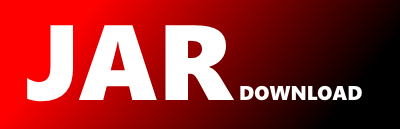
com.zipwhip.util.StringUtil Maven / Gradle / Ivy
package com.zipwhip.util;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Created by IntelliJ IDEA. User: Ali Date: Mar 22, 2010 Time: 2:04:58 PM
*
* A collection of useful utilities for working with strings.
*/
public class StringUtil {
public static final String EMPTY_STRING = "";
// private static final List VALID_NUMBERS = Arrays.asList("0", "1", "2", "3", "4", "5", "6", "7", "8",
// "9");
private static final String PLUS_MOBIFONE = "+84";
private static final String PLUS = "+";
private static final String NANP = "+1";
public static boolean equals(String string1, String string2) {
if (string1 == string2) {
return true; // covers both null, or both same instance
}
if (string1 == null) {
return false; // covers 1 null, other not.
}
return (string1.equals(string2)); // covers equals
}
public static boolean equalsIgnoreCase(String string1, String... strings) {
if (CollectionUtil.isNullOrEmpty(strings)) {
if (StringUtil.isNullOrEmpty(string1)) {
return true;
}
return false;
}
for (String string : strings) {
if (string1 == string) {
return true; // covers both null, or both same instance
}
if ((string1 == null) || (string1 == "") || (string == null) || (string == "")) {
return false;
}
if (string1 == null) {
return false; // covers 1 null, other not.
}
if (string1.equalsIgnoreCase(string)) {
// covers equals
return true;
}
}
return false;
}
public static String[] split(String s, String regex) {
if (isNullOrEmpty(s) || isNullOrEmpty(regex)) {
String[] out = new String[1];
out[0] = s;
return out;
}
return s.split(regex);
}
/**
* Strips all characters that are not numbers (0 - 9) and returns a new
* string. Returns and empty string if the mobile number is null or empty.
* Assumes US format cleaning "1" from the beginning if it exists.
*
* @param mobileNumber - mobile number string to parse
* @return String - parsed mobile number
*/
public static String safeCleanMobileNumber(String mobileNumber) {
return safeCleanMobileNumber(mobileNumber, false);
}
/**
* Strips all characters that are not numbers (0 - 9) and returns a new
* string. Returns and empty string if the mobile number is null or empty.
*
* @param mobileNumber - mobile number string to parse
* @param appendInternational appends (1) at the beginning of mobile number (international format)
* @return String - parsed mobile number
*/
public static String safeCleanMobileNumber(String mobileNumber, boolean appendInternational) {
String cleanMobileNumber = cleanMobileNumber(mobileNumber);
if (isNullOrEmpty(cleanMobileNumber)) {
return EMPTY_STRING;
}
if (appendInternational && (cleanMobileNumber.length() == 10)) {
return "1" + cleanMobileNumber;
} else if ((cleanMobileNumber.length() == 11) && equals(cleanMobileNumber.charAt(0), "1")) {
return cleanMobileNumber.substring(1);
} else if (!appendInternational && (cleanMobileNumber.length() == 13) && cleanMobileNumber.startsWith("001")) {
return cleanMobileNumber.substring(3);
}
return cleanMobileNumber;
}
private static boolean equals(char string1, String string2) {
return equals(new String(new char[] { string1 }), string2);
}
/**
* Strips all characters that are not numbers (0 - 9) and returns a new
* string. Returns an empty string if the mobile number is null or empty.
*
* @param mobileNumber - mobile number string to parse
* @return String - parsed mobile number
*/
private static final Pattern validMobileNumber = Pattern.compile("^(\\+84)?\\d+$");
protected static String cleanMobileNumber(String mobileNumber) {
if (isNullOrEmpty(mobileNumber)) {
return EMPTY_STRING;
}
Matcher match = validMobileNumber.matcher(mobileNumber);
if (match.find()) {
return mobileNumber;
} else {
StringBuilder cleanMobileNumber = new StringBuilder();
int index = 0;
if (mobileNumber.startsWith(PLUS_MOBIFONE)) {
index++;
cleanMobileNumber.append(PLUS);
} else if (mobileNumber.startsWith(NANP)) {
index = 2;
}
for (int i = index; i < mobileNumber.length(); i++) {
char c = mobileNumber.charAt(i);
if ((i == 0) && ((c == '+') || (((c >= 0x30) && (c <= 0x39))))) {
// if (contains(VALID_NUMBERS, mobileNumber.charAt(i))) {
cleanMobileNumber.append(c);
} else if (((c >= 0x30) && (c <= 0x39))) {
cleanMobileNumber.append(c);
}
}
return cleanMobileNumber.toString();
}
}
private static boolean contains(List validNumbers, char toFind) {
if (CollectionUtil.isNullOrEmpty(validNumbers)) {
return false;
}
String string = new String(new char[] { toFind });
return validNumbers.contains(string);
}
public static boolean exists(String string) {
return !isNullOrEmpty(string);
}
public static boolean isNullOrEmpty(String string) {
//used string.length() == 0 because not everyone has Java 1.6
return (string == null) || (string.length()==0) || StringUtil.equalsIgnoreCase(string, "null");
}
public static String defaultValue(String value, String defaultValue) {
return isNullOrEmpty(value) ? defaultValue : value;
}
public static String replaceAll(String initialString, String regex, String replacement) {
if ((initialString == null) || (regex == null) || (replacement == null)) {
return initialString;
}
return initialString.replaceAll(regex, replacement);
}
public static String replace(String s, CharSequence target, CharSequence newChar) {
if ((s == null) || (target == null) || (newChar == null)) {
return s;
}
return s.replace(target, newChar);
}
/**
* Case insensitive.
*
* @param source
* @param toFind
* @return
*/
public static boolean startsWith(String source, String toFind) {
if (source == null) {
return false;
}
if (toFind == null) {
return false;
}
return (source.toLowerCase().startsWith(toFind.toLowerCase()));
}
public static boolean contains(String source, String toFind) {
if (source == null) {
return false;
}
if (toFind == null) {
return false;
}
return (source.toLowerCase().contains(toFind.toLowerCase()));
}
public static String join(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy