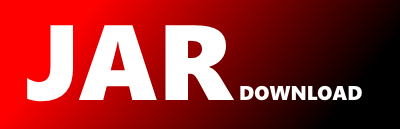
com.ziqni.admin.sdk.streaming.stomp.StompHeaders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ziqni-admin-sdk Show documentation
Show all versions of ziqni-admin-sdk Show documentation
ZIQNI Admin SDK Java Client
/*
* Copyright (c) 2024. ZIQNI LTD registered in England and Wales, company registration number-09693684
*/
package com.ziqni.admin.sdk.streaming.stomp;
import java.io.Serial;
import java.util.*;
import java.io.Serializable;
public class StompHeaders implements Serializable {
@Serial
private static final long serialVersionUID = 7514642206528452544L;
// Standard headers (as defined in the STOMP specification)
public static final String CONTENT_TYPE = "content-type";
public static final String CONTENT_LENGTH = "content-length";
public static final String RECEIPT = "receipt";
public static final String HOST = "host";
public static final String ACCEPT_VERSION = "accept-version";
public static final String LOGIN = "login";
public static final String PASSCODE = "passcode";
public static final String HEARTBEAT = "heart-beat";
public static final String SESSION = "session";
public static final String SERVER = "server";
public static final String DESTINATION = "destination";
public static final String ID = "id";
public static final String ACK = "ack";
public static final String SUBSCRIPTION = "subscription";
public static final String MESSAGE_ID = "message-id";
public static final String RECEIPT_ID = "receipt-id";
private final static String OBJECT_TYPE_KEY = "objectType";
private final Map> headers = new LinkedHashMap<>();
// Constructors
public StompHeaders() {
// Default constructor
}
public StompHeaders(String destination) {
setDestination(destination);
}
public StompHeaders(Map initialHeaders) {
initialHeaders.forEach((key, value) ->
headers.put(key, Collections.singletonList(value))
);
}
// Setters for standard headers
public void setContentType(String contentType) {
set(CONTENT_TYPE, contentType);
}
public String getContentType() {
return getFirst(CONTENT_TYPE);
}
public void setContentLength(long contentLength) {
set(CONTENT_LENGTH, String.valueOf(contentLength));
}
public long getContentLength() {
String value = getFirst(CONTENT_LENGTH);
return value != null ? Long.parseLong(value) : -1;
}
public void setReceipt(String receipt) {
set(RECEIPT, receipt);
}
public String getReceipt() {
return getFirst(RECEIPT);
}
public void setHost(String host) {
set(HOST, host);
}
public String getHost() {
return getFirst(HOST);
}
public void setAcceptVersion(String... versions) {
if (versions != null && versions.length > 0) {
set(ACCEPT_VERSION, String.join(",", versions));
}
}
public String[] getAcceptVersion() {
String value = getFirst(ACCEPT_VERSION);
return value != null ? value.split(",") : new String[0];
}
public void setLogin(String login) {
set(LOGIN, login);
}
public String getLogin() {
return getFirst(LOGIN);
}
public void setPasscode(String passcode) {
set(PASSCODE, passcode);
}
public String getPasscode() {
return getFirst(PASSCODE);
}
public void setHeartbeat(long send, long receive) {
if (send < 0 || receive < 0) {
throw new IllegalArgumentException("Heartbeat values must be non-negative.");
}
set(HEARTBEAT, String.format("%d,%d", send, receive));
}
public long[] getHeartbeat() {
String value = getFirst(HEARTBEAT);
if (value != null) {
String[] parts = value.split(",");
return new long[]{Long.parseLong(parts[0]), Long.parseLong(parts[1])};
}
return new long[]{0, 0};
}
public StompHeaders setDestination(String destination) {
set(DESTINATION, destination);
return this;
}
public String getDestination() {
return getFirst(DESTINATION);
}
public void setId(String id) {
set(ID, id);
}
public String getId() {
return getFirst(ID);
}
// Map-like operations
public void set(String headerName, String value) {
headers.put(headerName, Collections.singletonList(value));
}
public String getFirst(String headerName) {
List values = headers.get(headerName);
return (values != null && !values.isEmpty()) ? values.get(0) : null;
}
public void add(String headerName, String value) {
headers.computeIfAbsent(headerName, k -> new ArrayList<>()).add(value);
}
public List get(String headerName) {
return headers.getOrDefault(headerName, Collections.emptyList());
}
public Set keySet() {
return headers.keySet();
}
public Map> toMap() {
return Collections.unmodifiableMap(headers);
}
@Override
public String toString() {
return headers.toString();
}
public static StompHeaders fromMap(Map> source) {
StompHeaders headers = new StompHeaders();
headers.headers.putAll(source);
return headers;
}
public void setMessageId(String nextSeq) {
set(MESSAGE_ID, nextSeq);
}
public String getMessageId() {
return getFirst(MESSAGE_ID);
}
public String getReceiptId() {
return getFirst(RECEIPT_ID);
}
public void setReceiptId(String receiptId) {
set(RECEIPT_ID, receiptId);
}
public String getHeartBeat() {
return getFirst(HEARTBEAT);
}
public String getObjectType() {
return getFirst(OBJECT_TYPE_KEY);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy