullOrEmpty(typeString)) {
return NOVALUE;
}
// Cleanup the type string
String enumString = typeString.toUpperCase();
try {
return valueOf(enumString);
} catch (Exception ex) {
return UNKNOWN;
}
}
}
/**
* This method is final as it does the adaptation from Signal to DTO.
*
* @param sender The sender might not be the same object every time, so we'll let it just be object.
* @param signals A list of signals we are being notified about.
*/
@Override
final public void notify(Object sender, List signals) {
for (Signal signal : signals) {
logger.debug("Received signal of TYPE: " + signal.getType());
switch (SignalType.toSignalType(signal.getType())) {
case MESSAGE:
notifyMessage(signal, (Message) signal.getContent());
break;
case CONTACT:
notifyContact(signal, (Contact) signal.getContent());
break;
case CONVERSATION:
notifyConversation(signal, (Conversation) signal.getContent());
break;
case CARBON:
notifyCarbonEvent(signal, (CarbonEvent) signal.getContent());
break;
case DEVICE:
notifyDevice(signal, (Device) signal.getContent());
break;
default:
logger.warn("No match found for TYPE: " + signal.getType());
notifyUnrecognised(signal);
break;
}
}
}
public void notifyContact(Signal signal, Contact contact) {
logger.debug("notifyContact - Not implemented");
}
public void notifyConversation(Signal signal, Conversation conversation) {
logger.debug("notifyConversation - Not implemented");
}
public void notifyDevice(Signal signal, Device device) {
logger.debug("notifyDevice - Not implemented");
}
public void notifyMessage(Signal signal, Message message) {
logger.debug("notifyMessage - Not implemented");
}
public void notifyCarbonEvent(Signal signal, CarbonEvent carbonEvent) {
logger.debug("notifyCarbonEvent - Not implemented");
}
public void notifyUnrecognised(Signal signal) {
logger.debug("notifyUnrecognised - Not implemented");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy