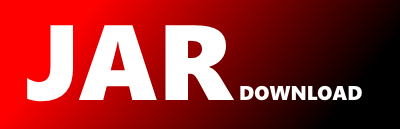
com.zuora.model.AccountBasicInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.AccountObjectNSFieldsCustomerTypeNS;
import com.zuora.model.AccountObjectNSFieldsSynctoNetSuiteNS;
import com.zuora.model.AccountStatus;
import java.io.IOException;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Container for basic information about the account.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class AccountBasicInfo {
public static final String SERIALIZED_NAME_CLASS_N_S = "Class__NS";
@SerializedName(SERIALIZED_NAME_CLASS_N_S)
private String classNS;
public static final String SERIALIZED_NAME_CUSTOMER_TYPE_N_S = "CustomerType__NS";
@SerializedName(SERIALIZED_NAME_CUSTOMER_TYPE_N_S)
private AccountObjectNSFieldsCustomerTypeNS customerTypeNS;
public static final String SERIALIZED_NAME_DEPARTMENT_N_S = "Department__NS";
@SerializedName(SERIALIZED_NAME_DEPARTMENT_N_S)
private String departmentNS;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_LOCATION_N_S = "Location__NS";
@SerializedName(SERIALIZED_NAME_LOCATION_N_S)
private String locationNS;
public static final String SERIALIZED_NAME_SUBSIDIARY_N_S = "Subsidiary__NS";
@SerializedName(SERIALIZED_NAME_SUBSIDIARY_N_S)
private String subsidiaryNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S = "SynctoNetSuite__NS";
@SerializedName(SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S)
private AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_ID = "communicationProfileId";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_ID)
private String communicationProfileId;
public static final String SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID = "creditMemoTemplateId";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID)
private String creditMemoTemplateId;
public static final String SERIALIZED_NAME_CRM_ID = "crmId";
@SerializedName(SERIALIZED_NAME_CRM_ID)
private String crmId;
public static final String SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID = "debitMemoTemplateId";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID)
private String debitMemoTemplateId;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_LAST_METRICS_UPDATE = "lastMetricsUpdate";
@SerializedName(SERIALIZED_NAME_LAST_METRICS_UPDATE)
private String lastMetricsUpdate;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_PARENT_ID = "parentId";
@SerializedName(SERIALIZED_NAME_PARENT_ID)
private String parentId;
public static final String SERIALIZED_NAME_PARTNER_ACCOUNT = "partnerAccount";
@SerializedName(SERIALIZED_NAME_PARTNER_ACCOUNT)
private Boolean partnerAccount;
public static final String SERIALIZED_NAME_PROFILE_NUMBER = "profileNumber";
@SerializedName(SERIALIZED_NAME_PROFILE_NUMBER)
private String profileNumber;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_SALES_REP = "salesRep";
@SerializedName(SERIALIZED_NAME_SALES_REP)
private String salesRep;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private AccountStatus status;
public static final String SERIALIZED_NAME_TAGS = "tags";
@SerializedName(SERIALIZED_NAME_TAGS)
private String tags;
public static final String SERIALIZED_NAME_CUSTOMER_SERVICE_REP_NAME = "customerServiceRepName";
@SerializedName(SERIALIZED_NAME_CUSTOMER_SERVICE_REP_NAME)
private String customerServiceRepName;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organizationLabel";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public static final String SERIALIZED_NAME_SUMMARY_STATEMENT_TEMPLATE_ID = "summaryStatementTemplateId";
@SerializedName(SERIALIZED_NAME_SUMMARY_STATEMENT_TEMPLATE_ID)
private String summaryStatementTemplateId;
public AccountBasicInfo() {
}
public AccountBasicInfo classNS(String classNS) {
this.classNS = classNS;
return this;
}
/**
* Value of the Class field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return classNS
*/
@javax.annotation.Nullable
public String getClassNS() {
return classNS;
}
public void setClassNS(String classNS) {
this.classNS = classNS;
}
public AccountBasicInfo customerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
return this;
}
/**
* Get customerTypeNS
* @return customerTypeNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsCustomerTypeNS getCustomerTypeNS() {
return customerTypeNS;
}
public void setCustomerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
}
public AccountBasicInfo departmentNS(String departmentNS) {
this.departmentNS = departmentNS;
return this;
}
/**
* Value of the Department field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return departmentNS
*/
@javax.annotation.Nullable
public String getDepartmentNS() {
return departmentNS;
}
public void setDepartmentNS(String departmentNS) {
this.departmentNS = departmentNS;
}
public AccountBasicInfo integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public AccountBasicInfo integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the account's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public AccountBasicInfo locationNS(String locationNS) {
this.locationNS = locationNS;
return this;
}
/**
* Value of the Location field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return locationNS
*/
@javax.annotation.Nullable
public String getLocationNS() {
return locationNS;
}
public void setLocationNS(String locationNS) {
this.locationNS = locationNS;
}
public AccountBasicInfo subsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
return this;
}
/**
* Value of the Subsidiary field for the corresponding customer account in NetSuite. The Subsidiary field is required if you use NetSuite OneWorld. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return subsidiaryNS
*/
@javax.annotation.Nullable
public String getSubsidiaryNS() {
return subsidiaryNS;
}
public void setSubsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
}
public AccountBasicInfo syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the account was sychronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public AccountBasicInfo synctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
return this;
}
/**
* Get synctoNetSuiteNS
* @return synctoNetSuiteNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsSynctoNetSuiteNS getSynctoNetSuiteNS() {
return synctoNetSuiteNS;
}
public void setSynctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
}
public AccountBasicInfo accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Account number.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public AccountBasicInfo batch(String batch) {
this.batch = batch;
return this;
}
/**
* The alias name given to a batch. A string of 50 characters or less.
* @return batch
*/
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public AccountBasicInfo communicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
return this;
}
/**
* The ID of the communication profile that this account is linked to.
* @return communicationProfileId
*/
@javax.annotation.Nullable
public String getCommunicationProfileId() {
return communicationProfileId;
}
public void setCommunicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
}
public AccountBasicInfo creditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
return this;
}
/**
* **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoicbe_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information. The unique ID of the credit memo template, configured in **Billing Settings** > **Manage Billing Document Configuration** through the Zuora UI. For example, 2c92c08a6246fdf101626b1b3fe0144b.
* @return creditMemoTemplateId
*/
@javax.annotation.Nullable
public String getCreditMemoTemplateId() {
return creditMemoTemplateId;
}
public void setCreditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
}
public AccountBasicInfo crmId(String crmId) {
this.crmId = crmId;
return this;
}
/**
* CRM account ID for the account, up to 100 characters.
* @return crmId
*/
@javax.annotation.Nullable
public String getCrmId() {
return crmId;
}
public void setCrmId(String crmId) {
this.crmId = crmId;
}
public AccountBasicInfo debitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
return this;
}
/**
* **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information. The unique ID of the debit memo template, configured in **Billing Settings** > **Manage Billing Document Configuration** through the Zuora UI. For example, 2c92c08d62470a8501626b19d24f19e2.
* @return debitMemoTemplateId
*/
@javax.annotation.Nullable
public String getDebitMemoTemplateId() {
return debitMemoTemplateId;
}
public void setDebitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
}
public AccountBasicInfo id(String id) {
this.id = id;
return this;
}
/**
* Account ID.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public AccountBasicInfo invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* Invoice template ID, configured in Billing Settings in the Zuora UI.
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public AccountBasicInfo lastMetricsUpdate(String lastMetricsUpdate) {
this.lastMetricsUpdate = lastMetricsUpdate;
return this;
}
/**
* The date and time when account metrics are last updated, if the account is a partner account. **Note**: - This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_customer_accounts/AAA_Overview_of_customer_accounts/Reseller_Account\" target=\"_blank\">Reseller Account</a> feature enabled. - If you have the Reseller Account feature enabled, and set the `partnerAccount` field to `false` for an account, the value of the `lastMetricsUpdate` field is automatically set to `null` in the response. - If you ever set the `partnerAccount` field to `true` for an account, the value of `lastMetricsUpdate` field is the time when the account metrics are last updated.
* @return lastMetricsUpdate
*/
@javax.annotation.Nullable
public String getLastMetricsUpdate() {
return lastMetricsUpdate;
}
public void setLastMetricsUpdate(String lastMetricsUpdate) {
this.lastMetricsUpdate = lastMetricsUpdate;
}
public AccountBasicInfo name(String name) {
this.name = name;
return this;
}
/**
* Account name.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public AccountBasicInfo notes(String notes) {
this.notes = notes;
return this;
}
/**
* Notes associated with the account, up to 65,535 characters.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public AccountBasicInfo parentId(String parentId) {
this.parentId = parentId;
return this;
}
/**
* Identifier of the parent customer account for this Account object. The length is 32 characters. Use this field if you have <a href=\"https://knowledgecenter.zuora.com/Billing/Subscriptions/Customer_Accounts/A_Customer_Account_Introduction#Customer_Hierarchy\" target=\"_blank\">Customer Hierarchy</a> enabled.
* @return parentId
*/
@javax.annotation.Nullable
public String getParentId() {
return parentId;
}
public void setParentId(String parentId) {
this.parentId = parentId;
}
public AccountBasicInfo partnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
return this;
}
/**
* Whether the customer account is a partner, distributor, or reseller. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_customer_accounts/AAA_Overview_of_customer_accounts/Reseller_Account\" target=\"_blank\">Reseller Account</a> feature enabled.
* @return partnerAccount
*/
@javax.annotation.Nullable
public Boolean getPartnerAccount() {
return partnerAccount;
}
public void setPartnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
}
public AccountBasicInfo profileNumber(String profileNumber) {
this.profileNumber = profileNumber;
return this;
}
/**
* The number of the communication profile that this account is linked to.
* @return profileNumber
*/
@javax.annotation.Nullable
public String getProfileNumber() {
return profileNumber;
}
public void setProfileNumber(String profileNumber) {
this.profileNumber = profileNumber;
}
public AccountBasicInfo purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* The purchase order number provided by your customer for services, products, or both purchased.
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public AccountBasicInfo salesRep(String salesRep) {
this.salesRep = salesRep;
return this;
}
/**
* The name of the sales representative associated with this account, if applicable. Maximum of 50 characters.
* @return salesRep
*/
@javax.annotation.Nullable
public String getSalesRep() {
return salesRep;
}
public void setSalesRep(String salesRep) {
this.salesRep = salesRep;
}
public AccountBasicInfo sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the billing document sequence set that is assigned to the customer account.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public AccountBasicInfo status(AccountStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public AccountStatus getStatus() {
return status;
}
public void setStatus(AccountStatus status) {
this.status = status;
}
public AccountBasicInfo tags(String tags) {
this.tags = tags;
return this;
}
/**
*
* @return tags
*/
@javax.annotation.Nullable
public String getTags() {
return tags;
}
public void setTags(String tags) {
this.tags = tags;
}
public AccountBasicInfo customerServiceRepName(String customerServiceRepName) {
this.customerServiceRepName = customerServiceRepName;
return this;
}
/**
* customer ServiceRep Name.
* @return customerServiceRepName
*/
@javax.annotation.Nullable
public String getCustomerServiceRepName() {
return customerServiceRepName;
}
public void setCustomerServiceRepName(String customerServiceRepName) {
this.customerServiceRepName = customerServiceRepName;
}
public AccountBasicInfo organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* organization label.
* @return organizationLabel
*/
@javax.annotation.Nullable
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
public AccountBasicInfo summaryStatementTemplateId(String summaryStatementTemplateId) {
this.summaryStatementTemplateId = summaryStatementTemplateId;
return this;
}
/**
* summary statement template ID.
* @return summaryStatementTemplateId
*/
@javax.annotation.Nullable
public String getSummaryStatementTemplateId() {
return summaryStatementTemplateId;
}
public void setSummaryStatementTemplateId(String summaryStatementTemplateId) {
this.summaryStatementTemplateId = summaryStatementTemplateId;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the AccountBasicInfo instance itself
*/
public AccountBasicInfo putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccountBasicInfo accountBasicInfo = (AccountBasicInfo) o;
return Objects.equals(this.classNS, accountBasicInfo.classNS) &&
Objects.equals(this.customerTypeNS, accountBasicInfo.customerTypeNS) &&
Objects.equals(this.departmentNS, accountBasicInfo.departmentNS) &&
Objects.equals(this.integrationIdNS, accountBasicInfo.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, accountBasicInfo.integrationStatusNS) &&
Objects.equals(this.locationNS, accountBasicInfo.locationNS) &&
Objects.equals(this.subsidiaryNS, accountBasicInfo.subsidiaryNS) &&
Objects.equals(this.syncDateNS, accountBasicInfo.syncDateNS) &&
Objects.equals(this.synctoNetSuiteNS, accountBasicInfo.synctoNetSuiteNS) &&
Objects.equals(this.accountNumber, accountBasicInfo.accountNumber) &&
Objects.equals(this.batch, accountBasicInfo.batch) &&
Objects.equals(this.communicationProfileId, accountBasicInfo.communicationProfileId) &&
Objects.equals(this.creditMemoTemplateId, accountBasicInfo.creditMemoTemplateId) &&
Objects.equals(this.crmId, accountBasicInfo.crmId) &&
Objects.equals(this.debitMemoTemplateId, accountBasicInfo.debitMemoTemplateId) &&
Objects.equals(this.id, accountBasicInfo.id) &&
Objects.equals(this.invoiceTemplateId, accountBasicInfo.invoiceTemplateId) &&
Objects.equals(this.lastMetricsUpdate, accountBasicInfo.lastMetricsUpdate) &&
Objects.equals(this.name, accountBasicInfo.name) &&
Objects.equals(this.notes, accountBasicInfo.notes) &&
Objects.equals(this.parentId, accountBasicInfo.parentId) &&
Objects.equals(this.partnerAccount, accountBasicInfo.partnerAccount) &&
Objects.equals(this.profileNumber, accountBasicInfo.profileNumber) &&
Objects.equals(this.purchaseOrderNumber, accountBasicInfo.purchaseOrderNumber) &&
Objects.equals(this.salesRep, accountBasicInfo.salesRep) &&
Objects.equals(this.sequenceSetId, accountBasicInfo.sequenceSetId) &&
Objects.equals(this.status, accountBasicInfo.status) &&
Objects.equals(this.tags, accountBasicInfo.tags) &&
Objects.equals(this.customerServiceRepName, accountBasicInfo.customerServiceRepName) &&
Objects.equals(this.organizationLabel, accountBasicInfo.organizationLabel) &&
Objects.equals(this.summaryStatementTemplateId, accountBasicInfo.summaryStatementTemplateId)&&
Objects.equals(this.additionalProperties, accountBasicInfo.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(classNS, customerTypeNS, departmentNS, integrationIdNS, integrationStatusNS, locationNS, subsidiaryNS, syncDateNS, synctoNetSuiteNS, accountNumber, batch, communicationProfileId, creditMemoTemplateId, crmId, debitMemoTemplateId, id, invoiceTemplateId, lastMetricsUpdate, name, notes, parentId, partnerAccount, profileNumber, purchaseOrderNumber, salesRep, sequenceSetId, status, tags, customerServiceRepName, organizationLabel, summaryStatementTemplateId, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccountBasicInfo {\n");
sb.append(" classNS: ").append(toIndentedString(classNS)).append("\n");
sb.append(" customerTypeNS: ").append(toIndentedString(customerTypeNS)).append("\n");
sb.append(" departmentNS: ").append(toIndentedString(departmentNS)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" locationNS: ").append(toIndentedString(locationNS)).append("\n");
sb.append(" subsidiaryNS: ").append(toIndentedString(subsidiaryNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" synctoNetSuiteNS: ").append(toIndentedString(synctoNetSuiteNS)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" communicationProfileId: ").append(toIndentedString(communicationProfileId)).append("\n");
sb.append(" creditMemoTemplateId: ").append(toIndentedString(creditMemoTemplateId)).append("\n");
sb.append(" crmId: ").append(toIndentedString(crmId)).append("\n");
sb.append(" debitMemoTemplateId: ").append(toIndentedString(debitMemoTemplateId)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" lastMetricsUpdate: ").append(toIndentedString(lastMetricsUpdate)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" parentId: ").append(toIndentedString(parentId)).append("\n");
sb.append(" partnerAccount: ").append(toIndentedString(partnerAccount)).append("\n");
sb.append(" profileNumber: ").append(toIndentedString(profileNumber)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" salesRep: ").append(toIndentedString(salesRep)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" customerServiceRepName: ").append(toIndentedString(customerServiceRepName)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append(" summaryStatementTemplateId: ").append(toIndentedString(summaryStatementTemplateId)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("Class__NS");
openapiFields.add("CustomerType__NS");
openapiFields.add("Department__NS");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Location__NS");
openapiFields.add("Subsidiary__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("SynctoNetSuite__NS");
openapiFields.add("accountNumber");
openapiFields.add("batch");
openapiFields.add("communicationProfileId");
openapiFields.add("creditMemoTemplateId");
openapiFields.add("crmId");
openapiFields.add("debitMemoTemplateId");
openapiFields.add("id");
openapiFields.add("invoiceTemplateId");
openapiFields.add("lastMetricsUpdate");
openapiFields.add("name");
openapiFields.add("notes");
openapiFields.add("parentId");
openapiFields.add("partnerAccount");
openapiFields.add("profileNumber");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("salesRep");
openapiFields.add("sequenceSetId");
openapiFields.add("status");
openapiFields.add("tags");
openapiFields.add("customerServiceRepName");
openapiFields.add("organizationLabel");
openapiFields.add("summaryStatementTemplateId");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to AccountBasicInfo
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!AccountBasicInfo.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in AccountBasicInfo is not found in the empty JSON string", AccountBasicInfo.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("Class__NS") != null && !jsonObj.get("Class__NS").isJsonNull()) && !jsonObj.get("Class__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Class__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Class__NS").toString()));
}
if ((jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) && !jsonObj.get("CustomerType__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `CustomerType__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("CustomerType__NS").toString()));
}
// validate the optional field `CustomerType__NS`
if (jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) {
AccountObjectNSFieldsCustomerTypeNS.validateJsonElement(jsonObj.get("CustomerType__NS"));
}
if ((jsonObj.get("Department__NS") != null && !jsonObj.get("Department__NS").isJsonNull()) && !jsonObj.get("Department__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Department__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Department__NS").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Location__NS") != null && !jsonObj.get("Location__NS").isJsonNull()) && !jsonObj.get("Location__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Location__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Location__NS").toString()));
}
if ((jsonObj.get("Subsidiary__NS") != null && !jsonObj.get("Subsidiary__NS").isJsonNull()) && !jsonObj.get("Subsidiary__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Subsidiary__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Subsidiary__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) && !jsonObj.get("SynctoNetSuite__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SynctoNetSuite__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SynctoNetSuite__NS").toString()));
}
// validate the optional field `SynctoNetSuite__NS`
if (jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) {
AccountObjectNSFieldsSynctoNetSuiteNS.validateJsonElement(jsonObj.get("SynctoNetSuite__NS"));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
if ((jsonObj.get("communicationProfileId") != null && !jsonObj.get("communicationProfileId").isJsonNull()) && !jsonObj.get("communicationProfileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `communicationProfileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("communicationProfileId").toString()));
}
if ((jsonObj.get("creditMemoTemplateId") != null && !jsonObj.get("creditMemoTemplateId").isJsonNull()) && !jsonObj.get("creditMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoTemplateId").toString()));
}
if ((jsonObj.get("crmId") != null && !jsonObj.get("crmId").isJsonNull()) && !jsonObj.get("crmId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `crmId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("crmId").toString()));
}
if ((jsonObj.get("debitMemoTemplateId") != null && !jsonObj.get("debitMemoTemplateId").isJsonNull()) && !jsonObj.get("debitMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `debitMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("debitMemoTemplateId").toString()));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if ((jsonObj.get("lastMetricsUpdate") != null && !jsonObj.get("lastMetricsUpdate").isJsonNull()) && !jsonObj.get("lastMetricsUpdate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastMetricsUpdate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastMetricsUpdate").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("parentId") != null && !jsonObj.get("parentId").isJsonNull()) && !jsonObj.get("parentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parentId").toString()));
}
if ((jsonObj.get("profileNumber") != null && !jsonObj.get("profileNumber").isJsonNull()) && !jsonObj.get("profileNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `profileNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("profileNumber").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("salesRep") != null && !jsonObj.get("salesRep").isJsonNull()) && !jsonObj.get("salesRep").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `salesRep` to be a primitive type in the JSON string but got `%s`", jsonObj.get("salesRep").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
AccountStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("tags") != null && !jsonObj.get("tags").isJsonNull()) && !jsonObj.get("tags").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tags` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tags").toString()));
}
if ((jsonObj.get("customerServiceRepName") != null && !jsonObj.get("customerServiceRepName").isJsonNull()) && !jsonObj.get("customerServiceRepName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `customerServiceRepName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("customerServiceRepName").toString()));
}
if ((jsonObj.get("organizationLabel") != null && !jsonObj.get("organizationLabel").isJsonNull()) && !jsonObj.get("organizationLabel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationLabel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationLabel").toString()));
}
if ((jsonObj.get("summaryStatementTemplateId") != null && !jsonObj.get("summaryStatementTemplateId").isJsonNull()) && !jsonObj.get("summaryStatementTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `summaryStatementTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("summaryStatementTemplateId").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!AccountBasicInfo.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'AccountBasicInfo' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(AccountBasicInfo.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, AccountBasicInfo value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public AccountBasicInfo read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
AccountBasicInfo instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of AccountBasicInfo given an JSON string
*
* @param jsonString JSON string
* @return An instance of AccountBasicInfo
* @throws IOException if the JSON string is invalid with respect to AccountBasicInfo
*/
public static AccountBasicInfo fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, AccountBasicInfo.class);
}
/**
* Convert an instance of AccountBasicInfo to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy