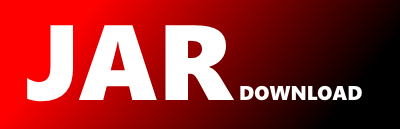
com.zoomlion.cloud.common.util.StringUtils Maven / Gradle / Ivy
package com.zoomlion.cloud.common.util;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
public class StringUtils {
/**
* An empty immutable String
array.
*/
public static final String[] EMPTY_STRING_ARRAY = new String[0];
/**
* 驼峰法转中划线
* @param name 驼峰类名 e.g. UserService
* @return 中划线 e.g. user-service
*/
public static String camel2Middleline(String name) {
StringBuilder result = new StringBuilder();
if (name != null && name.length() > 0) {
// 将第一个字符处理成大写
result.append(name.substring(0, 1).toLowerCase());
// 循环处理其余字符
for (int i = 1; i < name.length(); i++) {
String s = name.substring(i, i + 1);
// 在大写字母前添加中划线
if (s.equals(s.toUpperCase()) && !Character.isDigit(s.charAt(0))) {
result.append("-");
}
// 其他字符直接转成大写
result.append(s.toLowerCase());
}
}
return result.toString();
}
public static String toUnderscoreName(String name) {
if(name == null) return null;
String filteredName = name;
if(filteredName.indexOf("_") >= 0 && filteredName.equals(filteredName.toUpperCase())) {
filteredName = filteredName.toLowerCase();
}
if(filteredName.indexOf("_") == -1 && filteredName.equals(filteredName.toUpperCase())) {
filteredName = filteredName.toLowerCase();
}
StringBuffer result = new StringBuffer();
if (filteredName != null && filteredName.length() > 0) {
result.append(filteredName.substring(0, 1).toLowerCase());
for (int i = 1; i < filteredName.length(); i++) {
String preChart = filteredName.substring(i - 1, i);
String c = filteredName.substring(i, i + 1);
if(c.equals("_")) {
result.append("_");
continue;
}
if(preChart.equals("_")){
result.append(c.toLowerCase());
continue;
}
if(c.matches("\\d")) {
result.append(c);
}else if (c.equals(c.toUpperCase())) {
result.append("_");
result.append(c.toLowerCase());
}
else {
result.append(c);
}
}
}
return result.toString();
}
public static String makeAllWordFirstLetterUpperCase(String sqlName) {
String[] strs = sqlName.toLowerCase().split("_");
String result = "";
String preStr = "";
for(int i = 0; i < strs.length; i++) {
if(preStr.length() == 1) {
result += strs[i];
}else {
result += capitalize(strs[i]);
}
preStr = strs[i];
}
return result;
}
public static String capitalize(String str) {
return changeFirstCharacterCase(str, true);
}
private static String changeFirstCharacterCase(String str, boolean capitalize) {
if (str == null || str.length() == 0) {
return str;
}
StringBuffer buf = new StringBuffer(str.length());
if (capitalize) {
buf.append(Character.toUpperCase(str.charAt(0)));
}
else {
buf.append(Character.toLowerCase(str.charAt(0)));
}
buf.append(str.substring(1));
return buf.toString();
}
public static String uncapitalize(String str) {
return changeFirstCharacterCase(str, false);
}
/**
* Checks if a String is whitespace, empty ("") or null.
*
*
* StringUtils.isBlank(null) = true
* StringUtils.isBlank("") = true
* StringUtils.isBlank(" ") = true
* StringUtils.isBlank("bob") = false
* StringUtils.isBlank(" bob ") = false
*
*
* @param str the String to check, may be null
* @return true
if the String is null, empty or whitespace
* @since 2.0
*/
public static boolean isBlank(String str) {
int strLen;
if (str == null || (strLen = str.length()) == 0) {
return true;
}
for (int i = 0; i < strLen; i++) {
if ((Character.isWhitespace(str.charAt(i)) == false)) {
return false;
}
}
return true;
}
/**
* Checks if a String is not empty (""), not null and not whitespace only.
*
*
* StringUtils.isNotBlank(null) = false
* StringUtils.isNotBlank("") = false
* StringUtils.isNotBlank(" ") = false
* StringUtils.isNotBlank("bob") = true
* StringUtils.isNotBlank(" bob ") = true
*
*
* @param str the String to check, may be null
* @return true
if the String is
* not empty and not null and not whitespace
* @since 2.0
*/
public static boolean isNotBlank(String str) {
return !StringUtils.isBlank(str);
}
// Splitting
//-----------------------------------------------------------------------
/**
* Splits the provided text into an array, using whitespace as the
* separator.
* Whitespace is defined by {@link Character#isWhitespace(char)}.
*
* The separator is not included in the returned String array.
* Adjacent separators are treated as one separator.
* For more control over the split use the StrTokenizer class.
*
* A null
input String returns null
.
*
*
* StringUtils.split(null) = null
* StringUtils.split("") = []
* StringUtils.split("abc def") = ["abc", "def"]
* StringUtils.split("abc def") = ["abc", "def"]
* StringUtils.split(" abc ") = ["abc"]
*
*
* @param str the String to parse, may be null
* @return an array of parsed Strings, null
if null String input
*/
public static String[] split(String str) {
return split(str, null, -1);
}
/**
* Splits the provided text into an array, separator specified.
* This is an alternative to using StringTokenizer.
*
* The separator is not included in the returned String array.
* Adjacent separators are treated as one separator.
* For more control over the split use the StrTokenizer class.
*
* A null
input String returns null
.
*
*
* StringUtils.split(null, *) = null
* StringUtils.split("", *) = []
* StringUtils.split("a.b.c", '.') = ["a", "b", "c"]
* StringUtils.split("a..b.c", '.') = ["a", "b", "c"]
* StringUtils.split("a:b:c", '.') = ["a:b:c"]
* StringUtils.split("a b c", ' ') = ["a", "b", "c"]
*
*
* @param str the String to parse, may be null
* @param separatorChar the character used as the delimiter
* @return an array of parsed Strings, null
if null String input
* @since 2.0
*/
public static String[] split(String str, char separatorChar) {
return splitWorker(str, separatorChar, false);
}
/**
* Performs the logic for the split
and
* splitPreserveAllTokens
methods that do not return a
* maximum array length.
*
* @param str the String to parse, may be null
* @param separatorChar the separate character
* @param preserveAllTokens if true
, adjacent separators are
* treated as empty token separators; if false
, adjacent
* separators are treated as one separator.
* @return an array of parsed Strings, null
if null String input
*/
private static String[] splitWorker(String str, char separatorChar, boolean preserveAllTokens) {
// Performance tuned for 2.0 (JDK1.4)
if (str == null) {
return null;
}
int len = str.length();
if (len == 0) {
return EMPTY_STRING_ARRAY;
}
List list = new ArrayList();
int i = 0, start = 0;
boolean match = false;
boolean lastMatch = false;
while (i < len) {
if (str.charAt(i) == separatorChar) {
if (match || preserveAllTokens) {
list.add(str.substring(start, i));
match = false;
lastMatch = true;
}
start = ++i;
continue;
}
lastMatch = false;
match = true;
i++;
}
if (match || (preserveAllTokens && lastMatch)) {
list.add(str.substring(start, i));
}
return (String[]) list.toArray(new String[list.size()]);
}
/**
* Splits the provided text into an array with a maximum length,
* separators specified.
*
* The separator is not included in the returned String array.
* Adjacent separators are treated as one separator.
*
* A null
input String returns null
.
* A null
separatorChars splits on whitespace.
*
* If more than max
delimited substrings are found, the last
* returned string includes all characters after the first max - 1
* returned strings (including separator characters).
*
*
* StringUtils.split(null, *, *) = null
* StringUtils.split("", *, *) = []
* StringUtils.split("ab de fg", null, 0) = ["ab", "cd", "ef"]
* StringUtils.split("ab de fg", null, 0) = ["ab", "cd", "ef"]
* StringUtils.split("ab:cd:ef", ":", 0) = ["ab", "cd", "ef"]
* StringUtils.split("ab:cd:ef", ":", 2) = ["ab", "cd:ef"]
*
*
* @param str the String to parse, may be null
* @param separatorChars the characters used as the delimiters,
* null
splits on whitespace
* @param max the maximum number of elements to include in the
* array. A zero or negative value implies no limit
* @return an array of parsed Strings, null
if null String input
*/
public static String[] split(String str, String separatorChars, int max) {
return splitWorker(str, separatorChars, max, false);
}
/**
* Performs the logic for the split
and
* splitPreserveAllTokens
methods that return a maximum array
* length.
*
* @param str the String to parse, may be null
* @param separatorChars the separate character
* @param max the maximum number of elements to include in the
* array. A zero or negative value implies no limit.
* @param preserveAllTokens if true
, adjacent separators are
* treated as empty token separators; if false
, adjacent
* separators are treated as one separator.
* @return an array of parsed Strings, null
if null String input
*/
private static String[] splitWorker(String str, String separatorChars, int max, boolean preserveAllTokens) {
// Performance tuned for 2.0 (JDK1.4)
// Direct code is quicker than StringTokenizer.
// Also, StringTokenizer uses isSpace() not isWhitespace()
if (str == null) {
return null;
}
int len = str.length();
if (len == 0) {
return EMPTY_STRING_ARRAY;
}
List list = new ArrayList();
int sizePlus1 = 1;
int i = 0, start = 0;
boolean match = false;
boolean lastMatch = false;
if (separatorChars == null) {
// Null separator means use whitespace
while (i < len) {
if (Character.isWhitespace(str.charAt(i))) {
if (match || preserveAllTokens) {
lastMatch = true;
if (sizePlus1++ == max) {
i = len;
lastMatch = false;
}
list.add(str.substring(start, i));
match = false;
}
start = ++i;
continue;
}
lastMatch = false;
match = true;
i++;
}
} else if (separatorChars.length() == 1) {
// Optimise 1 character case
char sep = separatorChars.charAt(0);
while (i < len) {
if (str.charAt(i) == sep) {
if (match || preserveAllTokens) {
lastMatch = true;
if (sizePlus1++ == max) {
i = len;
lastMatch = false;
}
list.add(str.substring(start, i));
match = false;
}
start = ++i;
continue;
}
lastMatch = false;
match = true;
i++;
}
} else {
// standard case
while (i < len) {
if (separatorChars.indexOf(str.charAt(i)) >= 0) {
if (match || preserveAllTokens) {
lastMatch = true;
if (sizePlus1++ == max) {
i = len;
lastMatch = false;
}
list.add(str.substring(start, i));
match = false;
}
start = ++i;
continue;
}
lastMatch = false;
match = true;
i++;
}
}
if (match || (preserveAllTokens && lastMatch)) {
list.add(str.substring(start, i));
}
return (String[]) list.toArray(new String[list.size()]);
}
public static String lowerCase(String str) {
if (str == null) {
return null;
}
return str.toLowerCase();
}
/**
* Compares two Strings, returning true
if they are equal ignoring
* the case.
*
* null
s are handled without exceptions. Two null
* references are considered equal. Comparison is case insensitive.
*
*
* StringUtils.equalsIgnoreCase(null, null) = true
* StringUtils.equalsIgnoreCase(null, "abc") = false
* StringUtils.equalsIgnoreCase("abc", null) = false
* StringUtils.equalsIgnoreCase("abc", "abc") = true
* StringUtils.equalsIgnoreCase("abc", "ABC") = true
*
*
* @see java.lang.String#equalsIgnoreCase(String)
* @param str1 the first String, may be null
* @param str2 the second String, may be null
* @return true
if the Strings are equal, case insensitive, or
* both null
*/
public static boolean equalsIgnoreCase(String str1, String str2) {
return str1 == null ? str2 == null : str1.equalsIgnoreCase(str2);
}
private static final String EMPTY_STRING = "";
public static String join(String[] array) {
return join(Arrays.asList(array));
}
public static void join(String[] array, char separator, StringBuilder sb) {
join(Arrays.asList(array), separator, sb);
}
public static String join(Collection collection) {
return join(collection, ',');
}
public static String join(Collection collection, char separator) {
// Shortcut
if (collection.isEmpty()) {
return EMPTY_STRING;
}
StringBuilder result = new StringBuilder();
join(collection, separator, result);
return result.toString();
}
public static void join(Iterable iterable, char separator, StringBuilder sb) {
join(iterable, separator,
new Function() {
@Override public String apply(String t) { return t; }}, sb);
}
public static void join(T[] array, char separator, Function function,
StringBuilder sb) {
join(Arrays.asList(array), separator, function, sb);
}
public static void join(Iterable iterable, char separator, Function function,
StringBuilder sb) {
boolean first = true;
for (T value : iterable) {
if (first) {
first = false;
} else {
sb.append(separator);
}
sb.append(function.apply(value));
}
}
public interface Function {
public String apply(T t);
}
}