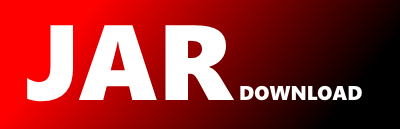
com.ziqni.admin.sdk.model.CreateUnitOfMeasureRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.ziqni.admin.sdk.model.CreateOptParamModels;
import com.ziqni.admin.sdk.model.CreateUnitOfMeasureRequestAllOf;
import com.ziqni.admin.sdk.model.UnitOfMeasureType;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* CreateUnitOfMeasureRequest
*/
@JsonPropertyOrder({
CreateUnitOfMeasureRequest.JSON_PROPERTY_CUSTOM_FIELDS,
CreateUnitOfMeasureRequest.JSON_PROPERTY_TAGS,
CreateUnitOfMeasureRequest.JSON_PROPERTY_METADATA,
CreateUnitOfMeasureRequest.JSON_PROPERTY_NAME,
CreateUnitOfMeasureRequest.JSON_PROPERTY_KEY,
CreateUnitOfMeasureRequest.JSON_PROPERTY_DESCRIPTION,
CreateUnitOfMeasureRequest.JSON_PROPERTY_ISO_CODE,
CreateUnitOfMeasureRequest.JSON_PROPERTY_SYMBOL,
CreateUnitOfMeasureRequest.JSON_PROPERTY_MULTIPLIER,
CreateUnitOfMeasureRequest.JSON_PROPERTY_UNIT_OF_MEASURE_TYPE
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class CreateUnitOfMeasureRequest {
public static final String JSON_PROPERTY_CUSTOM_FIELDS = "customFields";
private Map customFields = null;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags = null;
public static final String JSON_PROPERTY_METADATA = "metadata";
private Map metadata = null;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_KEY = "key";
private String key;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_ISO_CODE = "isoCode";
private String isoCode;
public static final String JSON_PROPERTY_SYMBOL = "symbol";
private String symbol;
public static final String JSON_PROPERTY_MULTIPLIER = "multiplier";
private Double multiplier;
public static final String JSON_PROPERTY_UNIT_OF_MEASURE_TYPE = "unitOfMeasureType";
private UnitOfMeasureType unitOfMeasureType;
public CreateUnitOfMeasureRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public CreateUnitOfMeasureRequest putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* A list of custom field entries
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of custom field entries")
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getCustomFields() {
return customFields;
}
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public CreateUnitOfMeasureRequest tags(List tags) {
this.tags = tags;
return this;
}
public CreateUnitOfMeasureRequest addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* A list of id's used to tag models
* @return tags
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"Dc4swmQBVd51K6gPQqFx\",\"Dc4swmQBVd51K6gPQqF2\"]", value = "A list of id's used to tag models")
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public CreateUnitOfMeasureRequest metadata(Map metadata) {
this.metadata = metadata;
return this;
}
public CreateUnitOfMeasureRequest putMetadataItem(String key, String metadataItem) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, metadataItem);
return this;
}
/**
* Get metadata
* @return metadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(Map metadata) {
this.metadata = metadata;
}
public CreateUnitOfMeasureRequest name(String name) {
this.name = name;
return this;
}
/**
* The name of a unit of measure
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "United States Dollar", required = true, value = "The name of a unit of measure")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public CreateUnitOfMeasureRequest key(String key) {
this.key = key;
return this;
}
/**
* The reference to the unit of measure in your system
* @return key
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "united-states-dollar", required = true, value = "The reference to the unit of measure in your system")
@JsonProperty(JSON_PROPERTY_KEY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getKey() {
return key;
}
@JsonProperty(JSON_PROPERTY_KEY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setKey(String key) {
this.key = key;
}
public CreateUnitOfMeasureRequest description(String description) {
this.description = description;
return this;
}
/**
* The description of a unit of measure
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "The United States dollar, or U.S. dollar, is made up of 100 cents and is presented with the symbol $ or US$ to differentiate it from other dollar-based currencies", value = "The description of a unit of measure")
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public CreateUnitOfMeasureRequest isoCode(String isoCode) {
this.isoCode = isoCode;
return this;
}
/**
* An alphabetical or numerical code to identify a unit of measure
* @return isoCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "An alphabetical or numerical code to identify a unit of measure")
@JsonProperty(JSON_PROPERTY_ISO_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIsoCode() {
return isoCode;
}
@JsonProperty(JSON_PROPERTY_ISO_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsoCode(String isoCode) {
this.isoCode = isoCode;
}
public CreateUnitOfMeasureRequest symbol(String symbol) {
this.symbol = symbol;
return this;
}
/**
* The symbol of a unit of measure
* @return symbol
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "$", value = "The symbol of a unit of measure")
@JsonProperty(JSON_PROPERTY_SYMBOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSymbol() {
return symbol;
}
@JsonProperty(JSON_PROPERTY_SYMBOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSymbol(String symbol) {
this.symbol = symbol;
}
public CreateUnitOfMeasureRequest multiplier(Double multiplier) {
this.multiplier = multiplier;
return this;
}
/**
* Is used to multiply the value from the standardised one that is being used
* @return multiplier
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "1", required = true, value = "Is used to multiply the value from the standardised one that is being used")
@JsonProperty(JSON_PROPERTY_MULTIPLIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getMultiplier() {
return multiplier;
}
@JsonProperty(JSON_PROPERTY_MULTIPLIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMultiplier(Double multiplier) {
this.multiplier = multiplier;
}
public CreateUnitOfMeasureRequest unitOfMeasureType(UnitOfMeasureType unitOfMeasureType) {
this.unitOfMeasureType = unitOfMeasureType;
return this;
}
/**
* Get unitOfMeasureType
* @return unitOfMeasureType
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_UNIT_OF_MEASURE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UnitOfMeasureType getUnitOfMeasureType() {
return unitOfMeasureType;
}
@JsonProperty(JSON_PROPERTY_UNIT_OF_MEASURE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUnitOfMeasureType(UnitOfMeasureType unitOfMeasureType) {
this.unitOfMeasureType = unitOfMeasureType;
}
/**
* Return true if this CreateUnitOfMeasureRequest object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateUnitOfMeasureRequest createUnitOfMeasureRequest = (CreateUnitOfMeasureRequest) o;
return Objects.equals(this.customFields, createUnitOfMeasureRequest.customFields) &&
Objects.equals(this.tags, createUnitOfMeasureRequest.tags) &&
Objects.equals(this.metadata, createUnitOfMeasureRequest.metadata) &&
Objects.equals(this.name, createUnitOfMeasureRequest.name) &&
Objects.equals(this.key, createUnitOfMeasureRequest.key) &&
Objects.equals(this.description, createUnitOfMeasureRequest.description) &&
Objects.equals(this.isoCode, createUnitOfMeasureRequest.isoCode) &&
Objects.equals(this.symbol, createUnitOfMeasureRequest.symbol) &&
Objects.equals(this.multiplier, createUnitOfMeasureRequest.multiplier) &&
Objects.equals(this.unitOfMeasureType, createUnitOfMeasureRequest.unitOfMeasureType);
}
@Override
public int hashCode() {
return Objects.hash(customFields, tags, metadata, name, key, description, isoCode, symbol, multiplier, unitOfMeasureType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateUnitOfMeasureRequest {\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" key: ").append(toIndentedString(key)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" isoCode: ").append(toIndentedString(isoCode)).append("\n");
sb.append(" symbol: ").append(toIndentedString(symbol)).append("\n");
sb.append(" multiplier: ").append(toIndentedString(multiplier)).append("\n");
sb.append(" unitOfMeasureType: ").append(toIndentedString(unitOfMeasureType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy