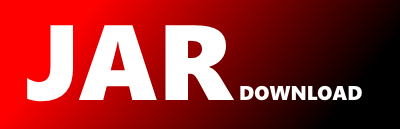
com.ziqni.transformer.test.concurrent.ZiqniConcurrentHashMap Maven / Gradle / Ivy
package com.ziqni.transformer.test.concurrent;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.TimeUnit;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
public class ZiqniConcurrentHashMap extends ConcurrentHashMap {
private static CompletableFuture completableFutureWrapper(Supplier supplier){
final var out = new CompletableFuture();
try{
out.complete(supplier.get());
}catch (Throwable t){
out.completeExceptionally(t);
}
return out;
}
public CompletableFuture> getAsync(Object key){
var result = Optional.ofNullable(this.get(key));
return CompletableFuture.supplyAsync(() -> result);
}
@Override
public V replace(K key, V value) {
return super.replace(key, value);
}
public CompletableFuture replaceAsync(K key, V value) {
final var out = new CompletableFuture();
out.complete(super.replace(key, value));
return out;
}
////////
@Override
public V computeIfAbsent(K key, Function super K, ? extends V> mappingFunction) {
return super.computeIfAbsent(key, mappingFunction);
}
public CompletableFuture computeIfAbsentAsync(K key, Function> mappingFunction) {
final var out = new CompletableFuture();
final var v = super.computeIfAbsent(key, k ->
mappingFunction
.apply(k)
.orTimeout(50, TimeUnit.SECONDS)
.join()
);
out.complete(v);
return out;
}
////////
@Override
public V computeIfPresent(K key, BiFunction super K, ? super V, ? extends V> remappingFunction) {
return super.computeIfPresent(key, remappingFunction);
}
public CompletableFuture computeIfPresentAsync(K key, BiFunction> mappingFunction) {
final var out = new CompletableFuture();
final var v = super.computeIfPresent(key,(k, v1) -> mappingFunction
.apply(k,v1)
.orTimeout(50, TimeUnit.SECONDS)
.join());
out.complete(v);
return out;
}
////////
@Override
public V compute(K key, BiFunction super K, ? super V, ? extends V> remappingFunction) {
return super.compute(key, remappingFunction);
}
public CompletableFuture computeAsync(K key, BiFunction> mappingFunction) {
final var out = new CompletableFuture();
final var v = super.computeIfPresent(key,(k, v1) -> mappingFunction
.apply(k,v1)
.orTimeout(50, TimeUnit.SECONDS)
.join());
out.complete(v);
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy