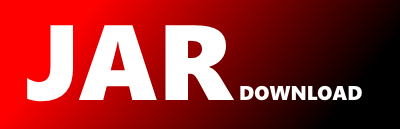
com.zoomlion.cloud.common.util.HttpClientUtils Maven / Gradle / Ivy
The newest version!
package com.zoomlion.cloud.common.util;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.http.HttpStatus;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
public class HttpClientUtils {
protected static Log log = LogFactory.getLog(HttpClientUtils.class);
public static String httpsRequest(String requestUrl, String requestMethod, Map params) {
if(requestMethod.equalsIgnoreCase("POST")){
return post(requestUrl, params, HttpClientFactory.getHttpsInstance(), false);
}
if(requestMethod.equalsIgnoreCase("GET")){
return get(requestUrl, HttpClientFactory.getHttpsInstance());
}
return null;
}
public static String httpRequest(String requestUrl, String requestMethod, Map params) {
if(requestMethod.equalsIgnoreCase("POST")){
return post(requestUrl, params, HttpClientFactory.getHttpInstance(), false);
}
if(requestMethod.equalsIgnoreCase("GET")){
return get(requestUrl, HttpClientFactory.getHttpInstance());
}
return null;
}
/**
* Post请求
* @param url 请求地址
* @param postData 参数
* @param noNeedResponse 不需要返回结果
* @return 返回字符串
*/
private static String post(String url, Map params, CloseableHttpClient httpClient, boolean noNeedResponse){
String responseData = null;
CloseableHttpResponse response = null;
try {
HttpPost method = new HttpPost(url);
if (null != params) {
//装填参数
List nvps = new ArrayList();
for (Entry entry : params.entrySet()) {
nvps.add(new BasicNameValuePair(entry.getKey(), entry.getValue()));
}
//设置参数到请求对象中
method.setEntity(new UrlEncodedFormEntity(nvps, "UTF-8"));
}
method.setHeader("Content-type", "application/x-www-form-urlencoded");
response = httpClient.execute(method);
/**请求发送成功,并得到响应**/
if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
/**读取服务器返回过来的字符串数据**/
responseData = EntityUtils.toString(response.getEntity(), "UTF-8");
if (noNeedResponse) {
return null;
}
}
} catch (IOException e) {
log.error("Post请求提交失败:" + url, e);
}catch (Exception e) {
log.error("http请求异常:{}", e);
}finally{
try {
if(response != null){
response.close();
}
if(httpClient != null) {
httpClient.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return responseData;
}
/**
* 发送Get请求
* @param url 请求地址
* @return 返回字符串
*/
private static String get(String url, CloseableHttpClient httpClient){
String responseData = null;
CloseableHttpResponse response = null;
try {
//发送get请求
HttpGet request = new HttpGet(url);
response = httpClient.execute(request);
/**请求发送成功,并得到响应**/
if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
/**读取服务器返回过来的json字符串数据**/
responseData = EntityUtils.toString(response.getEntity(), "UTF-8");
} else {
log.error("Get请求提交失败:" + url);
}
} catch (IOException e) {
log.error("Get请求提交失败:" + url, e);
}catch (Exception e) {
log.error("http请求异常:{}", e);
}finally{
try {
if(response != null){
response.close();
}
if(httpClient != null) {
httpClient.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return responseData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy