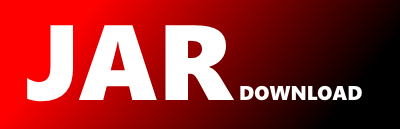
com.ziqni.admin.sdk.model.CreateEventRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces.
*
* The version of the OpenAPI document: 3.0.11
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* CreateEventRequest
*/
@JsonPropertyOrder({
CreateEventRequest.JSON_PROPERTY_MEMBER_REF_ID,
CreateEventRequest.JSON_PROPERTY_ACTION,
CreateEventRequest.JSON_PROPERTY_BATCH_ID,
CreateEventRequest.JSON_PROPERTY_ENTITY_REF_ID,
CreateEventRequest.JSON_PROPERTY_SOURCE_VALUE,
CreateEventRequest.JSON_PROPERTY_TRANSACTION_TIMESTAMP,
CreateEventRequest.JSON_PROPERTY_TAGS,
CreateEventRequest.JSON_PROPERTY_EVENT_REF_ID,
CreateEventRequest.JSON_PROPERTY_MEMBER_ID,
CreateEventRequest.JSON_PROPERTY_CUSTOM_FIELDS,
CreateEventRequest.JSON_PROPERTY_UNIT_OF_MEASURE
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class CreateEventRequest {
public static final String JSON_PROPERTY_MEMBER_REF_ID = "memberRefId";
private String memberRefId;
public static final String JSON_PROPERTY_ACTION = "action";
private String action;
public static final String JSON_PROPERTY_BATCH_ID = "batchId";
private String batchId;
public static final String JSON_PROPERTY_ENTITY_REF_ID = "entityRefId";
private String entityRefId;
public static final String JSON_PROPERTY_SOURCE_VALUE = "sourceValue";
private Double sourceValue;
public static final String JSON_PROPERTY_TRANSACTION_TIMESTAMP = "transactionTimestamp";
private OffsetDateTime transactionTimestamp;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags = null;
public static final String JSON_PROPERTY_EVENT_REF_ID = "eventRefId";
private String eventRefId;
public static final String JSON_PROPERTY_MEMBER_ID = "memberId";
private String memberId;
public static final String JSON_PROPERTY_CUSTOM_FIELDS = "customFields";
private Map customFields = null;
public static final String JSON_PROPERTY_UNIT_OF_MEASURE = "unitOfMeasure";
private String unitOfMeasure;
public CreateEventRequest memberRefId(String memberRefId) {
this.memberRefId = memberRefId;
return this;
}
/**
* The reference to this member in external system
* @return memberRefId
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "Player-1", required = true, value = "The reference to this member in external system")
@JsonProperty(JSON_PROPERTY_MEMBER_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getMemberRefId() {
return memberRefId;
}
@JsonProperty(JSON_PROPERTY_MEMBER_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMemberRefId(String memberRefId) {
this.memberRefId = memberRefId;
}
public CreateEventRequest action(String action) {
this.action = action;
return this;
}
/**
* The identifier that describes the meaning of this event
* @return action
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "win", required = true, value = "The identifier that describes the meaning of this event")
@JsonProperty(JSON_PROPERTY_ACTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAction() {
return action;
}
@JsonProperty(JSON_PROPERTY_ACTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAction(String action) {
this.action = action;
}
public CreateEventRequest batchId(String batchId) {
this.batchId = batchId;
return this;
}
/**
* The batch identifier is used to group related event stream data together. This could be as simple as a UUID or your internal reference to that distinct player session event
* @return batchId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "ecrsamQm23r61KgaPQq7x", value = "The batch identifier is used to group related event stream data together. This could be as simple as a UUID or your internal reference to that distinct player session event")
@JsonProperty(JSON_PROPERTY_BATCH_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getBatchId() {
return batchId;
}
@JsonProperty(JSON_PROPERTY_BATCH_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBatchId(String batchId) {
this.batchId = batchId;
}
public CreateEventRequest entityRefId(String entityRefId) {
this.entityRefId = entityRefId;
return this;
}
/**
* It is a reference to a game/product ID from external system
* @return entityRefId
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "fruits", required = true, value = "It is a reference to a game/product ID from external system")
@JsonProperty(JSON_PROPERTY_ENTITY_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEntityRefId() {
return entityRefId;
}
@JsonProperty(JSON_PROPERTY_ENTITY_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEntityRefId(String entityRefId) {
this.entityRefId = entityRefId;
}
public CreateEventRequest sourceValue(Double sourceValue) {
this.sourceValue = sourceValue;
return this;
}
/**
* The actual numerical value related to the event
* @return sourceValue
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "12.345", required = true, value = "The actual numerical value related to the event")
@JsonProperty(JSON_PROPERTY_SOURCE_VALUE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getSourceValue() {
return sourceValue;
}
@JsonProperty(JSON_PROPERTY_SOURCE_VALUE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSourceValue(Double sourceValue) {
this.sourceValue = sourceValue;
}
public CreateEventRequest transactionTimestamp(OffsetDateTime transactionTimestamp) {
this.transactionTimestamp = transactionTimestamp;
return this;
}
/**
* ISO8601 timestamp for when this event happened. All records are stored in UTC time zone
* @return transactionTimestamp
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "ISO8601 timestamp for when this event happened. All records are stored in UTC time zone")
@JsonProperty(JSON_PROPERTY_TRANSACTION_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getTransactionTimestamp() {
return transactionTimestamp;
}
@JsonProperty(JSON_PROPERTY_TRANSACTION_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTransactionTimestamp(OffsetDateTime transactionTimestamp) {
this.transactionTimestamp = transactionTimestamp;
}
public CreateEventRequest tags(List tags) {
this.tags = tags;
return this;
}
public CreateEventRequest addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* A list of Strings of groups that the tag belongs to
* @return tags
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"Dc4swmQBVd51K6gPQqFx\",\"Dc4swmQBVd51K6gPQqF2\"]", value = "A list of Strings of groups that the tag belongs to")
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public CreateEventRequest eventRefId(String eventRefId) {
this.eventRefId = eventRefId;
return this;
}
/**
* Get eventRefId
* @return eventRefId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_EVENT_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEventRefId() {
return eventRefId;
}
@JsonProperty(JSON_PROPERTY_EVENT_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEventRefId(String eventRefId) {
this.eventRefId = eventRefId;
}
public CreateEventRequest memberId(String memberId) {
this.memberId = memberId;
return this;
}
/**
* Get memberId
* @return memberId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_MEMBER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMemberId() {
return memberId;
}
@JsonProperty(JSON_PROPERTY_MEMBER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMemberId(String memberId) {
this.memberId = memberId;
}
public CreateEventRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public CreateEventRequest putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* A list of custom field entries
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of custom field entries")
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getCustomFields() {
return customFields;
}
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public CreateEventRequest unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* The unit of measure is used to determine the type of the source value. It is used to normalize points values for currency based calculations by normalizing the source value.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unit of measure is used to determine the type of the source value. It is used to normalize points values for currency based calculations by normalizing the source value.")
@JsonProperty(JSON_PROPERTY_UNIT_OF_MEASURE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUnitOfMeasure() {
return unitOfMeasure;
}
@JsonProperty(JSON_PROPERTY_UNIT_OF_MEASURE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
/**
* Return true if this CreateEventRequest object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateEventRequest createEventRequest = (CreateEventRequest) o;
return Objects.equals(this.memberRefId, createEventRequest.memberRefId) &&
Objects.equals(this.action, createEventRequest.action) &&
Objects.equals(this.batchId, createEventRequest.batchId) &&
Objects.equals(this.entityRefId, createEventRequest.entityRefId) &&
Objects.equals(this.sourceValue, createEventRequest.sourceValue) &&
Objects.equals(this.transactionTimestamp, createEventRequest.transactionTimestamp) &&
Objects.equals(this.tags, createEventRequest.tags) &&
Objects.equals(this.eventRefId, createEventRequest.eventRefId) &&
Objects.equals(this.memberId, createEventRequest.memberId) &&
Objects.equals(this.customFields, createEventRequest.customFields) &&
Objects.equals(this.unitOfMeasure, createEventRequest.unitOfMeasure);
}
@Override
public int hashCode() {
return Objects.hash(memberRefId, action, batchId, entityRefId, sourceValue, transactionTimestamp, tags, eventRefId, memberId, customFields, unitOfMeasure);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateEventRequest {\n");
sb.append(" memberRefId: ").append(toIndentedString(memberRefId)).append("\n");
sb.append(" action: ").append(toIndentedString(action)).append("\n");
sb.append(" batchId: ").append(toIndentedString(batchId)).append("\n");
sb.append(" entityRefId: ").append(toIndentedString(entityRefId)).append("\n");
sb.append(" sourceValue: ").append(toIndentedString(sourceValue)).append("\n");
sb.append(" transactionTimestamp: ").append(toIndentedString(transactionTimestamp)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" eventRefId: ").append(toIndentedString(eventRefId)).append("\n");
sb.append(" memberId: ").append(toIndentedString(memberId)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy