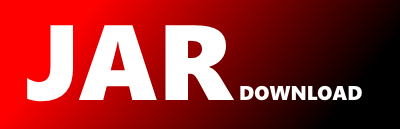
com.ziqni.admin.sdk.model.InstantWinAllOf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.ziqni.admin.sdk.model.InstantWinTile;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* InstantWinAllOf
*/
@JsonPropertyOrder({
InstantWinAllOf.JSON_PROPERTY_NAME,
InstantWinAllOf.JSON_PROPERTY_DESCRIPTION,
InstantWinAllOf.JSON_PROPERTY_TERMS_AND_CONDITIONS,
InstantWinAllOf.JSON_PROPERTY_CONSTRAINTS,
InstantWinAllOf.JSON_PROPERTY_STATUS_CODE,
InstantWinAllOf.JSON_PROPERTY_INSTANT_WIN_TYPE,
InstantWinAllOf.JSON_PROPERTY_ICON,
InstantWinAllOf.JSON_PROPERTY_BANNER,
InstantWinAllOf.JSON_PROPERTY_BANNER_LOW_RESOLUTION,
InstantWinAllOf.JSON_PROPERTY_BANNER_HIGH_RESOLUTION,
InstantWinAllOf.JSON_PROPERTY_TILES,
InstantWinAllOf.JSON_PROPERTY_VERSION,
InstantWinAllOf.JSON_PROPERTY_STYLE
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class InstantWinAllOf {
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_TERMS_AND_CONDITIONS = "termsAndConditions";
private String termsAndConditions;
public static final String JSON_PROPERTY_CONSTRAINTS = "constraints";
private List constraints = null;
public static final String JSON_PROPERTY_STATUS_CODE = "statusCode";
private Integer statusCode;
public static final String JSON_PROPERTY_INSTANT_WIN_TYPE = "instantWinType";
private Integer instantWinType;
public static final String JSON_PROPERTY_ICON = "icon";
private String icon;
public static final String JSON_PROPERTY_BANNER = "banner";
private String banner;
public static final String JSON_PROPERTY_BANNER_LOW_RESOLUTION = "bannerLowResolution";
private String bannerLowResolution;
public static final String JSON_PROPERTY_BANNER_HIGH_RESOLUTION = "bannerHighResolution";
private String bannerHighResolution;
public static final String JSON_PROPERTY_TILES = "tiles";
private List tiles = new ArrayList<>();
public static final String JSON_PROPERTY_VERSION = "version";
private Long version;
public static final String JSON_PROPERTY_STYLE = "style";
private String style;
public InstantWinAllOf name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public InstantWinAllOf description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public InstantWinAllOf termsAndConditions(String termsAndConditions) {
this.termsAndConditions = termsAndConditions;
return this;
}
/**
* Get termsAndConditions
* @return termsAndConditions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TERMS_AND_CONDITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTermsAndConditions() {
return termsAndConditions;
}
@JsonProperty(JSON_PROPERTY_TERMS_AND_CONDITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTermsAndConditions(String termsAndConditions) {
this.termsAndConditions = termsAndConditions;
}
public InstantWinAllOf constraints(List constraints) {
this.constraints = constraints;
return this;
}
public InstantWinAllOf addConstraintsItem(String constraintsItem) {
if (this.constraints == null) {
this.constraints = new ArrayList<>();
}
this.constraints.add(constraintsItem);
return this;
}
/**
* Get constraints
* @return constraints
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "hasRewards", value = "")
@JsonProperty(JSON_PROPERTY_CONSTRAINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getConstraints() {
return constraints;
}
@JsonProperty(JSON_PROPERTY_CONSTRAINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setConstraints(List constraints) {
this.constraints = constraints;
}
public InstantWinAllOf statusCode(Integer statusCode) {
this.statusCode = statusCode;
return this;
}
/**
* integer
* @return statusCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "integer")
@JsonProperty(JSON_PROPERTY_STATUS_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getStatusCode() {
return statusCode;
}
@JsonProperty(JSON_PROPERTY_STATUS_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatusCode(Integer statusCode) {
this.statusCode = statusCode;
}
public InstantWinAllOf instantWinType(Integer instantWinType) {
this.instantWinType = instantWinType;
return this;
}
/**
* integer
* @return instantWinType
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "integer")
@JsonProperty(JSON_PROPERTY_INSTANT_WIN_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getInstantWinType() {
return instantWinType;
}
@JsonProperty(JSON_PROPERTY_INSTANT_WIN_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setInstantWinType(Integer instantWinType) {
this.instantWinType = instantWinType;
}
public InstantWinAllOf icon(String icon) {
this.icon = icon;
return this;
}
/**
* Attachement id for the corresponding icon image.
* @return icon
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Attachement id for the corresponding icon image.")
@JsonProperty(JSON_PROPERTY_ICON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIcon() {
return icon;
}
@JsonProperty(JSON_PROPERTY_ICON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIcon(String icon) {
this.icon = icon;
}
public InstantWinAllOf banner(String banner) {
this.banner = banner;
return this;
}
/**
* Link to the banner
* @return banner
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "https://.cdn.ziqni.com/_id/", value = "Link to the banner")
@JsonProperty(JSON_PROPERTY_BANNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getBanner() {
return banner;
}
@JsonProperty(JSON_PROPERTY_BANNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBanner(String banner) {
this.banner = banner;
}
public InstantWinAllOf bannerLowResolution(String bannerLowResolution) {
this.bannerLowResolution = bannerLowResolution;
return this;
}
/**
* Link to the bannerLowResolution
* @return bannerLowResolution
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "https://.cdn.ziqni.com/_id/", value = "Link to the bannerLowResolution")
@JsonProperty(JSON_PROPERTY_BANNER_LOW_RESOLUTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getBannerLowResolution() {
return bannerLowResolution;
}
@JsonProperty(JSON_PROPERTY_BANNER_LOW_RESOLUTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBannerLowResolution(String bannerLowResolution) {
this.bannerLowResolution = bannerLowResolution;
}
public InstantWinAllOf bannerHighResolution(String bannerHighResolution) {
this.bannerHighResolution = bannerHighResolution;
return this;
}
/**
* Link to the bannerHighResolution
* @return bannerHighResolution
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "https://.cdn.ziqni.com/_id/", value = "Link to the bannerHighResolution")
@JsonProperty(JSON_PROPERTY_BANNER_HIGH_RESOLUTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getBannerHighResolution() {
return bannerHighResolution;
}
@JsonProperty(JSON_PROPERTY_BANNER_HIGH_RESOLUTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBannerHighResolution(String bannerHighResolution) {
this.bannerHighResolution = bannerHighResolution;
}
public InstantWinAllOf tiles(List tiles) {
this.tiles = tiles;
return this;
}
public InstantWinAllOf addTilesItem(InstantWinTile tilesItem) {
this.tiles.add(tilesItem);
return this;
}
/**
* Get tiles
* @return tiles
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_TILES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTiles() {
return tiles;
}
@JsonProperty(JSON_PROPERTY_TILES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTiles(List tiles) {
this.tiles = tiles;
}
public InstantWinAllOf version(Long version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getVersion() {
return version;
}
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVersion(Long version) {
this.version = version;
}
public InstantWinAllOf style(String style) {
this.style = style;
return this;
}
/**
* A link link to the cms entry for this objects style sheet
* @return style
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A link link to the cms entry for this objects style sheet")
@JsonProperty(JSON_PROPERTY_STYLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getStyle() {
return style;
}
@JsonProperty(JSON_PROPERTY_STYLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStyle(String style) {
this.style = style;
}
/**
* Return true if this InstantWin_allOf object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InstantWinAllOf instantWinAllOf = (InstantWinAllOf) o;
return Objects.equals(this.name, instantWinAllOf.name) &&
Objects.equals(this.description, instantWinAllOf.description) &&
Objects.equals(this.termsAndConditions, instantWinAllOf.termsAndConditions) &&
Objects.equals(this.constraints, instantWinAllOf.constraints) &&
Objects.equals(this.statusCode, instantWinAllOf.statusCode) &&
Objects.equals(this.instantWinType, instantWinAllOf.instantWinType) &&
Objects.equals(this.icon, instantWinAllOf.icon) &&
Objects.equals(this.banner, instantWinAllOf.banner) &&
Objects.equals(this.bannerLowResolution, instantWinAllOf.bannerLowResolution) &&
Objects.equals(this.bannerHighResolution, instantWinAllOf.bannerHighResolution) &&
Objects.equals(this.tiles, instantWinAllOf.tiles) &&
Objects.equals(this.version, instantWinAllOf.version) &&
Objects.equals(this.style, instantWinAllOf.style);
}
@Override
public int hashCode() {
return Objects.hash(name, description, termsAndConditions, constraints, statusCode, instantWinType, icon, banner, bannerLowResolution, bannerHighResolution, tiles, version, style);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InstantWinAllOf {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" termsAndConditions: ").append(toIndentedString(termsAndConditions)).append("\n");
sb.append(" constraints: ").append(toIndentedString(constraints)).append("\n");
sb.append(" statusCode: ").append(toIndentedString(statusCode)).append("\n");
sb.append(" instantWinType: ").append(toIndentedString(instantWinType)).append("\n");
sb.append(" icon: ").append(toIndentedString(icon)).append("\n");
sb.append(" banner: ").append(toIndentedString(banner)).append("\n");
sb.append(" bannerLowResolution: ").append(toIndentedString(bannerLowResolution)).append("\n");
sb.append(" bannerHighResolution: ").append(toIndentedString(bannerHighResolution)).append("\n");
sb.append(" tiles: ").append(toIndentedString(tiles)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" style: ").append(toIndentedString(style)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy