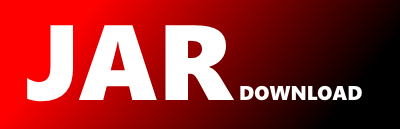
com.zuora.model.AccountSummaryBasicInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.AccountObjectNSFieldsCustomerTypeNS;
import com.zuora.model.AccountObjectNSFieldsSynctoNetSuiteNS;
import com.zuora.model.AccountStatus;
import com.zuora.model.AccountSummaryDefaultPaymentMethod;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Container for basic information about the account.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class AccountSummaryBasicInfo {
public static final String SERIALIZED_NAME_CLASS_N_S = "Class__NS";
@SerializedName(SERIALIZED_NAME_CLASS_N_S)
private String classNS;
public static final String SERIALIZED_NAME_CUSTOMER_TYPE_N_S = "CustomerType__NS";
@SerializedName(SERIALIZED_NAME_CUSTOMER_TYPE_N_S)
private AccountObjectNSFieldsCustomerTypeNS customerTypeNS;
public static final String SERIALIZED_NAME_DEPARTMENT_N_S = "Department__NS";
@SerializedName(SERIALIZED_NAME_DEPARTMENT_N_S)
private String departmentNS;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_LOCATION_N_S = "Location__NS";
@SerializedName(SERIALIZED_NAME_LOCATION_N_S)
private String locationNS;
public static final String SERIALIZED_NAME_SUBSIDIARY_N_S = "Subsidiary__NS";
@SerializedName(SERIALIZED_NAME_SUBSIDIARY_N_S)
private String subsidiaryNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S = "SynctoNetSuite__NS";
@SerializedName(SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S)
private AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES = "additionalEmailAddresses";
@SerializedName(SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES)
private List additionalEmailAddresses;
public static final String SERIALIZED_NAME_AUTO_PAY = "autoPay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_BALANCE = "balance";
@SerializedName(SERIALIZED_NAME_BALANCE)
private BigDecimal balance;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD = "defaultPaymentMethod";
@SerializedName(SERIALIZED_NAME_DEFAULT_PAYMENT_METHOD)
private AccountSummaryDefaultPaymentMethod defaultPaymentMethod;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL = "invoiceDeliveryPrefsEmail";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL)
private Boolean invoiceDeliveryPrefsEmail;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT = "invoiceDeliveryPrefsPrint";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT)
private Boolean invoiceDeliveryPrefsPrint;
public static final String SERIALIZED_NAME_LAST_INVOICE_DATE = "lastInvoiceDate";
@SerializedName(SERIALIZED_NAME_LAST_INVOICE_DATE)
private LocalDate lastInvoiceDate;
public static final String SERIALIZED_NAME_LAST_METRICS_UPDATE = "lastMetricsUpdate";
@SerializedName(SERIALIZED_NAME_LAST_METRICS_UPDATE)
private String lastMetricsUpdate;
public static final String SERIALIZED_NAME_LAST_PAYMENT_AMOUNT = "lastPaymentAmount";
@SerializedName(SERIALIZED_NAME_LAST_PAYMENT_AMOUNT)
private BigDecimal lastPaymentAmount;
public static final String SERIALIZED_NAME_LAST_PAYMENT_DATE = "lastPaymentDate";
@SerializedName(SERIALIZED_NAME_LAST_PAYMENT_DATE)
private LocalDate lastPaymentDate;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_PARTNER_ACCOUNT = "partnerAccount";
@SerializedName(SERIALIZED_NAME_PARTNER_ACCOUNT)
private Boolean partnerAccount;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private AccountStatus status;
public static final String SERIALIZED_NAME_TAGS = "tags";
@SerializedName(SERIALIZED_NAME_TAGS)
private String tags;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organizationLabel";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_CASCADING_CONSENT = "paymentMethodCascadingConsent";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_CASCADING_CONSENT)
private Boolean paymentMethodCascadingConsent;
public AccountSummaryBasicInfo() {
}
public AccountSummaryBasicInfo classNS(String classNS) {
this.classNS = classNS;
return this;
}
/**
* Value of the Class field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return classNS
*/
@javax.annotation.Nullable
public String getClassNS() {
return classNS;
}
public void setClassNS(String classNS) {
this.classNS = classNS;
}
public AccountSummaryBasicInfo customerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
return this;
}
/**
* Get customerTypeNS
* @return customerTypeNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsCustomerTypeNS getCustomerTypeNS() {
return customerTypeNS;
}
public void setCustomerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
}
public AccountSummaryBasicInfo departmentNS(String departmentNS) {
this.departmentNS = departmentNS;
return this;
}
/**
* Value of the Department field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return departmentNS
*/
@javax.annotation.Nullable
public String getDepartmentNS() {
return departmentNS;
}
public void setDepartmentNS(String departmentNS) {
this.departmentNS = departmentNS;
}
public AccountSummaryBasicInfo integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public AccountSummaryBasicInfo integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the account's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public AccountSummaryBasicInfo locationNS(String locationNS) {
this.locationNS = locationNS;
return this;
}
/**
* Value of the Location field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return locationNS
*/
@javax.annotation.Nullable
public String getLocationNS() {
return locationNS;
}
public void setLocationNS(String locationNS) {
this.locationNS = locationNS;
}
public AccountSummaryBasicInfo subsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
return this;
}
/**
* Value of the Subsidiary field for the corresponding customer account in NetSuite. The Subsidiary field is required if you use NetSuite OneWorld. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return subsidiaryNS
*/
@javax.annotation.Nullable
public String getSubsidiaryNS() {
return subsidiaryNS;
}
public void setSubsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
}
public AccountSummaryBasicInfo syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the account was sychronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public AccountSummaryBasicInfo synctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
return this;
}
/**
* Get synctoNetSuiteNS
* @return synctoNetSuiteNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsSynctoNetSuiteNS getSynctoNetSuiteNS() {
return synctoNetSuiteNS;
}
public void setSynctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
}
public AccountSummaryBasicInfo accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Account number.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public AccountSummaryBasicInfo additionalEmailAddresses(List additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
return this;
}
public AccountSummaryBasicInfo addAdditionalEmailAddressesItem(String additionalEmailAddressesItem) {
if (this.additionalEmailAddresses == null) {
this.additionalEmailAddresses = new ArrayList<>();
}
this.additionalEmailAddresses.add(additionalEmailAddressesItem);
return this;
}
/**
* A list of additional email addresses to receive email notifications.
* @return additionalEmailAddresses
*/
@javax.annotation.Nullable
public List getAdditionalEmailAddresses() {
return additionalEmailAddresses;
}
public void setAdditionalEmailAddresses(List additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
}
public AccountSummaryBasicInfo autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Whether future payments are automatically collected when they are due during a payment run.
* @return autoPay
*/
@javax.annotation.Nullable
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public AccountSummaryBasicInfo balance(BigDecimal balance) {
this.balance = balance;
return this;
}
/**
* Current outstanding balance.
* @return balance
*/
@javax.annotation.Nullable
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public AccountSummaryBasicInfo batch(String batch) {
this.batch = batch;
return this;
}
/**
* The alias name given to a batch. A string of 50 characters or less.
* @return batch
*/
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public AccountSummaryBasicInfo billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* Billing cycle day (BCD), the day of the month when a bill run generates invoices for the account.
* @return billCycleDay
*/
@javax.annotation.Nullable
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public AccountSummaryBasicInfo currency(String currency) {
this.currency = currency;
return this;
}
/**
* A currency as defined in Billing Settings in the Zuora UI.
* @return currency
*/
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public AccountSummaryBasicInfo defaultPaymentMethod(AccountSummaryDefaultPaymentMethod defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* Get defaultPaymentMethod
* @return defaultPaymentMethod
*/
@javax.annotation.Nullable
public AccountSummaryDefaultPaymentMethod getDefaultPaymentMethod() {
return defaultPaymentMethod;
}
public void setDefaultPaymentMethod(AccountSummaryDefaultPaymentMethod defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
}
public AccountSummaryBasicInfo id(String id) {
this.id = id;
return this;
}
/**
* Account ID.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public AccountSummaryBasicInfo invoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
return this;
}
/**
* Whether the customer wants to receive invoices through email.
* @return invoiceDeliveryPrefsEmail
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsEmail() {
return invoiceDeliveryPrefsEmail;
}
public void setInvoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
}
public AccountSummaryBasicInfo invoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
return this;
}
/**
* Whether the customer wants to receive printed invoices, such as through postal mail.
* @return invoiceDeliveryPrefsPrint
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsPrint() {
return invoiceDeliveryPrefsPrint;
}
public void setInvoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
}
public AccountSummaryBasicInfo lastInvoiceDate(LocalDate lastInvoiceDate) {
this.lastInvoiceDate = lastInvoiceDate;
return this;
}
/**
* Date of the most recent invoice for the account; null if no invoice has ever been generated.
* @return lastInvoiceDate
*/
@javax.annotation.Nullable
public LocalDate getLastInvoiceDate() {
return lastInvoiceDate;
}
public void setLastInvoiceDate(LocalDate lastInvoiceDate) {
this.lastInvoiceDate = lastInvoiceDate;
}
public AccountSummaryBasicInfo lastMetricsUpdate(String lastMetricsUpdate) {
this.lastMetricsUpdate = lastMetricsUpdate;
return this;
}
/**
* The date and time when account metrics are last updated, if the account is a partner account. **Note**: - This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_customer_accounts/AAA_Overview_of_customer_accounts/Reseller_Account\" target=\"_blank\">Reseller Account</a> feature enabled. - If you have the Reseller Account feature enabled, and set the `partnerAccount` field to `false` for an account, the value of the `lastMetricsUpdate` field is automatically set to `null` in the response. - If you ever set the `partnerAccount` field to `true` for an account, the value of `lastMetricsUpdate` field is the time when the account metrics are last updated.
* @return lastMetricsUpdate
*/
@javax.annotation.Nullable
public String getLastMetricsUpdate() {
return lastMetricsUpdate;
}
public void setLastMetricsUpdate(String lastMetricsUpdate) {
this.lastMetricsUpdate = lastMetricsUpdate;
}
public AccountSummaryBasicInfo lastPaymentAmount(BigDecimal lastPaymentAmount) {
this.lastPaymentAmount = lastPaymentAmount;
return this;
}
/**
* Amount of the most recent payment collected for the account; null if no payment has ever been collected.
* @return lastPaymentAmount
*/
@javax.annotation.Nullable
public BigDecimal getLastPaymentAmount() {
return lastPaymentAmount;
}
public void setLastPaymentAmount(BigDecimal lastPaymentAmount) {
this.lastPaymentAmount = lastPaymentAmount;
}
public AccountSummaryBasicInfo lastPaymentDate(LocalDate lastPaymentDate) {
this.lastPaymentDate = lastPaymentDate;
return this;
}
/**
* Date of the most recent payment collected for the account. Null if no payment has ever been collected.
* @return lastPaymentDate
*/
@javax.annotation.Nullable
public LocalDate getLastPaymentDate() {
return lastPaymentDate;
}
public void setLastPaymentDate(LocalDate lastPaymentDate) {
this.lastPaymentDate = lastPaymentDate;
}
public AccountSummaryBasicInfo name(String name) {
this.name = name;
return this;
}
/**
* Account name.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public AccountSummaryBasicInfo partnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
return this;
}
/**
* Whether the customer account is a partner, distributor, or reseller. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_customer_accounts/AAA_Overview_of_customer_accounts/Reseller_Account\" target=\"_blank\">Reseller Account</a> feature enabled.
* @return partnerAccount
*/
@javax.annotation.Nullable
public Boolean getPartnerAccount() {
return partnerAccount;
}
public void setPartnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
}
public AccountSummaryBasicInfo purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* The purchase order number provided by your customer for services, products, or both purchased.
* @return purchaseOrderNumber
*/
@javax.annotation.Nullable
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public AccountSummaryBasicInfo status(AccountStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public AccountStatus getStatus() {
return status;
}
public void setStatus(AccountStatus status) {
this.status = status;
}
public AccountSummaryBasicInfo tags(String tags) {
this.tags = tags;
return this;
}
/**
*
* @return tags
*/
@javax.annotation.Nullable
public String getTags() {
return tags;
}
public void setTags(String tags) {
this.tags = tags;
}
public AccountSummaryBasicInfo organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* organization label.
* @return organizationLabel
*/
@javax.annotation.Nullable
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
public AccountSummaryBasicInfo paymentMethodCascadingConsent(Boolean paymentMethodCascadingConsent) {
this.paymentMethodCascadingConsent = paymentMethodCascadingConsent;
return this;
}
/**
* payment method cascading consent
* @return paymentMethodCascadingConsent
*/
@javax.annotation.Nullable
public Boolean getPaymentMethodCascadingConsent() {
return paymentMethodCascadingConsent;
}
public void setPaymentMethodCascadingConsent(Boolean paymentMethodCascadingConsent) {
this.paymentMethodCascadingConsent = paymentMethodCascadingConsent;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the AccountSummaryBasicInfo instance itself
*/
public AccountSummaryBasicInfo putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccountSummaryBasicInfo accountSummaryBasicInfo = (AccountSummaryBasicInfo) o;
return Objects.equals(this.classNS, accountSummaryBasicInfo.classNS) &&
Objects.equals(this.customerTypeNS, accountSummaryBasicInfo.customerTypeNS) &&
Objects.equals(this.departmentNS, accountSummaryBasicInfo.departmentNS) &&
Objects.equals(this.integrationIdNS, accountSummaryBasicInfo.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, accountSummaryBasicInfo.integrationStatusNS) &&
Objects.equals(this.locationNS, accountSummaryBasicInfo.locationNS) &&
Objects.equals(this.subsidiaryNS, accountSummaryBasicInfo.subsidiaryNS) &&
Objects.equals(this.syncDateNS, accountSummaryBasicInfo.syncDateNS) &&
Objects.equals(this.synctoNetSuiteNS, accountSummaryBasicInfo.synctoNetSuiteNS) &&
Objects.equals(this.accountNumber, accountSummaryBasicInfo.accountNumber) &&
Objects.equals(this.additionalEmailAddresses, accountSummaryBasicInfo.additionalEmailAddresses) &&
Objects.equals(this.autoPay, accountSummaryBasicInfo.autoPay) &&
Objects.equals(this.balance, accountSummaryBasicInfo.balance) &&
Objects.equals(this.batch, accountSummaryBasicInfo.batch) &&
Objects.equals(this.billCycleDay, accountSummaryBasicInfo.billCycleDay) &&
Objects.equals(this.currency, accountSummaryBasicInfo.currency) &&
Objects.equals(this.defaultPaymentMethod, accountSummaryBasicInfo.defaultPaymentMethod) &&
Objects.equals(this.id, accountSummaryBasicInfo.id) &&
Objects.equals(this.invoiceDeliveryPrefsEmail, accountSummaryBasicInfo.invoiceDeliveryPrefsEmail) &&
Objects.equals(this.invoiceDeliveryPrefsPrint, accountSummaryBasicInfo.invoiceDeliveryPrefsPrint) &&
Objects.equals(this.lastInvoiceDate, accountSummaryBasicInfo.lastInvoiceDate) &&
Objects.equals(this.lastMetricsUpdate, accountSummaryBasicInfo.lastMetricsUpdate) &&
Objects.equals(this.lastPaymentAmount, accountSummaryBasicInfo.lastPaymentAmount) &&
Objects.equals(this.lastPaymentDate, accountSummaryBasicInfo.lastPaymentDate) &&
Objects.equals(this.name, accountSummaryBasicInfo.name) &&
Objects.equals(this.partnerAccount, accountSummaryBasicInfo.partnerAccount) &&
Objects.equals(this.purchaseOrderNumber, accountSummaryBasicInfo.purchaseOrderNumber) &&
Objects.equals(this.status, accountSummaryBasicInfo.status) &&
Objects.equals(this.tags, accountSummaryBasicInfo.tags) &&
Objects.equals(this.organizationLabel, accountSummaryBasicInfo.organizationLabel) &&
Objects.equals(this.paymentMethodCascadingConsent, accountSummaryBasicInfo.paymentMethodCascadingConsent)&&
Objects.equals(this.additionalProperties, accountSummaryBasicInfo.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(classNS, customerTypeNS, departmentNS, integrationIdNS, integrationStatusNS, locationNS, subsidiaryNS, syncDateNS, synctoNetSuiteNS, accountNumber, additionalEmailAddresses, autoPay, balance, batch, billCycleDay, currency, defaultPaymentMethod, id, invoiceDeliveryPrefsEmail, invoiceDeliveryPrefsPrint, lastInvoiceDate, lastMetricsUpdate, lastPaymentAmount, lastPaymentDate, name, partnerAccount, purchaseOrderNumber, status, tags, organizationLabel, paymentMethodCascadingConsent, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccountSummaryBasicInfo {\n");
sb.append(" classNS: ").append(toIndentedString(classNS)).append("\n");
sb.append(" customerTypeNS: ").append(toIndentedString(customerTypeNS)).append("\n");
sb.append(" departmentNS: ").append(toIndentedString(departmentNS)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" locationNS: ").append(toIndentedString(locationNS)).append("\n");
sb.append(" subsidiaryNS: ").append(toIndentedString(subsidiaryNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" synctoNetSuiteNS: ").append(toIndentedString(synctoNetSuiteNS)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" additionalEmailAddresses: ").append(toIndentedString(additionalEmailAddresses)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" balance: ").append(toIndentedString(balance)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" defaultPaymentMethod: ").append(toIndentedString(defaultPaymentMethod)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" invoiceDeliveryPrefsEmail: ").append(toIndentedString(invoiceDeliveryPrefsEmail)).append("\n");
sb.append(" invoiceDeliveryPrefsPrint: ").append(toIndentedString(invoiceDeliveryPrefsPrint)).append("\n");
sb.append(" lastInvoiceDate: ").append(toIndentedString(lastInvoiceDate)).append("\n");
sb.append(" lastMetricsUpdate: ").append(toIndentedString(lastMetricsUpdate)).append("\n");
sb.append(" lastPaymentAmount: ").append(toIndentedString(lastPaymentAmount)).append("\n");
sb.append(" lastPaymentDate: ").append(toIndentedString(lastPaymentDate)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" partnerAccount: ").append(toIndentedString(partnerAccount)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append(" paymentMethodCascadingConsent: ").append(toIndentedString(paymentMethodCascadingConsent)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("Class__NS");
openapiFields.add("CustomerType__NS");
openapiFields.add("Department__NS");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Location__NS");
openapiFields.add("Subsidiary__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("SynctoNetSuite__NS");
openapiFields.add("accountNumber");
openapiFields.add("additionalEmailAddresses");
openapiFields.add("autoPay");
openapiFields.add("balance");
openapiFields.add("batch");
openapiFields.add("billCycleDay");
openapiFields.add("currency");
openapiFields.add("defaultPaymentMethod");
openapiFields.add("id");
openapiFields.add("invoiceDeliveryPrefsEmail");
openapiFields.add("invoiceDeliveryPrefsPrint");
openapiFields.add("lastInvoiceDate");
openapiFields.add("lastMetricsUpdate");
openapiFields.add("lastPaymentAmount");
openapiFields.add("lastPaymentDate");
openapiFields.add("name");
openapiFields.add("partnerAccount");
openapiFields.add("purchaseOrderNumber");
openapiFields.add("status");
openapiFields.add("tags");
openapiFields.add("organizationLabel");
openapiFields.add("paymentMethodCascadingConsent");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to AccountSummaryBasicInfo
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!AccountSummaryBasicInfo.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in AccountSummaryBasicInfo is not found in the empty JSON string", AccountSummaryBasicInfo.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("Class__NS") != null && !jsonObj.get("Class__NS").isJsonNull()) && !jsonObj.get("Class__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Class__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Class__NS").toString()));
}
if ((jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) && !jsonObj.get("CustomerType__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `CustomerType__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("CustomerType__NS").toString()));
}
// validate the optional field `CustomerType__NS`
if (jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) {
AccountObjectNSFieldsCustomerTypeNS.validateJsonElement(jsonObj.get("CustomerType__NS"));
}
if ((jsonObj.get("Department__NS") != null && !jsonObj.get("Department__NS").isJsonNull()) && !jsonObj.get("Department__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Department__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Department__NS").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Location__NS") != null && !jsonObj.get("Location__NS").isJsonNull()) && !jsonObj.get("Location__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Location__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Location__NS").toString()));
}
if ((jsonObj.get("Subsidiary__NS") != null && !jsonObj.get("Subsidiary__NS").isJsonNull()) && !jsonObj.get("Subsidiary__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Subsidiary__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Subsidiary__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) && !jsonObj.get("SynctoNetSuite__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SynctoNetSuite__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SynctoNetSuite__NS").toString()));
}
// validate the optional field `SynctoNetSuite__NS`
if (jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) {
AccountObjectNSFieldsSynctoNetSuiteNS.validateJsonElement(jsonObj.get("SynctoNetSuite__NS"));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("additionalEmailAddresses") != null && !jsonObj.get("additionalEmailAddresses").isJsonNull() && !jsonObj.get("additionalEmailAddresses").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `additionalEmailAddresses` to be an array in the JSON string but got `%s`", jsonObj.get("additionalEmailAddresses").toString()));
}
if ((jsonObj.get("balance") != null && !jsonObj.get("balance").isJsonNull()) && !jsonObj.get("balance").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `balance` to be a primitive type in the JSON string but got `%s`", jsonObj.get("balance").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
if ((jsonObj.get("currency") != null && !jsonObj.get("currency").isJsonNull()) && !jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
// validate the optional field `defaultPaymentMethod`
if (jsonObj.get("defaultPaymentMethod") != null && !jsonObj.get("defaultPaymentMethod").isJsonNull()) {
AccountSummaryDefaultPaymentMethod.validateJsonElement(jsonObj.get("defaultPaymentMethod"));
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("lastMetricsUpdate") != null && !jsonObj.get("lastMetricsUpdate").isJsonNull()) && !jsonObj.get("lastMetricsUpdate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastMetricsUpdate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastMetricsUpdate").toString()));
}
if ((jsonObj.get("lastPaymentAmount") != null && !jsonObj.get("lastPaymentAmount").isJsonNull()) && !jsonObj.get("lastPaymentAmount").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `lastPaymentAmount` to be a primitive type in the JSON string but got `%s`", jsonObj.get("lastPaymentAmount").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("purchaseOrderNumber") != null && !jsonObj.get("purchaseOrderNumber").isJsonNull()) && !jsonObj.get("purchaseOrderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `purchaseOrderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("purchaseOrderNumber").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
AccountStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("tags") != null && !jsonObj.get("tags").isJsonNull()) && !jsonObj.get("tags").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tags` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tags").toString()));
}
if ((jsonObj.get("organizationLabel") != null && !jsonObj.get("organizationLabel").isJsonNull()) && !jsonObj.get("organizationLabel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationLabel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationLabel").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!AccountSummaryBasicInfo.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'AccountSummaryBasicInfo' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(AccountSummaryBasicInfo.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, AccountSummaryBasicInfo value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public AccountSummaryBasicInfo read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
AccountSummaryBasicInfo instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of AccountSummaryBasicInfo given an JSON string
*
* @param jsonString JSON string
* @return An instance of AccountSummaryBasicInfo
* @throws IOException if the JSON string is invalid with respect to AccountSummaryBasicInfo
*/
public static AccountSummaryBasicInfo fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, AccountSummaryBasicInfo.class);
}
/**
* Convert an instance of AccountSummaryBasicInfo to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy