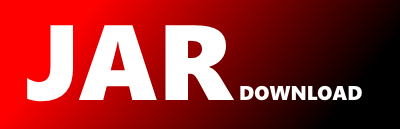
com.ziqni.admin.sdk.model.LeaderboardEntry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* ZIQNI Admin API
* Ziqni Application Services are used to manage and configure spaces. Change log: 2024-02-27 Added rewards reduced to the LeaderboardEntry response
*
* The version of the OpenAPI document: 3.0.17
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.ziqni.admin.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.ziqni.admin.sdk.model.LeaderboardMember;
import com.ziqni.admin.sdk.model.RewardReduced;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* LeaderboardEntry
*/
@JsonPropertyOrder({
LeaderboardEntry.JSON_PROPERTY_RANK,
LeaderboardEntry.JSON_PROPERTY_SCORE,
LeaderboardEntry.JSON_PROPERTY_BEST_SCORES,
LeaderboardEntry.JSON_PROPERTY_MEMBERS,
LeaderboardEntry.JSON_PROPERTY_REWARDS
})
@javax.annotation.processing.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class LeaderboardEntry {
public static final String JSON_PROPERTY_RANK = "rank";
private Integer rank;
public static final String JSON_PROPERTY_SCORE = "score";
private Double score;
public static final String JSON_PROPERTY_BEST_SCORES = "bestScores";
private List bestScores = null;
public static final String JSON_PROPERTY_MEMBERS = "members";
private List members = null;
public static final String JSON_PROPERTY_REWARDS = "rewards";
private List rewards = null;
public LeaderboardEntry rank(Integer rank) {
this.rank = rank;
return this;
}
/**
* The rank of the player
* @return rank
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "1", required = true, value = "The rank of the player")
@JsonProperty(JSON_PROPERTY_RANK)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getRank() {
return rank;
}
@JsonProperty(JSON_PROPERTY_RANK)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRank(Integer rank) {
this.rank = rank;
}
public LeaderboardEntry score(Double score) {
this.score = score;
return this;
}
/**
* The members current score
* @return score
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "100", value = "The members current score")
@JsonProperty(JSON_PROPERTY_SCORE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getScore() {
return score;
}
@JsonProperty(JSON_PROPERTY_SCORE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setScore(Double score) {
this.score = score;
}
public LeaderboardEntry bestScores(List bestScores) {
this.bestScores = bestScores;
return this;
}
public LeaderboardEntry addBestScoresItem(Double bestScoresItem) {
if (this.bestScores == null) {
this.bestScores = new ArrayList<>();
}
this.bestScores.add(bestScoresItem);
return this;
}
/**
* The multiplier to apply to source values received for this product action helper events
* @return bestScores
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "100", value = "The multiplier to apply to source values received for this product action helper events")
@JsonProperty(JSON_PROPERTY_BEST_SCORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getBestScores() {
return bestScores;
}
@JsonProperty(JSON_PROPERTY_BEST_SCORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBestScores(List bestScores) {
this.bestScores = bestScores;
}
public LeaderboardEntry members(List members) {
this.members = members;
return this;
}
public LeaderboardEntry addMembersItem(LeaderboardMember membersItem) {
if (this.members == null) {
this.members = new ArrayList<>();
}
this.members.add(membersItem);
return this;
}
/**
* Member details
* @return members
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Member details")
@JsonProperty(JSON_PROPERTY_MEMBERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMembers() {
return members;
}
@JsonProperty(JSON_PROPERTY_MEMBERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMembers(List members) {
this.members = members;
}
public LeaderboardEntry rewards(List rewards) {
this.rewards = rewards;
return this;
}
public LeaderboardEntry addRewardsItem(RewardReduced rewardsItem) {
if (this.rewards == null) {
this.rewards = new ArrayList<>();
}
this.rewards.add(rewardsItem);
return this;
}
/**
* The rewards associated with this rank
* @return rewards
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The rewards associated with this rank")
@JsonProperty(JSON_PROPERTY_REWARDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getRewards() {
return rewards;
}
@JsonProperty(JSON_PROPERTY_REWARDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRewards(List rewards) {
this.rewards = rewards;
}
/**
* Return true if this LeaderboardEntry object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LeaderboardEntry leaderboardEntry = (LeaderboardEntry) o;
return Objects.equals(this.rank, leaderboardEntry.rank) &&
Objects.equals(this.score, leaderboardEntry.score) &&
Objects.equals(this.bestScores, leaderboardEntry.bestScores) &&
Objects.equals(this.members, leaderboardEntry.members) &&
Objects.equals(this.rewards, leaderboardEntry.rewards);
}
@Override
public int hashCode() {
return Objects.hash(rank, score, bestScores, members, rewards);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LeaderboardEntry {\n");
sb.append(" rank: ").append(toIndentedString(rank)).append("\n");
sb.append(" score: ").append(toIndentedString(score)).append("\n");
sb.append(" bestScores: ").append(toIndentedString(bestScores)).append("\n");
sb.append(" members: ").append(toIndentedString(members)).append("\n");
sb.append(" rewards: ").append(toIndentedString(rewards)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy