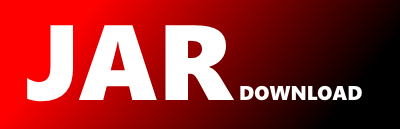
com.ziqni.admin.sdk.model.LogEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces.
*
* The version of the OpenAPI document: 3.0.11
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* LogEvent
*/
@JsonPropertyOrder({
LogEvent.JSON_PROPERTY_ACCOUNT_ID,
LogEvent.JSON_PROPERTY_LOG_LEVEL,
LogEvent.JSON_PROPERTY_MESSAGE,
LogEvent.JSON_PROPERTY_HOST_NAME,
LogEvent.JSON_PROPERTY_CREATED,
LogEvent.JSON_PROPERTY_SERVER_TYPE,
LogEvent.JSON_PROPERTY_ID,
LogEvent.JSON_PROPERTY_STACK_TRACE,
LogEvent.JSON_PROPERTY_PRIVATE_IP_ADDRESS,
LogEvent.JSON_PROPERTY_VERSION,
LogEvent.JSON_PROPERTY_ENTITY_ID,
LogEvent.JSON_PROPERTY_ENTITY_TYPE
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class LogEvent {
public static final String JSON_PROPERTY_ACCOUNT_ID = "accountId";
private String accountId;
public static final String JSON_PROPERTY_LOG_LEVEL = "logLevel";
private String logLevel;
public static final String JSON_PROPERTY_MESSAGE = "message";
private String message;
public static final String JSON_PROPERTY_HOST_NAME = "hostName";
private String hostName;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_SERVER_TYPE = "serverType";
private String serverType;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_STACK_TRACE = "stackTrace";
private String stackTrace;
public static final String JSON_PROPERTY_PRIVATE_IP_ADDRESS = "privateIpAddress";
private String privateIpAddress;
public static final String JSON_PROPERTY_VERSION = "version";
private Long version;
public static final String JSON_PROPERTY_ENTITY_ID = "entityId";
private String entityId;
public static final String JSON_PROPERTY_ENTITY_TYPE = "entityType";
private String entityType;
public LogEvent accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAccountId() {
return accountId;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public LogEvent logLevel(String logLevel) {
this.logLevel = logLevel;
return this;
}
/**
* Get logLevel
* @return logLevel
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_LOG_LEVEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getLogLevel() {
return logLevel;
}
@JsonProperty(JSON_PROPERTY_LOG_LEVEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLogLevel(String logLevel) {
this.logLevel = logLevel;
}
public LogEvent message(String message) {
this.message = message;
return this;
}
/**
* Get message
* @return message
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_MESSAGE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getMessage() {
return message;
}
@JsonProperty(JSON_PROPERTY_MESSAGE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMessage(String message) {
this.message = message;
}
public LogEvent hostName(String hostName) {
this.hostName = hostName;
return this;
}
/**
* Get hostName
* @return hostName
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_HOST_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getHostName() {
return hostName;
}
@JsonProperty(JSON_PROPERTY_HOST_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHostName(String hostName) {
this.hostName = hostName;
}
public LogEvent created(OffsetDateTime created) {
this.created = created;
return this;
}
/**
* date-time
* @return created
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "date-time")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getCreated() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreated(OffsetDateTime created) {
this.created = created;
}
public LogEvent serverType(String serverType) {
this.serverType = serverType;
return this;
}
/**
* Get serverType
* @return serverType
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_SERVER_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getServerType() {
return serverType;
}
@JsonProperty(JSON_PROPERTY_SERVER_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setServerType(String serverType) {
this.serverType = serverType;
}
public LogEvent id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public LogEvent stackTrace(String stackTrace) {
this.stackTrace = stackTrace;
return this;
}
/**
* Get stackTrace
* @return stackTrace
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_STACK_TRACE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getStackTrace() {
return stackTrace;
}
@JsonProperty(JSON_PROPERTY_STACK_TRACE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStackTrace(String stackTrace) {
this.stackTrace = stackTrace;
}
public LogEvent privateIpAddress(String privateIpAddress) {
this.privateIpAddress = privateIpAddress;
return this;
}
/**
* Get privateIpAddress
* @return privateIpAddress
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_PRIVATE_IP_ADDRESS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getPrivateIpAddress() {
return privateIpAddress;
}
@JsonProperty(JSON_PROPERTY_PRIVATE_IP_ADDRESS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPrivateIpAddress(String privateIpAddress) {
this.privateIpAddress = privateIpAddress;
}
public LogEvent version(Long version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getVersion() {
return version;
}
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVersion(Long version) {
this.version = version;
}
public LogEvent entityId(String entityId) {
this.entityId = entityId;
return this;
}
/**
* Get entityId
* @return entityId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_ENTITY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEntityId() {
return entityId;
}
@JsonProperty(JSON_PROPERTY_ENTITY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEntityId(String entityId) {
this.entityId = entityId;
}
public LogEvent entityType(String entityType) {
this.entityType = entityType;
return this;
}
/**
* Get entityType
* @return entityType
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEntityType() {
return entityType;
}
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEntityType(String entityType) {
this.entityType = entityType;
}
/**
* Return true if this LogEvent object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LogEvent logEvent = (LogEvent) o;
return Objects.equals(this.accountId, logEvent.accountId) &&
Objects.equals(this.logLevel, logEvent.logLevel) &&
Objects.equals(this.message, logEvent.message) &&
Objects.equals(this.hostName, logEvent.hostName) &&
Objects.equals(this.created, logEvent.created) &&
Objects.equals(this.serverType, logEvent.serverType) &&
Objects.equals(this.id, logEvent.id) &&
Objects.equals(this.stackTrace, logEvent.stackTrace) &&
Objects.equals(this.privateIpAddress, logEvent.privateIpAddress) &&
Objects.equals(this.version, logEvent.version) &&
Objects.equals(this.entityId, logEvent.entityId) &&
Objects.equals(this.entityType, logEvent.entityType);
}
@Override
public int hashCode() {
return Objects.hash(accountId, logLevel, message, hostName, created, serverType, id, stackTrace, privateIpAddress, version, entityId, entityType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LogEvent {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" logLevel: ").append(toIndentedString(logLevel)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" hostName: ").append(toIndentedString(hostName)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" serverType: ").append(toIndentedString(serverType)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" stackTrace: ").append(toIndentedString(stackTrace)).append("\n");
sb.append(" privateIpAddress: ").append(toIndentedString(privateIpAddress)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" entityId: ").append(toIndentedString(entityId)).append("\n");
sb.append(" entityType: ").append(toIndentedString(entityType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy