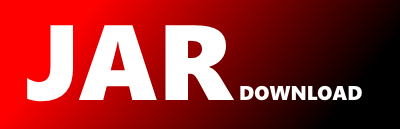
com.ziqni.admin.sdk.model.GoalMetrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* GoalMetrics
*/
@JsonPropertyOrder({
GoalMetrics.JSON_PROPERTY_ACCOUNT_ID,
GoalMetrics.JSON_PROPERTY_MEMBER_ID,
GoalMetrics.JSON_PROPERTY_ENTITY_ID,
GoalMetrics.JSON_PROPERTY_VALUE,
GoalMetrics.JSON_PROPERTY_PERCENTAGE_COMPLETE,
GoalMetrics.JSON_PROPERTY_MOST_SIGNIFICANT_SCORES,
GoalMetrics.JSON_PROPERTY_TIMESTAMP,
GoalMetrics.JSON_PROPERTY_UPDATE_COUNT,
GoalMetrics.JSON_PROPERTY_ENTITY_TYPE,
GoalMetrics.JSON_PROPERTY_MARKER_TIME_STAMP,
GoalMetrics.JSON_PROPERTY_GOAL_REACHED,
GoalMetrics.JSON_PROPERTY_STATUS_CODE,
GoalMetrics.JSON_PROPERTY_POSITION,
GoalMetrics.JSON_PROPERTY_USER_DEFINED_VALUES
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class GoalMetrics {
public static final String JSON_PROPERTY_ACCOUNT_ID = "accountId";
private String accountId;
public static final String JSON_PROPERTY_MEMBER_ID = "memberId";
private String memberId;
public static final String JSON_PROPERTY_ENTITY_ID = "entityId";
private String entityId;
public static final String JSON_PROPERTY_VALUE = "value";
private BigDecimal value;
public static final String JSON_PROPERTY_PERCENTAGE_COMPLETE = "percentageComplete";
private Double percentageComplete;
public static final String JSON_PROPERTY_MOST_SIGNIFICANT_SCORES = "mostSignificantScores";
private List mostSignificantScores = null;
public static final String JSON_PROPERTY_TIMESTAMP = "timestamp";
private Long timestamp;
public static final String JSON_PROPERTY_UPDATE_COUNT = "updateCount";
private Long updateCount;
public static final String JSON_PROPERTY_ENTITY_TYPE = "entityType";
private String entityType;
public static final String JSON_PROPERTY_MARKER_TIME_STAMP = "markerTimeStamp";
private Long markerTimeStamp;
public static final String JSON_PROPERTY_GOAL_REACHED = "goalReached";
private Boolean goalReached;
public static final String JSON_PROPERTY_STATUS_CODE = "statusCode";
private Integer statusCode;
public static final String JSON_PROPERTY_POSITION = "position";
private Integer position;
public static final String JSON_PROPERTY_USER_DEFINED_VALUES = "userDefinedValues";
private Map userDefinedValues = null;
public GoalMetrics accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The account identifier
* @return accountId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The account identifier")
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAccountId() {
return accountId;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public GoalMetrics memberId(String memberId) {
this.memberId = memberId;
return this;
}
/**
* The member identifier
* @return memberId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The member identifier")
@JsonProperty(JSON_PROPERTY_MEMBER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMemberId() {
return memberId;
}
@JsonProperty(JSON_PROPERTY_MEMBER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMemberId(String memberId) {
this.memberId = memberId;
}
public GoalMetrics entityId(String entityId) {
this.entityId = entityId;
return this;
}
/**
* The entity identifier
* @return entityId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The entity identifier")
@JsonProperty(JSON_PROPERTY_ENTITY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEntityId() {
return entityId;
}
@JsonProperty(JSON_PROPERTY_ENTITY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEntityId(String entityId) {
this.entityId = entityId;
}
public GoalMetrics value(BigDecimal value) {
this.value = value;
return this;
}
/**
* The current value assigned to this goal
* @return value
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The current value assigned to this goal")
@JsonProperty(JSON_PROPERTY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public BigDecimal getValue() {
return value;
}
@JsonProperty(JSON_PROPERTY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setValue(BigDecimal value) {
this.value = value;
}
public GoalMetrics percentageComplete(Double percentageComplete) {
this.percentageComplete = percentageComplete;
return this;
}
/**
* What percentage of this goal has been completed
* @return percentageComplete
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "What percentage of this goal has been completed")
@JsonProperty(JSON_PROPERTY_PERCENTAGE_COMPLETE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getPercentageComplete() {
return percentageComplete;
}
@JsonProperty(JSON_PROPERTY_PERCENTAGE_COMPLETE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPercentageComplete(Double percentageComplete) {
this.percentageComplete = percentageComplete;
}
public GoalMetrics mostSignificantScores(List mostSignificantScores) {
this.mostSignificantScores = mostSignificantScores;
return this;
}
public GoalMetrics addMostSignificantScoresItem(Double mostSignificantScoresItem) {
if (this.mostSignificantScores == null) {
this.mostSignificantScores = new ArrayList<>();
}
this.mostSignificantScores.add(mostSignificantScoresItem);
return this;
}
/**
* The most significant scores
* @return mostSignificantScores
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The most significant scores")
@JsonProperty(JSON_PROPERTY_MOST_SIGNIFICANT_SCORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMostSignificantScores() {
return mostSignificantScores;
}
@JsonProperty(JSON_PROPERTY_MOST_SIGNIFICANT_SCORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMostSignificantScores(List mostSignificantScores) {
this.mostSignificantScores = mostSignificantScores;
}
public GoalMetrics timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Internal timestamp associated with this goal
* @return timestamp
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Internal timestamp associated with this goal")
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getTimestamp() {
return timestamp;
}
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTimestamp(Long timestamp) {
this.timestamp = timestamp;
}
public GoalMetrics updateCount(Long updateCount) {
this.updateCount = updateCount;
return this;
}
/**
* A count of the total number of mutations this goal has undergone
* @return updateCount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A count of the total number of mutations this goal has undergone")
@JsonProperty(JSON_PROPERTY_UPDATE_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getUpdateCount() {
return updateCount;
}
@JsonProperty(JSON_PROPERTY_UPDATE_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUpdateCount(Long updateCount) {
this.updateCount = updateCount;
}
public GoalMetrics entityType(String entityType) {
this.entityType = entityType;
return this;
}
/**
* The type of entity
* @return entityType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of entity")
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEntityType() {
return entityType;
}
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEntityType(String entityType) {
this.entityType = entityType;
}
public GoalMetrics markerTimeStamp(Long markerTimeStamp) {
this.markerTimeStamp = markerTimeStamp;
return this;
}
/**
* A point in time marker
* @return markerTimeStamp
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A point in time marker")
@JsonProperty(JSON_PROPERTY_MARKER_TIME_STAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getMarkerTimeStamp() {
return markerTimeStamp;
}
@JsonProperty(JSON_PROPERTY_MARKER_TIME_STAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMarkerTimeStamp(Long markerTimeStamp) {
this.markerTimeStamp = markerTimeStamp;
}
public GoalMetrics goalReached(Boolean goalReached) {
this.goalReached = goalReached;
return this;
}
/**
* Has the minimum requirements been met
* @return goalReached
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Has the minimum requirements been met")
@JsonProperty(JSON_PROPERTY_GOAL_REACHED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getGoalReached() {
return goalReached;
}
@JsonProperty(JSON_PROPERTY_GOAL_REACHED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGoalReached(Boolean goalReached) {
this.goalReached = goalReached;
}
public GoalMetrics statusCode(Integer statusCode) {
this.statusCode = statusCode;
return this;
}
/**
* The system status assigned to this goal
* @return statusCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The system status assigned to this goal")
@JsonProperty(JSON_PROPERTY_STATUS_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getStatusCode() {
return statusCode;
}
@JsonProperty(JSON_PROPERTY_STATUS_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatusCode(Integer statusCode) {
this.statusCode = statusCode;
}
public GoalMetrics position(Integer position) {
this.position = position;
return this;
}
/**
* The position of this record in a sorted index if relevant
* @return position
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The position of this record in a sorted index if relevant")
@JsonProperty(JSON_PROPERTY_POSITION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPosition() {
return position;
}
@JsonProperty(JSON_PROPERTY_POSITION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPosition(Integer position) {
this.position = position;
}
public GoalMetrics userDefinedValues(Map userDefinedValues) {
this.userDefinedValues = userDefinedValues;
return this;
}
public GoalMetrics putUserDefinedValuesItem(String key, Double userDefinedValuesItem) {
if (this.userDefinedValues == null) {
this.userDefinedValues = new HashMap<>();
}
this.userDefinedValues.put(key, userDefinedValuesItem);
return this;
}
/**
* The user defined values for this goal
* @return userDefinedValues
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The user defined values for this goal")
@JsonProperty(JSON_PROPERTY_USER_DEFINED_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getUserDefinedValues() {
return userDefinedValues;
}
@JsonProperty(JSON_PROPERTY_USER_DEFINED_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserDefinedValues(Map userDefinedValues) {
this.userDefinedValues = userDefinedValues;
}
/**
* Return true if this GoalMetrics object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GoalMetrics goalMetrics = (GoalMetrics) o;
return Objects.equals(this.accountId, goalMetrics.accountId) &&
Objects.equals(this.memberId, goalMetrics.memberId) &&
Objects.equals(this.entityId, goalMetrics.entityId) &&
Objects.equals(this.value, goalMetrics.value) &&
Objects.equals(this.percentageComplete, goalMetrics.percentageComplete) &&
Objects.equals(this.mostSignificantScores, goalMetrics.mostSignificantScores) &&
Objects.equals(this.timestamp, goalMetrics.timestamp) &&
Objects.equals(this.updateCount, goalMetrics.updateCount) &&
Objects.equals(this.entityType, goalMetrics.entityType) &&
Objects.equals(this.markerTimeStamp, goalMetrics.markerTimeStamp) &&
Objects.equals(this.goalReached, goalMetrics.goalReached) &&
Objects.equals(this.statusCode, goalMetrics.statusCode) &&
Objects.equals(this.position, goalMetrics.position) &&
Objects.equals(this.userDefinedValues, goalMetrics.userDefinedValues);
}
@Override
public int hashCode() {
return Objects.hash(accountId, memberId, entityId, value, percentageComplete, mostSignificantScores, timestamp, updateCount, entityType, markerTimeStamp, goalReached, statusCode, position, userDefinedValues);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GoalMetrics {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" memberId: ").append(toIndentedString(memberId)).append("\n");
sb.append(" entityId: ").append(toIndentedString(entityId)).append("\n");
sb.append(" value: ").append(toIndentedString(value)).append("\n");
sb.append(" percentageComplete: ").append(toIndentedString(percentageComplete)).append("\n");
sb.append(" mostSignificantScores: ").append(toIndentedString(mostSignificantScores)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" updateCount: ").append(toIndentedString(updateCount)).append("\n");
sb.append(" entityType: ").append(toIndentedString(entityType)).append("\n");
sb.append(" markerTimeStamp: ").append(toIndentedString(markerTimeStamp)).append("\n");
sb.append(" goalReached: ").append(toIndentedString(goalReached)).append("\n");
sb.append(" statusCode: ").append(toIndentedString(statusCode)).append("\n");
sb.append(" position: ").append(toIndentedString(position)).append("\n");
sb.append(" userDefinedValues: ").append(toIndentedString(userDefinedValues)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy