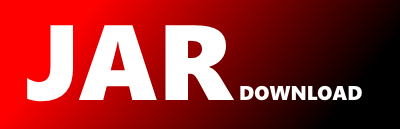
com.ziqni.admin.sdk.model.InstantWinTile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.ziqni.admin.sdk.model.GridLocation;
import com.ziqni.admin.sdk.model.RewardReduced;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* InstantWinTile
*/
@JsonPropertyOrder({
InstantWinTile.JSON_PROPERTY_ICON,
InstantWinTile.JSON_PROPERTY_TEXT,
InstantWinTile.JSON_PROPERTY_REWARD,
InstantWinTile.JSON_PROPERTY_LOCATION,
InstantWinTile.JSON_PROPERTY_PROBABILITY,
InstantWinTile.JSON_PROPERTY_BACKGROUND,
InstantWinTile.JSON_PROPERTY_CONTRAINTS
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class InstantWinTile {
public static final String JSON_PROPERTY_ICON = "icon";
private String icon;
public static final String JSON_PROPERTY_TEXT = "text";
private String text;
public static final String JSON_PROPERTY_REWARD = "reward";
private RewardReduced reward;
public static final String JSON_PROPERTY_LOCATION = "location";
private GridLocation location;
public static final String JSON_PROPERTY_PROBABILITY = "probability";
private Double probability;
public static final String JSON_PROPERTY_BACKGROUND = "background";
private String background;
public static final String JSON_PROPERTY_CONTRAINTS = "contraints";
private List contraints = null;
public InstantWinTile icon(String icon) {
this.icon = icon;
return this;
}
/**
* The id to the image file
* @return icon
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The id to the image file")
@JsonProperty(JSON_PROPERTY_ICON)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getIcon() {
return icon;
}
@JsonProperty(JSON_PROPERTY_ICON)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIcon(String icon) {
this.icon = icon;
}
public InstantWinTile text(String text) {
this.text = text;
return this;
}
/**
* Get text
* @return text
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getText() {
return text;
}
@JsonProperty(JSON_PROPERTY_TEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setText(String text) {
this.text = text;
}
public InstantWinTile reward(RewardReduced reward) {
this.reward = reward;
return this;
}
/**
* Get reward
* @return reward
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REWARD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public RewardReduced getReward() {
return reward;
}
@JsonProperty(JSON_PROPERTY_REWARD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReward(RewardReduced reward) {
this.reward = reward;
}
public InstantWinTile location(GridLocation location) {
this.location = location;
return this;
}
/**
* Get location
* @return location
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LOCATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public GridLocation getLocation() {
return location;
}
@JsonProperty(JSON_PROPERTY_LOCATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLocation(GridLocation location) {
this.location = location;
}
public InstantWinTile probability(Double probability) {
this.probability = probability;
return this;
}
/**
* Get probability
* @return probability
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_PROBABILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getProbability() {
return probability;
}
@JsonProperty(JSON_PROPERTY_PROBABILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProbability(Double probability) {
this.probability = probability;
}
public InstantWinTile background(String background) {
this.background = background;
return this;
}
/**
* Get background
* @return background
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_BACKGROUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getBackground() {
return background;
}
@JsonProperty(JSON_PROPERTY_BACKGROUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBackground(String background) {
this.background = background;
}
public InstantWinTile contraints(List contraints) {
this.contraints = contraints;
return this;
}
public InstantWinTile addContraintsItem(String contraintsItem) {
if (this.contraints == null) {
this.contraints = new ArrayList<>();
}
this.contraints.add(contraintsItem);
return this;
}
/**
* hasPrizes, glow
* @return contraints
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "hasPrizes", value = "hasPrizes, glow")
@JsonProperty(JSON_PROPERTY_CONTRAINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getContraints() {
return contraints;
}
@JsonProperty(JSON_PROPERTY_CONTRAINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContraints(List contraints) {
this.contraints = contraints;
}
/**
* Return true if this InstantWinTile object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InstantWinTile instantWinTile = (InstantWinTile) o;
return Objects.equals(this.icon, instantWinTile.icon) &&
Objects.equals(this.text, instantWinTile.text) &&
Objects.equals(this.reward, instantWinTile.reward) &&
Objects.equals(this.location, instantWinTile.location) &&
Objects.equals(this.probability, instantWinTile.probability) &&
Objects.equals(this.background, instantWinTile.background) &&
Objects.equals(this.contraints, instantWinTile.contraints);
}
@Override
public int hashCode() {
return Objects.hash(icon, text, reward, location, probability, background, contraints);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InstantWinTile {\n");
sb.append(" icon: ").append(toIndentedString(icon)).append("\n");
sb.append(" text: ").append(toIndentedString(text)).append("\n");
sb.append(" reward: ").append(toIndentedString(reward)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" probability: ").append(toIndentedString(probability)).append("\n");
sb.append(" background: ").append(toIndentedString(background)).append("\n");
sb.append(" contraints: ").append(toIndentedString(contraints)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy