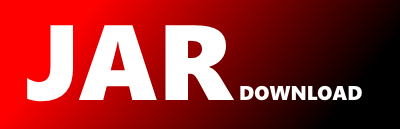
com.ziqni.admin.sdk.model.EntityStateChanged Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* EntityStateChanged
*/
@JsonPropertyOrder({
EntityStateChanged.JSON_PROPERTY_ENTITY_ID,
EntityStateChanged.JSON_PROPERTY_ENTITY_TYPE,
EntityStateChanged.JSON_PROPERTY_CHANGED_AT,
EntityStateChanged.JSON_PROPERTY_CHANGED_BY,
EntityStateChanged.JSON_PROPERTY_TYPE_OFF_CHANGE,
EntityStateChanged.JSON_PROPERTY_DISPLAY_NAME,
EntityStateChanged.JSON_PROPERTY_ACCOUNT_ID,
EntityStateChanged.JSON_PROPERTY_SEQUENCE_NUMBER,
EntityStateChanged.JSON_PROPERTY_ENTITY_REF_ID,
EntityStateChanged.JSON_PROPERTY_ENTITY_PARENT_ID,
EntityStateChanged.JSON_PROPERTY_METADATA,
EntityStateChanged.JSON_PROPERTY_PREVIOUS_STATE,
EntityStateChanged.JSON_PROPERTY_CURRENT_STATE
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class EntityStateChanged {
public static final String JSON_PROPERTY_ENTITY_ID = "entityId";
private String entityId;
public static final String JSON_PROPERTY_ENTITY_TYPE = "entityType";
private String entityType;
public static final String JSON_PROPERTY_CHANGED_AT = "changedAt";
private OffsetDateTime changedAt;
public static final String JSON_PROPERTY_CHANGED_BY = "changedBy";
private String changedBy;
public static final String JSON_PROPERTY_TYPE_OFF_CHANGE = "typeOffChange";
private Integer typeOffChange;
public static final String JSON_PROPERTY_DISPLAY_NAME = "displayName";
private String displayName;
public static final String JSON_PROPERTY_ACCOUNT_ID = "accountId";
private String accountId;
public static final String JSON_PROPERTY_SEQUENCE_NUMBER = "sequenceNumber";
private Long sequenceNumber;
public static final String JSON_PROPERTY_ENTITY_REF_ID = "entityRefId";
private String entityRefId;
public static final String JSON_PROPERTY_ENTITY_PARENT_ID = "entityParentId";
private String entityParentId;
public static final String JSON_PROPERTY_METADATA = "metadata";
private Object metadata;
public static final String JSON_PROPERTY_PREVIOUS_STATE = "previousState";
private Integer previousState;
public static final String JSON_PROPERTY_CURRENT_STATE = "currentState";
private Integer currentState;
public EntityStateChanged entityId(String entityId) {
this.entityId = entityId;
return this;
}
/**
* Get entityId
* @return entityId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENTITY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEntityId() {
return entityId;
}
@JsonProperty(JSON_PROPERTY_ENTITY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEntityId(String entityId) {
this.entityId = entityId;
}
public EntityStateChanged entityType(String entityType) {
this.entityType = entityType;
return this;
}
/**
* Get entityType
* @return entityType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEntityType() {
return entityType;
}
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEntityType(String entityType) {
this.entityType = entityType;
}
public EntityStateChanged changedAt(OffsetDateTime changedAt) {
this.changedAt = changedAt;
return this;
}
/**
* Get changedAt
* @return changedAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CHANGED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getChangedAt() {
return changedAt;
}
@JsonProperty(JSON_PROPERTY_CHANGED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setChangedAt(OffsetDateTime changedAt) {
this.changedAt = changedAt;
}
public EntityStateChanged changedBy(String changedBy) {
this.changedBy = changedBy;
return this;
}
/**
* Get changedBy
* @return changedBy
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CHANGED_BY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getChangedBy() {
return changedBy;
}
@JsonProperty(JSON_PROPERTY_CHANGED_BY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setChangedBy(String changedBy) {
this.changedBy = changedBy;
}
public EntityStateChanged typeOffChange(Integer typeOffChange) {
this.typeOffChange = typeOffChange;
return this;
}
/**
* Get typeOffChange
* @return typeOffChange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TYPE_OFF_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getTypeOffChange() {
return typeOffChange;
}
@JsonProperty(JSON_PROPERTY_TYPE_OFF_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTypeOffChange(Integer typeOffChange) {
this.typeOffChange = typeOffChange;
}
public EntityStateChanged displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get displayName
* @return displayName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDisplayName() {
return displayName;
}
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public EntityStateChanged accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAccountId() {
return accountId;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public EntityStateChanged sequenceNumber(Long sequenceNumber) {
this.sequenceNumber = sequenceNumber;
return this;
}
/**
* Get sequenceNumber
* @return sequenceNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SEQUENCE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSequenceNumber() {
return sequenceNumber;
}
@JsonProperty(JSON_PROPERTY_SEQUENCE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSequenceNumber(Long sequenceNumber) {
this.sequenceNumber = sequenceNumber;
}
public EntityStateChanged entityRefId(String entityRefId) {
this.entityRefId = entityRefId;
return this;
}
/**
* Get entityRefId
* @return entityRefId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENTITY_REF_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEntityRefId() {
return entityRefId;
}
@JsonProperty(JSON_PROPERTY_ENTITY_REF_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEntityRefId(String entityRefId) {
this.entityRefId = entityRefId;
}
public EntityStateChanged entityParentId(String entityParentId) {
this.entityParentId = entityParentId;
return this;
}
/**
* Get entityParentId
* @return entityParentId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENTITY_PARENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEntityParentId() {
return entityParentId;
}
@JsonProperty(JSON_PROPERTY_ENTITY_PARENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEntityParentId(String entityParentId) {
this.entityParentId = entityParentId;
}
public EntityStateChanged metadata(Object metadata) {
this.metadata = metadata;
return this;
}
/**
* Get metadata
* @return metadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Object getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(Object metadata) {
this.metadata = metadata;
}
public EntityStateChanged previousState(Integer previousState) {
this.previousState = previousState;
return this;
}
/**
* Get previousState
* @return previousState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PREVIOUS_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPreviousState() {
return previousState;
}
@JsonProperty(JSON_PROPERTY_PREVIOUS_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPreviousState(Integer previousState) {
this.previousState = previousState;
}
public EntityStateChanged currentState(Integer currentState) {
this.currentState = currentState;
return this;
}
/**
* Get currentState
* @return currentState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CURRENT_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getCurrentState() {
return currentState;
}
@JsonProperty(JSON_PROPERTY_CURRENT_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCurrentState(Integer currentState) {
this.currentState = currentState;
}
/**
* Return true if this EntityStateChanged object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EntityStateChanged entityStateChanged = (EntityStateChanged) o;
return Objects.equals(this.entityId, entityStateChanged.entityId) &&
Objects.equals(this.entityType, entityStateChanged.entityType) &&
Objects.equals(this.changedAt, entityStateChanged.changedAt) &&
Objects.equals(this.changedBy, entityStateChanged.changedBy) &&
Objects.equals(this.typeOffChange, entityStateChanged.typeOffChange) &&
Objects.equals(this.displayName, entityStateChanged.displayName) &&
Objects.equals(this.accountId, entityStateChanged.accountId) &&
Objects.equals(this.sequenceNumber, entityStateChanged.sequenceNumber) &&
Objects.equals(this.entityRefId, entityStateChanged.entityRefId) &&
Objects.equals(this.entityParentId, entityStateChanged.entityParentId) &&
Objects.equals(this.metadata, entityStateChanged.metadata) &&
Objects.equals(this.previousState, entityStateChanged.previousState) &&
Objects.equals(this.currentState, entityStateChanged.currentState);
}
@Override
public int hashCode() {
return Objects.hash(entityId, entityType, changedAt, changedBy, typeOffChange, displayName, accountId, sequenceNumber, entityRefId, entityParentId, metadata, previousState, currentState);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EntityStateChanged {\n");
sb.append(" entityId: ").append(toIndentedString(entityId)).append("\n");
sb.append(" entityType: ").append(toIndentedString(entityType)).append("\n");
sb.append(" changedAt: ").append(toIndentedString(changedAt)).append("\n");
sb.append(" changedBy: ").append(toIndentedString(changedBy)).append("\n");
sb.append(" typeOffChange: ").append(toIndentedString(typeOffChange)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" sequenceNumber: ").append(toIndentedString(sequenceNumber)).append("\n");
sb.append(" entityRefId: ").append(toIndentedString(entityRefId)).append("\n");
sb.append(" entityParentId: ").append(toIndentedString(entityParentId)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" previousState: ").append(toIndentedString(previousState)).append("\n");
sb.append(" currentState: ").append(toIndentedString(currentState)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy