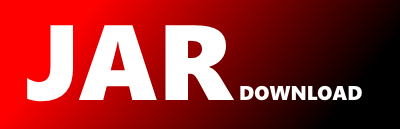
com.zuora.model.CancelSubscriptionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateOrderResponseSubscriptions;
import com.zuora.model.OrderStatus;
import java.io.IOException;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CancelSubscriptionResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CancelSubscriptionResponse {
public static final String SERIALIZED_NAME_CANCELLED_DATE = "cancelledDate";
@SerializedName(SERIALIZED_NAME_CANCELLED_DATE)
private LocalDate cancelledDate;
public static final String SERIALIZED_NAME_CREDIT_MEMO_ID = "creditMemoId";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_ID)
private String creditMemoId;
public static final String SERIALIZED_NAME_INVOICE_ID = "invoiceId";
@SerializedName(SERIALIZED_NAME_INVOICE_ID)
private String invoiceId;
public static final String SERIALIZED_NAME_PAID_AMOUNT = "paidAmount";
@SerializedName(SERIALIZED_NAME_PAID_AMOUNT)
private BigDecimal paidAmount;
public static final String SERIALIZED_NAME_PAYMENT_ID = "paymentId";
@SerializedName(SERIALIZED_NAME_PAYMENT_ID)
private String paymentId;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ID = "subscriptionId";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ID)
private String subscriptionId;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_TOTAL_DELTA_MRR = "totalDeltaMrr";
@SerializedName(SERIALIZED_NAME_TOTAL_DELTA_MRR)
private BigDecimal totalDeltaMrr;
public static final String SERIALIZED_NAME_TOTAL_DELTA_TCV = "totalDeltaTcv";
@SerializedName(SERIALIZED_NAME_TOTAL_DELTA_TCV)
private BigDecimal totalDeltaTcv;
public static final String SERIALIZED_NAME_ORDER_NUMBER = "orderNumber";
@SerializedName(SERIALIZED_NAME_ORDER_NUMBER)
private String orderNumber;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private OrderStatus status;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBERS = "subscriptionNumbers";
@Deprecated
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBERS)
private List subscriptionNumbers;
public static final String SERIALIZED_NAME_SUBSCRIPTIONS = "subscriptions";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTIONS)
private List subscriptions;
public CancelSubscriptionResponse() {
}
public CancelSubscriptionResponse cancelledDate(LocalDate cancelledDate) {
this.cancelledDate = cancelledDate;
return this;
}
/**
* The date that the subscription was canceled. It is available for Orders Harmonization and Subscribe/Amend tenants.
* @return cancelledDate
*/
@javax.annotation.Nullable
public LocalDate getCancelledDate() {
return cancelledDate;
}
public void setCancelledDate(LocalDate cancelledDate) {
this.cancelledDate = cancelledDate;
}
public CancelSubscriptionResponse creditMemoId(String creditMemoId) {
this.creditMemoId = creditMemoId;
return this;
}
/**
* The credit memo ID, if a credit memo is generated during the subscription process. **Note:** This container is only available if you set the Zuora REST API minor version to 207.0 or later in the request header, and you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return creditMemoId
*/
@javax.annotation.Nullable
public String getCreditMemoId() {
return creditMemoId;
}
public void setCreditMemoId(String creditMemoId) {
this.creditMemoId = creditMemoId;
}
public CancelSubscriptionResponse invoiceId(String invoiceId) {
this.invoiceId = invoiceId;
return this;
}
/**
* ID of the invoice, if one is generated.
* @return invoiceId
*/
@javax.annotation.Nullable
public String getInvoiceId() {
return invoiceId;
}
public void setInvoiceId(String invoiceId) {
this.invoiceId = invoiceId;
}
public CancelSubscriptionResponse paidAmount(BigDecimal paidAmount) {
this.paidAmount = paidAmount;
return this;
}
/**
* Amount paid.
* @return paidAmount
*/
@javax.annotation.Nullable
public BigDecimal getPaidAmount() {
return paidAmount;
}
public void setPaidAmount(BigDecimal paidAmount) {
this.paidAmount = paidAmount;
}
public CancelSubscriptionResponse paymentId(String paymentId) {
this.paymentId = paymentId;
return this;
}
/**
* ID of the payment, if a payment is collected.
* @return paymentId
*/
@javax.annotation.Nullable
public String getPaymentId() {
return paymentId;
}
public void setPaymentId(String paymentId) {
this.paymentId = paymentId;
}
public CancelSubscriptionResponse subscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* The subscription ID. It is available for Orders Harmonization and Subscribe/Amend Tenants.
* @return subscriptionId
*/
@javax.annotation.Nullable
public String getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
}
public CancelSubscriptionResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Returns `true` if the request was processed successfully.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public CancelSubscriptionResponse totalDeltaMrr(BigDecimal totalDeltaMrr) {
this.totalDeltaMrr = totalDeltaMrr;
return this;
}
/**
* Change in the subscription monthly recurring revenue as a result of the update. It is available for Orders Harmonization and Subscribe/Amend tenants.
* @return totalDeltaMrr
*/
@javax.annotation.Nullable
public BigDecimal getTotalDeltaMrr() {
return totalDeltaMrr;
}
public void setTotalDeltaMrr(BigDecimal totalDeltaMrr) {
this.totalDeltaMrr = totalDeltaMrr;
}
public CancelSubscriptionResponse totalDeltaTcv(BigDecimal totalDeltaTcv) {
this.totalDeltaTcv = totalDeltaTcv;
return this;
}
/**
* Change in the total contracted value of the subscription as a result of the update. It is available for Orders Harmonization and Subscribe/Amend tenants.
* @return totalDeltaTcv
*/
@javax.annotation.Nullable
public BigDecimal getTotalDeltaTcv() {
return totalDeltaTcv;
}
public void setTotalDeltaTcv(BigDecimal totalDeltaTcv) {
this.totalDeltaTcv = totalDeltaTcv;
}
public CancelSubscriptionResponse orderNumber(String orderNumber) {
this.orderNumber = orderNumber;
return this;
}
/**
* The order number. It is available for Orders Tenants.
* @return orderNumber
*/
@javax.annotation.Nullable
public String getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(String orderNumber) {
this.orderNumber = orderNumber;
}
public CancelSubscriptionResponse status(OrderStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nullable
public OrderStatus getStatus() {
return status;
}
public void setStatus(OrderStatus status) {
this.status = status;
}
public CancelSubscriptionResponse accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The account number that this order has been created under. This is also the invoice owner of the subscriptions included in this order. It is available for Orders Tenants.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
@Deprecated
public CancelSubscriptionResponse subscriptionNumbers(List subscriptionNumbers) {
this.subscriptionNumbers = subscriptionNumbers;
return this;
}
public CancelSubscriptionResponse addSubscriptionNumbersItem(String subscriptionNumbersItem) {
if (this.subscriptionNumbers == null) {
this.subscriptionNumbers = new ArrayList<>();
}
this.subscriptionNumbers.add(subscriptionNumbersItem);
return this;
}
/**
* The subscription numbers. It is available for Orders Tenants. This field is in Zuora REST API version control. Supported max version is 206.0.
* @return subscriptionNumbers
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public List getSubscriptionNumbers() {
return subscriptionNumbers;
}
@Deprecated
public void setSubscriptionNumbers(List subscriptionNumbers) {
this.subscriptionNumbers = subscriptionNumbers;
}
public CancelSubscriptionResponse subscriptions(List subscriptions) {
this.subscriptions = subscriptions;
return this;
}
public CancelSubscriptionResponse addSubscriptionsItem(CreateOrderResponseSubscriptions subscriptionsItem) {
if (this.subscriptions == null) {
this.subscriptions = new ArrayList<>();
}
this.subscriptions.add(subscriptionsItem);
return this;
}
/**
* This field is in Zuora REST API version control. Supported minor versions are 223.0 or later. It is available for Orders Tenants.
* @return subscriptions
*/
@javax.annotation.Nullable
public List getSubscriptions() {
return subscriptions;
}
public void setSubscriptions(List subscriptions) {
this.subscriptions = subscriptions;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CancelSubscriptionResponse instance itself
*/
public CancelSubscriptionResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CancelSubscriptionResponse cancelSubscriptionResponse = (CancelSubscriptionResponse) o;
return Objects.equals(this.cancelledDate, cancelSubscriptionResponse.cancelledDate) &&
Objects.equals(this.creditMemoId, cancelSubscriptionResponse.creditMemoId) &&
Objects.equals(this.invoiceId, cancelSubscriptionResponse.invoiceId) &&
Objects.equals(this.paidAmount, cancelSubscriptionResponse.paidAmount) &&
Objects.equals(this.paymentId, cancelSubscriptionResponse.paymentId) &&
Objects.equals(this.subscriptionId, cancelSubscriptionResponse.subscriptionId) &&
Objects.equals(this.success, cancelSubscriptionResponse.success) &&
Objects.equals(this.totalDeltaMrr, cancelSubscriptionResponse.totalDeltaMrr) &&
Objects.equals(this.totalDeltaTcv, cancelSubscriptionResponse.totalDeltaTcv) &&
Objects.equals(this.orderNumber, cancelSubscriptionResponse.orderNumber) &&
Objects.equals(this.status, cancelSubscriptionResponse.status) &&
Objects.equals(this.accountNumber, cancelSubscriptionResponse.accountNumber) &&
Objects.equals(this.subscriptionNumbers, cancelSubscriptionResponse.subscriptionNumbers) &&
Objects.equals(this.subscriptions, cancelSubscriptionResponse.subscriptions)&&
Objects.equals(this.additionalProperties, cancelSubscriptionResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(cancelledDate, creditMemoId, invoiceId, paidAmount, paymentId, subscriptionId, success, totalDeltaMrr, totalDeltaTcv, orderNumber, status, accountNumber, subscriptionNumbers, subscriptions, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CancelSubscriptionResponse {\n");
sb.append(" cancelledDate: ").append(toIndentedString(cancelledDate)).append("\n");
sb.append(" creditMemoId: ").append(toIndentedString(creditMemoId)).append("\n");
sb.append(" invoiceId: ").append(toIndentedString(invoiceId)).append("\n");
sb.append(" paidAmount: ").append(toIndentedString(paidAmount)).append("\n");
sb.append(" paymentId: ").append(toIndentedString(paymentId)).append("\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" totalDeltaMrr: ").append(toIndentedString(totalDeltaMrr)).append("\n");
sb.append(" totalDeltaTcv: ").append(toIndentedString(totalDeltaTcv)).append("\n");
sb.append(" orderNumber: ").append(toIndentedString(orderNumber)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" subscriptionNumbers: ").append(toIndentedString(subscriptionNumbers)).append("\n");
sb.append(" subscriptions: ").append(toIndentedString(subscriptions)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("cancelledDate");
openapiFields.add("creditMemoId");
openapiFields.add("invoiceId");
openapiFields.add("paidAmount");
openapiFields.add("paymentId");
openapiFields.add("subscriptionId");
openapiFields.add("success");
openapiFields.add("totalDeltaMrr");
openapiFields.add("totalDeltaTcv");
openapiFields.add("orderNumber");
openapiFields.add("status");
openapiFields.add("accountNumber");
openapiFields.add("subscriptionNumbers");
openapiFields.add("subscriptions");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CancelSubscriptionResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CancelSubscriptionResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CancelSubscriptionResponse is not found in the empty JSON string", CancelSubscriptionResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("creditMemoId") != null && !jsonObj.get("creditMemoId").isJsonNull()) && !jsonObj.get("creditMemoId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoId").toString()));
}
if ((jsonObj.get("invoiceId") != null && !jsonObj.get("invoiceId").isJsonNull()) && !jsonObj.get("invoiceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceId").toString()));
}
if ((jsonObj.get("paymentId") != null && !jsonObj.get("paymentId").isJsonNull()) && !jsonObj.get("paymentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentId").toString()));
}
if ((jsonObj.get("subscriptionId") != null && !jsonObj.get("subscriptionId").isJsonNull()) && !jsonObj.get("subscriptionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionId").toString()));
}
if ((jsonObj.get("orderNumber") != null && !jsonObj.get("orderNumber").isJsonNull()) && !jsonObj.get("orderNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `orderNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("orderNumber").toString()));
}
if ((jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) && !jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// validate the optional field `status`
if (jsonObj.get("status") != null && !jsonObj.get("status").isJsonNull()) {
OrderStatus.validateJsonElement(jsonObj.get("status"));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("subscriptionNumbers") != null && !jsonObj.get("subscriptionNumbers").isJsonNull() && !jsonObj.get("subscriptionNumbers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionNumbers` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptionNumbers").toString()));
}
if (jsonObj.get("subscriptions") != null && !jsonObj.get("subscriptions").isJsonNull()) {
JsonArray jsonArraysubscriptions = jsonObj.getAsJsonArray("subscriptions");
if (jsonArraysubscriptions != null) {
// ensure the json data is an array
if (!jsonObj.get("subscriptions").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptions` to be an array in the JSON string but got `%s`", jsonObj.get("subscriptions").toString()));
}
// validate the optional field `subscriptions` (array)
for (int i = 0; i < jsonArraysubscriptions.size(); i++) {
CreateOrderResponseSubscriptions.validateJsonElement(jsonArraysubscriptions.get(i));
};
}
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CancelSubscriptionResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CancelSubscriptionResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CancelSubscriptionResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CancelSubscriptionResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CancelSubscriptionResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CancelSubscriptionResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CancelSubscriptionResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of CancelSubscriptionResponse
* @throws IOException if the JSON string is invalid with respect to CancelSubscriptionResponse
*/
public static CancelSubscriptionResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CancelSubscriptionResponse.class);
}
/**
* Convert an instance of CancelSubscriptionResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy