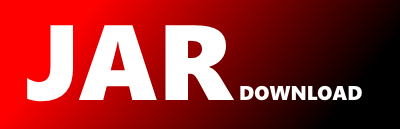
com.zuora.model.ChargeOverride Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.ChargeFunction;
import com.zuora.model.ChargeModel;
import com.zuora.model.ChargeOverrideBilling;
import com.zuora.model.ChargeOverridePricing;
import com.zuora.model.ChargeType;
import com.zuora.model.CommitmentLevel;
import com.zuora.model.CommitmentType;
import com.zuora.model.EndConditions;
import com.zuora.model.PrepaidDrawdownCreditOption;
import com.zuora.model.PrepaidOperationType;
import com.zuora.model.ProrationOption;
import com.zuora.model.RatingPropertiesOverride;
import com.zuora.model.RevRecTriggerCondition;
import com.zuora.model.RolloverApply;
import com.zuora.model.TaxMode;
import com.zuora.model.TriggerParams;
import com.zuora.model.ValidityPeriodType;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Charge associated with a rate plan.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class ChargeOverride {
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_ACCOUNT_RECEIVABLE_ACCOUNTING_CODE = "accountReceivableAccountingCode";
@SerializedName(SERIALIZED_NAME_ACCOUNT_RECEIVABLE_ACCOUNTING_CODE)
private String accountReceivableAccountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE = "adjustmentLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_LIABILITY_ACCOUNTING_CODE)
private String adjustmentLiabilityAccountingCode;
public static final String SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE = "adjustmentRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_ADJUSTMENT_REVENUE_ACCOUNTING_CODE)
private String adjustmentRevenueAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE = "contractAssetAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_ASSET_ACCOUNTING_CODE)
private String contractAssetAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE = "contractLiabilityAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_LIABILITY_ACCOUNTING_CODE)
private String contractLiabilityAccountingCode;
public static final String SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "contractRecognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_CONTRACT_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String contractRecognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE = "deferredRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_DEFERRED_REVENUE_ACCOUNTING_CODE)
private String deferredRevenueAccountingCode;
public static final String SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE = "recognizedRevenueAccountingCode";
@SerializedName(SERIALIZED_NAME_RECOGNIZED_REVENUE_ACCOUNTING_CODE)
private String recognizedRevenueAccountingCode;
public static final String SERIALIZED_NAME_UN_BILLED_RECEIVABLES_ACCOUNTING_CODE = "unBilledReceivablesAccountingCode";
@SerializedName(SERIALIZED_NAME_UN_BILLED_RECEIVABLES_ACCOUNTING_CODE)
private String unBilledReceivablesAccountingCode;
public static final String SERIALIZED_NAME_PRODUCT_CATEGORY = "productCategory";
@SerializedName(SERIALIZED_NAME_PRODUCT_CATEGORY)
private String productCategory;
public static final String SERIALIZED_NAME_PRODUCT_CLASS = "productClass";
@SerializedName(SERIALIZED_NAME_PRODUCT_CLASS)
private String productClass;
public static final String SERIALIZED_NAME_PRODUCT_FAMILY = "productFamily";
@SerializedName(SERIALIZED_NAME_PRODUCT_FAMILY)
private String productFamily;
public static final String SERIALIZED_NAME_PRODUCT_LINE = "productLine";
@SerializedName(SERIALIZED_NAME_PRODUCT_LINE)
private String productLine;
public static final String SERIALIZED_NAME_CHARGE_MODEL = "chargeModel";
@SerializedName(SERIALIZED_NAME_CHARGE_MODEL)
private ChargeModel chargeModel;
public static final String SERIALIZED_NAME_CHARGE_TYPE = "chargeType";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE)
private ChargeType chargeType;
public static final String SERIALIZED_NAME_TAX_CODE = "taxCode";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_MODE = "taxMode";
@SerializedName(SERIALIZED_NAME_TAX_MODE)
private TaxMode taxMode;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING = "revenueRecognitionTiming";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_TIMING)
private String revenueRecognitionTiming;
public static final String SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD = "revenueAmortizationMethod";
@SerializedName(SERIALIZED_NAME_REVENUE_AMORTIZATION_METHOD)
private String revenueAmortizationMethod;
public static final String SERIALIZED_NAME_IS_CHARGE_LEVEL_MIN_COMMIT = "isChargeLevelMinCommit";
@SerializedName(SERIALIZED_NAME_IS_CHARGE_LEVEL_MIN_COMMIT)
private Boolean isChargeLevelMinCommit;
public static final String SERIALIZED_NAME_COMMITMENT_TYPE = "commitmentType";
@SerializedName(SERIALIZED_NAME_COMMITMENT_TYPE)
private CommitmentType commitmentType;
public static final String SERIALIZED_NAME_PREPAID_OPERATION_TYPE = "prepaidOperationType";
@SerializedName(SERIALIZED_NAME_PREPAID_OPERATION_TYPE)
private PrepaidOperationType prepaidOperationType;
public static final String SERIALIZED_NAME_IS_COMMITTED = "isCommitted";
@SerializedName(SERIALIZED_NAME_IS_COMMITTED)
private Boolean isCommitted;
public static final String SERIALIZED_NAME_CHARGE_FUNCTION = "chargeFunction";
@SerializedName(SERIALIZED_NAME_CHARGE_FUNCTION)
private ChargeFunction chargeFunction;
public static final String SERIALIZED_NAME_PREPAID_UOM = "prepaidUom";
@SerializedName(SERIALIZED_NAME_PREPAID_UOM)
private String prepaidUom;
public static final String SERIALIZED_NAME_DRAWDOWN_UOM = "drawdownUom";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_UOM)
private String drawdownUom;
public static final String SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY = "prepaidTotalQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_TOTAL_QUANTITY)
private BigDecimal prepaidTotalQuantity;
public static final String SERIALIZED_NAME_IS_PREPAID = "isPrepaid";
@SerializedName(SERIALIZED_NAME_IS_PREPAID)
private Boolean isPrepaid;
public static final String SERIALIZED_NAME_CREDIT_OPTION = "creditOption";
@SerializedName(SERIALIZED_NAME_CREDIT_OPTION)
private PrepaidDrawdownCreditOption creditOption;
public static final String SERIALIZED_NAME_BILLING = "billing";
@SerializedName(SERIALIZED_NAME_BILLING)
private ChargeOverrideBilling billing;
public static final String SERIALIZED_NAME_CHARGE_NUMBER = "chargeNumber";
@SerializedName(SERIALIZED_NAME_CHARGE_NUMBER)
private String chargeNumber;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "customFields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
private Map customFields;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DRAWDOWN_RATE = "drawdownRate";
@SerializedName(SERIALIZED_NAME_DRAWDOWN_RATE)
private BigDecimal drawdownRate;
public static final String SERIALIZED_NAME_END_DATE = "endDate";
@SerializedName(SERIALIZED_NAME_END_DATE)
private EndConditions endDate;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING = "excludeItemBillingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BILLING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBillingFromRevenueAccounting;
public static final String SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING = "excludeItemBookingFromRevenueAccounting";
@SerializedName(SERIALIZED_NAME_EXCLUDE_ITEM_BOOKING_FROM_REVENUE_ACCOUNTING)
private Boolean excludeItemBookingFromRevenueAccounting;
public static final String SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE = "isAllocationEligible";
@SerializedName(SERIALIZED_NAME_IS_ALLOCATION_ELIGIBLE)
private Boolean isAllocationEligible;
public static final String SERIALIZED_NAME_IS_ROLLOVER = "isRollover";
@SerializedName(SERIALIZED_NAME_IS_ROLLOVER)
private Boolean isRollover;
public static final String SERIALIZED_NAME_IS_UNBILLED = "isUnbilled";
@SerializedName(SERIALIZED_NAME_IS_UNBILLED)
private Boolean isUnbilled;
public static final String SERIALIZED_NAME_PREPAID_QUANTITY = "prepaidQuantity";
@SerializedName(SERIALIZED_NAME_PREPAID_QUANTITY)
private BigDecimal prepaidQuantity;
public static final String SERIALIZED_NAME_PRICING = "pricing";
@SerializedName(SERIALIZED_NAME_PRICING)
private ChargeOverridePricing pricing;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_NUMBER = "productRatePlanChargeNumber";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_NUMBER)
private String productRatePlanChargeNumber;
public static final String SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID = "productRatePlanChargeId";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATE_PLAN_CHARGE_ID)
private String productRatePlanChargeId;
public static final String SERIALIZED_NAME_PRORATION_OPTION = "prorationOption";
@SerializedName(SERIALIZED_NAME_PRORATION_OPTION)
private ProrationOption prorationOption;
public static final String SERIALIZED_NAME_RATING_PROPERTIES_OVERRIDE = "ratingPropertiesOverride";
@SerializedName(SERIALIZED_NAME_RATING_PROPERTIES_OVERRIDE)
private RatingPropertiesOverride ratingPropertiesOverride;
public static final String SERIALIZED_NAME_REV_REC_CODE = "revRecCode";
@SerializedName(SERIALIZED_NAME_REV_REC_CODE)
private String revRecCode;
public static final String SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION = "revRecTriggerCondition";
@SerializedName(SERIALIZED_NAME_REV_REC_TRIGGER_CONDITION)
private RevRecTriggerCondition revRecTriggerCondition;
public static final String SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME = "revenueRecognitionRuleName";
@SerializedName(SERIALIZED_NAME_REVENUE_RECOGNITION_RULE_NAME)
private String revenueRecognitionRuleName;
public static final String SERIALIZED_NAME_ROLLOVER_APPLY = "rolloverApply";
@SerializedName(SERIALIZED_NAME_ROLLOVER_APPLY)
private RolloverApply rolloverApply;
public static final String SERIALIZED_NAME_ROLLOVER_PERIODS = "rolloverPeriods";
@SerializedName(SERIALIZED_NAME_ROLLOVER_PERIODS)
private BigDecimal rolloverPeriods;
public static final String SERIALIZED_NAME_START_DATE = "startDate";
@SerializedName(SERIALIZED_NAME_START_DATE)
private TriggerParams startDate;
public static final String SERIALIZED_NAME_UNIQUE_TOKEN = "uniqueToken";
@SerializedName(SERIALIZED_NAME_UNIQUE_TOKEN)
private String uniqueToken;
public static final String SERIALIZED_NAME_UPSELL_ORIGIN_CHARGE_NUMBER = "upsellOriginChargeNumber";
@SerializedName(SERIALIZED_NAME_UPSELL_ORIGIN_CHARGE_NUMBER)
private String upsellOriginChargeNumber;
public static final String SERIALIZED_NAME_VALIDITY_PERIOD_TYPE = "validityPeriodType";
@SerializedName(SERIALIZED_NAME_VALIDITY_PERIOD_TYPE)
private ValidityPeriodType validityPeriodType;
public static final String SERIALIZED_NAME_COMMITMENT_LEVEL = "commitmentLevel";
@SerializedName(SERIALIZED_NAME_COMMITMENT_LEVEL)
private CommitmentLevel commitmentLevel;
public ChargeOverride() {
}
public ChargeOverride name(String name) {
this.name = name;
return this;
}
/**
* The name of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature is enabled.
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public ChargeOverride accountReceivableAccountingCode(String accountReceivableAccountingCode) {
this.accountReceivableAccountingCode = accountReceivableAccountingCode;
return this;
}
/**
* The accountReceivableAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a>, <a href=\"https://knowledgecenter.zuora.com/Zuora_Payments/Zuora_Finance\" target=\"_blank\">Zuora Finance</a>, and <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Adjust_invoice_amounts/Invoice_Settlement/Get_started_with_Invoice_Settlement/AA_Overview_of_Invoice_Settlement\" target=\"_blank\">Invoice Settlement</a> features are enabled.
* @return accountReceivableAccountingCode
*/
@javax.annotation.Nullable
public String getAccountReceivableAccountingCode() {
return accountReceivableAccountingCode;
}
public void setAccountReceivableAccountingCode(String accountReceivableAccountingCode) {
this.accountReceivableAccountingCode = accountReceivableAccountingCode;
}
public ChargeOverride adjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
return this;
}
/**
* The adjustmentLiabilityAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature and the <a href=\"https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration\" target=\"_blank\">Billing - Revenue Integration</a> or <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Enable_Order_to_Revenue/Order_to_Revenue_introduction/AA_Overview_of_Order_to_Revenue\" target=\"_blank\">Order to Revenue</a> feature are enabled.
* @return adjustmentLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentLiabilityAccountingCode() {
return adjustmentLiabilityAccountingCode;
}
public void setAdjustmentLiabilityAccountingCode(String adjustmentLiabilityAccountingCode) {
this.adjustmentLiabilityAccountingCode = adjustmentLiabilityAccountingCode;
}
public ChargeOverride adjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
return this;
}
/**
* The adjustmentRevenueAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature and the <a href=\"https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration\" target=\"_blank\">Billing - Revenue Integration</a> or <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Enable_Order_to_Revenue/Order_to_Revenue_introduction/AA_Overview_of_Order_to_Revenue\" target=\"_blank\">Order to Revenue</a> feature are enabled.
* @return adjustmentRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getAdjustmentRevenueAccountingCode() {
return adjustmentRevenueAccountingCode;
}
public void setAdjustmentRevenueAccountingCode(String adjustmentRevenueAccountingCode) {
this.adjustmentRevenueAccountingCode = adjustmentRevenueAccountingCode;
}
public ChargeOverride contractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
return this;
}
/**
* The contractAssetAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature and the <a href=\"https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration\" target=\"_blank\">Billing - Revenue Integration</a> or <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Enable_Order_to_Revenue/Order_to_Revenue_introduction/AA_Overview_of_Order_to_Revenue\" target=\"_blank\">Order to Revenue</a> feature are enabled.
* @return contractAssetAccountingCode
*/
@javax.annotation.Nullable
public String getContractAssetAccountingCode() {
return contractAssetAccountingCode;
}
public void setContractAssetAccountingCode(String contractAssetAccountingCode) {
this.contractAssetAccountingCode = contractAssetAccountingCode;
}
public ChargeOverride contractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
return this;
}
/**
* The contractLiabilityAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature and the <a href=\"https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration\" target=\"_blank\">Billing - Revenue Integration</a> or <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Enable_Order_to_Revenue/Order_to_Revenue_introduction/AA_Overview_of_Order_to_Revenue\" target=\"_blank\">Order to Revenue</a> feature are enabled.
* @return contractLiabilityAccountingCode
*/
@javax.annotation.Nullable
public String getContractLiabilityAccountingCode() {
return contractLiabilityAccountingCode;
}
public void setContractLiabilityAccountingCode(String contractLiabilityAccountingCode) {
this.contractLiabilityAccountingCode = contractLiabilityAccountingCode;
}
public ChargeOverride contractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
return this;
}
/**
* The contractRecognizedRevenueAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature and the <a href=\"https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration\" target=\"_blank\">Billing - Revenue Integration</a> or <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Enable_Order_to_Revenue/Order_to_Revenue_introduction/AA_Overview_of_Order_to_Revenue\" target=\"_blank\">Order to Revenue</a> feature are enabled.
* @return contractRecognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getContractRecognizedRevenueAccountingCode() {
return contractRecognizedRevenueAccountingCode;
}
public void setContractRecognizedRevenueAccountingCode(String contractRecognizedRevenueAccountingCode) {
this.contractRecognizedRevenueAccountingCode = contractRecognizedRevenueAccountingCode;
}
public ChargeOverride deferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
return this;
}
/**
* The deferredRevenueAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> and <a href=\"https://knowledgecenter.zuora.com/Zuora_Payments/Zuora_Finance\" target=\"_blank\">Zuora Finance</a> features are enabled.
* @return deferredRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getDeferredRevenueAccountingCode() {
return deferredRevenueAccountingCode;
}
public void setDeferredRevenueAccountingCode(String deferredRevenueAccountingCode) {
this.deferredRevenueAccountingCode = deferredRevenueAccountingCode;
}
public ChargeOverride recognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
return this;
}
/**
* The recognizedRevenueAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> and <a href=\"https://knowledgecenter.zuora.com/Zuora_Payments/Zuora_Finance\" target=\"_blank\">Zuora Finance</a> features are enabled.
* @return recognizedRevenueAccountingCode
*/
@javax.annotation.Nullable
public String getRecognizedRevenueAccountingCode() {
return recognizedRevenueAccountingCode;
}
public void setRecognizedRevenueAccountingCode(String recognizedRevenueAccountingCode) {
this.recognizedRevenueAccountingCode = recognizedRevenueAccountingCode;
}
public ChargeOverride unBilledReceivablesAccountingCode(String unBilledReceivablesAccountingCode) {
this.unBilledReceivablesAccountingCode = unBilledReceivablesAccountingCode;
return this;
}
/**
* The unBilledReceivablesAccountingCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature and the <a href=\"https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration\" target=\"_blank\">Billing - Revenue Integration</a> or <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Enable_Order_to_Revenue/Order_to_Revenue_introduction/AA_Overview_of_Order_to_Revenue\" target=\"_blank\">Order to Revenue</a> feature are enabled.
* @return unBilledReceivablesAccountingCode
*/
@javax.annotation.Nullable
public String getUnBilledReceivablesAccountingCode() {
return unBilledReceivablesAccountingCode;
}
public void setUnBilledReceivablesAccountingCode(String unBilledReceivablesAccountingCode) {
this.unBilledReceivablesAccountingCode = unBilledReceivablesAccountingCode;
}
public ChargeOverride productCategory(String productCategory) {
this.productCategory = productCategory;
return this;
}
/**
* The productCategory of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature is enabled.
* @return productCategory
*/
@javax.annotation.Nullable
public String getProductCategory() {
return productCategory;
}
public void setProductCategory(String productCategory) {
this.productCategory = productCategory;
}
public ChargeOverride productClass(String productClass) {
this.productClass = productClass;
return this;
}
/**
* The productClass of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature is enabled.
* @return productClass
*/
@javax.annotation.Nullable
public String getProductClass() {
return productClass;
}
public void setProductClass(String productClass) {
this.productClass = productClass;
}
public ChargeOverride productFamily(String productFamily) {
this.productFamily = productFamily;
return this;
}
/**
* The productFamily of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature is enabled.
* @return productFamily
*/
@javax.annotation.Nullable
public String getProductFamily() {
return productFamily;
}
public void setProductFamily(String productFamily) {
this.productFamily = productFamily;
}
public ChargeOverride productLine(String productLine) {
this.productLine = productLine;
return this;
}
/**
* The productLine of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature is enabled.
* @return productLine
*/
@javax.annotation.Nullable
public String getProductLine() {
return productLine;
}
public void setProductLine(String productLine) {
this.productLine = productLine;
}
public ChargeOverride chargeModel(ChargeModel chargeModel) {
this.chargeModel = chargeModel;
return this;
}
/**
* Get chargeModel
* @return chargeModel
*/
@javax.annotation.Nullable
public ChargeModel getChargeModel() {
return chargeModel;
}
public void setChargeModel(ChargeModel chargeModel) {
this.chargeModel = chargeModel;
}
public ChargeOverride chargeType(ChargeType chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Get chargeType
* @return chargeType
*/
@javax.annotation.Nullable
public ChargeType getChargeType() {
return chargeType;
}
public void setChargeType(ChargeType chargeType) {
this.chargeType = chargeType;
}
public ChargeOverride taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* The taxCode of a standalone charge. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature is enabled.
* @return taxCode
*/
@javax.annotation.Nullable
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public ChargeOverride taxMode(TaxMode taxMode) {
this.taxMode = taxMode;
return this;
}
/**
* Get taxMode
* @return taxMode
*/
@javax.annotation.Nullable
public TaxMode getTaxMode() {
return taxMode;
}
public void setTaxMode(TaxMode taxMode) {
this.taxMode = taxMode;
}
public ChargeOverride revenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
return this;
}
/**
* This field is used to dictate the type of revenue recognition timing.
* @return revenueRecognitionTiming
*/
@javax.annotation.Nullable
public String getRevenueRecognitionTiming() {
return revenueRecognitionTiming;
}
public void setRevenueRecognitionTiming(String revenueRecognitionTiming) {
this.revenueRecognitionTiming = revenueRecognitionTiming;
}
public ChargeOverride revenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
return this;
}
/**
* This field is used to dictate the type of revenue amortization method.
* @return revenueAmortizationMethod
*/
@javax.annotation.Nullable
public String getRevenueAmortizationMethod() {
return revenueAmortizationMethod;
}
public void setRevenueAmortizationMethod(String revenueAmortizationMethod) {
this.revenueAmortizationMethod = revenueAmortizationMethod;
}
public ChargeOverride isChargeLevelMinCommit(Boolean isChargeLevelMinCommit) {
this.isChargeLevelMinCommit = isChargeLevelMinCommit;
return this;
}
/**
* The flag to indicate whether the charge is charge level min commit. **Note:** This field is available when the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_subscription_transactions/Orders/Standalone_Orders/AA_Overview_of_Standalone_Orders\" target=\"_blank\">Standalone Orders</a> feature is enabled.
* @return isChargeLevelMinCommit
*/
@javax.annotation.Nullable
public Boolean getIsChargeLevelMinCommit() {
return isChargeLevelMinCommit;
}
public void setIsChargeLevelMinCommit(Boolean isChargeLevelMinCommit) {
this.isChargeLevelMinCommit = isChargeLevelMinCommit;
}
public ChargeOverride commitmentType(CommitmentType commitmentType) {
this.commitmentType = commitmentType;
return this;
}
/**
* Get commitmentType
* @return commitmentType
*/
@javax.annotation.Nullable
public CommitmentType getCommitmentType() {
return commitmentType;
}
public void setCommitmentType(CommitmentType commitmentType) {
this.commitmentType = commitmentType;
}
public ChargeOverride prepaidOperationType(PrepaidOperationType prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
return this;
}
/**
* Get prepaidOperationType
* @return prepaidOperationType
*/
@javax.annotation.Nullable
public PrepaidOperationType getPrepaidOperationType() {
return prepaidOperationType;
}
public void setPrepaidOperationType(PrepaidOperationType prepaidOperationType) {
this.prepaidOperationType = prepaidOperationType;
}
public ChargeOverride isCommitted(Boolean isCommitted) {
this.isCommitted = isCommitted;
return this;
}
/**
* The flag to indicate whether the charge is committed.
* @return isCommitted
*/
@javax.annotation.Nullable
public Boolean getIsCommitted() {
return isCommitted;
}
public void setIsCommitted(Boolean isCommitted) {
this.isCommitted = isCommitted;
}
public ChargeOverride chargeFunction(ChargeFunction chargeFunction) {
this.chargeFunction = chargeFunction;
return this;
}
/**
* Get chargeFunction
* @return chargeFunction
*/
@javax.annotation.Nullable
public ChargeFunction getChargeFunction() {
return chargeFunction;
}
public void setChargeFunction(ChargeFunction chargeFunction) {
this.chargeFunction = chargeFunction;
}
public ChargeOverride prepaidUom(String prepaidUom) {
this.prepaidUom = prepaidUom;
return this;
}
/**
* The prepaid unit of measure of the charge.
* @return prepaidUom
*/
@javax.annotation.Nullable
public String getPrepaidUom() {
return prepaidUom;
}
public void setPrepaidUom(String prepaidUom) {
this.prepaidUom = prepaidUom;
}
public ChargeOverride drawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
return this;
}
/**
* The drawdown unit of measure of the charge.
* @return drawdownUom
*/
@javax.annotation.Nullable
public String getDrawdownUom() {
return drawdownUom;
}
public void setDrawdownUom(String drawdownUom) {
this.drawdownUom = drawdownUom;
}
public ChargeOverride prepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
return this;
}
/**
* The prepaid total quantity of the charge.
* @return prepaidTotalQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidTotalQuantity() {
return prepaidTotalQuantity;
}
public void setPrepaidTotalQuantity(BigDecimal prepaidTotalQuantity) {
this.prepaidTotalQuantity = prepaidTotalQuantity;
}
public ChargeOverride isPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
return this;
}
/**
* The flag to indicate whether the charge is prepaid.
* @return isPrepaid
*/
@javax.annotation.Nullable
public Boolean getIsPrepaid() {
return isPrepaid;
}
public void setIsPrepaid(Boolean isPrepaid) {
this.isPrepaid = isPrepaid;
}
public ChargeOverride creditOption(PrepaidDrawdownCreditOption creditOption) {
this.creditOption = creditOption;
return this;
}
/**
* Get creditOption
* @return creditOption
*/
@javax.annotation.Nullable
public PrepaidDrawdownCreditOption getCreditOption() {
return creditOption;
}
public void setCreditOption(PrepaidDrawdownCreditOption creditOption) {
this.creditOption = creditOption;
}
public ChargeOverride billing(ChargeOverrideBilling billing) {
this.billing = billing;
return this;
}
/**
* Get billing
* @return billing
*/
@javax.annotation.Nullable
public ChargeOverrideBilling getBilling() {
return billing;
}
public void setBilling(ChargeOverrideBilling billing) {
this.billing = billing;
}
public ChargeOverride chargeNumber(String chargeNumber) {
this.chargeNumber = chargeNumber;
return this;
}
/**
* Charge number of the charge. For example, C-00000307. If you do not set this field, Zuora will generate the charge number.
* @return chargeNumber
*/
@javax.annotation.Nullable
public String getChargeNumber() {
return chargeNumber;
}
public void setChargeNumber(String chargeNumber) {
this.chargeNumber = chargeNumber;
}
public ChargeOverride customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public ChargeOverride putCustomFieldsItem(String key, Object customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap<>();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Container for custom fields of a Rate Plan Charge object.
* @return customFields
*/
@javax.annotation.Nullable
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public ChargeOverride description(String description) {
this.description = description;
return this;
}
/**
* Description of the charge.
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public ChargeOverride drawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. The [conversion rate](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_drawdown_charge#UOM_Conversion) between Usage UOM and Drawdown UOM for a [drawdown charge](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_drawdown_charge). Must be a positive number (>0).
* @return drawdownRate
*/
@javax.annotation.Nullable
public BigDecimal getDrawdownRate() {
return drawdownRate;
}
public void setDrawdownRate(BigDecimal drawdownRate) {
this.drawdownRate = drawdownRate;
}
public ChargeOverride endDate(EndConditions endDate) {
this.endDate = endDate;
return this;
}
/**
* Get endDate
* @return endDate
*/
@javax.annotation.Nullable
public EndConditions getEndDate() {
return endDate;
}
public void setEndDate(EndConditions endDate) {
this.endDate = endDate;
}
public ChargeOverride excludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude rate plan charge related invoice items, invoice item adjustments, credit memo items, and debit memo items from revenue accounting. **Note**: This field is only available if you have the [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration) feature enabled.
* @return excludeItemBillingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBillingFromRevenueAccounting() {
return excludeItemBillingFromRevenueAccounting;
}
public void setExcludeItemBillingFromRevenueAccounting(Boolean excludeItemBillingFromRevenueAccounting) {
this.excludeItemBillingFromRevenueAccounting = excludeItemBillingFromRevenueAccounting;
}
public ChargeOverride excludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
return this;
}
/**
* The flag to exclude rate plan charges from revenue accounting. **Note**: This field is only available if you have the [Zuora Billing - Revenue Integration](https://knowledgecenter.zuora.com/Zuora_Revenue/Zuora_Billing_-_Revenue_Integration) feature enabled.
* @return excludeItemBookingFromRevenueAccounting
*/
@javax.annotation.Nullable
public Boolean getExcludeItemBookingFromRevenueAccounting() {
return excludeItemBookingFromRevenueAccounting;
}
public void setExcludeItemBookingFromRevenueAccounting(Boolean excludeItemBookingFromRevenueAccounting) {
this.excludeItemBookingFromRevenueAccounting = excludeItemBookingFromRevenueAccounting;
}
public ChargeOverride isAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
return this;
}
/**
* This field is used to identify if the charge segment is allocation eligible in revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isAllocationEligible
*/
@javax.annotation.Nullable
public Boolean getIsAllocationEligible() {
return isAllocationEligible;
}
public void setIsAllocationEligible(Boolean isAllocationEligible) {
this.isAllocationEligible = isAllocationEligible;
}
public ChargeOverride isRollover(Boolean isRollover) {
this.isRollover = isRollover;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. To use this field, you must set the `X-Zuora-WSDL-Version` request header to 114 or higher. Otherwise, an error occurs. The value is either \"True\" or \"False\". It determines whether the rollover fields are needed.
* @return isRollover
*/
@javax.annotation.Nullable
public Boolean getIsRollover() {
return isRollover;
}
public void setIsRollover(Boolean isRollover) {
this.isRollover = isRollover;
}
public ChargeOverride isUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
return this;
}
/**
* This field is used to dictate how to perform the accounting during revenue recognition. **Note**: This feature is in the **Early Adopter** phase. If you want to use the feature, submit a request at <a href=\"https://support.zuora.com/\" target=\"_blank\">Zuora Global Support</a>, and we will evaluate whether the feature is suitable for your use cases.
* @return isUnbilled
*/
@javax.annotation.Nullable
public Boolean getIsUnbilled() {
return isUnbilled;
}
public void setIsUnbilled(Boolean isUnbilled) {
this.isUnbilled = isUnbilled;
}
public ChargeOverride prepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. The number of units included in a [prepayment charge](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown/Create_prepayment_charge). Must be a positive number (>0).
* @return prepaidQuantity
*/
@javax.annotation.Nullable
public BigDecimal getPrepaidQuantity() {
return prepaidQuantity;
}
public void setPrepaidQuantity(BigDecimal prepaidQuantity) {
this.prepaidQuantity = prepaidQuantity;
}
public ChargeOverride pricing(ChargeOverridePricing pricing) {
this.pricing = pricing;
return this;
}
/**
* Get pricing
* @return pricing
*/
@javax.annotation.Nullable
public ChargeOverridePricing getPricing() {
return pricing;
}
public void setPricing(ChargeOverridePricing pricing) {
this.pricing = pricing;
}
public ChargeOverride productRatePlanChargeNumber(String productRatePlanChargeNumber) {
this.productRatePlanChargeNumber = productRatePlanChargeNumber;
return this;
}
/**
* Number of a product rate-plan charge for this subscription.
* @return productRatePlanChargeNumber
*/
@javax.annotation.Nullable
public String getProductRatePlanChargeNumber() {
return productRatePlanChargeNumber;
}
public void setProductRatePlanChargeNumber(String productRatePlanChargeNumber) {
this.productRatePlanChargeNumber = productRatePlanChargeNumber;
}
public ChargeOverride productRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
return this;
}
/**
* Internal identifier of the product rate plan charge that the charge is based on.
* @return productRatePlanChargeId
*/
@javax.annotation.Nonnull
public String getProductRatePlanChargeId() {
return productRatePlanChargeId;
}
public void setProductRatePlanChargeId(String productRatePlanChargeId) {
this.productRatePlanChargeId = productRatePlanChargeId;
}
public ChargeOverride prorationOption(ProrationOption prorationOption) {
this.prorationOption = prorationOption;
return this;
}
/**
* Get prorationOption
* @return prorationOption
*/
@javax.annotation.Nullable
public ProrationOption getProrationOption() {
return prorationOption;
}
public void setProrationOption(ProrationOption prorationOption) {
this.prorationOption = prorationOption;
}
public ChargeOverride ratingPropertiesOverride(RatingPropertiesOverride ratingPropertiesOverride) {
this.ratingPropertiesOverride = ratingPropertiesOverride;
return this;
}
/**
* Get ratingPropertiesOverride
* @return ratingPropertiesOverride
*/
@javax.annotation.Nullable
public RatingPropertiesOverride getRatingPropertiesOverride() {
return ratingPropertiesOverride;
}
public void setRatingPropertiesOverride(RatingPropertiesOverride ratingPropertiesOverride) {
this.ratingPropertiesOverride = ratingPropertiesOverride;
}
public ChargeOverride revRecCode(String revRecCode) {
this.revRecCode = revRecCode;
return this;
}
/**
* Revenue Recognition Code
* @return revRecCode
*/
@javax.annotation.Nullable
public String getRevRecCode() {
return revRecCode;
}
public void setRevRecCode(String revRecCode) {
this.revRecCode = revRecCode;
}
public ChargeOverride revRecTriggerCondition(RevRecTriggerCondition revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
return this;
}
/**
* Get revRecTriggerCondition
* @return revRecTriggerCondition
*/
@javax.annotation.Nullable
public RevRecTriggerCondition getRevRecTriggerCondition() {
return revRecTriggerCondition;
}
public void setRevRecTriggerCondition(RevRecTriggerCondition revRecTriggerCondition) {
this.revRecTriggerCondition = revRecTriggerCondition;
}
public ChargeOverride revenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
return this;
}
/**
* Specifies the revenue recognition rule, such as `Recognize upon invoicing` or `Recognize daily over time`.
* @return revenueRecognitionRuleName
*/
@javax.annotation.Nullable
public String getRevenueRecognitionRuleName() {
return revenueRecognitionRuleName;
}
public void setRevenueRecognitionRuleName(String revenueRecognitionRuleName) {
this.revenueRecognitionRuleName = revenueRecognitionRuleName;
}
public ChargeOverride rolloverApply(RolloverApply rolloverApply) {
this.rolloverApply = rolloverApply;
return this;
}
/**
* Get rolloverApply
* @return rolloverApply
*/
@javax.annotation.Nullable
public RolloverApply getRolloverApply() {
return rolloverApply;
}
public void setRolloverApply(RolloverApply rolloverApply) {
this.rolloverApply = rolloverApply;
}
public ChargeOverride rolloverPeriods(BigDecimal rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
return this;
}
/**
* **Note**: This field is only available if you have the [Prepaid with Drawdown](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/J_Billing_Operations/Prepaid_with_Drawdown) feature enabled. To use this field, you must set the `X-Zuora-WSDL-Version` request header to 114 or higher. Otherwise, an error occurs. This field defines the number of rollover periods, it is restricted to 3.
* @return rolloverPeriods
*/
@javax.annotation.Nullable
public BigDecimal getRolloverPeriods() {
return rolloverPeriods;
}
public void setRolloverPeriods(BigDecimal rolloverPeriods) {
this.rolloverPeriods = rolloverPeriods;
}
public ChargeOverride startDate(TriggerParams startDate) {
this.startDate = startDate;
return this;
}
/**
* Get startDate
* @return startDate
*/
@javax.annotation.Nullable
public TriggerParams getStartDate() {
return startDate;
}
public void setStartDate(TriggerParams startDate) {
this.startDate = startDate;
}
public ChargeOverride uniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
return this;
}
/**
* Unique identifier for the charge. This identifier enables you to refer to the charge before the charge has an internal identifier in Zuora. For instance, suppose that you want to use a single order to add a product to a subscription and later update the same product. When you add the product, you can set a unique identifier for the charge. Then when you update the product, you can use the same unique identifier to specify which charge to modify.
* @return uniqueToken
*/
@javax.annotation.Nullable
public String getUniqueToken() {
return uniqueToken;
}
public void setUniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
}
public ChargeOverride upsellOriginChargeNumber(String upsellOriginChargeNumber) {
this.upsellOriginChargeNumber = upsellOriginChargeNumber;
return this;
}
/**
* **Note**: The Quantity Upsell feature is in Limited Availability. If you wish to have access to the feature, submit a request at [Zuora Global Support](https://support.zuora.com). The identifier of the original upselling charge associated with the current charge.
* @return upsellOriginChargeNumber
*/
@javax.annotation.Nullable
public String getUpsellOriginChargeNumber() {
return upsellOriginChargeNumber;
}
public void setUpsellOriginChargeNumber(String upsellOriginChargeNumber) {
this.upsellOriginChargeNumber = upsellOriginChargeNumber;
}
public ChargeOverride validityPeriodType(ValidityPeriodType validityPeriodType) {
this.validityPeriodType = validityPeriodType;
return this;
}
/**
* Get validityPeriodType
* @return validityPeriodType
*/
@javax.annotation.Nullable
public ValidityPeriodType getValidityPeriodType() {
return validityPeriodType;
}
public void setValidityPeriodType(ValidityPeriodType validityPeriodType) {
this.validityPeriodType = validityPeriodType;
}
public ChargeOverride commitmentLevel(CommitmentLevel commitmentLevel) {
this.commitmentLevel = commitmentLevel;
return this;
}
/**
* Get commitmentLevel
* @return commitmentLevel
*/
@javax.annotation.Nullable
public CommitmentLevel getCommitmentLevel() {
return commitmentLevel;
}
public void setCommitmentLevel(CommitmentLevel commitmentLevel) {
this.commitmentLevel = commitmentLevel;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the ChargeOverride instance itself
*/
public ChargeOverride putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ChargeOverride chargeOverride = (ChargeOverride) o;
return Objects.equals(this.name, chargeOverride.name) &&
Objects.equals(this.accountReceivableAccountingCode, chargeOverride.accountReceivableAccountingCode) &&
Objects.equals(this.adjustmentLiabilityAccountingCode, chargeOverride.adjustmentLiabilityAccountingCode) &&
Objects.equals(this.adjustmentRevenueAccountingCode, chargeOverride.adjustmentRevenueAccountingCode) &&
Objects.equals(this.contractAssetAccountingCode, chargeOverride.contractAssetAccountingCode) &&
Objects.equals(this.contractLiabilityAccountingCode, chargeOverride.contractLiabilityAccountingCode) &&
Objects.equals(this.contractRecognizedRevenueAccountingCode, chargeOverride.contractRecognizedRevenueAccountingCode) &&
Objects.equals(this.deferredRevenueAccountingCode, chargeOverride.deferredRevenueAccountingCode) &&
Objects.equals(this.recognizedRevenueAccountingCode, chargeOverride.recognizedRevenueAccountingCode) &&
Objects.equals(this.unBilledReceivablesAccountingCode, chargeOverride.unBilledReceivablesAccountingCode) &&
Objects.equals(this.productCategory, chargeOverride.productCategory) &&
Objects.equals(this.productClass, chargeOverride.productClass) &&
Objects.equals(this.productFamily, chargeOverride.productFamily) &&
Objects.equals(this.productLine, chargeOverride.productLine) &&
Objects.equals(this.chargeModel, chargeOverride.chargeModel) &&
Objects.equals(this.chargeType, chargeOverride.chargeType) &&
Objects.equals(this.taxCode, chargeOverride.taxCode) &&
Objects.equals(this.taxMode, chargeOverride.taxMode) &&
Objects.equals(this.revenueRecognitionTiming, chargeOverride.revenueRecognitionTiming) &&
Objects.equals(this.revenueAmortizationMethod, chargeOverride.revenueAmortizationMethod) &&
Objects.equals(this.isChargeLevelMinCommit, chargeOverride.isChargeLevelMinCommit) &&
Objects.equals(this.commitmentType, chargeOverride.commitmentType) &&
Objects.equals(this.prepaidOperationType, chargeOverride.prepaidOperationType) &&
Objects.equals(this.isCommitted, chargeOverride.isCommitted) &&
Objects.equals(this.chargeFunction, chargeOverride.chargeFunction) &&
Objects.equals(this.prepaidUom, chargeOverride.prepaidUom) &&
Objects.equals(this.drawdownUom, chargeOverride.drawdownUom) &&
Objects.equals(this.prepaidTotalQuantity, chargeOverride.prepaidTotalQuantity) &&
Objects.equals(this.isPrepaid, chargeOverride.isPrepaid) &&
Objects.equals(this.creditOption, chargeOverride.creditOption) &&
Objects.equals(this.billing, chargeOverride.billing) &&
Objects.equals(this.chargeNumber, chargeOverride.chargeNumber) &&
Objects.equals(this.customFields, chargeOverride.customFields) &&
Objects.equals(this.description, chargeOverride.description) &&
Objects.equals(this.drawdownRate, chargeOverride.drawdownRate) &&
Objects.equals(this.endDate, chargeOverride.endDate) &&
Objects.equals(this.excludeItemBillingFromRevenueAccounting, chargeOverride.excludeItemBillingFromRevenueAccounting) &&
Objects.equals(this.excludeItemBookingFromRevenueAccounting, chargeOverride.excludeItemBookingFromRevenueAccounting) &&
Objects.equals(this.isAllocationEligible, chargeOverride.isAllocationEligible) &&
Objects.equals(this.isRollover, chargeOverride.isRollover) &&
Objects.equals(this.isUnbilled, chargeOverride.isUnbilled) &&
Objects.equals(this.prepaidQuantity, chargeOverride.prepaidQuantity) &&
Objects.equals(this.pricing, chargeOverride.pricing) &&
Objects.equals(this.productRatePlanChargeNumber, chargeOverride.productRatePlanChargeNumber) &&
Objects.equals(this.productRatePlanChargeId, chargeOverride.productRatePlanChargeId) &&
Objects.equals(this.prorationOption, chargeOverride.prorationOption) &&
Objects.equals(this.ratingPropertiesOverride, chargeOverride.ratingPropertiesOverride) &&
Objects.equals(this.revRecCode, chargeOverride.revRecCode) &&
Objects.equals(this.revRecTriggerCondition, chargeOverride.revRecTriggerCondition) &&
Objects.equals(this.revenueRecognitionRuleName, chargeOverride.revenueRecognitionRuleName) &&
Objects.equals(this.rolloverApply, chargeOverride.rolloverApply) &&
Objects.equals(this.rolloverPeriods, chargeOverride.rolloverPeriods) &&
Objects.equals(this.startDate, chargeOverride.startDate) &&
Objects.equals(this.uniqueToken, chargeOverride.uniqueToken) &&
Objects.equals(this.upsellOriginChargeNumber, chargeOverride.upsellOriginChargeNumber) &&
Objects.equals(this.validityPeriodType, chargeOverride.validityPeriodType) &&
Objects.equals(this.commitmentLevel, chargeOverride.commitmentLevel)&&
Objects.equals(this.additionalProperties, chargeOverride.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(name, accountReceivableAccountingCode, adjustmentLiabilityAccountingCode, adjustmentRevenueAccountingCode, contractAssetAccountingCode, contractLiabilityAccountingCode, contractRecognizedRevenueAccountingCode, deferredRevenueAccountingCode, recognizedRevenueAccountingCode, unBilledReceivablesAccountingCode, productCategory, productClass, productFamily, productLine, chargeModel, chargeType, taxCode, taxMode, revenueRecognitionTiming, revenueAmortizationMethod, isChargeLevelMinCommit, commitmentType, prepaidOperationType, isCommitted, chargeFunction, prepaidUom, drawdownUom, prepaidTotalQuantity, isPrepaid, creditOption, billing, chargeNumber, customFields, description, drawdownRate, endDate, excludeItemBillingFromRevenueAccounting, excludeItemBookingFromRevenueAccounting, isAllocationEligible, isRollover, isUnbilled, prepaidQuantity, pricing, productRatePlanChargeNumber, productRatePlanChargeId, prorationOption, ratingPropertiesOverride, revRecCode, revRecTriggerCondition, revenueRecognitionRuleName, rolloverApply, rolloverPeriods, startDate, uniqueToken, upsellOriginChargeNumber, validityPeriodType, commitmentLevel, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ChargeOverride {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" accountReceivableAccountingCode: ").append(toIndentedString(accountReceivableAccountingCode)).append("\n");
sb.append(" adjustmentLiabilityAccountingCode: ").append(toIndentedString(adjustmentLiabilityAccountingCode)).append("\n");
sb.append(" adjustmentRevenueAccountingCode: ").append(toIndentedString(adjustmentRevenueAccountingCode)).append("\n");
sb.append(" contractAssetAccountingCode: ").append(toIndentedString(contractAssetAccountingCode)).append("\n");
sb.append(" contractLiabilityAccountingCode: ").append(toIndentedString(contractLiabilityAccountingCode)).append("\n");
sb.append(" contractRecognizedRevenueAccountingCode: ").append(toIndentedString(contractRecognizedRevenueAccountingCode)).append("\n");
sb.append(" deferredRevenueAccountingCode: ").append(toIndentedString(deferredRevenueAccountingCode)).append("\n");
sb.append(" recognizedRevenueAccountingCode: ").append(toIndentedString(recognizedRevenueAccountingCode)).append("\n");
sb.append(" unBilledReceivablesAccountingCode: ").append(toIndentedString(unBilledReceivablesAccountingCode)).append("\n");
sb.append(" productCategory: ").append(toIndentedString(productCategory)).append("\n");
sb.append(" productClass: ").append(toIndentedString(productClass)).append("\n");
sb.append(" productFamily: ").append(toIndentedString(productFamily)).append("\n");
sb.append(" productLine: ").append(toIndentedString(productLine)).append("\n");
sb.append(" chargeModel: ").append(toIndentedString(chargeModel)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxMode: ").append(toIndentedString(taxMode)).append("\n");
sb.append(" revenueRecognitionTiming: ").append(toIndentedString(revenueRecognitionTiming)).append("\n");
sb.append(" revenueAmortizationMethod: ").append(toIndentedString(revenueAmortizationMethod)).append("\n");
sb.append(" isChargeLevelMinCommit: ").append(toIndentedString(isChargeLevelMinCommit)).append("\n");
sb.append(" commitmentType: ").append(toIndentedString(commitmentType)).append("\n");
sb.append(" prepaidOperationType: ").append(toIndentedString(prepaidOperationType)).append("\n");
sb.append(" isCommitted: ").append(toIndentedString(isCommitted)).append("\n");
sb.append(" chargeFunction: ").append(toIndentedString(chargeFunction)).append("\n");
sb.append(" prepaidUom: ").append(toIndentedString(prepaidUom)).append("\n");
sb.append(" drawdownUom: ").append(toIndentedString(drawdownUom)).append("\n");
sb.append(" prepaidTotalQuantity: ").append(toIndentedString(prepaidTotalQuantity)).append("\n");
sb.append(" isPrepaid: ").append(toIndentedString(isPrepaid)).append("\n");
sb.append(" creditOption: ").append(toIndentedString(creditOption)).append("\n");
sb.append(" billing: ").append(toIndentedString(billing)).append("\n");
sb.append(" chargeNumber: ").append(toIndentedString(chargeNumber)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" drawdownRate: ").append(toIndentedString(drawdownRate)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" excludeItemBillingFromRevenueAccounting: ").append(toIndentedString(excludeItemBillingFromRevenueAccounting)).append("\n");
sb.append(" excludeItemBookingFromRevenueAccounting: ").append(toIndentedString(excludeItemBookingFromRevenueAccounting)).append("\n");
sb.append(" isAllocationEligible: ").append(toIndentedString(isAllocationEligible)).append("\n");
sb.append(" isRollover: ").append(toIndentedString(isRollover)).append("\n");
sb.append(" isUnbilled: ").append(toIndentedString(isUnbilled)).append("\n");
sb.append(" prepaidQuantity: ").append(toIndentedString(prepaidQuantity)).append("\n");
sb.append(" pricing: ").append(toIndentedString(pricing)).append("\n");
sb.append(" productRatePlanChargeNumber: ").append(toIndentedString(productRatePlanChargeNumber)).append("\n");
sb.append(" productRatePlanChargeId: ").append(toIndentedString(productRatePlanChargeId)).append("\n");
sb.append(" prorationOption: ").append(toIndentedString(prorationOption)).append("\n");
sb.append(" ratingPropertiesOverride: ").append(toIndentedString(ratingPropertiesOverride)).append("\n");
sb.append(" revRecCode: ").append(toIndentedString(revRecCode)).append("\n");
sb.append(" revRecTriggerCondition: ").append(toIndentedString(revRecTriggerCondition)).append("\n");
sb.append(" revenueRecognitionRuleName: ").append(toIndentedString(revenueRecognitionRuleName)).append("\n");
sb.append(" rolloverApply: ").append(toIndentedString(rolloverApply)).append("\n");
sb.append(" rolloverPeriods: ").append(toIndentedString(rolloverPeriods)).append("\n");
sb.append(" startDate: ").append(toIndentedString(startDate)).append("\n");
sb.append(" uniqueToken: ").append(toIndentedString(uniqueToken)).append("\n");
sb.append(" upsellOriginChargeNumber: ").append(toIndentedString(upsellOriginChargeNumber)).append("\n");
sb.append(" validityPeriodType: ").append(toIndentedString(validityPeriodType)).append("\n");
sb.append(" commitmentLevel: ").append(toIndentedString(commitmentLevel)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("name");
openapiFields.add("accountReceivableAccountingCode");
openapiFields.add("adjustmentLiabilityAccountingCode");
openapiFields.add("adjustmentRevenueAccountingCode");
openapiFields.add("contractAssetAccountingCode");
openapiFields.add("contractLiabilityAccountingCode");
openapiFields.add("contractRecognizedRevenueAccountingCode");
openapiFields.add("deferredRevenueAccountingCode");
openapiFields.add("recognizedRevenueAccountingCode");
openapiFields.add("unBilledReceivablesAccountingCode");
openapiFields.add("productCategory");
openapiFields.add("productClass");
openapiFields.add("productFamily");
openapiFields.add("productLine");
openapiFields.add("chargeModel");
openapiFields.add("chargeType");
openapiFields.add("taxCode");
openapiFields.add("taxMode");
openapiFields.add("revenueRecognitionTiming");
openapiFields.add("revenueAmortizationMethod");
openapiFields.add("isChargeLevelMinCommit");
openapiFields.add("commitmentType");
openapiFields.add("prepaidOperationType");
openapiFields.add("isCommitted");
openapiFields.add("chargeFunction");
openapiFields.add("prepaidUom");
openapiFields.add("drawdownUom");
openapiFields.add("prepaidTotalQuantity");
openapiFields.add("isPrepaid");
openapiFields.add("creditOption");
openapiFields.add("billing");
openapiFields.add("chargeNumber");
openapiFields.add("customFields");
openapiFields.add("description");
openapiFields.add("drawdownRate");
openapiFields.add("endDate");
openapiFields.add("excludeItemBillingFromRevenueAccounting");
openapiFields.add("excludeItemBookingFromRevenueAccounting");
openapiFields.add("isAllocationEligible");
openapiFields.add("isRollover");
openapiFields.add("isUnbilled");
openapiFields.add("prepaidQuantity");
openapiFields.add("pricing");
openapiFields.add("productRatePlanChargeNumber");
openapiFields.add("productRatePlanChargeId");
openapiFields.add("prorationOption");
openapiFields.add("ratingPropertiesOverride");
openapiFields.add("revRecCode");
openapiFields.add("revRecTriggerCondition");
openapiFields.add("revenueRecognitionRuleName");
openapiFields.add("rolloverApply");
openapiFields.add("rolloverPeriods");
openapiFields.add("startDate");
openapiFields.add("uniqueToken");
openapiFields.add("upsellOriginChargeNumber");
openapiFields.add("validityPeriodType");
openapiFields.add("commitmentLevel");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("productRatePlanChargeId");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to ChargeOverride
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!ChargeOverride.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in ChargeOverride is not found in the empty JSON string", ChargeOverride.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : ChargeOverride.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("accountReceivableAccountingCode") != null && !jsonObj.get("accountReceivableAccountingCode").isJsonNull()) && !jsonObj.get("accountReceivableAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountReceivableAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountReceivableAccountingCode").toString()));
}
if ((jsonObj.get("adjustmentLiabilityAccountingCode") != null && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("adjustmentRevenueAccountingCode") != null && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonNull()) && !jsonObj.get("adjustmentRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `adjustmentRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("adjustmentRevenueAccountingCode").toString()));
}
if ((jsonObj.get("contractAssetAccountingCode") != null && !jsonObj.get("contractAssetAccountingCode").isJsonNull()) && !jsonObj.get("contractAssetAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractAssetAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractAssetAccountingCode").toString()));
}
if ((jsonObj.get("contractLiabilityAccountingCode") != null && !jsonObj.get("contractLiabilityAccountingCode").isJsonNull()) && !jsonObj.get("contractLiabilityAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractLiabilityAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractLiabilityAccountingCode").toString()));
}
if ((jsonObj.get("contractRecognizedRevenueAccountingCode") != null && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("contractRecognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `contractRecognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("contractRecognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("deferredRevenueAccountingCode") != null && !jsonObj.get("deferredRevenueAccountingCode").isJsonNull()) && !jsonObj.get("deferredRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `deferredRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("deferredRevenueAccountingCode").toString()));
}
if ((jsonObj.get("recognizedRevenueAccountingCode") != null && !jsonObj.get("recognizedRevenueAccountingCode").isJsonNull()) && !jsonObj.get("recognizedRevenueAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `recognizedRevenueAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("recognizedRevenueAccountingCode").toString()));
}
if ((jsonObj.get("unBilledReceivablesAccountingCode") != null && !jsonObj.get("unBilledReceivablesAccountingCode").isJsonNull()) && !jsonObj.get("unBilledReceivablesAccountingCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `unBilledReceivablesAccountingCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("unBilledReceivablesAccountingCode").toString()));
}
if ((jsonObj.get("productCategory") != null && !jsonObj.get("productCategory").isJsonNull()) && !jsonObj.get("productCategory").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productCategory` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productCategory").toString()));
}
if ((jsonObj.get("productClass") != null && !jsonObj.get("productClass").isJsonNull()) && !jsonObj.get("productClass").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productClass` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productClass").toString()));
}
if ((jsonObj.get("productFamily") != null && !jsonObj.get("productFamily").isJsonNull()) && !jsonObj.get("productFamily").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productFamily` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productFamily").toString()));
}
if ((jsonObj.get("productLine") != null && !jsonObj.get("productLine").isJsonNull()) && !jsonObj.get("productLine").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productLine` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productLine").toString()));
}
// validate the optional field `chargeModel`
if (jsonObj.get("chargeModel") != null && !jsonObj.get("chargeModel").isJsonNull()) {
ChargeModel.validateJsonElement(jsonObj.get("chargeModel"));
}
// validate the optional field `chargeType`
if (jsonObj.get("chargeType") != null && !jsonObj.get("chargeType").isJsonNull()) {
ChargeType.validateJsonElement(jsonObj.get("chargeType"));
}
if ((jsonObj.get("taxCode") != null && !jsonObj.get("taxCode").isJsonNull()) && !jsonObj.get("taxCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `taxCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("taxCode").toString()));
}
// validate the optional field `taxMode`
if (jsonObj.get("taxMode") != null && !jsonObj.get("taxMode").isJsonNull()) {
TaxMode.validateJsonElement(jsonObj.get("taxMode"));
}
if ((jsonObj.get("revenueRecognitionTiming") != null && !jsonObj.get("revenueRecognitionTiming").isJsonNull()) && !jsonObj.get("revenueRecognitionTiming").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionTiming` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionTiming").toString()));
}
if ((jsonObj.get("revenueAmortizationMethod") != null && !jsonObj.get("revenueAmortizationMethod").isJsonNull()) && !jsonObj.get("revenueAmortizationMethod").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueAmortizationMethod` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueAmortizationMethod").toString()));
}
// validate the optional field `commitmentType`
if (jsonObj.get("commitmentType") != null && !jsonObj.get("commitmentType").isJsonNull()) {
CommitmentType.validateJsonElement(jsonObj.get("commitmentType"));
}
// validate the optional field `prepaidOperationType`
if (jsonObj.get("prepaidOperationType") != null && !jsonObj.get("prepaidOperationType").isJsonNull()) {
PrepaidOperationType.validateJsonElement(jsonObj.get("prepaidOperationType"));
}
// validate the optional field `chargeFunction`
if (jsonObj.get("chargeFunction") != null && !jsonObj.get("chargeFunction").isJsonNull()) {
ChargeFunction.validateJsonElement(jsonObj.get("chargeFunction"));
}
if ((jsonObj.get("prepaidUom") != null && !jsonObj.get("prepaidUom").isJsonNull()) && !jsonObj.get("prepaidUom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prepaidUom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prepaidUom").toString()));
}
if ((jsonObj.get("drawdownUom") != null && !jsonObj.get("drawdownUom").isJsonNull()) && !jsonObj.get("drawdownUom").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `drawdownUom` to be a primitive type in the JSON string but got `%s`", jsonObj.get("drawdownUom").toString()));
}
// validate the optional field `creditOption`
if (jsonObj.get("creditOption") != null && !jsonObj.get("creditOption").isJsonNull()) {
PrepaidDrawdownCreditOption.validateJsonElement(jsonObj.get("creditOption"));
}
// validate the optional field `billing`
if (jsonObj.get("billing") != null && !jsonObj.get("billing").isJsonNull()) {
ChargeOverrideBilling.validateJsonElement(jsonObj.get("billing"));
}
if ((jsonObj.get("chargeNumber") != null && !jsonObj.get("chargeNumber").isJsonNull()) && !jsonObj.get("chargeNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeNumber").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
// validate the optional field `endDate`
if (jsonObj.get("endDate") != null && !jsonObj.get("endDate").isJsonNull()) {
EndConditions.validateJsonElement(jsonObj.get("endDate"));
}
// validate the optional field `pricing`
if (jsonObj.get("pricing") != null && !jsonObj.get("pricing").isJsonNull()) {
ChargeOverridePricing.validateJsonElement(jsonObj.get("pricing"));
}
if ((jsonObj.get("productRatePlanChargeNumber") != null && !jsonObj.get("productRatePlanChargeNumber").isJsonNull()) && !jsonObj.get("productRatePlanChargeNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeNumber").toString()));
}
if (!jsonObj.get("productRatePlanChargeId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `productRatePlanChargeId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("productRatePlanChargeId").toString()));
}
if ((jsonObj.get("prorationOption") != null && !jsonObj.get("prorationOption").isJsonNull()) && !jsonObj.get("prorationOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `prorationOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("prorationOption").toString()));
}
// validate the optional field `prorationOption`
if (jsonObj.get("prorationOption") != null && !jsonObj.get("prorationOption").isJsonNull()) {
ProrationOption.validateJsonElement(jsonObj.get("prorationOption"));
}
if ((jsonObj.get("ratingPropertiesOverride") != null && !jsonObj.get("ratingPropertiesOverride").isJsonNull()) && !jsonObj.get("ratingPropertiesOverride").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ratingPropertiesOverride` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ratingPropertiesOverride").toString()));
}
// validate the optional field `ratingPropertiesOverride`
if (jsonObj.get("ratingPropertiesOverride") != null && !jsonObj.get("ratingPropertiesOverride").isJsonNull()) {
RatingPropertiesOverride.validateJsonElement(jsonObj.get("ratingPropertiesOverride"));
}
if ((jsonObj.get("revRecCode") != null && !jsonObj.get("revRecCode").isJsonNull()) && !jsonObj.get("revRecCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecCode").toString()));
}
if ((jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) && !jsonObj.get("revRecTriggerCondition").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revRecTriggerCondition` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revRecTriggerCondition").toString()));
}
// validate the optional field `revRecTriggerCondition`
if (jsonObj.get("revRecTriggerCondition") != null && !jsonObj.get("revRecTriggerCondition").isJsonNull()) {
RevRecTriggerCondition.validateJsonElement(jsonObj.get("revRecTriggerCondition"));
}
if ((jsonObj.get("revenueRecognitionRuleName") != null && !jsonObj.get("revenueRecognitionRuleName").isJsonNull()) && !jsonObj.get("revenueRecognitionRuleName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `revenueRecognitionRuleName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("revenueRecognitionRuleName").toString()));
}
if ((jsonObj.get("rolloverApply") != null && !jsonObj.get("rolloverApply").isJsonNull()) && !jsonObj.get("rolloverApply").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `rolloverApply` to be a primitive type in the JSON string but got `%s`", jsonObj.get("rolloverApply").toString()));
}
// validate the optional field `rolloverApply`
if (jsonObj.get("rolloverApply") != null && !jsonObj.get("rolloverApply").isJsonNull()) {
RolloverApply.validateJsonElement(jsonObj.get("rolloverApply"));
}
// validate the optional field `startDate`
if (jsonObj.get("startDate") != null && !jsonObj.get("startDate").isJsonNull()) {
TriggerParams.validateJsonElement(jsonObj.get("startDate"));
}
if ((jsonObj.get("uniqueToken") != null && !jsonObj.get("uniqueToken").isJsonNull()) && !jsonObj.get("uniqueToken").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `uniqueToken` to be a primitive type in the JSON string but got `%s`", jsonObj.get("uniqueToken").toString()));
}
if ((jsonObj.get("upsellOriginChargeNumber") != null && !jsonObj.get("upsellOriginChargeNumber").isJsonNull()) && !jsonObj.get("upsellOriginChargeNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `upsellOriginChargeNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("upsellOriginChargeNumber").toString()));
}
if ((jsonObj.get("validityPeriodType") != null && !jsonObj.get("validityPeriodType").isJsonNull()) && !jsonObj.get("validityPeriodType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `validityPeriodType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("validityPeriodType").toString()));
}
// validate the optional field `validityPeriodType`
if (jsonObj.get("validityPeriodType") != null && !jsonObj.get("validityPeriodType").isJsonNull()) {
ValidityPeriodType.validateJsonElement(jsonObj.get("validityPeriodType"));
}
// validate the optional field `commitmentLevel`
if (jsonObj.get("commitmentLevel") != null && !jsonObj.get("commitmentLevel").isJsonNull()) {
CommitmentLevel.validateJsonElement(jsonObj.get("commitmentLevel"));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!ChargeOverride.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'ChargeOverride' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(ChargeOverride.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, ChargeOverride value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public ChargeOverride read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
ChargeOverride instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of ChargeOverride given an JSON string
*
* @param jsonString JSON string
* @return An instance of ChargeOverride
* @throws IOException if the JSON string is invalid with respect to ChargeOverride
*/
public static ChargeOverride fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, ChargeOverride.class);
}
/**
* Convert an instance of ChargeOverride to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy