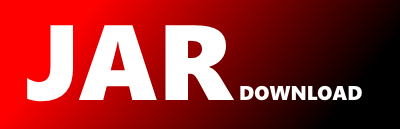
com.zuora.model.BillRunResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.BillRunFilter;
import com.zuora.model.BillRunSchedule;
import com.zuora.model.BillRunStatus;
import com.zuora.model.ChargeType;
import com.zuora.model.FailedReason;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* BillRunResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class BillRunResponse {
public static final String SERIALIZED_NAME_PROCESS_ID = "processId";
@SerializedName(SERIALIZED_NAME_PROCESS_ID)
private String processId;
public static final String SERIALIZED_NAME_REQUEST_ID = "requestId";
@SerializedName(SERIALIZED_NAME_REQUEST_ID)
private String requestId;
public static final String SERIALIZED_NAME_REASONS = "reasons";
@SerializedName(SERIALIZED_NAME_REASONS)
private List reasons;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_BILL_RUN_NUMBER = "billRunNumber";
@SerializedName(SERIALIZED_NAME_BILL_RUN_NUMBER)
private String billRunNumber;
public static final String SERIALIZED_NAME_BATCHES = "batches";
@SerializedName(SERIALIZED_NAME_BATCHES)
private List batches;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private String billCycleDay;
public static final String SERIALIZED_NAME_BILL_RUN_FILTERS = "billRunFilters";
@SerializedName(SERIALIZED_NAME_BILL_RUN_FILTERS)
private List billRunFilters;
public static final String SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE = "chargeTypeToExclude";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE)
private List chargeTypeToExclude;
public static final String SERIALIZED_NAME_AUTO_EMAIL = "autoEmail";
@SerializedName(SERIALIZED_NAME_AUTO_EMAIL)
private Boolean autoEmail;
public static final String SERIALIZED_NAME_AUTO_POST = "autoPost";
@SerializedName(SERIALIZED_NAME_AUTO_POST)
private Boolean autoPost;
public static final String SERIALIZED_NAME_AUTO_RENEWAL = "autoRenewal";
@SerializedName(SERIALIZED_NAME_AUTO_RENEWAL)
private Boolean autoRenewal;
public static final String SERIALIZED_NAME_NO_EMAIL_FOR_ZERO_AMOUNT_INVOICE = "noEmailForZeroAmountInvoice";
@SerializedName(SERIALIZED_NAME_NO_EMAIL_FOR_ZERO_AMOUNT_INVOICE)
private Boolean noEmailForZeroAmountInvoice;
public static final String SERIALIZED_NAME_SCHEDULE = "schedule";
@SerializedName(SERIALIZED_NAME_SCHEDULE)
private BillRunSchedule schedule;
public static final String SERIALIZED_NAME_SCHEDULED_EXECUTION_TIME = "scheduledExecutionTime";
@SerializedName(SERIALIZED_NAME_SCHEDULED_EXECUTION_TIME)
private String scheduledExecutionTime;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private BillRunStatus status;
public static final String SERIALIZED_NAME_INVOICE_DATE = "invoiceDate";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE)
private LocalDate invoiceDate;
public static final String SERIALIZED_NAME_INVOICE_DATE_OFFSET = "invoiceDateOffset";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE_OFFSET)
private Integer invoiceDateOffset;
public static final String SERIALIZED_NAME_INVOICE_DATE_MONTH_OFFSET = "invoiceDateMonthOffset";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE_MONTH_OFFSET)
private Integer invoiceDateMonthOffset;
public static final String SERIALIZED_NAME_INVOICE_DATE_DAY_OF_MONTH = "invoiceDateDayOfMonth";
@SerializedName(SERIALIZED_NAME_INVOICE_DATE_DAY_OF_MONTH)
private Integer invoiceDateDayOfMonth;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TARGET_DATE_OFFSET = "targetDateOffset";
@SerializedName(SERIALIZED_NAME_TARGET_DATE_OFFSET)
private Integer targetDateOffset;
public static final String SERIALIZED_NAME_TARGET_DATE_MONTH_OFFSET = "targetDateMonthOffset";
@SerializedName(SERIALIZED_NAME_TARGET_DATE_MONTH_OFFSET)
private Integer targetDateMonthOffset;
public static final String SERIALIZED_NAME_TARGET_DATE_DAY_OF_MONTH = "targetDateDayOfMonth";
@SerializedName(SERIALIZED_NAME_TARGET_DATE_DAY_OF_MONTH)
private Integer targetDateDayOfMonth;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "createdById";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private String createdDate;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updatedById";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_DATE = "updatedDate";
@SerializedName(SERIALIZED_NAME_UPDATED_DATE)
private String updatedDate;
public BillRunResponse() {
}
public BillRunResponse processId(String processId) {
this.processId = processId;
return this;
}
/**
* The Id of the process that handle the operation.
* @return processId
*/
@javax.annotation.Nullable
public String getProcessId() {
return processId;
}
public void setProcessId(String processId) {
this.processId = processId;
}
public BillRunResponse requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Unique request identifier. If you need to contact us about a specific request, providing the request identifier will ensure the fastest possible resolution.
* @return requestId
*/
@javax.annotation.Nullable
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public BillRunResponse reasons(List reasons) {
this.reasons = reasons;
return this;
}
public BillRunResponse addReasonsItem(FailedReason reasonsItem) {
if (this.reasons == null) {
this.reasons = new ArrayList<>();
}
this.reasons.add(reasonsItem);
return this;
}
/**
* Get reasons
* @return reasons
*/
@javax.annotation.Nullable
public List getReasons() {
return reasons;
}
public void setReasons(List reasons) {
this.reasons = reasons;
}
public BillRunResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Indicates whether the call succeeded.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public BillRunResponse id(String id) {
this.id = id;
return this;
}
/**
* The unique ID of the bill run.
* @return id
*/
@javax.annotation.Nullable
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public BillRunResponse name(String name) {
this.name = name;
return this;
}
/**
* The name of the bill run.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public BillRunResponse billRunNumber(String billRunNumber) {
this.billRunNumber = billRunNumber;
return this;
}
/**
* The number of the bill run.
* @return billRunNumber
*/
@javax.annotation.Nullable
public String getBillRunNumber() {
return billRunNumber;
}
public void setBillRunNumber(String billRunNumber) {
this.billRunNumber = billRunNumber;
}
public BillRunResponse batches(List batches) {
this.batches = batches;
return this;
}
public BillRunResponse addBatchesItem(String batchesItem) {
if (this.batches == null) {
this.batches = new ArrayList<>();
}
this.batches.add(batchesItem);
return this;
}
/**
* The batch of accounts for this bill run, this field can not exist with `billRunFilters` together. **Values:** `AllBatches` or an array of `Batch`*n* where *n* is a number between 1 and 50, for example, `Batch7`.
* @return batches
*/
@javax.annotation.Nullable
public List getBatches() {
return batches;
}
public void setBatches(List batches) {
this.batches = batches;
}
public BillRunResponse billCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* The day of the bill cycle, this field is only valid when `batches` is specified. **Values:** - `AllBillCycleDays` or one of numbers 1 - 31 for an ad-hoc bill run - `AllBillCycleDays`, one of numbers 1 - 31, or `AsRunDay` for a scheduled bill run
* @return billCycleDay
*/
@javax.annotation.Nullable
public String getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(String billCycleDay) {
this.billCycleDay = billCycleDay;
}
public BillRunResponse billRunFilters(List billRunFilters) {
this.billRunFilters = billRunFilters;
return this;
}
public BillRunResponse addBillRunFiltersItem(BillRunFilter billRunFiltersItem) {
if (this.billRunFilters == null) {
this.billRunFilters = new ArrayList<>();
}
this.billRunFilters.add(billRunFiltersItem);
return this;
}
/**
* The target account or subscriptions for this bill run.
* @return billRunFilters
*/
@javax.annotation.Nullable
public List getBillRunFilters() {
return billRunFilters;
}
public void setBillRunFilters(List billRunFilters) {
this.billRunFilters = billRunFilters;
}
public BillRunResponse chargeTypeToExclude(List chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
return this;
}
public BillRunResponse addChargeTypeToExcludeItem(ChargeType chargeTypeToExcludeItem) {
if (this.chargeTypeToExclude == null) {
this.chargeTypeToExclude = new ArrayList<>();
}
this.chargeTypeToExclude.add(chargeTypeToExcludeItem);
return this;
}
/**
* The types of the charges to be excluded from the generation of billing documents.
* @return chargeTypeToExclude
*/
@javax.annotation.Nullable
public List getChargeTypeToExclude() {
return chargeTypeToExclude;
}
public void setChargeTypeToExclude(List chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
}
public BillRunResponse autoEmail(Boolean autoEmail) {
this.autoEmail = autoEmail;
return this;
}
/**
* Whether to automatically send emails after Auto-Post is complete.
* @return autoEmail
*/
@javax.annotation.Nullable
public Boolean getAutoEmail() {
return autoEmail;
}
public void setAutoEmail(Boolean autoEmail) {
this.autoEmail = autoEmail;
}
public BillRunResponse autoPost(Boolean autoPost) {
this.autoPost = autoPost;
return this;
}
/**
* Whether to automatically post the bill run after the bill run is created.
* @return autoPost
*/
@javax.annotation.Nullable
public Boolean getAutoPost() {
return autoPost;
}
public void setAutoPost(Boolean autoPost) {
this.autoPost = autoPost;
}
public BillRunResponse autoRenewal(Boolean autoRenewal) {
this.autoRenewal = autoRenewal;
return this;
}
/**
* Whether to automatically renew auto-renew subscriptions that are up for renewal.
* @return autoRenewal
*/
@javax.annotation.Nullable
public Boolean getAutoRenewal() {
return autoRenewal;
}
public void setAutoRenewal(Boolean autoRenewal) {
this.autoRenewal = autoRenewal;
}
public BillRunResponse noEmailForZeroAmountInvoice(Boolean noEmailForZeroAmountInvoice) {
this.noEmailForZeroAmountInvoice = noEmailForZeroAmountInvoice;
return this;
}
/**
* Whether to suppress emails for invoices with zero total amount generated in this bill run after the bill run is complete.
* @return noEmailForZeroAmountInvoice
*/
@javax.annotation.Nullable
public Boolean getNoEmailForZeroAmountInvoice() {
return noEmailForZeroAmountInvoice;
}
public void setNoEmailForZeroAmountInvoice(Boolean noEmailForZeroAmountInvoice) {
this.noEmailForZeroAmountInvoice = noEmailForZeroAmountInvoice;
}
public BillRunResponse schedule(BillRunSchedule schedule) {
this.schedule = schedule;
return this;
}
/**
* Get schedule
* @return schedule
*/
@javax.annotation.Nullable
public BillRunSchedule getSchedule() {
return schedule;
}
public void setSchedule(BillRunSchedule schedule) {
this.schedule = schedule;
}
public BillRunResponse scheduledExecutionTime(String scheduledExecutionTime) {
this.scheduledExecutionTime = scheduledExecutionTime;
return this;
}
/**
* The scheduled execution time for a bill run.
* @return scheduledExecutionTime
*/
@javax.annotation.Nullable
public String getScheduledExecutionTime() {
return scheduledExecutionTime;
}
public void setScheduledExecutionTime(String scheduledExecutionTime) {
this.scheduledExecutionTime = scheduledExecutionTime;
}
public BillRunResponse status(BillRunStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public BillRunStatus getStatus() {
return status;
}
public void setStatus(BillRunStatus status) {
this.status = status;
}
public BillRunResponse invoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
return this;
}
/**
* The invoice date for this bill run, only valid for ad-hoc bill runs.
* @return invoiceDate
*/
@javax.annotation.Nullable
public LocalDate getInvoiceDate() {
return invoiceDate;
}
public void setInvoiceDate(LocalDate invoiceDate) {
this.invoiceDate = invoiceDate;
}
public BillRunResponse invoiceDateOffset(Integer invoiceDateOffset) {
this.invoiceDateOffset = invoiceDateOffset;
return this;
}
/**
* The offset compared to bill run execution date, only valid for scheduled bill runs.
* @return invoiceDateOffset
*/
@javax.annotation.Nullable
public Integer getInvoiceDateOffset() {
return invoiceDateOffset;
}
public void setInvoiceDateOffset(Integer invoiceDateOffset) {
this.invoiceDateOffset = invoiceDateOffset;
}
public BillRunResponse invoiceDateMonthOffset(Integer invoiceDateMonthOffset) {
this.invoiceDateMonthOffset = invoiceDateMonthOffset;
return this;
}
/**
* The month offset of invoice date for this bill run based on run date, only valid for monthly scheduled bill runs. invoiceDateOffset and invoiceDateMonthOffset/invoiceDateDayOfMonth are mutually exclusive. invoiceDateMonthOffset and invoiceDateDayOfMonth coexist.
* @return invoiceDateMonthOffset
*/
@javax.annotation.Nullable
public Integer getInvoiceDateMonthOffset() {
return invoiceDateMonthOffset;
}
public void setInvoiceDateMonthOffset(Integer invoiceDateMonthOffset) {
this.invoiceDateMonthOffset = invoiceDateMonthOffset;
}
public BillRunResponse invoiceDateDayOfMonth(Integer invoiceDateDayOfMonth) {
this.invoiceDateDayOfMonth = invoiceDateDayOfMonth;
return this;
}
/**
* The day of month of invoice date for this bill run, only valid for monthly scheduled bill runs. The value is between 1 and 31, where 31 = end-of-month. invoiceDateOffset and invoiceDateMonthOffset/invoiceDateDayOfMonth are mutually exclusive. invoiceDateMonthOffset and invoiceDateDayOfMonth coexist.
* minimum: 1
* maximum: 31
* @return invoiceDateDayOfMonth
*/
@javax.annotation.Nullable
public Integer getInvoiceDateDayOfMonth() {
return invoiceDateDayOfMonth;
}
public void setInvoiceDateDayOfMonth(Integer invoiceDateDayOfMonth) {
this.invoiceDateDayOfMonth = invoiceDateDayOfMonth;
}
public BillRunResponse targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date for this bill run, only valid for ad-hoc bill runs.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public BillRunResponse targetDateOffset(Integer targetDateOffset) {
this.targetDateOffset = targetDateOffset;
return this;
}
/**
* The offset compared to bill run execution date, only valid for scheduled bill runs.
* @return targetDateOffset
*/
@javax.annotation.Nullable
public Integer getTargetDateOffset() {
return targetDateOffset;
}
public void setTargetDateOffset(Integer targetDateOffset) {
this.targetDateOffset = targetDateOffset;
}
public BillRunResponse targetDateMonthOffset(Integer targetDateMonthOffset) {
this.targetDateMonthOffset = targetDateMonthOffset;
return this;
}
/**
* The month offset of target date for this bill run based on run date, only valid for monthly scheduled bill runs. targetDateOffset and targetDateMonthOffset/targetDateDayOfMonth are mutually exclusive. targetDateMonthOffset and targetDateDayOfMonth coexist.
* @return targetDateMonthOffset
*/
@javax.annotation.Nullable
public Integer getTargetDateMonthOffset() {
return targetDateMonthOffset;
}
public void setTargetDateMonthOffset(Integer targetDateMonthOffset) {
this.targetDateMonthOffset = targetDateMonthOffset;
}
public BillRunResponse targetDateDayOfMonth(Integer targetDateDayOfMonth) {
this.targetDateDayOfMonth = targetDateDayOfMonth;
return this;
}
/**
* The day of month of target date for this bill run, only valid for monthly scheduled bill runs. The value is between 1 and 31, where 31 = end-of-month. targetDateOffset and targetDateMonthOffset/targetDateDayOfMonth are mutually exclusive. targetDateMonthOffset and targetDateDayOfMonth coexist.
* minimum: 1
* maximum: 31
* @return targetDateDayOfMonth
*/
@javax.annotation.Nullable
public Integer getTargetDateDayOfMonth() {
return targetDateDayOfMonth;
}
public void setTargetDateDayOfMonth(Integer targetDateDayOfMonth) {
this.targetDateDayOfMonth = targetDateDayOfMonth;
}
public BillRunResponse createdById(String createdById) {
this.createdById = createdById;
return this;
}
/**
* The ID of the user who created the bill run.
* @return createdById
*/
@javax.annotation.Nullable
public String getCreatedById() {
return createdById;
}
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
public BillRunResponse createdDate(String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* The date and time when the bill run was created.
* @return createdDate
*/
@javax.annotation.Nullable
public String getCreatedDate() {
return createdDate;
}
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
public BillRunResponse updatedById(String updatedById) {
this.updatedById = updatedById;
return this;
}
/**
* The ID of the user who last updated the bill run.
* @return updatedById
*/
@javax.annotation.Nullable
public String getUpdatedById() {
return updatedById;
}
public void setUpdatedById(String updatedById) {
this.updatedById = updatedById;
}
public BillRunResponse updatedDate(String updatedDate) {
this.updatedDate = updatedDate;
return this;
}
/**
* The date and time when the bill run was last updated.
* @return updatedDate
*/
@javax.annotation.Nullable
public String getUpdatedDate() {
return updatedDate;
}
public void setUpdatedDate(String updatedDate) {
this.updatedDate = updatedDate;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the BillRunResponse instance itself
*/
public BillRunResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BillRunResponse billRunResponse = (BillRunResponse) o;
return Objects.equals(this.processId, billRunResponse.processId) &&
Objects.equals(this.requestId, billRunResponse.requestId) &&
Objects.equals(this.reasons, billRunResponse.reasons) &&
Objects.equals(this.success, billRunResponse.success) &&
Objects.equals(this.id, billRunResponse.id) &&
Objects.equals(this.name, billRunResponse.name) &&
Objects.equals(this.billRunNumber, billRunResponse.billRunNumber) &&
Objects.equals(this.batches, billRunResponse.batches) &&
Objects.equals(this.billCycleDay, billRunResponse.billCycleDay) &&
Objects.equals(this.billRunFilters, billRunResponse.billRunFilters) &&
Objects.equals(this.chargeTypeToExclude, billRunResponse.chargeTypeToExclude) &&
Objects.equals(this.autoEmail, billRunResponse.autoEmail) &&
Objects.equals(this.autoPost, billRunResponse.autoPost) &&
Objects.equals(this.autoRenewal, billRunResponse.autoRenewal) &&
Objects.equals(this.noEmailForZeroAmountInvoice, billRunResponse.noEmailForZeroAmountInvoice) &&
Objects.equals(this.schedule, billRunResponse.schedule) &&
Objects.equals(this.scheduledExecutionTime, billRunResponse.scheduledExecutionTime) &&
Objects.equals(this.status, billRunResponse.status) &&
Objects.equals(this.invoiceDate, billRunResponse.invoiceDate) &&
Objects.equals(this.invoiceDateOffset, billRunResponse.invoiceDateOffset) &&
Objects.equals(this.invoiceDateMonthOffset, billRunResponse.invoiceDateMonthOffset) &&
Objects.equals(this.invoiceDateDayOfMonth, billRunResponse.invoiceDateDayOfMonth) &&
Objects.equals(this.targetDate, billRunResponse.targetDate) &&
Objects.equals(this.targetDateOffset, billRunResponse.targetDateOffset) &&
Objects.equals(this.targetDateMonthOffset, billRunResponse.targetDateMonthOffset) &&
Objects.equals(this.targetDateDayOfMonth, billRunResponse.targetDateDayOfMonth) &&
Objects.equals(this.createdById, billRunResponse.createdById) &&
Objects.equals(this.createdDate, billRunResponse.createdDate) &&
Objects.equals(this.updatedById, billRunResponse.updatedById) &&
Objects.equals(this.updatedDate, billRunResponse.updatedDate)&&
Objects.equals(this.additionalProperties, billRunResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(processId, requestId, reasons, success, id, name, billRunNumber, batches, billCycleDay, billRunFilters, chargeTypeToExclude, autoEmail, autoPost, autoRenewal, noEmailForZeroAmountInvoice, schedule, scheduledExecutionTime, status, invoiceDate, invoiceDateOffset, invoiceDateMonthOffset, invoiceDateDayOfMonth, targetDate, targetDateOffset, targetDateMonthOffset, targetDateDayOfMonth, createdById, createdDate, updatedById, updatedDate, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BillRunResponse {\n");
sb.append(" processId: ").append(toIndentedString(processId)).append("\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append(" reasons: ").append(toIndentedString(reasons)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" billRunNumber: ").append(toIndentedString(billRunNumber)).append("\n");
sb.append(" batches: ").append(toIndentedString(batches)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billRunFilters: ").append(toIndentedString(billRunFilters)).append("\n");
sb.append(" chargeTypeToExclude: ").append(toIndentedString(chargeTypeToExclude)).append("\n");
sb.append(" autoEmail: ").append(toIndentedString(autoEmail)).append("\n");
sb.append(" autoPost: ").append(toIndentedString(autoPost)).append("\n");
sb.append(" autoRenewal: ").append(toIndentedString(autoRenewal)).append("\n");
sb.append(" noEmailForZeroAmountInvoice: ").append(toIndentedString(noEmailForZeroAmountInvoice)).append("\n");
sb.append(" schedule: ").append(toIndentedString(schedule)).append("\n");
sb.append(" scheduledExecutionTime: ").append(toIndentedString(scheduledExecutionTime)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" invoiceDate: ").append(toIndentedString(invoiceDate)).append("\n");
sb.append(" invoiceDateOffset: ").append(toIndentedString(invoiceDateOffset)).append("\n");
sb.append(" invoiceDateMonthOffset: ").append(toIndentedString(invoiceDateMonthOffset)).append("\n");
sb.append(" invoiceDateDayOfMonth: ").append(toIndentedString(invoiceDateDayOfMonth)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" targetDateOffset: ").append(toIndentedString(targetDateOffset)).append("\n");
sb.append(" targetDateMonthOffset: ").append(toIndentedString(targetDateMonthOffset)).append("\n");
sb.append(" targetDateDayOfMonth: ").append(toIndentedString(targetDateDayOfMonth)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedDate: ").append(toIndentedString(updatedDate)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("processId");
openapiFields.add("requestId");
openapiFields.add("reasons");
openapiFields.add("success");
openapiFields.add("id");
openapiFields.add("name");
openapiFields.add("billRunNumber");
openapiFields.add("batches");
openapiFields.add("billCycleDay");
openapiFields.add("billRunFilters");
openapiFields.add("chargeTypeToExclude");
openapiFields.add("autoEmail");
openapiFields.add("autoPost");
openapiFields.add("autoRenewal");
openapiFields.add("noEmailForZeroAmountInvoice");
openapiFields.add("schedule");
openapiFields.add("scheduledExecutionTime");
openapiFields.add("status");
openapiFields.add("invoiceDate");
openapiFields.add("invoiceDateOffset");
openapiFields.add("invoiceDateMonthOffset");
openapiFields.add("invoiceDateDayOfMonth");
openapiFields.add("targetDate");
openapiFields.add("targetDateOffset");
openapiFields.add("targetDateMonthOffset");
openapiFields.add("targetDateDayOfMonth");
openapiFields.add("createdById");
openapiFields.add("createdDate");
openapiFields.add("updatedById");
openapiFields.add("updatedDate");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to BillRunResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!BillRunResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in BillRunResponse is not found in the empty JSON string", BillRunResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("processId") != null && !jsonObj.get("processId").isJsonNull()) && !jsonObj.get("processId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processId").toString()));
}
if ((jsonObj.get("requestId") != null && !jsonObj.get("requestId").isJsonNull()) && !jsonObj.get("requestId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `requestId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("requestId").toString()));
}
if (jsonObj.get("reasons") != null && !jsonObj.get("reasons").isJsonNull()) {
JsonArray jsonArrayreasons = jsonObj.getAsJsonArray("reasons");
if (jsonArrayreasons != null) {
// ensure the json data is an array
if (!jsonObj.get("reasons").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `reasons` to be an array in the JSON string but got `%s`", jsonObj.get("reasons").toString()));
}
// validate the optional field `reasons` (array)
for (int i = 0; i < jsonArrayreasons.size(); i++) {
FailedReason.validateJsonElement(jsonArrayreasons.get(i));
};
}
}
if ((jsonObj.get("id") != null && !jsonObj.get("id").isJsonNull()) && !jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("billRunNumber") != null && !jsonObj.get("billRunNumber").isJsonNull()) && !jsonObj.get("billRunNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billRunNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billRunNumber").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("batches") != null && !jsonObj.get("batches").isJsonNull() && !jsonObj.get("batches").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `batches` to be an array in the JSON string but got `%s`", jsonObj.get("batches").toString()));
}
if ((jsonObj.get("billCycleDay") != null && !jsonObj.get("billCycleDay").isJsonNull()) && !jsonObj.get("billCycleDay").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `billCycleDay` to be a primitive type in the JSON string but got `%s`", jsonObj.get("billCycleDay").toString()));
}
if (jsonObj.get("billRunFilters") != null && !jsonObj.get("billRunFilters").isJsonNull()) {
JsonArray jsonArraybillRunFilters = jsonObj.getAsJsonArray("billRunFilters");
if (jsonArraybillRunFilters != null) {
// ensure the json data is an array
if (!jsonObj.get("billRunFilters").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `billRunFilters` to be an array in the JSON string but got `%s`", jsonObj.get("billRunFilters").toString()));
}
// validate the optional field `billRunFilters` (array)
for (int i = 0; i < jsonArraybillRunFilters.size(); i++) {
BillRunFilter.validateJsonElement(jsonArraybillRunFilters.get(i));
};
}
}
// ensure the optional json data is an array if present
if (jsonObj.get("chargeTypeToExclude") != null && !jsonObj.get("chargeTypeToExclude").isJsonNull() && !jsonObj.get("chargeTypeToExclude").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeTypeToExclude` to be an array in the JSON string but got `%s`", jsonObj.get("chargeTypeToExclude").toString()));
}
// validate the optional field `schedule`
if (jsonObj.get("schedule") != null && !jsonObj.get("schedule").isJsonNull()) {
BillRunSchedule.validateJsonElement(jsonObj.get("schedule"));
}
if ((jsonObj.get("scheduledExecutionTime") != null && !jsonObj.get("scheduledExecutionTime").isJsonNull()) && !jsonObj.get("scheduledExecutionTime").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `scheduledExecutionTime` to be a primitive type in the JSON string but got `%s`", jsonObj.get("scheduledExecutionTime").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
BillRunStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("createdById") != null && !jsonObj.get("createdById").isJsonNull()) && !jsonObj.get("createdById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdById").toString()));
}
if ((jsonObj.get("createdDate") != null && !jsonObj.get("createdDate").isJsonNull()) && !jsonObj.get("createdDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `createdDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("createdDate").toString()));
}
if ((jsonObj.get("updatedById") != null && !jsonObj.get("updatedById").isJsonNull()) && !jsonObj.get("updatedById").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedById` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedById").toString()));
}
if ((jsonObj.get("updatedDate") != null && !jsonObj.get("updatedDate").isJsonNull()) && !jsonObj.get("updatedDate").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `updatedDate` to be a primitive type in the JSON string but got `%s`", jsonObj.get("updatedDate").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!BillRunResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'BillRunResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(BillRunResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, BillRunResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public BillRunResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
BillRunResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of BillRunResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of BillRunResponse
* @throws IOException if the JSON string is invalid with respect to BillRunResponse
*/
public static BillRunResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, BillRunResponse.class);
}
/**
* Convert an instance of BillRunResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy