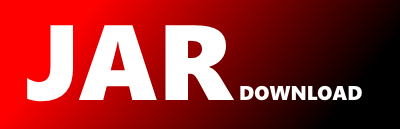
com.ziqni.admin.sdk.model.CreateWebhookRequestAllOf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* CreateWebhookRequestAllOf
*/
@JsonPropertyOrder({
CreateWebhookRequestAllOf.JSON_PROPERTY_POST_TO_URL,
CreateWebhookRequestAllOf.JSON_PROPERTY_TRIGGERS,
CreateWebhookRequestAllOf.JSON_PROPERTY_DESCRIPTION,
CreateWebhookRequestAllOf.JSON_PROPERTY_NAME,
CreateWebhookRequestAllOf.JSON_PROPERTY_HEADERS,
CreateWebhookRequestAllOf.JSON_PROPERTY_USERNAME,
CreateWebhookRequestAllOf.JSON_PROPERTY_PASSWORD
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class CreateWebhookRequestAllOf {
public static final String JSON_PROPERTY_POST_TO_URL = "postToUrl";
private String postToUrl;
public static final String JSON_PROPERTY_TRIGGERS = "triggers";
private List triggers = new ArrayList<>();
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_HEADERS = "headers";
private Map headers = null;
public static final String JSON_PROPERTY_USERNAME = "username";
private String username;
public static final String JSON_PROPERTY_PASSWORD = "password";
private String password;
public CreateWebhookRequestAllOf postToUrl(String postToUrl) {
this.postToUrl = postToUrl;
return this;
}
/**
* A URL to post the webhook to
* @return postToUrl
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "http://www.my-domain.com/abcd", required = true, value = "A URL to post the webhook to")
@JsonProperty(JSON_PROPERTY_POST_TO_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getPostToUrl() {
return postToUrl;
}
@JsonProperty(JSON_PROPERTY_POST_TO_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPostToUrl(String postToUrl) {
this.postToUrl = postToUrl;
}
public CreateWebhookRequestAllOf triggers(List triggers) {
this.triggers = triggers;
return this;
}
public CreateWebhookRequestAllOf addTriggersItem(String triggersItem) {
this.triggers.add(triggersItem);
return this;
}
/**
* A list of event triggers
* @return triggers
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "- com.ziqni.transformers.domain.CLWebhookTriggers.TriggersByClassName.getOrElse - onNewProductTrigger.getClass.getSimpleName.replace", required = true, value = "A list of event triggers")
@JsonProperty(JSON_PROPERTY_TRIGGERS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTriggers() {
return triggers;
}
@JsonProperty(JSON_PROPERTY_TRIGGERS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTriggers(List triggers) {
this.triggers = triggers;
}
public CreateWebhookRequestAllOf description(String description) {
this.description = description;
return this;
}
/**
* The description of a Webhook
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "http://www.my-domain.com/abcd", value = "The description of a Webhook")
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public CreateWebhookRequestAllOf name(String name) {
this.name = name;
return this;
}
/**
* The name of a Webhook
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "test", required = true, value = "The name of a Webhook")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public CreateWebhookRequestAllOf headers(Map headers) {
this.headers = headers;
return this;
}
public CreateWebhookRequestAllOf putHeadersItem(String key, String headersItem) {
if (this.headers == null) {
this.headers = new HashMap<>();
}
this.headers.put(key, headersItem);
return this;
}
/**
* The headers to add to the request
* @return headers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The headers to add to the request")
@JsonProperty(JSON_PROPERTY_HEADERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getHeaders() {
return headers;
}
@JsonProperty(JSON_PROPERTY_HEADERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHeaders(Map headers) {
this.headers = headers;
}
public CreateWebhookRequestAllOf username(String username) {
this.username = username;
return this;
}
/**
* Basic auth username
* @return username
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Basic auth username")
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUsername() {
return username;
}
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUsername(String username) {
this.username = username;
}
public CreateWebhookRequestAllOf password(String password) {
this.password = password;
return this;
}
/**
* Basic auth password
* @return password
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Basic auth password")
@JsonProperty(JSON_PROPERTY_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPassword() {
return password;
}
@JsonProperty(JSON_PROPERTY_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPassword(String password) {
this.password = password;
}
/**
* Return true if this CreateWebhookRequest_allOf object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateWebhookRequestAllOf createWebhookRequestAllOf = (CreateWebhookRequestAllOf) o;
return Objects.equals(this.postToUrl, createWebhookRequestAllOf.postToUrl) &&
Objects.equals(this.triggers, createWebhookRequestAllOf.triggers) &&
Objects.equals(this.description, createWebhookRequestAllOf.description) &&
Objects.equals(this.name, createWebhookRequestAllOf.name) &&
Objects.equals(this.headers, createWebhookRequestAllOf.headers) &&
Objects.equals(this.username, createWebhookRequestAllOf.username) &&
Objects.equals(this.password, createWebhookRequestAllOf.password);
}
@Override
public int hashCode() {
return Objects.hash(postToUrl, triggers, description, name, headers, username, password);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateWebhookRequestAllOf {\n");
sb.append(" postToUrl: ").append(toIndentedString(postToUrl)).append("\n");
sb.append(" triggers: ").append(toIndentedString(triggers)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" headers: ").append(toIndentedString(headers)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" password: ").append(toIndentedString(password)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy