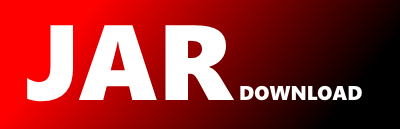
com.zuora.model.ChangeSubscriptionRatePlan Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.AddSubscriptionComponent;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* ChangeSubscriptionRatePlan
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ChangeSubscriptionRatePlan {
public static final String SERIALIZED_NAME_BOOKING_DATE = "bookingDate";
@SerializedName(SERIALIZED_NAME_BOOKING_DATE)
private LocalDate bookingDate;
public static final String SERIALIZED_NAME_CHARGE_OVERRIDES = "chargeOverrides";
@SerializedName(SERIALIZED_NAME_CHARGE_OVERRIDES)
private List chargeOverrides;
public static final String SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE = "contractEffectiveDate";
@SerializedName(SERIALIZED_NAME_CONTRACT_EFFECTIVE_DATE)
private LocalDate contractEffectiveDate;
public static final String SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE = "customerAcceptanceDate";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCEPTANCE_DATE)
private LocalDate customerAcceptanceDate;
/**
* The default value for the `effectivePolicy` field is as follows: * If the rate plan change (from old to new) is an upgrade, the effective policy is `EffectiveImmediately` by default. * If the rate plan change (from old to new) is a downgrade, the effective policy is `EffectiveEndOfBillingPeriod` by default. * Otherwise, the effective policy is `SpecificDate` by default. Note that if the `effectivePolicy` field is set to `EffectiveEndOfBillingPeriod`, you cannot set the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Subscriptions/Subscriptions/W_Subscription_and_Amendment_Dates#Billing_Trigger_Dates\" target=\"_blank\">billing trigger dates</a> for the subscription as the system will automatically set the trigger dates to the end of billing period.
*/
@JsonAdapter(EffectivePolicyEnum.Adapter.class)
public enum EffectivePolicyEnum {
EFFECTIVEIMMEDIATELY("EffectiveImmediately"),
EFFECTIVEENDOFBILLINGPERIOD("EffectiveEndOfBillingPeriod"),
SPECIFICDATE("SpecificDate");
private String value;
EffectivePolicyEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static EffectivePolicyEnum fromValue(String value) {
for (EffectivePolicyEnum b : EffectivePolicyEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final EffectivePolicyEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public EffectivePolicyEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return EffectivePolicyEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
EffectivePolicyEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_EFFECTIVE_POLICY = "effectivePolicy";
@SerializedName(SERIALIZED_NAME_EFFECTIVE_POLICY)
private EffectivePolicyEnum effectivePolicy;
public static final String SERIALIZED_NAME_EXTERNAL_CATALOG_PLAN_ID = "externalCatalogPlanId";
@SerializedName(SERIALIZED_NAME_EXTERNAL_CATALOG_PLAN_ID)
private String externalCatalogPlanId;
public static final String SERIALIZED_NAME_EXTERNAL_ID_SOURCE_SYSTEM = "externalIdSourceSystem";
@SerializedName(SERIALIZED_NAME_EXTERNAL_ID_SOURCE_SYSTEM)
private String externalIdSourceSystem;
public static final String SERIALIZED_NAME_NEW_EXTERNAL_CATALOG_PLAN_ID = "newExternalCatalogPlanId";
@SerializedName(SERIALIZED_NAME_NEW_EXTERNAL_CATALOG_PLAN_ID)
private String newExternalCatalogPlanId;
public static final String SERIALIZED_NAME_NEW_EXTERNAL_ID_SOURCE_SYSTEM = "newExternalIdSourceSystem";
@SerializedName(SERIALIZED_NAME_NEW_EXTERNAL_ID_SOURCE_SYSTEM)
private String newExternalIdSourceSystem;
public static final String SERIALIZED_NAME_NEW_PRODUCT_RATE_PLAN_ID = "newProductRatePlanId";
@SerializedName(SERIALIZED_NAME_NEW_PRODUCT_RATE_PLAN_ID)
private String newProductRatePlanId;
public static final String SERIALIZED_NAME_NEW_PRODUCT_RATE_PLAN_NUMBER = "newProductRatePlanNumber";
@SerializedName(SERIALIZED_NAME_NEW_PRODUCT_RATE_PLAN_NUMBER)
private String newProductRatePlanNumber;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID = "productRatePlanId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_ID)
private String productRatePlanId;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_NUMBER = "productRatePlanNumber";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_NUMBER)
private String productRatePlanNumber;
public static final String SERIALIZED_NAME_RATE_PLAN_ID = "ratePlanId";
@SerializedName(SERIALIZED_NAME_RATE_PLAN_ID)
private String ratePlanId;
public static final String SERIALIZED_NAME_RESET_BCD = "resetBcd";
@SerializedName(SERIALIZED_NAME_RESET_BCD)
private Boolean resetBcd = false;
public static final String SERIALIZED_NAME_SERVICE_ACTIVATION_DATE = "serviceActivationDate";
@SerializedName(SERIALIZED_NAME_SERVICE_ACTIVATION_DATE)
private LocalDate serviceActivationDate;
/**
* Use this field to choose the sub type for your change plan amendment. However, if you do not set this field, the field will be automatically generated by the system according to the following rules: When the old and new rate plans are within the same Grading catalog group: * If the grade of new plan is greater than that of the old plan, this is an \"Upgrade\". * If the grade of new plan is less than that of the old plan, this is a \"Downgrade\". * If the grade of new plan equals that of the old plan, this is a \"Crossgrade\". When the old and new rate plans are not in the same Grading catalog group, or either has no group, this is \"PlanChanged\".
*/
@JsonAdapter(SubTypeEnum.Adapter.class)
public enum SubTypeEnum {
UPGRADE("Upgrade"),
DOWNGRADE("Downgrade"),
CROSSGRADE("Crossgrade"),
PLANCHANGED("PlanChanged");
private String value;
SubTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static SubTypeEnum fromValue(String value) {
for (SubTypeEnum b : SubTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final SubTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public SubTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return SubTypeEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
SubTypeEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_SUB_TYPE = "subType";
@SerializedName(SERIALIZED_NAME_SUB_TYPE)
private SubTypeEnum subType;
public static final String SERIALIZED_NAME_SUBSCRIPTION_RATE_PLAN_NUMBER = "subscriptionRatePlanNumber";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_RATE_PLAN_NUMBER)
private String subscriptionRatePlanNumber;
public ChangeSubscriptionRatePlan() {
}
public ChangeSubscriptionRatePlan bookingDate(LocalDate bookingDate) {
this.bookingDate = bookingDate;
return this;
}
/**
* The booking date that you want to set for the amendment contract. The booking date of an amendment is the equivalent of the order date of an order. This field must be in the `yyyy-mm-dd` format. The default value is the current date when you make the API call.
* @return bookingDate
*/
@javax.annotation.Nullable
public LocalDate getBookingDate() {
return bookingDate;
}
public void setBookingDate(LocalDate bookingDate) {
this.bookingDate = bookingDate;
}
public ChangeSubscriptionRatePlan chargeOverrides(List chargeOverrides) {
this.chargeOverrides = chargeOverrides;
return this;
}
public ChangeSubscriptionRatePlan addChargeOverridesItem(AddSubscriptionComponent chargeOverridesItem) {
if (this.chargeOverrides == null) {
this.chargeOverrides = new ArrayList<>();
}
this.chargeOverrides.add(chargeOverridesItem);
return this;
}
/**
* This optional container is used to override one or more product rate plan charges for this subscription.
* @return chargeOverrides
*/
@javax.annotation.Nullable
public List getChargeOverrides() {
return chargeOverrides;
}
public void setChargeOverrides(List chargeOverrides) {
this.chargeOverrides = chargeOverrides;
}
public ChangeSubscriptionRatePlan contractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
return this;
}
/**
* Effective date of the new subscription, as yyyy-mm-dd.
* @return contractEffectiveDate
*/
@javax.annotation.Nullable
public LocalDate getContractEffectiveDate() {
return contractEffectiveDate;
}
public void setContractEffectiveDate(LocalDate contractEffectiveDate) {
this.contractEffectiveDate = contractEffectiveDate;
}
public ChangeSubscriptionRatePlan customerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
return this;
}
/**
* The date when the customer accepts the contract in yyyy-mm-dd format. When this field is not set: * If the `serviceActivationDate` field is not set, the value of this field is set to be the contract effective date. * If the `serviceActivationDate` field is set, the value of this field is set to be the service activation date. The billing trigger dates must follow this rule: contractEffectiveDate <= serviceActivationDate <= contractAcceptanceDate
* @return customerAcceptanceDate
*/
@javax.annotation.Nullable
public LocalDate getCustomerAcceptanceDate() {
return customerAcceptanceDate;
}
public void setCustomerAcceptanceDate(LocalDate customerAcceptanceDate) {
this.customerAcceptanceDate = customerAcceptanceDate;
}
public ChangeSubscriptionRatePlan effectivePolicy(EffectivePolicyEnum effectivePolicy) {
this.effectivePolicy = effectivePolicy;
return this;
}
/**
* The default value for the `effectivePolicy` field is as follows: * If the rate plan change (from old to new) is an upgrade, the effective policy is `EffectiveImmediately` by default. * If the rate plan change (from old to new) is a downgrade, the effective policy is `EffectiveEndOfBillingPeriod` by default. * Otherwise, the effective policy is `SpecificDate` by default. Note that if the `effectivePolicy` field is set to `EffectiveEndOfBillingPeriod`, you cannot set the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Subscriptions/Subscriptions/W_Subscription_and_Amendment_Dates#Billing_Trigger_Dates\" target=\"_blank\">billing trigger dates</a> for the subscription as the system will automatically set the trigger dates to the end of billing period.
* @return effectivePolicy
*/
@javax.annotation.Nullable
public EffectivePolicyEnum getEffectivePolicy() {
return effectivePolicy;
}
public void setEffectivePolicy(EffectivePolicyEnum effectivePolicy) {
this.effectivePolicy = effectivePolicy;
}
public ChangeSubscriptionRatePlan externalCatalogPlanId(String externalCatalogPlanId) {
this.externalCatalogPlanId = externalCatalogPlanId;
return this;
}
/**
* An external ID of the rate plan to be removed. You can use this field to specify an existing rate plan in your subscription. The value of the `externalCatalogPlanId` field must match one of the values that are predefined in the `externallyManagedPlanIds` field on a product rate plan. However, if there are multiple rate plans with the same `productRatePlanId` value existing in the subscription, you must use the `ratePlanId` field to remove the rate plan. The `externalCatalogPlanId` field cannot be used to distinguish multiple rate plans in this case. **Note:** Provide only one of `externalCatalogPlanId`, `ratePlanId` or `productRatePlanId`. If more than one field is provided then the request would fail.
* @return externalCatalogPlanId
*/
@javax.annotation.Nullable
public String getExternalCatalogPlanId() {
return externalCatalogPlanId;
}
public void setExternalCatalogPlanId(String externalCatalogPlanId) {
this.externalCatalogPlanId = externalCatalogPlanId;
}
public ChangeSubscriptionRatePlan externalIdSourceSystem(String externalIdSourceSystem) {
this.externalIdSourceSystem = externalIdSourceSystem;
return this;
}
/**
* The ID of the external source system. You can use this field and `externalCatalogPlanId` to specify a product rate plan that is imported from an external system. **Note:** If both `externalCatalogPlanId`, `externalIdSourceSystem` and `productRatePlanId` are provided. They must point to the same product rate plan. Otherwise, the request would fail.
* @return externalIdSourceSystem
*/
@javax.annotation.Nullable
public String getExternalIdSourceSystem() {
return externalIdSourceSystem;
}
public void setExternalIdSourceSystem(String externalIdSourceSystem) {
this.externalIdSourceSystem = externalIdSourceSystem;
}
public ChangeSubscriptionRatePlan newExternalCatalogPlanId(String newExternalCatalogPlanId) {
this.newExternalCatalogPlanId = newExternalCatalogPlanId;
return this;
}
/**
* An external ID of the product rate plan to be added. You can use this field to specify a product rate plan that is imported from an external system. The value of the `externalCatalogPlanId` field must match one of the values that are predefined in the `externallyManagedPlanIds` field on a product rate plan. **Note:** Provide only one of `newExternalCatalogPlanId` or `newProductRatePlanId`. If both fields are provided then the request would fail.
* @return newExternalCatalogPlanId
*/
@javax.annotation.Nullable
public String getNewExternalCatalogPlanId() {
return newExternalCatalogPlanId;
}
public void setNewExternalCatalogPlanId(String newExternalCatalogPlanId) {
this.newExternalCatalogPlanId = newExternalCatalogPlanId;
}
public ChangeSubscriptionRatePlan newExternalIdSourceSystem(String newExternalIdSourceSystem) {
this.newExternalIdSourceSystem = newExternalIdSourceSystem;
return this;
}
/**
* The ID of the external source system. You can use this field and `newExternalCatalogPlanId` to specify a product rate plan that is imported from an external system. **Note:** If both `newExternalCatalogPlanId`, `newExternalIdSourceSystem` and `newProductRatePlanId` are provided. They must point to the same product rate plan. Otherwise, the request would fail.
* @return newExternalIdSourceSystem
*/
@javax.annotation.Nullable
public String getNewExternalIdSourceSystem() {
return newExternalIdSourceSystem;
}
public void setNewExternalIdSourceSystem(String newExternalIdSourceSystem) {
this.newExternalIdSourceSystem = newExternalIdSourceSystem;
}
public ChangeSubscriptionRatePlan newProductRatePlanId(String newProductRatePlanId) {
this.newProductRatePlanId = newProductRatePlanId;
return this;
}
/**
* ID of a product rate plan for this subscription.
* @return newProductRatePlanId
*/
@javax.annotation.Nullable
public String getNewProductRatePlanId() {
return newProductRatePlanId;
}
public void setNewProductRatePlanId(String newProductRatePlanId) {
this.newProductRatePlanId = newProductRatePlanId;
}
public ChangeSubscriptionRatePlan newProductRatePlanNumber(String newProductRatePlanNumber) {
this.newProductRatePlanNumber = newProductRatePlanNumber;
return this;
}
/**
* Number of a product rate plan for this subscription.
* @return newProductRatePlanNumber
*/
@javax.annotation.Nullable
public String getNewProductRatePlanNumber() {
return newProductRatePlanNumber;
}
public void setNewProductRatePlanNumber(String newProductRatePlanNumber) {
this.newProductRatePlanNumber = newProductRatePlanNumber;
}
public ChangeSubscriptionRatePlan productRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
return this;
}
/**
* ID of the product rate plan that the removed rate plan is based on.
* @return productRatePlanId
*/
@javax.annotation.Nullable
public String getProductRatePlanId() {
return productRatePlanId;
}
public void setProductRatePlanId(String productRatePlanId) {
this.productRatePlanId = productRatePlanId;
}
public ChangeSubscriptionRatePlan productRatePlanNumber(String productRatePlanNumber) {
this.productRatePlanNumber = productRatePlanNumber;
return this;
}
/**
* Number of a product rate plan for this subscription.
* @return productRatePlanNumber
*/
@javax.annotation.Nullable
public String getProductRatePlanNumber() {
return productRatePlanNumber;
}
public void setProductRatePlanNumber(String productRatePlanNumber) {
this.productRatePlanNumber = productRatePlanNumber;
}
public ChangeSubscriptionRatePlan ratePlanId(String ratePlanId) {
this.ratePlanId = ratePlanId;
return this;
}
/**
* ID of a rate plan to remove. Note that the removal of a rate plan through the Change Plan amendment supports the function of <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Subscriptions/Subscriptions/Subscribe_and_Amend/E_Amendments/EB_Remove_rate_plan_on_subscription_before_future-dated_removals\" target=\"_blank\">removal before future-dated removals</a>, as in a Remove Product amendment.
* @return ratePlanId
*/
@javax.annotation.Nullable
public String getRatePlanId() {
return ratePlanId;
}
public void setRatePlanId(String ratePlanId) {
this.ratePlanId = ratePlanId;
}
public ChangeSubscriptionRatePlan resetBcd(Boolean resetBcd) {
this.resetBcd = resetBcd;
return this;
}
/**
* If resetBcd is true then reset the Account BCD to the effective date; if it is false keep the original BCD.
* @return resetBcd
*/
@javax.annotation.Nullable
public Boolean getResetBcd() {
return resetBcd;
}
public void setResetBcd(Boolean resetBcd) {
this.resetBcd = resetBcd;
}
public ChangeSubscriptionRatePlan serviceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
return this;
}
/**
* The date when the change in the subscription is activated in yyyy-mm-dd format. You must specify a Service Activation date if the Customer Acceptance date is set. If the Customer Acceptance date is not set, the value of the `serviceActivationDate` field defaults to be the Contract Effective Date. The billing trigger dates must follow this rule: contractEffectiveDate <= serviceActivationDate <= contractAcceptanceDate
* @return serviceActivationDate
*/
@javax.annotation.Nullable
public LocalDate getServiceActivationDate() {
return serviceActivationDate;
}
public void setServiceActivationDate(LocalDate serviceActivationDate) {
this.serviceActivationDate = serviceActivationDate;
}
public ChangeSubscriptionRatePlan subType(SubTypeEnum subType) {
this.subType = subType;
return this;
}
/**
* Use this field to choose the sub type for your change plan amendment. However, if you do not set this field, the field will be automatically generated by the system according to the following rules: When the old and new rate plans are within the same Grading catalog group: * If the grade of new plan is greater than that of the old plan, this is an \"Upgrade\". * If the grade of new plan is less than that of the old plan, this is a \"Downgrade\". * If the grade of new plan equals that of the old plan, this is a \"Crossgrade\". When the old and new rate plans are not in the same Grading catalog group, or either has no group, this is \"PlanChanged\".
* @return subType
*/
@javax.annotation.Nullable
public SubTypeEnum getSubType() {
return subType;
}
public void setSubType(SubTypeEnum subType) {
this.subType = subType;
}
public ChangeSubscriptionRatePlan subscriptionRatePlanNumber(String subscriptionRatePlanNumber) {
this.subscriptionRatePlanNumber = subscriptionRatePlanNumber;
return this;
}
/**
* Number of a rate plan for this subscription.
* @return subscriptionRatePlanNumber
*/
@javax.annotation.Nullable
public String getSubscriptionRatePlanNumber() {
return subscriptionRatePlanNumber;
}
public void setSubscriptionRatePlanNumber(String subscriptionRatePlanNumber) {
this.subscriptionRatePlanNumber = subscriptionRatePlanNumber;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ChangeSubscriptionRatePlan instance itself
*/
public ChangeSubscriptionRatePlan putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ChangeSubscriptionRatePlan changeSubscriptionRatePlan = (ChangeSubscriptionRatePlan) o;
return Objects.equals(this.bookingDate, changeSubscriptionRatePlan.bookingDate) &&
Objects.equals(this.chargeOverrides, changeSubscriptionRatePlan.chargeOverrides) &&
Objects.equals(this.contractEffectiveDate, changeSubscriptionRatePlan.contractEffectiveDate) &&
Objects.equals(this.customerAcceptanceDate, changeSubscriptionRatePlan.customerAcceptanceDate) &&
Objects.equals(this.effectivePolicy, changeSubscriptionRatePlan.effectivePolicy) &&
Objects.equals(this.externalCatalogPlanId, changeSubscriptionRatePlan.externalCatalogPlanId) &&
Objects.equals(this.externalIdSourceSystem, changeSubscriptionRatePlan.externalIdSourceSystem) &&
Objects.equals(this.newExternalCatalogPlanId, changeSubscriptionRatePlan.newExternalCatalogPlanId) &&
Objects.equals(this.newExternalIdSourceSystem, changeSubscriptionRatePlan.newExternalIdSourceSystem) &&
Objects.equals(this.newProductRatePlanId, changeSubscriptionRatePlan.newProductRatePlanId) &&
Objects.equals(this.newProductRatePlanNumber, changeSubscriptionRatePlan.newProductRatePlanNumber) &&
Objects.equals(this.productRatePlanId, changeSubscriptionRatePlan.productRatePlanId) &&
Objects.equals(this.productRatePlanNumber, changeSubscriptionRatePlan.productRatePlanNumber) &&
Objects.equals(this.ratePlanId, changeSubscriptionRatePlan.ratePlanId) &&
Objects.equals(this.resetBcd, changeSubscriptionRatePlan.resetBcd) &&
Objects.equals(this.serviceActivationDate, changeSubscriptionRatePlan.serviceActivationDate) &&
Objects.equals(this.subType, changeSubscriptionRatePlan.subType) &&
Objects.equals(this.subscriptionRatePlanNumber, changeSubscriptionRatePlan.subscriptionRatePlanNumber)&&
Objects.equals(this.additionalProperties, changeSubscriptionRatePlan.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(bookingDate, chargeOverrides, contractEffectiveDate, customerAcceptanceDate, effectivePolicy, externalCatalogPlanId, externalIdSourceSystem, newExternalCatalogPlanId, newExternalIdSourceSystem, newProductRatePlanId, newProductRatePlanNumber, productRatePlanId, productRatePlanNumber, ratePlanId, resetBcd, serviceActivationDate, subType, subscriptionRatePlanNumber, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ChangeSubscriptionRatePlan {\n");
sb.append(" bookingDate: ").append(toIndentedString(bookingDate)).append("\n");
sb.append(" chargeOverrides: ").append(toIndentedString(chargeOverrides)).append("\n");
sb.append(" contractEffectiveDate: ").append(toIndentedString(contractEffectiveDate)).append("\n");
sb.append(" customerAcceptanceDate: ").append(toIndentedString(customerAcceptanceDate)).append("\n");
sb.append(" effectivePolicy: ").append(toIndentedString(effectivePolicy)).append("\n");
sb.append(" externalCatalogPlanId: ").append(toIndentedString(externalCatalogPlanId)).append("\n");
sb.append(" externalIdSourceSystem: ").append(toIndentedString(externalIdSourceSystem)).append("\n");
sb.append(" newExternalCatalogPlanId: ").append(toIndentedString(newExternalCatalogPlanId)).append("\n");
sb.append(" newExternalIdSourceSystem: ").append(toIndentedString(newExternalIdSourceSystem)).append("\n");
sb.append(" newProductRatePlanId: ").append(toIndentedString(newProductRatePlanId)).append("\n");
sb.append(" newProductRatePlanNumber: ").append(toIndentedString(newProductRatePlanNumber)).append("\n");
sb.append(" productRatePlanId: ").append(toIndentedString(productRatePlanId)).append("\n");
sb.append(" productRatePlanNumber: ").append(toIndentedString(productRatePlanNumber)).append("\n");
sb.append(" ratePlanId: ").append(toIndentedString(ratePlanId)).append("\n");
sb.append(" resetBcd: ").append(toIndentedString(resetBcd)).append("\n");
sb.append(" serviceActivationDate: ").append(toIndentedString(serviceActivationDate)).append("\n");
sb.append(" subType: ").append(toIndentedString(subType)).append("\n");
sb.append(" subscriptionRatePlanNumber: ").append(toIndentedString(subscriptionRatePlanNumber)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("bookingDate");
openapiFields.add("chargeOverrides");
openapiFields.add("contractEffectiveDate");
openapiFields.add("customerAcceptanceDate");
openapiFields.add("effectivePolicy");
openapiFields.add("externalCatalogPlanId");
openapiFields.add("externalIdSourceSystem");
openapiFields.add("newExternalCatalogPlanId");
openapiFields.add("newExternalIdSourceSystem");
openapiFields.add("newProductRatePlanId");
openapiFields.add("newProductRatePlanNumber");
openapiFields.add("productRatePlanId");
openapiFields.add("productRatePlanNumber");
openapiFields.add("ratePlanId");
openapiFields.add("resetBcd");
openapiFields.add("serviceActivationDate");
openapiFields.add("subType");
openapiFields.add("subscriptionRatePlanNumber");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ChangeSubscriptionRatePlan
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ChangeSubscriptionRatePlan.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ChangeSubscriptionRatePlan is not found in the empty JSON string", ChangeSubscriptionRatePlan.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if (jsonObj.get("chargeOverrides") != null && !jsonObj.get("chargeOverrides").isJsonNull()) {
JsonArray jsonArraychargeOverrides = jsonObj.getAsJsonArray("chargeOverrides");
if (jsonArraychargeOverrides != null) {
// ensure the json data is an array
if (!jsonObj.get("chargeOverrides").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeOverrides` to be an array in the JSON string but got `%s`", jsonObj.get("chargeOverrides").toString()));
}
// validate the optional field `chargeOverrides` (array)
for (int i = 0; i < jsonArraychargeOverrides.size(); i++) {
AddSubscriptionComponent.validateJsonElement(jsonArraychargeOverrides.get(i));
};
}
}
if ((jsonObj.get("effectivePolicy") != null && !jsonObj.get("effectivePolicy").isJsonNull()) && !jsonObj.get("effectivePolicy").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `effectivePolicy` to be a primitive type in the JSON string but got `%s`", jsonObj.get("effectivePolicy").toString()));
}
// validate the optional field `effectivePolicy`
if (jsonObj.get("effectivePolicy") != null && !jsonObj.get("effectivePolicy").isJsonNull()) {
EffectivePolicyEnum.validateJsonElement(jsonObj.get("effectivePolicy"));
}
if ((jsonObj.get("externalCatalogPlanId") != null && !jsonObj.get("externalCatalogPlanId").isJsonNull()) && !jsonObj.get("externalCatalogPlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `externalCatalogPlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("externalCatalogPlanId").toString()));
}
if ((jsonObj.get("externalIdSourceSystem") != null && !jsonObj.get("externalIdSourceSystem").isJsonNull()) && !jsonObj.get("externalIdSourceSystem").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `externalIdSourceSystem` to be a primitive type in the JSON string but got `%s`", jsonObj.get("externalIdSourceSystem").toString()));
}
if ((jsonObj.get("newExternalCatalogPlanId") != null && !jsonObj.get("newExternalCatalogPlanId").isJsonNull()) && !jsonObj.get("newExternalCatalogPlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `newExternalCatalogPlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("newExternalCatalogPlanId").toString()));
}
if ((jsonObj.get("newExternalIdSourceSystem") != null && !jsonObj.get("newExternalIdSourceSystem").isJsonNull()) && !jsonObj.get("newExternalIdSourceSystem").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `newExternalIdSourceSystem` to be a primitive type in the JSON string but got `%s`", jsonObj.get("newExternalIdSourceSystem").toString()));
}
if ((jsonObj.get("newProductRatePlanId") != null && !jsonObj.get("newProductRatePlanId").isJsonNull()) && !jsonObj.get("newProductRatePlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `newProductRatePlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("newProductRatePlanId").toString()));
}
if ((jsonObj.get("newProductRatePlanNumber") != null && !jsonObj.get("newProductRatePlanNumber").isJsonNull()) && !jsonObj.get("newProductRatePlanNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `newProductRatePlanNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("newProductRatePlanNumber").toString()));
}
if ((jsonObj.get("productRatePlanId") != null && !jsonObj.get("productRatePlanId").isJsonNull()) && !jsonObj.get("productRatePlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanId").toString()));
}
if ((jsonObj.get("productRatePlanNumber") != null && !jsonObj.get("productRatePlanNumber").isJsonNull()) && !jsonObj.get("productRatePlanNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanNumber").toString()));
}
if ((jsonObj.get("ratePlanId") != null && !jsonObj.get("ratePlanId").isJsonNull()) && !jsonObj.get("ratePlanId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ratePlanId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ratePlanId").toString()));
}
if ((jsonObj.get("subType") != null && !jsonObj.get("subType").isJsonNull()) && !jsonObj.get("subType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subType").toString()));
}
// validate the optional field `subType`
if (jsonObj.get("subType") != null && !jsonObj.get("subType").isJsonNull()) {
SubTypeEnum.validateJsonElement(jsonObj.get("subType"));
}
if ((jsonObj.get("subscriptionRatePlanNumber") != null && !jsonObj.get("subscriptionRatePlanNumber").isJsonNull()) && !jsonObj.get("subscriptionRatePlanNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `subscriptionRatePlanNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("subscriptionRatePlanNumber").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ChangeSubscriptionRatePlan.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ChangeSubscriptionRatePlan' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ChangeSubscriptionRatePlan.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ChangeSubscriptionRatePlan value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ChangeSubscriptionRatePlan read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ChangeSubscriptionRatePlan instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ChangeSubscriptionRatePlan given an JSON string
*
* @param jsonString JSON string
* @return An instance of ChangeSubscriptionRatePlan
* @throws IOException if the JSON string is invalid with respect to ChangeSubscriptionRatePlan
*/
public static ChangeSubscriptionRatePlan fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ChangeSubscriptionRatePlan.class);
}
/**
* Convert an instance of ChangeSubscriptionRatePlan to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy