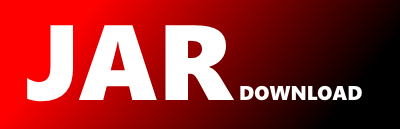
com.zipwhip.util.GenericLocalDirectory Maven / Gradle / Ivy
package com.zipwhip.util;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
/**
* Created by IntelliJ IDEA.
* User: Michael
* Date: 11/1/11
* Time: 6:39 PM
*
* Something basic/generic
*/
public class GenericLocalDirectory extends GenericDirectory implements LocalDirectory {
protected static final Comparator COMPARATOR = new HashCodeComparator();
protected Factory> factory = null;
protected Map> data = null;
public GenericLocalDirectory(Factory> factory) {
this.factory = factory;
}
@Override
public Set keySet() {
if (data == null) {
return Collections.emptySet();
}
return data.keySet();
}
@Override
public boolean isEmpty() {
if (data == null) {
return true;
}
return data.isEmpty();
}
@Override
public void clear() {
if (data == null) {
return;
}
data.clear();
}
@Override
public Collection get(TKey key) {
if (data == null){
return null;
}
return data.get(key);
}
@Override
protected void removeFromStore(TKey key) {
if (data == null){
return;
}
data.remove(key);
}
@Override
protected Collection getOrCreateCollection(TKey key) {
if (data == null) {
synchronized (this) {
if (data == null) {
data = createStore();
}
}
}
Collection collection = data.get(key);
if (collection == null) {
synchronized (data) {
collection = data.get(key);
if (collection == null) {
try {
collection = factory.create();
} catch (Exception e) {
// TODO: figure out if we should add an exception to the .add() method
throw new RuntimeException("Cannot create collection via factory", e);
}
data.put(key, collection);
}
}
}
return collection;
}
protected Map> createStore() {
return Collections.synchronizedMap(new TreeMap>(COMPARATOR));
}
@Override
protected void onDestroy() {
if(data != null) {
data.clear();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy