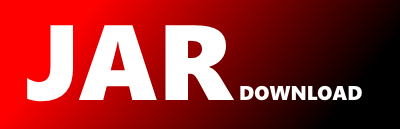
com.zipwhip.important.schedulers.ZipwhipTimerScheduler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zipwhip-api Show documentation
Show all versions of zipwhip-api Show documentation
Java client to support applications powered by the Zipwhip Cloud
The newest version!
package com.zipwhip.important.schedulers;
import com.zipwhip.events.ObservableHelper;
import com.zipwhip.events.Observer;
import com.zipwhip.executors.NamedThreadFactory;
import com.zipwhip.important.Scheduler;
import com.zipwhip.lifecycle.CascadingDestroyableBase;
import com.zipwhip.lifecycle.DestroyableBase;
import com.zipwhip.timers.HashedWheelTimer;
import com.zipwhip.timers.Timeout;
import com.zipwhip.timers.Timer;
import com.zipwhip.timers.TimerTask;
import com.zipwhip.util.HashCodeComparator;
import java.util.Collections;
import java.util.Date;
import java.util.Map;
import java.util.TreeMap;
import java.util.concurrent.TimeUnit;
/**
* Created by IntelliJ IDEA.
* User: Russ
* Date: 8/29/12
* Time: 12:37 PM
*/
public class ZipwhipTimerScheduler extends CascadingDestroyableBase implements Scheduler {
private final Timer timer;
private final ObservableHelper observableHelper = new ObservableHelper();
private Map map = Collections.synchronizedMap(new TreeMap(HashCodeComparator.getInstance()));
public ZipwhipTimerScheduler() {
this(null, "ZipwhipTimerScheduler");
}
public ZipwhipTimerScheduler(String name) {
this(null, name);
}
public ZipwhipTimerScheduler(Timer timer) {
this.timer = timer;
}
protected ZipwhipTimerScheduler(final Timer timer, String name) {
this(timer == null ? new HashedWheelTimer(new NamedThreadFactory("HashedWheelScheduler-" + name), 1, TimeUnit.SECONDS) : timer);
// since we created it, destroy it when we get destroyed.
if (timer == null){
this.link(new DestroyableBase() {
@Override
protected void onDestroy() {
ZipwhipTimerScheduler.this.timer.stop();
}
});
}
}
@Override
public synchronized void schedule(final String requestId, Date exitTime) {
Timeout timeout = timer.newTimeout(new TimerTask() {
@Override
public void run(Timeout timeout) throws Exception {
synchronized (ZipwhipTimerScheduler.this) {
observableHelper.notifyObservers(ZipwhipTimerScheduler.this, requestId);
if (isSameRequest(requestId, timeout)) {
map.remove(requestId);
}
}
}
}, exitTime.getTime() - System.currentTimeMillis(), TimeUnit.MILLISECONDS);
map.put(requestId, timeout);
}
@Override
public void scheduleRecurring(final String requestId, final long interval, final TimeUnit units) {
TimerTask task = new TimerTask() {
@Override
public void run(Timeout timeout) throws Exception {
synchronized (ZipwhipTimerScheduler.this) {
observableHelper.notifyObservers(ZipwhipTimerScheduler.this, requestId);
if (isSameRequest(requestId, timeout)) {
map.remove(requestId);
}
}
Timeout t = timer.newTimeout(this, interval, units);
map.put(requestId, t);
}
};
Timeout timeout = timer.newTimeout(task, interval, units);
map.put(requestId, timeout);
}
@Override
public synchronized void cancel(String requestId) {
Timeout timeout = map.get(requestId);
if (isSameRequest(requestId, timeout)) {
timeout.cancel();
map.remove(requestId);
}
}
@Override
public void onScheduleComplete(Observer observer) {
observableHelper.addObserver(observer);
}
@Override
public void removeOnScheduleComplete(Observer observer) {
observableHelper.removeObserver(observer);
}
@Override
protected void onDestroy() {
}
private boolean isSameRequest(String requestId, Timeout timeout) {
return (map.get(requestId) == timeout);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy