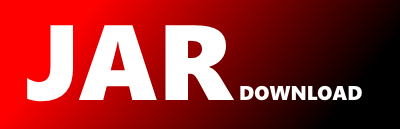
com.ziqni.admin.sdk.model.AccountMessageAllOf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.ziqni.admin.sdk.model.EventRefType;
import com.ziqni.admin.sdk.model.MessageLink;
import com.ziqni.admin.sdk.model.MessageStatus;
import com.ziqni.admin.sdk.model.MessageType;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* AccountMessageAllOf
*/
@JsonPropertyOrder({
AccountMessageAllOf.JSON_PROPERTY_EXPIRY,
AccountMessageAllOf.JSON_PROPERTY_EVENT_REF_TYPE,
AccountMessageAllOf.JSON_PROPERTY_EVENT_REF_ID,
AccountMessageAllOf.JSON_PROPERTY_MESSAGE_TYPE,
AccountMessageAllOf.JSON_PROPERTY_SUBJECT,
AccountMessageAllOf.JSON_PROPERTY_BODY,
AccountMessageAllOf.JSON_PROPERTY_STATUS,
AccountMessageAllOf.JSON_PROPERTY_USER_ID,
AccountMessageAllOf.JSON_PROPERTY_LINKS
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AccountMessageAllOf {
public static final String JSON_PROPERTY_EXPIRY = "expiry";
private OffsetDateTime expiry;
public static final String JSON_PROPERTY_EVENT_REF_TYPE = "eventRefType";
private EventRefType eventRefType;
public static final String JSON_PROPERTY_EVENT_REF_ID = "eventRefId";
private String eventRefId;
public static final String JSON_PROPERTY_MESSAGE_TYPE = "messageType";
private MessageType messageType;
public static final String JSON_PROPERTY_SUBJECT = "subject";
private String subject;
public static final String JSON_PROPERTY_BODY = "body";
private String body;
public static final String JSON_PROPERTY_STATUS = "status";
private MessageStatus status;
public static final String JSON_PROPERTY_USER_ID = "userId";
private String userId;
public static final String JSON_PROPERTY_LINKS = "links";
private List links = new ArrayList<>();
public AccountMessageAllOf expiry(OffsetDateTime expiry) {
this.expiry = expiry;
return this;
}
/**
* The time that the message will disappear after. ISO8601 timestamp
* @return expiry
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The time that the message will disappear after. ISO8601 timestamp")
@JsonProperty(JSON_PROPERTY_EXPIRY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getExpiry() {
return expiry;
}
@JsonProperty(JSON_PROPERTY_EXPIRY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExpiry(OffsetDateTime expiry) {
this.expiry = expiry;
}
public AccountMessageAllOf eventRefType(EventRefType eventRefType) {
this.eventRefType = eventRefType;
return this;
}
/**
* Get eventRefType
* @return eventRefType
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_EVENT_REF_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public EventRefType getEventRefType() {
return eventRefType;
}
@JsonProperty(JSON_PROPERTY_EVENT_REF_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEventRefType(EventRefType eventRefType) {
this.eventRefType = eventRefType;
}
public AccountMessageAllOf eventRefId(String eventRefId) {
this.eventRefId = eventRefId;
return this;
}
/**
* The reference ID of the event object
* @return eventRefId
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "Dc4swmQBVd51K6gPQqFx", required = true, value = "The reference ID of the event object")
@JsonProperty(JSON_PROPERTY_EVENT_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEventRefId() {
return eventRefId;
}
@JsonProperty(JSON_PROPERTY_EVENT_REF_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEventRefId(String eventRefId) {
this.eventRefId = eventRefId;
}
public AccountMessageAllOf messageType(MessageType messageType) {
this.messageType = messageType;
return this;
}
/**
* Get messageType
* @return messageType
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_MESSAGE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public MessageType getMessageType() {
return messageType;
}
@JsonProperty(JSON_PROPERTY_MESSAGE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMessageType(MessageType messageType) {
this.messageType = messageType;
}
public AccountMessageAllOf subject(String subject) {
this.subject = subject;
return this;
}
/**
* The title of the message
* @return subject
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "Congratulations!", required = true, value = "The title of the message")
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSubject() {
return subject;
}
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSubject(String subject) {
this.subject = subject;
}
public AccountMessageAllOf body(String body) {
this.body = body;
return this;
}
/**
* The context of the message
* @return body
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "You have won", required = true, value = "The context of the message")
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getBody() {
return body;
}
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setBody(String body) {
this.body = body;
}
public AccountMessageAllOf status(MessageStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public MessageStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(MessageStatus status) {
this.status = status;
}
public AccountMessageAllOf userId(String userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_USER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getUserId() {
return userId;
}
@JsonProperty(JSON_PROPERTY_USER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUserId(String userId) {
this.userId = userId;
}
public AccountMessageAllOf links(List links) {
this.links = links;
return this;
}
public AccountMessageAllOf addLinksItem(MessageLink linksItem) {
this.links.add(linksItem);
return this;
}
/**
* Get links
* @return links
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLinks(List links) {
this.links = links;
}
/**
* Return true if this AccountMessage_allOf object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccountMessageAllOf accountMessageAllOf = (AccountMessageAllOf) o;
return Objects.equals(this.expiry, accountMessageAllOf.expiry) &&
Objects.equals(this.eventRefType, accountMessageAllOf.eventRefType) &&
Objects.equals(this.eventRefId, accountMessageAllOf.eventRefId) &&
Objects.equals(this.messageType, accountMessageAllOf.messageType) &&
Objects.equals(this.subject, accountMessageAllOf.subject) &&
Objects.equals(this.body, accountMessageAllOf.body) &&
Objects.equals(this.status, accountMessageAllOf.status) &&
Objects.equals(this.userId, accountMessageAllOf.userId) &&
Objects.equals(this.links, accountMessageAllOf.links);
}
@Override
public int hashCode() {
return Objects.hash(expiry, eventRefType, eventRefId, messageType, subject, body, status, userId, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccountMessageAllOf {\n");
sb.append(" expiry: ").append(toIndentedString(expiry)).append("\n");
sb.append(" eventRefType: ").append(toIndentedString(eventRefType)).append("\n");
sb.append(" eventRefId: ").append(toIndentedString(eventRefId)).append("\n");
sb.append(" messageType: ").append(toIndentedString(messageType)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" body: ").append(toIndentedString(body)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy