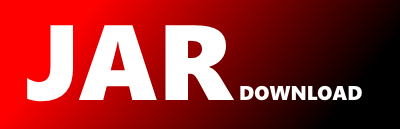
com.zuora.JSON Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapter;
import com.google.gson.internal.bind.util.ISO8601Utils;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.google.gson.JsonElement;
import io.gsonfire.GsonFireBuilder;
import io.gsonfire.TypeSelector;
import okio.ByteString;
import java.io.IOException;
import java.io.StringReader;
import java.lang.reflect.Type;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.ParsePosition;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.Date;
import java.util.Locale;
import java.util.Map;
import java.util.HashMap;
import java.util.Arrays;
import java.util.List;
import com.google.gson.ExclusionStrategy;
import com.google.gson.FieldAttributes;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Set;
/*
* A JSON utility class
*
* NOTE: in the future, this class may be converted to static, which may break
* backward-compatibility
*/
public class JSON {
private static Gson gson;
private static boolean isLenientOnJson = false;
private static DateTypeAdapter dateTypeAdapter = new DateTypeAdapter();
private static SqlDateTypeAdapter sqlDateTypeAdapter = new SqlDateTypeAdapter();
private static OffsetDateTimeTypeAdapter offsetDateTimeTypeAdapter = new OffsetDateTimeTypeAdapter();
private static LocalDateTypeAdapter localDateTypeAdapter = new LocalDateTypeAdapter();
private static ByteArrayAdapter byteArrayAdapter = new ByteArrayAdapter();
@SuppressWarnings("unchecked")
public static GsonBuilder createGson() {
GsonFireBuilder fireBuilder = new GsonFireBuilder()
.registerTypeSelector(com.zuora.model.BulkCreateCreditMemosRequest.class, new TypeSelector() {
@Override
public Class extends com.zuora.model.BulkCreateCreditMemosRequest> getClassForElement(JsonElement readElement) {
Map classByDiscriminatorValue = new HashMap();
classByDiscriminatorValue.put("Invoice", com.zuora.model.BulkCreateCreditMemosFromInvoiceRequest.class);
classByDiscriminatorValue.put("Standalone", com.zuora.model.BulkCreateCreditMemosFromChargeRequest.class);
classByDiscriminatorValue.put("BulkCreateCreditMemosFromChargeRequest", com.zuora.model.BulkCreateCreditMemosFromChargeRequest.class);
classByDiscriminatorValue.put("BulkCreateCreditMemosFromInvoiceRequest", com.zuora.model.BulkCreateCreditMemosFromInvoiceRequest.class);
classByDiscriminatorValue.put("BulkCreateCreditMemosRequest", com.zuora.model.BulkCreateCreditMemosRequest.class);
return getClassByDiscriminator(classByDiscriminatorValue,
getDiscriminatorValue(readElement, "sourceType"));
}
})
.registerTypeSelector(com.zuora.model.BulkCreateDebitMemosRequest.class, new TypeSelector() {
@Override
public Class extends com.zuora.model.BulkCreateDebitMemosRequest> getClassForElement(JsonElement readElement) {
Map classByDiscriminatorValue = new HashMap();
classByDiscriminatorValue.put("Invoice", com.zuora.model.BulkCreateDebitMemosFromInvoiceRequest.class);
classByDiscriminatorValue.put("Standalone", com.zuora.model.BulkCreateDebitMemosFromChargeRequest.class);
classByDiscriminatorValue.put("BulkCreateDebitMemosFromChargeRequest", com.zuora.model.BulkCreateDebitMemosFromChargeRequest.class);
classByDiscriminatorValue.put("BulkCreateDebitMemosFromInvoiceRequest", com.zuora.model.BulkCreateDebitMemosFromInvoiceRequest.class);
classByDiscriminatorValue.put("BulkCreateDebitMemosRequest", com.zuora.model.BulkCreateDebitMemosRequest.class);
return getClassByDiscriminator(classByDiscriminatorValue,
getDiscriminatorValue(readElement, "sourceType"));
}
})
;
GsonBuilder builder = fireBuilder.createGsonBuilder();
return builder;
}
private static String getDiscriminatorValue(JsonElement readElement, String discriminatorField) {
JsonElement element = readElement.getAsJsonObject().get(discriminatorField);
if (null == element) {
throw new IllegalArgumentException("missing discriminator field: <" + discriminatorField + ">");
}
return element.getAsString();
}
/**
* Returns the Java class that implements the OpenAPI schema for the specified discriminator value.
*
* @param classByDiscriminatorValue The map of discriminator values to Java classes.
* @param discriminatorValue The value of the OpenAPI discriminator in the input data.
* @return The Java class that implements the OpenAPI schema
*/
private static Class getClassByDiscriminator(Map classByDiscriminatorValue, String discriminatorValue) {
Class clazz = (Class) classByDiscriminatorValue.get(discriminatorValue);
if (null == clazz) {
throw new IllegalArgumentException("cannot determine model class of name: <" + discriminatorValue + ">");
}
return clazz;
}
{
GsonBuilder gsonBuilder = createGson();
gsonBuilder.registerTypeAdapter(Date.class, dateTypeAdapter);
gsonBuilder.registerTypeAdapter(java.sql.Date.class, sqlDateTypeAdapter);
gsonBuilder.registerTypeAdapter(OffsetDateTime.class, offsetDateTimeTypeAdapter);
gsonBuilder.registerTypeAdapter(LocalDate.class, localDateTypeAdapter);
gsonBuilder.registerTypeAdapter(byte[].class, byteArrayAdapter);
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountBasicInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountBillingAndPayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountCreditCardHolder.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountData.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountDetailResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountEInvoiceProfile.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountPMMandateInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryBasicInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryDefaultPaymentMethod.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryPayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryPaymentInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummarySubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountSummaryUsage.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AccountingCodeItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ActionsErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AddSubscriptionComponent.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AddSubscriptionRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApiVolumeSummaryRecord.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyCreditMemoItemToDebitMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyCreditMemoItemToInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyCreditMemoToDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyCreditMemoToInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyPaymentDebitMemoApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyPaymentDebitMemoApplicationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyPaymentInvoiceApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyPaymentInvoiceApplicationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ApplyPaymentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AsyncOperationResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AsyncOrderJobResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AsyncOrderProcessingOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AsyncOrderResultSubscriptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AuthenticationErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AuthenticationFailResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.AuthenticationSuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BadRequestErrors.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BadRequestResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsColumnsDescriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsColumnsErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsColumnsServerErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsCountSuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsNoDataResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsServerErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsStatusErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsStatusServerErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsTaskStatusResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Bi3ViewsV2SuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BiViews1ErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BiViews1SelectSuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BiViews1SuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillRun.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillRunFilter.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillRunSchedule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillingAdjustment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillingAdjustmentExclusion.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillingDocVolumeSummaryRecord.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillingDocumentQueryResponseElement.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillingOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BillingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BookingDateJob.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateCreditMemosFromChargeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateCreditMemosFromInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateCreditMemosRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateDebitMemosFromChargeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateDebitMemosFromInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateDebitMemosRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateInvoicesRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreateInvoicesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkCreditMemosResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkDebitMemosResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkPdfGenerationJobRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkPdfGenerationJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkPostInvoicesRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkPostInvoicesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkUpdateCreditMemosRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkUpdateDebitMemosRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkUpdateInvoicesRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkUpdateOrderLineItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BulkUpdateOrderLineItemsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BusinessRegion.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.BusinessRegionsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CalloutAuth.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelAuthorizationRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelAuthorizationResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelBillRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelBillRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelBillingAdjustmentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelBillingAdjustmentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelOrderRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelOrderResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelPaymentSchedule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CancelSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CatalogGroup.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CatalogGroupProductRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChangeLogField.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChangePlanRatePlanOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChangeSubscriptionRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeModelConfiguration.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeModelConfigurationForSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeModelConfigurationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeModelDataOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeOverrideBilling.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeOverridePricing.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeTier.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChargeUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ChildrenSettingValueRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CollectDebitMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CollectDebitMemoRequestPayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CollectDebitMemoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CollectDebitMemoResponseAppliedCreditMemos.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CollectDebitMemoResponseAppliedPayments.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CollectDebitMemoResponseDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CollectDebitMemoResponseProcessedPayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CommonErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CommonResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CompareSchemaInfoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CompareSchemaKeyValue.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ConfigTemplateErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ConfigTemplateErrorResponseReasonsInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ConfigurationTemplateContent.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Contact.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ContactInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ContactResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ContactSnapshot.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ContactSnapshotResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountContact.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountCreditCard.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountCreditCardHolderInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountPaymentMethod.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountingCodeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountingCodeResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountingPeriodRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAccountingPeriodResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAuthorizationRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAuthorizationResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAuthorizationResponsePaymentGateway.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateAuthorizationResponseReasons.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBatchQueryJobRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBatchQueryJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBatchQueryRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBatchQueryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingAdjustmentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingDocumentFilesDeletionJobRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingDocumentFilesDeletionJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingPreviewCreditMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingPreviewInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingPreviewRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingPreviewResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingPreviewRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateBillingPreviewRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateCatalogGroupRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateContactRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateCreditMemoFromCharge.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateCreditMemoFromChargeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateCreditMemoFromInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateCreditMemoFromInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateCreditMemoTaxationItemsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateDebitMemoFromCharge.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateDebitMemoFromChargeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateDebitMemoFromInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateDebitMemosFromInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateDiscountItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillmentItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillmentItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillmentItemsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillmentItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillmentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillmentsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateFulfillmentsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceCollectCreditMemos.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceCollectInvoices.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceCollectRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceCollectResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceScheduleItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateInvoiceScheduleRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateJournalEntryRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateJournalEntryRequestItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateJournalEntryRequestSegment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateJournalEntryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateJournalRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateJournalRunRequestTransactionType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateJournalRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateMassUpdateResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateMassUpdaterRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateNonRefRefundFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOpenPaymentMethodTypeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrUpdateCatalogGroupProductRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrUpdateEmailTemplatesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderAddProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderAsyncRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderAsyncResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderChangePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderChangePlanRatePlanOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderChargeUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderCreateSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderOrderLineItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderProductOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderProductRatePlanOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderRatePlanFeatureOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderRatePlanOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderRatePlanUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponseOrderAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponseOrderLineItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponseOrderMetric.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponseRamps.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponseRefunds.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponseSubscriptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResponseWriteOff.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderResume.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderSubscriptionOwnerAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderSuspend.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderTermsAndConditions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateOrderUpdateProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentDebitMemoApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentDebitMemoApplicationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentInvoiceApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentInvoiceApplicationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodACH.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodApplePayAdyen.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodBankTransfer.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodBankTransferAccountHolderInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodCCReferenceTransaction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodCardholderInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodCommon.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodCreditCard.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodDecryptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodDecryptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodGooglePayAdyenChase.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodPayPalAdaptive.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodPayPalECPayPalNativeECPayPalCP.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodResponseReason.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodUpdaterBatchRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodUpdaterBatchResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentMethodUpdaterBatchResponseReasons.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentRunData.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentRunDataItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentScheduleItemsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentScheduleItemsRequestItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentScheduleRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentScheduleRequestItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentSchedulesRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentSchedulesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentSchedulesResponseItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentSessionInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentSessionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreatePaymentSessionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateProductRatePlanChargeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateProductRatePlanRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateProductRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateRSASignatureRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateRamp.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateRefundRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateRefundwithAutoUnapply.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSequenceSetRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSequenceSetsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSequenceSetsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateStoredCredentialProfileRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSubscribeToProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSubscriptionComponent.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSubscriptionRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItemForCreditMemoFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItemForCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItemForDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItemForDebitMemoFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItemForInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItemsForDebitMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTaxationItemsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTemplateRequestContent.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateTokenRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateUpdateOpenPaymentMethodTypeResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateUpdateStoredCredentialProfileResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreateUsageRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditCard.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoEntityPrefix.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemFromChargeDetail.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemFromChargeDetailFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemFromInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemFromInvoiceItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemFromWriteOffInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemPart.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoPart.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoTaxItemFromInvoiceTaxItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoTaxItemFromInvoiceTaxItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemoTaxationItemFromWriteOffInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CreditMemosResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomAccountPaymentMethod.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectAllFieldsDefinition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectAllFieldsDefinitionAllOfCreatedById.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectAllFieldsDefinitionAllOfCreatedDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectAllFieldsDefinitionAllOfId.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectAllFieldsDefinitionAllOfUpdatedById.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectAllFieldsDefinitionAllOfUpdatedDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkDeleteFilter.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkDeleteFilterCondition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkJobErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkJobErrorResponseCollection.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkJobRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkJobResponseCollection.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectBulkJobResponseError.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectCustomFieldDefinition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectCustomFieldDefinitionUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectCustomFieldsDefinition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectDefinition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectDefinitionSchema.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectDefinitionUpdateActionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectDefinitionUpdateActionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordBatchAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordBatchRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordWithAllFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordWithOnlyCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordsBatchUpdatePartialSuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordsErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordsThrottledResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomObjectRecordsWithError.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.CustomRates.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DailyConsumptionRevRecRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DataQueryErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DataQueryJob.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DataQueryJobCancelled.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DataQueryJobCommon.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoDueDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoEntityPrefix.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemFromChargeDetail.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemFromChargeDetailFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemFromInvoiceItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemFromInvoiceItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemTaxationItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemWriteOffRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoTaxItemFromInvoiceTaxItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoTaxItemFromInvoiceTaxItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemoTaxationItemWriteOffRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DebitMemosResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DecryptRSASignatureRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DecryptRSASignatureResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteAccountResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteAttachmentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteBatchQueryJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteBatchQueryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteDataQueryJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteWorkflowError.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeleteWorkflowSuccess.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeletedRecord.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeliverySchedule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeliveryScheduleParams.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DeliveryScheduleProductRatePlanCharge.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DetailedWorkflow.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DiscountApplyDetail.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DiscountPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DiscountPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.DocumentIdList.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ElectronicPaymentOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ElectronicPaymentOptionsWithDelayedCapturePayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.EmailBillRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.EmailBillingDocumentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.EndConditions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Error401.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ErrorResponse401Record.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ErrorResponseReasonsInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.EventTrigger.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.EventType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExecuteInvoiceScheduleRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedAmendment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedBillingRun.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedContact.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedCreditMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedCreditMemoApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedCreditMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedDailyConsumptionSummary.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedDebitMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedExternalProductRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedOrderAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedOrderLineItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedOrders.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPaymentApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPaymentMethod.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPaymentMethodSnapshot.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPaymentRun.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPaymentSchedule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPaymentScheduleItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPrepaidBalance.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPrepaidBalanceFund.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedPrepaidBalanceTransaction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedProcessedUsage.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedProductRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedProductRatePlanCharge.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedProductRatePlanChargeTier.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedRatePlanCharge.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedRatePlanChargeTier.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedRatingResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedRefund.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedRefundApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedRefundApplicationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedTaxationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedUsage.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExpandedValidityPeriodSummary.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ExportWorkflowVersionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.FailedReason.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.FaultResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.FilterRuleParameterDefinition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Fulfillment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.FulfillmentItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GETBookingDateBackfillJobById200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GETDataBackfillJobById200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GETListBookingDateBackfillJobs200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GETListDataBackfillJobs200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GatewayOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GenerateBillingDocumentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GenerateBillingDocumentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GenerateBillingDocumentResponseCreditMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GenerateBillingDocumentResponseInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountPMAccountHolderInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountPaymentMethodResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountingCodeResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountingCodesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountingPeriodAllOfFieIdsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountingPeriodResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountingPeriodWithoutSuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAccountingPeriodsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAllCustomObjectDefinitionsInNamespaceResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetApiVolumeSummaryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAsyncOrderJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAttachmentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetAttachmentsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBIViewStatus200ResponseInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBatchQueryJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBatchQueryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBillingAdjustmentsBySubscriptionNumberResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBillingAdjustmentsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBillingDocVolumeSummaryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBillingDocumentFilesDeletionJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBillingDocumentsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBillingPreviewRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBulkPdfGenerationJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetBulkUpdateOrderLineItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCalloutHistoryVOType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCalloutHistoryVOsType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCatalogGroupResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCatalogGroupsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetChargeOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoItemPartResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoItemPartsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoItemTaxationItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoPartResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoPartsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoPdfStatusBatchResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoPdfStatusResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoTaxItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoTaxItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCreditMemoTaxationItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCustomExchangeRatesDataType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetCustomExchangeRatesType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDataQueryJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDataQueryJobsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoApplicationPart.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoApplicationPartsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoPdfStatusBatchResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoPdfStatusResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoTaxItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoTaxItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetDebitMemoTaxItemNew.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetEmailHistoryVOType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetEmailHistoryVOsType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetEventTriggers200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetFulfillment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetFulfillmentItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetFulfillmentItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetFulfillmentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetInvoiceApplicationPartRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetInvoiceApplicationPartsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetInvoicePdfStatusBatchResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetInvoicePdfStatusResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetJournalEntriesInJournalRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetJournalEntryDetailResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetJournalEntryDetailTypeWithoutSuccess.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetJournalEntryItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetJournalEntrySegmentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetJournalRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetJournalRunTransactionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetMassUpdateResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetMetricsBySubscriptionNumbersResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetOrderActionRatePlanResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetOrderLineItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetOrderLineItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetOrderResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetOrdersResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPMAccountHolderInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentItemPartResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentItemPartsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentMethodForAccountResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentMethodResponseACHForAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentMethodResponseApplePayForAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentMethodResponseBankTransferForAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentMethodResponseCreditCardForAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentMethodResponseGooglePayForAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentMethodResponsePayPalForAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentPartResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentPartsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentRunDataArrayResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentRunDataElementResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentRunDataTransactionElementResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentRunStatisticResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentRunSummaryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentRunSummaryTotalValuesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentRunsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentScheduleStatisticResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentScheduleStatisticResponsePaymentScheduleItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentSchedulesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentVolumeSummaryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPaymentsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetProductRatePlanChargeResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetProductRatePlanChargeTierResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetProductRatePlanResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetProductRatePlansByExternalIdResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetProductRatePlansByProductResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetProductResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetProductsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPublicEmailTemplateResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPublicNotificationDefinitionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPublicNotificationDefinitionResponseCallout.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetPublicNotificationDefinitionResponseFilterRule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetQueryEmailTemplates200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetQueryNotificationDefinitions200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRampByRampNumberResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRampMetricsByOrderNumberResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRampMetricsByRampNumberResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRampMetricsBySubscriptionKeyResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRampsBySubscriptionKeyResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRefundCreditMemoFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRefundItemPartResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRefundItemPartsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRefundPartResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRefundPartsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetRefundsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetScheduledEventResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetScheduledEventResponseParametersValue.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetScheduledEvents200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSequenceSetResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSequenceSetsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSubscriptionChangeLogResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSubscriptionChangeLogsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSubscriptionProductFeature.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSubscriptionRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSubscriptionRatePlanChargesWithAllSegments.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetSubscriptionsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetTaxationItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetTaxationItemsOfCreditMemoItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetTaxationItemsOfDebitMemoItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetTaxationItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetUsageRateDetailRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetUsageRateDetailRequestData.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetUsageResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetUsagesByAccountResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetVersionsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetWorkflowResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetWorkflowResponseTasks.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetWorkflowsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.GetWorkflowsResponsePagination.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.HostedPageResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.HostedPagesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.IneligibleBillingAdjustment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InitialTerm.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.IntervalPricing.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.IntervalPricingTier.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Invoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceEntityPrefix.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceFile.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceFilesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceItemObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceItemPreviewResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceItemPreviewResultAdditionalInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceItemPreviewResultTaxationItems.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoicePostResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceSchedule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceScheduleCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceScheduleItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceScheduleItemCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceScheduleResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceScheduleSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.InvoiceTaxationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Job.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.LastTerm.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Linkage.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ListAllSettingsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ListOfExchangeRates.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.MappingResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.MigrationClientResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.MigrationComponentContent.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.MigrationUpdateCustomObjectDefinitionsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.MigrationUpdateCustomObjectDefinitionsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.NotificationsHistoryDeletionTaskResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ObjectPostImportRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OneTimeFlatFeePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OneTimePerUnitPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OneTimeTieredPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OneTimeVolumePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OpenPaymentMethodTypeRequestFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OpenPaymentMethodTypeResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OpenPaymentMethodTypeResponseFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OperationJobResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Options.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Order.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionAddProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionCancelSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionChangePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionCreateSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionCreateSubscriptionTerms.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionOwnerTransfer.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanAmendment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanBillingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanChargeModelDataOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanChargeOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanChargeRemove.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanChargeUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanOrder.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanOrderAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanRecurringDeliveryPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanRecurringDeliveryPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanRecurringFlatFeePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanRecurringPerUnitPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanRecurringTieredPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanRecurringVolumePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanRemoveProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRatePlanUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRemoveProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionRenewSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionResume.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionSuspend.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionTermsAndConditions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderActionUpdateProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderCreateAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderCreateAccountContact.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderDeltaMetric.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderDeltaMrr.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderDeltaTcb.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderDeltaTcv.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderExistingAccountDetails.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderLineItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderMetric.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderRampIntervalMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderRampMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderSchedulingOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderSubscriptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrderSubscriptionsSubscriptionOwnerAccountDetails.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OrganizationLabel.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.OverrideDiscountApplyDetail.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PATCHUpdateWorkflowRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.POSTCreateBookingDateBackfillJob200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.POSTCreateDataBackfillJob200Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.POSTCreateDataBackfillJobRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PUTStopBookingDateBackfillJobByIdRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Payment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentData.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentEntityPrefix.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentGatewaysResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentGatwayResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentItemPart.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodObjectCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodObjectCustomFieldsForAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodRequestMandateInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodRequestProcessingOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponseACH.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponseApplePay.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponseBankTransfer.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponseCreditCard.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponseGooglePay.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponseMandateInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodResponsePayPal.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodSnapshotResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodTransactionLogResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodUpdaterInstanceResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentMethodUpdaterInstancesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentPart.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentRequestFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentRun.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentRunResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentSchedule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentScheduleBillingDocument.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentScheduleItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentScheduleItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentScheduleLinkedPaymentID.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentSchedulePaymentOptionFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentSchedulePaymentOptionFieldsDetail.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentScheduleResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentTransactionLogResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentVolumeSummaryRecord.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PaymentWithCustomRates.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostAsyncInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostAttachmentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostAttachmentsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostBillRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostBusinessRegionsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCompareTemplateRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCreateOrUpdateEmailTemplateRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCreateOrUpdateEmailTemplateRequestFormat.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCustomObjectDefinitionFieldDefinitionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCustomObjectDefinitionsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCustomObjectDefinitionsRequestDefinition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCustomObjectRecordsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostCustomObjectRecordsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostEventTriggerRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostPublicEmailTemplateRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostPublicNotificationDefinitionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostPublicNotificationDefinitionRequestCallout.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostPublicNotificationDefinitionRequestFilterRule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostResendCalloutNotifications.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostResendEmailNotifications.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostRunWorkflow400Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostRunWorkflow406Response.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostScheduledEventRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostScheduledEventRequestParametersValue.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostServiceProviderRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostWorkflowDefinitionImportRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PostWorkflowVersionsImportRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewAccountInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewChargeMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewChargeMetricsCmrr.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewChargeMetricsTax.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewChargeMetricsTcb.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewChargeMetricsTcv.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewContactInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewExistingSubscriptionCreditMemoItemResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewExistingSubscriptionDiscountDetails.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewExistingSubscriptionInvoiceItemResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewExistingSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewExistingSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewExistingSubscriptionResultCreditMemos.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewExistingSubscriptionResultInvoices.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderAsyncRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderAsyncResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderChargeOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderChargeUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderCreateSubscription.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderCreateSubscriptionTerms.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderOrderAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderRatePlanOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderRatePlanUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderResultChargeMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderResultCreditMemos.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderResultDeltaMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderResultInvoices.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderSubscriptionOwnerAccount.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewOrderSubscriptionsAsync.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewPaymentSchedule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewResultOrderMetricsInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewResultOrderMetricsInnerOrderActionsInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewStartDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewSubscriptionAccountInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewSubscriptionBillToContact.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewSubscriptionInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewSubscriptionInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PreviewThroughDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PriceChangeParams.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProcessingOptions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProcessingOptionsWithDelayedCapturePayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Product.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductFeature.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductRatePlanCharge.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductRatePlanChargeObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductRatePlanChargeTier.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductRatePlanChargeTierData.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductRatePlanObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProductRatePlanWithExternalId.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActioncreateRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActiondeleteRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActionqueryMoreRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActionqueryMoreRequestConf.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActionqueryMoreResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActionqueryRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActionqueryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyActionupdateRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyBadRequestResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyBadRequestResponseErrorsInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyCreateOrModifyResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyCreateTaxationItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyDeleteResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyGetImport.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyNoDataResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyPostImport.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ProxyUnauthorizedResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutAttachmentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutBusinessRegionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutEventTriggerRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutEventTriggerRequestEventType.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutPublicEmailTemplateRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutPublicNotificationDefinitionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutPublicNotificationDefinitionRequestCallout.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutPublicNotificationDefinitionRequestFilterRule.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutRevproAccCodeResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutScheduledEventRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutServiceProviderRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.PutTasksRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QuantityForUsageCharges.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryAccountsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryAmendmentsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryBillingRunsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryContactsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryCreditMemoApplicationsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryCreditMemoItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryCreditMemosResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryCustomObjectRecordsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryCustomObjectsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryDailyConsumptionSummarysResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryDebitMemoItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryDebitMemosResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryInvoiceItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryInvoicesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryOrderActionsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryOrderLineItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryOrderssResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPaymentApplicationsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPaymentMethodSnapshotsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPaymentMethodsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPaymentRunsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPaymentScheduleItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPaymentSchedulesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPaymentsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPrepaidBalanceFundsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPrepaidBalanceTransactionsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryPrepaidBalancesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryProcessedUsagesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryProductRatePlanChargeTiersResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryProductRatePlanChargesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryProductRatePlansResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryProductsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryRatePlanChargeTiersResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryRatePlanChargesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryRatePlansResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryRatingResultsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryRefundApplicationItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryRefundApplicationsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryRefundsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QuerySubscriptionsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryTaxationItemsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryUsagesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QueryValidityPeriodSummarysResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.QuoteObjectFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RSASignatureResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampChargeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampChargeResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalChargeDeltaMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalChargeDeltaMetricsDeltaMrrInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalChargeDeltaMetricsDeltaQuantityInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalChargeMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalChargeMetricsMrrInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampIntervalResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RampResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanChangeLog.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanChargeChangeLog.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanChargeObjectCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanChargeOverridePricing.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanChargeSegment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanChargeSegmentInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanChargeTier.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanFeatureOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanObjectCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatePlanUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RatingPropertiesOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReconcileRefundRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringDeliveryPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringDeliveryPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringFlatFeePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringFlatFeePricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringPerUnitPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringPerUnitPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringTieredPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringTieredPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringVolumePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RecurringVolumePricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Refund.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundCreditMemoItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundCreditMemoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundEntityPrefix.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundItemPart.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundObjectCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundPart.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundRequestFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RefundResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RegenerateBillingRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RegenerateBookingRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RegenerateRevRecEventsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RegenerateTransactionObjectResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RejectPaymentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RemoveCatalogGroupProductRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RemoveSubscriptionRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RenewSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RenewSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RenewalTerm.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReportDownloadErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReportFileNotExistsResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReportListErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReportListResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ResendCalloutNotificationsFailedResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ResendCalloutNotificationsFailedResponseValue.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ResendEmailNotificationsFailedResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ResendEmailNotificationsFailedResponseValue.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ResumeSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ResumeSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RetryPaymentScheduleItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RetryPaymentScheduleItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RetryPaymentScheduleItemResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseCreditMemoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseCreditMemoResponseCreditMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseCreditMemoResponseDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseInvoiceResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseInvoiceResponseCreditMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReverseInvoiceResponseDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ReversePaymentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RevertOrderRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RevertOrderResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.RevproAccountingCodes.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SaveResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ServiceProvider.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ServiceProviderResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingComponentKeyValue.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingItemHttpOperation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingItemHttpRequestParameter.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingItemWithOperationsInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingSourceComponentResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingValueRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingValueResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingValueResponseWrapper.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingsBatchRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettingsBatchResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SettlePaymentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpCreatePMPayPalECPayPalNativeEC.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpCreatePaymentMethodCardholderInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpCreatePaymentMethodCommon.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpCreatePaymentMethodCreditCard.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpCreatePaymentMethodCreditCardReferenceTransaction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpCreatePaymentMethodPayPalAdaptive.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpPaymentMethod.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpPaymentMethodObjectCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpResponseReasons.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignUpTaxInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignedUrlInvalidReportResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignedUrlReportNotFoundResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SignedUrlSuccessResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.StageErrorNoRecordResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.StageErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.StageErrorResponseResultInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.StoredCredentialProfileResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.StoredCredentialProfilesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubmitDataQueryRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubmitDataQueryRequestOutput.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubmitDataQueryResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscribeToProduct.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionChangeLog.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionCreditMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionCreditMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionData.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionObjectNSFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionObjectQTFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionProductFeature.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionStatusHistory.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SubscriptionTaxationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SuspendSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SuspendSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.SystemHealthErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Task.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TasksResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TasksResponsePagination.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TaxInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TaxItemsFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TaxationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TaxationItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TaxationItemsData.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TaxationItemsDataResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TemplateDetailResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TemplateMigrationClientRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TemplateResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TermInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Tier.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TimeSlicedElpNetMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TimeSlicedMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TimeSlicedNetMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TimeSlicedTcbNetMetrics.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TokenResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TransferPayment.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TriggerDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.TriggerParams.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyCreditMemoItemToDebitMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyCreditMemoItemToInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyCreditMemoToDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyCreditMemoToInvoice.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyPaymentDebitMemoApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyPaymentDebitMemoApplicationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyPaymentInvoiceApplication.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyPaymentInvoiceApplicationItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UnapplyPaymentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateAccountContact.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateAccountRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateAccountingCodeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateAccountingPeriodRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateBasicSummaryJournalEntryRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateCatalogGroupRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateContactRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateCreditMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateCreditMemoTaxItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateCreditMemoTaxItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateCreditMemoWithId.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateCustomObjectCusotmField.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateDebitMemoItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateDebitMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateDebitMemoTaxItemFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateDebitMemoTaxItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateDebitMemoWithId.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateDebitMemosDueDatesRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateDiscountInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateFulfillmentItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateFulfillmentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateInvoiceItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateInvoiceResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateInvoiceScheduleItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateInvoiceScheduleRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateJournalEntryItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOpenPaymentMethodTypeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderAction.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderActionChargeTriggerDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderActionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderActionTriggerDate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderActionTriggerDates.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderActionTriggerDatesRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderCustomFieldsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderLineItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderLineItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderSubscriptionsCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderSubscriptionsOrderActionsCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderTriggerDateResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderTriggerDatesRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateOrderTriggerDatesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdatePaymentMethodRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdatePaymentMethodRequestCreditCardInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdatePaymentMethodResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdatePaymentRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdatePaymentRunRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdatePaymentScheduleItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdatePaymentScheduleRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateProductRatePlanChargeRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateProductRatePlanChargeTierRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateProductRatePlanRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateProductRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateRatePlanCharge.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateRefundRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSequenceSetRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSequenceSetResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionChargeCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionChargeCustomFieldsOfASpecifiedVersion.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionCustomFieldsOfASpecifiedVersionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionCustomFieldsRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionRatePlan.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionRatePlanCustomFields.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionRatePlanCustomFieldsOfASpecifiedVersion.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateSubscriptionResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateTask.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateTaxationItemForFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateTaxationItemRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdateUsageRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UpdaterPaymentMethodRequestAccountHolderInfo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadCsvErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadCsvErrorStatusResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadCsvResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadCsvResponseResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadCsvStatusResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadCsvStatusResponseResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileErrorResponseResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileForCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileForDebitMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileForInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileResponse2.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileResponseResult.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileStatusErrorResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileStatusNoContent.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileStatusResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadFileStatusResponseResultInner.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadUsageFileRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UploadUsageFileResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Usage.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageFlatFeePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageFlatFeePricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageItem.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageOveragePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageOveragePricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsagePerUnitPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsagePerUnitPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageTieredPricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageTieredPricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageTieredWithOveragePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageTieredWithOveragePricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageValues.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageVolumePricingOverride.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsageVolumePricingUpdate.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.UsagesResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ValidationErrors.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ValidationReasons.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.VerifyPaymentMethodRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.VerifyPaymentMethodResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.Workflow.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WorkflowDefinition.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WorkflowDefinitionActiveVersion.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WorkflowDefinitionAndVersions.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WorkflowError.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WorkflowInstance.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffBehavior.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffBehaviorFinanceInformation.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffCreditMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffCreditMemoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffCreditMemoResponseDebitMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffDebitMemoRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffDebitMemoResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffInvoiceRequest.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffInvoiceResponse.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.WriteOffInvoiceResponseCreditMemo.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ZObject.CustomTypeAdapterFactory());
gsonBuilder.registerTypeAdapterFactory(new com.zuora.model.ZObjectUpdate.CustomTypeAdapterFactory());
gsonBuilder.addSerializationExclusionStrategy(new ZuoraExclusionStrategy());
gsonBuilder.addDeserializationExclusionStrategy(new ZuoraExclusionStrategy());
gson = gsonBuilder.create();
}
/**
* Get Gson.
*
* @return Gson
*/
public static Gson getGson() {
return gson;
}
/**
* Set Gson.
*
* @param gson Gson
*/
public static void setGson(Gson gson) {
JSON.gson = gson;
}
public static void setLenientOnJson(boolean lenientOnJson) {
isLenientOnJson = lenientOnJson;
}
/**
* Serialize the given Java object into JSON string.
*
* @param obj Object
* @return String representation of the JSON
*/
public static String serialize(Object obj) {
return gson.toJson(obj);
}
/**
* Deserialize the given JSON string to Java object.
*
* @param Type
* @param body The JSON string
* @param returnType The type to deserialize into
* @return The deserialized Java object
*/
@SuppressWarnings("unchecked")
public static T deserialize(String body, Type returnType) {
try {
if (isLenientOnJson) {
JsonReader jsonReader = new JsonReader(new StringReader(body));
// see https://google-gson.googlecode.com/svn/trunk/gson/docs/javadocs/com/google/gson/stream/JsonReader.html#setLenient(boolean)
jsonReader.setLenient(true);
return gson.fromJson(jsonReader, returnType);
} else {
return gson.fromJson(body, returnType);
}
} catch (JsonParseException e) {
// Fallback processing when failed to parse JSON form response body:
// return the response body string directly for the String return type;
if (returnType.equals(String.class)) {
return (T) body;
} else {
throw (e);
}
}
}
/**
* Gson TypeAdapter for Byte Array type
*/
public static class ByteArrayAdapter extends TypeAdapter {
@Override
public void write(JsonWriter out, byte[] value) throws IOException {
if (value == null) {
out.nullValue();
} else {
out.value(ByteString.of(value).base64());
}
}
@Override
public byte[] read(JsonReader in) throws IOException {
switch (in.peek()) {
case NULL:
in.nextNull();
return null;
default:
String bytesAsBase64 = in.nextString();
ByteString byteString = ByteString.decodeBase64(bytesAsBase64);
return byteString.toByteArray();
}
}
}
/**
* Gson TypeAdapter for JSR310 OffsetDateTime type
*/
public static class OffsetDateTimeTypeAdapter extends TypeAdapter {
private DateTimeFormatter formatter;
public OffsetDateTimeTypeAdapter() {
this(DateTimeFormatter.ISO_OFFSET_DATE_TIME);
}
public OffsetDateTimeTypeAdapter(DateTimeFormatter formatter) {
this.formatter = formatter;
}
public void setFormat(DateTimeFormatter dateFormat) {
this.formatter = dateFormat;
}
@Override
public void write(JsonWriter out, OffsetDateTime date) throws IOException {
if (date == null) {
out.nullValue();
} else {
out.value(formatter.format(date));
}
}
@Override
public OffsetDateTime read(JsonReader in) throws IOException {
switch (in.peek()) {
case NULL:
in.nextNull();
return null;
default:
String date = in.nextString();
if (date.endsWith("+0000")) {
date = date.substring(0, date.length()-5) + "Z";
}
return OffsetDateTime.parse(date, formatter);
}
}
}
/**
* Gson TypeAdapter for JSR310 LocalDate type
*/
public static class LocalDateTypeAdapter extends TypeAdapter {
private DateTimeFormatter formatter;
public LocalDateTypeAdapter() {
this(DateTimeFormatter.ISO_LOCAL_DATE);
}
public LocalDateTypeAdapter(DateTimeFormatter formatter) {
this.formatter = formatter;
}
public void setFormat(DateTimeFormatter dateFormat) {
this.formatter = dateFormat;
}
@Override
public void write(JsonWriter out, LocalDate date) throws IOException {
if (date == null) {
out.nullValue();
} else {
out.value(formatter.format(date));
}
}
@Override
public LocalDate read(JsonReader in) throws IOException {
switch (in.peek()) {
case NULL:
in.nextNull();
return null;
default:
String date = in.nextString();
return LocalDate.parse(date, formatter);
}
}
}
public static void setOffsetDateTimeFormat(DateTimeFormatter dateFormat) {
offsetDateTimeTypeAdapter.setFormat(dateFormat);
}
public static void setLocalDateFormat(DateTimeFormatter dateFormat) {
localDateTypeAdapter.setFormat(dateFormat);
}
/**
* Gson TypeAdapter for java.sql.Date type
* If the dateFormat is null, a simple "yyyy-MM-dd" format will be used
* (more efficient than SimpleDateFormat).
*/
public static class SqlDateTypeAdapter extends TypeAdapter {
private DateFormat dateFormat;
public SqlDateTypeAdapter() {}
public SqlDateTypeAdapter(DateFormat dateFormat) {
this.dateFormat = dateFormat;
}
public void setFormat(DateFormat dateFormat) {
this.dateFormat = dateFormat;
}
@Override
public void write(JsonWriter out, java.sql.Date date) throws IOException {
if (date == null) {
out.nullValue();
} else {
String value;
if (dateFormat != null) {
value = dateFormat.format(date);
} else {
value = date.toString();
}
out.value(value);
}
}
@Override
public java.sql.Date read(JsonReader in) throws IOException {
switch (in.peek()) {
case NULL:
in.nextNull();
return null;
default:
String date = in.nextString();
try {
if (dateFormat != null) {
return new java.sql.Date(dateFormat.parse(date).getTime());
}
return new java.sql.Date(ISO8601Utils.parse(date, new ParsePosition(0)).getTime());
} catch (ParseException e) {
throw new JsonParseException(e);
}
}
}
}
/**
* Gson TypeAdapter for java.util.Date type
* If the dateFormat is null, ISO8601Utils will be used.
*/
public static class DateTypeAdapter extends TypeAdapter {
private DateFormat dateFormat;
public DateTypeAdapter() {}
public DateTypeAdapter(DateFormat dateFormat) {
this.dateFormat = dateFormat;
}
public void setFormat(DateFormat dateFormat) {
this.dateFormat = dateFormat;
}
@Override
public void write(JsonWriter out, Date date) throws IOException {
if (date == null) {
out.nullValue();
} else {
String value;
if (dateFormat != null) {
value = dateFormat.format(date);
} else {
value = ISO8601Utils.format(date, true);
}
out.value(value);
}
}
@Override
public Date read(JsonReader in) throws IOException {
try {
switch (in.peek()) {
case NULL:
in.nextNull();
return null;
default:
String date = in.nextString();
try {
if (dateFormat != null) {
return dateFormat.parse(date);
}
return ISO8601Utils.parse(date, new ParsePosition(0));
} catch (ParseException e) {
throw new JsonParseException(e);
}
}
} catch (IllegalArgumentException e) {
throw new JsonParseException(e);
}
}
}
public static void setDateFormat(DateFormat dateFormat) {
dateTypeAdapter.setFormat(dateFormat);
}
public static void setSqlDateFormat(DateFormat dateFormat) {
sqlDateTypeAdapter.setFormat(dateFormat);
}
class ZuoraExclusionStrategy implements ExclusionStrategy {
final HashMap> processingFields = new HashMap<>();
@Override
public boolean shouldSkipField(FieldAttributes field) {
final String className = field.getDeclaringClass().getTypeName();
final String name = field.getName();
if (!isZuoraClass(className)) {
return false;
}
if (processingFields.containsKey(className)) {
if (!processingFields.get(className).contains(name)) {
processingFields.get(className).add(name);
return false;
} else {
return true;
}
} else {
return true;
}
}
@Override
public boolean shouldSkipClass(Class> aClass) {
final String className = aClass.getTypeName();
if (isZuoraClass(className)) {
if (!processingFields.containsKey(className)) {
processingFields.put(className, new HashSet<>());
}
}
return false;
}
boolean isZuoraClass(final String className) {
final List ignoreClassList = Arrays.asList("PreviewOrderSubscriptions", "PreviewOrderSubscriptionsAsync");
for (String ignoreName: ignoreClassList) {
if (className.startsWith("com.zuora") && className.endsWith(ignoreName)) {
return true;
}
}
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy