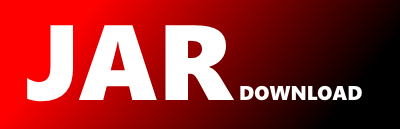
com.zipwhip.util.DownloadURL Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zipwhip-api Show documentation
Show all versions of zipwhip-api Show documentation
Java client to support applications powered by the Zipwhip Cloud
The newest version!
package com.zipwhip.util;
//------------------------------------------------------------//
// DnldURL.java: //
//------------------------------------------------------------//
// A Java program that demonstrates a procedure that can be //
// used to download the contents of a specified URL. //
//------------------------------------------------------------//
// Code created by Developer's Daily //
// http://www.DevDaily.com //
//------------------------------------------------------------//
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.BufferedInputStream;
import java.io.DataInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
public class DownloadURL {
private static Logger LOGGER = LoggerFactory.getLogger(DownloadURL.class);
public static String get(String url) throws Exception {
String result = "";
//-----------------------------------------------------//
// Step 1: Start creating a few objects we'll need.
//-----------------------------------------------------//
URL u;
InputStream is = null;
DataInputStream dis;
String s;
try {
//------------------------------------------------------------//
// Step 2: Create the URL. //
//------------------------------------------------------------//
// Note: Put your real URL here, or better yet, read it as a //
// command-line arg, or read it from a file. //
//------------------------------------------------------------//
u = new URL(url);
//----------------------------------------------//
// Step 3: Open an input stream from the url. //
//----------------------------------------------//
is = u.openStream(); // throws an IOException
//-------------------------------------------------------------//
// Step 4: //
//-------------------------------------------------------------//
// Convert the InputStream to a buffered DataInputStream. //
// Buffering the stream makes the reading faster; the //
// readLine() method of the DataInputStream makes the reading //
// easier. //
//-------------------------------------------------------------//
dis = new DataInputStream(new BufferedInputStream(is));
//------------------------------------------------------------//
// Step 5: //
//------------------------------------------------------------//
// Now just read each record of the input stream, and print //
// it out. Note that it's assumed that this problem is run //
// from a command-line, not from an application or applet. //
//------------------------------------------------------------//
while ((s = dis.readLine()) != null) {
result += s;
}
} catch (MalformedURLException mue) {
LOGGER.error("Ouch - a MalformedURLException happened.", mue);
mue.printStackTrace();
throw mue;
} catch (IOException ioe) {
LOGGER.error("Oops- an IOException happened.", ioe);
throw ioe;
} finally {
//---------------------------------//
// Step 6: Close the InputStream //
//---------------------------------//
try {
if (is != null) {
is.close();
}
} catch (IOException ioe) {
// just going to ignore this one
}
} // end of 'finally' clause
return result;
} // end of main
} // end of class definition
© 2015 - 2025 Weber Informatics LLC | Privacy Policy