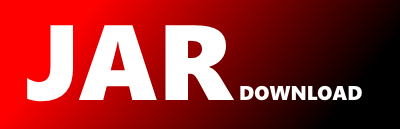
com.zuora.model.CreateAccountPaymentMethod Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreatePaymentMethodBankTransferAccountHolderInfo;
import com.zuora.model.CreatePaymentMethodCardholderInfo;
import com.zuora.model.PaymentMethodACHBankAccountType;
import com.zuora.model.StoredCredentialProfileAction;
import com.zuora.model.StoredCredentialProfileConsentAgreementSrc;
import com.zuora.model.StoredCredentialProfileType;
import java.io.IOException;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* Container for information on payment methods to associate with this account. To associate a credit card payment method when creating an account, Zuora recommends that you use this field instead of the `creditCard` field. Note that you can not use this field together with `creditCard` or `hpmCreditCardPaymentMethodId` to associate a payment method to an account.
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateAccountPaymentMethod {
public static final String SERIALIZED_NAME_ACCOUNT_KEY = "accountKey";
@SerializedName(SERIALIZED_NAME_ACCOUNT_KEY)
private String accountKey;
public static final String SERIALIZED_NAME_AUTH_GATEWAY = "authGateway";
@SerializedName(SERIALIZED_NAME_AUTH_GATEWAY)
private String authGateway;
public static final String SERIALIZED_NAME_IP_ADDRESS = "ipAddress";
@SerializedName(SERIALIZED_NAME_IP_ADDRESS)
private String ipAddress;
public static final String SERIALIZED_NAME_MAKE_DEFAULT = "makeDefault";
@SerializedName(SERIALIZED_NAME_MAKE_DEFAULT)
private Boolean makeDefault = false;
public static final String SERIALIZED_NAME_ADDRESS_LINE1 = "addressLine1";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE1)
private String addressLine1;
public static final String SERIALIZED_NAME_ADDRESS_LINE2 = "addressLine2";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE2)
private String addressLine2;
public static final String SERIALIZED_NAME_BANK_A_B_A_CODE = "bankABACode";
@SerializedName(SERIALIZED_NAME_BANK_A_B_A_CODE)
private String bankABACode;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_NAME = "bankAccountName";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_NAME)
private String bankAccountName;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_NUMBER = "bankAccountNumber";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_NUMBER)
private String bankAccountNumber;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_MASK_NUMBER = "bankAccountMaskNumber";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_MASK_NUMBER)
private String bankAccountMaskNumber;
public static final String SERIALIZED_NAME_BANK_ACCOUNT_TYPE = "bankAccountType";
@SerializedName(SERIALIZED_NAME_BANK_ACCOUNT_TYPE)
private PaymentMethodACHBankAccountType bankAccountType;
public static final String SERIALIZED_NAME_BANK_NAME = "bankName";
@SerializedName(SERIALIZED_NAME_BANK_NAME)
private String bankName;
public static final String SERIALIZED_NAME_CITY = "city";
@SerializedName(SERIALIZED_NAME_CITY)
private String city;
public static final String SERIALIZED_NAME_COUNTRY = "country";
@SerializedName(SERIALIZED_NAME_COUNTRY)
private String country;
public static final String SERIALIZED_NAME_PHONE = "phone";
@SerializedName(SERIALIZED_NAME_PHONE)
private String phone;
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private String state;
public static final String SERIALIZED_NAME_ZIP_CODE = "zipCode";
@SerializedName(SERIALIZED_NAME_ZIP_CODE)
private String zipCode;
public static final String SERIALIZED_NAME_I_B_A_N = "IBAN";
@SerializedName(SERIALIZED_NAME_I_B_A_N)
private String IBAN;
public static final String SERIALIZED_NAME_ACCOUNT_HOLDER_INFO = "accountHolderInfo";
@SerializedName(SERIALIZED_NAME_ACCOUNT_HOLDER_INFO)
private CreatePaymentMethodBankTransferAccountHolderInfo accountHolderInfo;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_BANK_CODE = "bankCode";
@SerializedName(SERIALIZED_NAME_BANK_CODE)
private String bankCode;
public static final String SERIALIZED_NAME_BRANCH_CODE = "branchCode";
@SerializedName(SERIALIZED_NAME_BRANCH_CODE)
private String branchCode;
public static final String SERIALIZED_NAME_BUSINESS_IDENTIFICATION_CODE = "businessIdentificationCode";
@SerializedName(SERIALIZED_NAME_BUSINESS_IDENTIFICATION_CODE)
private String businessIdentificationCode;
public static final String SERIALIZED_NAME_CURRENCY_CODE = "currencyCode";
@SerializedName(SERIALIZED_NAME_CURRENCY_CODE)
private String currencyCode;
public static final String SERIALIZED_NAME_IDENTITY_NUMBER = "identityNumber";
@SerializedName(SERIALIZED_NAME_IDENTITY_NUMBER)
private String identityNumber;
public static final String SERIALIZED_NAME_CARD_HOLDER_INFO = "cardHolderInfo";
@SerializedName(SERIALIZED_NAME_CARD_HOLDER_INFO)
private CreatePaymentMethodCardholderInfo cardHolderInfo;
public static final String SERIALIZED_NAME_CARD_NUMBER = "cardNumber";
@SerializedName(SERIALIZED_NAME_CARD_NUMBER)
private String cardNumber;
public static final String SERIALIZED_NAME_CARD_MASK_NUMBER = "cardMaskNumber";
@SerializedName(SERIALIZED_NAME_CARD_MASK_NUMBER)
private String cardMaskNumber;
public static final String SERIALIZED_NAME_CARD_TYPE = "cardType";
@SerializedName(SERIALIZED_NAME_CARD_TYPE)
private String cardType;
public static final String SERIALIZED_NAME_CHECK_DUPLICATED = "checkDuplicated";
@SerializedName(SERIALIZED_NAME_CHECK_DUPLICATED)
private Boolean checkDuplicated;
public static final String SERIALIZED_NAME_EXPIRATION_MONTH = "expirationMonth";
@SerializedName(SERIALIZED_NAME_EXPIRATION_MONTH)
private Integer expirationMonth;
public static final String SERIALIZED_NAME_EXPIRATION_YEAR = "expirationYear";
@SerializedName(SERIALIZED_NAME_EXPIRATION_YEAR)
private Integer expirationYear;
public static final String SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_REF = "mitConsentAgreementRef";
@SerializedName(SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_REF)
private String mitConsentAgreementRef;
public static final String SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_SRC = "mitConsentAgreementSrc";
@SerializedName(SERIALIZED_NAME_MIT_CONSENT_AGREEMENT_SRC)
private StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc;
public static final String SERIALIZED_NAME_MIT_NETWORK_TRANSACTION_ID = "mitNetworkTransactionId";
@SerializedName(SERIALIZED_NAME_MIT_NETWORK_TRANSACTION_ID)
private String mitNetworkTransactionId;
public static final String SERIALIZED_NAME_MIT_PROFILE_ACTION = "mitProfileAction";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_ACTION)
private StoredCredentialProfileAction mitProfileAction;
public static final String SERIALIZED_NAME_MIT_PROFILE_AGREED_ON = "mitProfileAgreedOn";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_AGREED_ON)
private LocalDate mitProfileAgreedOn;
public static final String SERIALIZED_NAME_MIT_PROFILE_TYPE = "mitProfileType";
@SerializedName(SERIALIZED_NAME_MIT_PROFILE_TYPE)
private StoredCredentialProfileType mitProfileType;
public static final String SERIALIZED_NAME_SECURITY_CODE = "securityCode";
@SerializedName(SERIALIZED_NAME_SECURITY_CODE)
private String securityCode;
public static final String SERIALIZED_NAME_CREDIT_CARD_MASK_NUMBER = "creditCardMaskNumber";
@SerializedName(SERIALIZED_NAME_CREDIT_CARD_MASK_NUMBER)
private String creditCardMaskNumber;
public static final String SERIALIZED_NAME_SECOND_TOKEN_ID = "secondTokenId";
@SerializedName(SERIALIZED_NAME_SECOND_TOKEN_ID)
private String secondTokenId;
public static final String SERIALIZED_NAME_TOKEN_ID = "tokenId";
@SerializedName(SERIALIZED_NAME_TOKEN_ID)
private String tokenId;
public static final String SERIALIZED_NAME_B_A_I_D = "BAID";
@SerializedName(SERIALIZED_NAME_B_A_I_D)
private String BAID;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public static final String SERIALIZED_NAME_PREAPPROVAL_KEY = "preapprovalKey";
@SerializedName(SERIALIZED_NAME_PREAPPROVAL_KEY)
private String preapprovalKey;
public static final String SERIALIZED_NAME_APPLE_PAYMENT_DATA = "applePaymentData";
@SerializedName(SERIALIZED_NAME_APPLE_PAYMENT_DATA)
private String applePaymentData;
public static final String SERIALIZED_NAME_GOOGLE_PAYMENT_TOKEN = "googlePaymentToken";
@SerializedName(SERIALIZED_NAME_GOOGLE_PAYMENT_TOKEN)
private String googlePaymentToken;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public CreateAccountPaymentMethod() {
}
public CreateAccountPaymentMethod accountKey(String accountKey) {
this.accountKey = accountKey;
return this;
}
/**
* Internal ID of the customer account that will own the payment method.
* @return accountKey
*/
@javax.annotation.Nullable
public String getAccountKey() {
return accountKey;
}
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
public CreateAccountPaymentMethod authGateway(String authGateway) {
this.authGateway = authGateway;
return this;
}
/**
* Internal ID of the payment gateway that Zuora will use to authorize the payments that are made with the payment method. If you do not set this field, Zuora will use one of the following payment gateways instead: * The default payment gateway of the customer account that owns the payment method, if the `accountKey` field is set. * The default payment gateway of your Zuora tenant, if the `accountKey` field is not set.
* @return authGateway
*/
@javax.annotation.Nullable
public String getAuthGateway() {
return authGateway;
}
public void setAuthGateway(String authGateway) {
this.authGateway = authGateway;
}
public CreateAccountPaymentMethod ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* The IPv4 or IPv6 information of the user when the payment method is created or updated. Some gateways use this field for fraud prevention. If this field is passed to Zuora, Zuora directly passes it to gateways. If the IP address length is beyond 45 characters, a validation error occurs. For validating SEPA payment methods on Stripe v2, this field is required.
* @return ipAddress
*/
@javax.annotation.Nullable
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public CreateAccountPaymentMethod makeDefault(Boolean makeDefault) {
this.makeDefault = makeDefault;
return this;
}
/**
* Specifies whether the payment method will be the default payment method of the customer account that owns the payment method. Only applicable if the `accountKey` field is set.
* @return makeDefault
*/
@javax.annotation.Nullable
public Boolean getMakeDefault() {
return makeDefault;
}
public void setMakeDefault(Boolean makeDefault) {
this.makeDefault = makeDefault;
}
public CreateAccountPaymentMethod addressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
return this;
}
/**
* First address line, 255 characters or less.
* @return addressLine1
*/
@javax.annotation.Nullable
public String getAddressLine1() {
return addressLine1;
}
public void setAddressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
}
public CreateAccountPaymentMethod addressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
return this;
}
/**
* Second address line, 255 characters or less.
* @return addressLine2
*/
@javax.annotation.Nullable
public String getAddressLine2() {
return addressLine2;
}
public void setAddressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
}
public CreateAccountPaymentMethod bankABACode(String bankABACode) {
this.bankABACode = bankABACode;
return this;
}
/**
* The nine-digit routing number or ABA number used by banks. This field is only required if the `type` field is set to `ACH`.
* @return bankABACode
*/
@javax.annotation.Nullable
public String getBankABACode() {
return bankABACode;
}
public void setBankABACode(String bankABACode) {
this.bankABACode = bankABACode;
}
public CreateAccountPaymentMethod bankAccountName(String bankAccountName) {
this.bankAccountName = bankAccountName;
return this;
}
/**
* The name of the account holder, which can be either a person or a company. This field is only required if the `type` field is set to `ACH`. For ACH payment methods on the BlueSnap integration, see [Overview of BlueSnap gateway integration](https://knowledgecenter.zuora.com/Zuora_Billing/Billing_and_Payments/M_Payment_Gateways/Supported_Payment_Gateways/BlueSnap_Gateway/Overview_of_BlueSnap_gateway_integration#Payer_Name_Extraction) for more information about how Zuora splits the string in this field into two parts and passes them to BlueSnap's `firstName` and `lastName` fields.
* @return bankAccountName
*/
@javax.annotation.Nullable
public String getBankAccountName() {
return bankAccountName;
}
public void setBankAccountName(String bankAccountName) {
this.bankAccountName = bankAccountName;
}
public CreateAccountPaymentMethod bankAccountNumber(String bankAccountNumber) {
this.bankAccountNumber = bankAccountNumber;
return this;
}
/**
* The bank account number associated with the ACH payment. This field is only required if the `type` field is set to `ACH`. However, for creating tokenized ACH payment methods on Stripe v2, this field is optional if the `tokens` and `bankAccountMaskNumber` fields are specified.
* @return bankAccountNumber
*/
@javax.annotation.Nullable
public String getBankAccountNumber() {
return bankAccountNumber;
}
public void setBankAccountNumber(String bankAccountNumber) {
this.bankAccountNumber = bankAccountNumber;
}
public CreateAccountPaymentMethod bankAccountMaskNumber(String bankAccountMaskNumber) {
this.bankAccountMaskNumber = bankAccountMaskNumber;
return this;
}
/**
* The masked bank account number associated with the ACH payment. This field is only required if the ACH payment method is created using tokens.
* @return bankAccountMaskNumber
*/
@javax.annotation.Nullable
public String getBankAccountMaskNumber() {
return bankAccountMaskNumber;
}
public void setBankAccountMaskNumber(String bankAccountMaskNumber) {
this.bankAccountMaskNumber = bankAccountMaskNumber;
}
public CreateAccountPaymentMethod bankAccountType(PaymentMethodACHBankAccountType bankAccountType) {
this.bankAccountType = bankAccountType;
return this;
}
/**
* Get bankAccountType
* @return bankAccountType
*/
@javax.annotation.Nullable
public PaymentMethodACHBankAccountType getBankAccountType() {
return bankAccountType;
}
public void setBankAccountType(PaymentMethodACHBankAccountType bankAccountType) {
this.bankAccountType = bankAccountType;
}
public CreateAccountPaymentMethod bankName(String bankName) {
this.bankName = bankName;
return this;
}
/**
* The name of the bank where the ACH payment account is held. This field is only required if the `type` field is set to `ACH`. When creating an ACH payment method on Adyen, this field is required by Zuora but it is not required by Adyen. To create the ACH payment method successfully, specify a real value for this field if you can. If it is not possible to get the real value for it, specify a dummy value.
* @return bankName
*/
@javax.annotation.Nullable
public String getBankName() {
return bankName;
}
public void setBankName(String bankName) {
this.bankName = bankName;
}
public CreateAccountPaymentMethod city(String city) {
this.city = city;
return this;
}
/**
* City, 40 characters or less. It is recommended to provide the city and country information when creating a payment method. The information will be used to process payments. If the information is not provided during payment method creation, the city and country data will be missing during payment processing.
* @return city
*/
@javax.annotation.Nullable
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public CreateAccountPaymentMethod country(String country) {
this.country = country;
return this;
}
/**
* Country, must be a valid country name or abbreviation. See [Country Names and Their ISO Standard 2- and 3-Digit Codes](https://knowledgecenter.zuora.com/BB_Introducing_Z_Business/D_Country%2C_State%2C_and_Province_Codes/A_Country_Names_and_Their_ISO_Codes) for the list of supported country names and abbreviations. It is recommended to provide the city and country information when creating a payment method. The information will be used to process payments. If the information is not provided during payment method creation, the city and country data will be missing during payment processing.
* @return country
*/
@javax.annotation.Nullable
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public CreateAccountPaymentMethod phone(String phone) {
this.phone = phone;
return this;
}
/**
* Phone number, 40 characters or less.
* @return phone
*/
@javax.annotation.Nullable
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public CreateAccountPaymentMethod state(String state) {
this.state = state;
return this;
}
/**
* State, must be a valid state name or 2-character abbreviation. See [United States Standard State Codes](https://knowledgecenter.zuora.com/BB_Introducing_Z_Business/D_Country%2C_State%2C_and_Province_Codes/B_State_Names_and_2-Digit_Codes) and [Canadian Standard Province Codes](https://knowledgecenter.zuora.com/BB_Introducing_Z_Business/D_Country%2C_State%2C_and_Province_Codes/C_Canadian_Province_Names_and_2-Digit_Codes) for the list of supported names and abbreviations.
* @return state
*/
@javax.annotation.Nullable
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public CreateAccountPaymentMethod zipCode(String zipCode) {
this.zipCode = zipCode;
return this;
}
/**
* Zip code, 20 characters or less.
* @return zipCode
*/
@javax.annotation.Nullable
public String getZipCode() {
return zipCode;
}
public void setZipCode(String zipCode) {
this.zipCode = zipCode;
}
public CreateAccountPaymentMethod IBAN(String IBAN) {
this.IBAN = IBAN;
return this;
}
/**
* The International Bank Account Number. This field is required if the `type` field is set to `SEPA`.
* @return IBAN
*/
@javax.annotation.Nullable
public String getIBAN() {
return IBAN;
}
public void setIBAN(String IBAN) {
this.IBAN = IBAN;
}
public CreateAccountPaymentMethod accountHolderInfo(CreatePaymentMethodBankTransferAccountHolderInfo accountHolderInfo) {
this.accountHolderInfo = accountHolderInfo;
return this;
}
/**
* Get accountHolderInfo
* @return accountHolderInfo
*/
@javax.annotation.Nullable
public CreatePaymentMethodBankTransferAccountHolderInfo getAccountHolderInfo() {
return accountHolderInfo;
}
public void setAccountHolderInfo(CreatePaymentMethodBankTransferAccountHolderInfo accountHolderInfo) {
this.accountHolderInfo = accountHolderInfo;
}
public CreateAccountPaymentMethod accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* The number of the customer's bank account. This field is required for the following bank transfer payment methods: - Direct Entry AU (`Becs`) - Direct Debit NZ (`Becsnz`) - Direct Debit UK (`Bacs`) - Denmark Direct Debit (`Betalingsservice`) - Sweden Direct Debit (`Autogiro`) - Canadian Pre-Authorized Debit (`PAD`)
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public CreateAccountPaymentMethod bankCode(String bankCode) {
this.bankCode = bankCode;
return this;
}
/**
* The sort code or number that identifies the bank. This is also known as the sort code. This field is required for the following bank transfer payment methods: - Direct Debit UK (`Bacs`) - Denmark Direct Debit (`Betalingsservice`) - Direct Debit NZ (`Becsnz`) - Canadian Pre-Authorized Debit (`PAD`)
* @return bankCode
*/
@javax.annotation.Nullable
public String getBankCode() {
return bankCode;
}
public void setBankCode(String bankCode) {
this.bankCode = bankCode;
}
public CreateAccountPaymentMethod branchCode(String branchCode) {
this.branchCode = branchCode;
return this;
}
/**
* The branch code of the bank used for direct debit. This field is required for the following bank transfer payment methods: - Sweden Direct Debit (`Autogiro`) - Direct Entry AU (`Becs`) - Direct Debit NZ (`Becsnz`) - Canadian Pre-Authorized Debit (`PAD`)
* @return branchCode
*/
@javax.annotation.Nullable
public String getBranchCode() {
return branchCode;
}
public void setBranchCode(String branchCode) {
this.branchCode = branchCode;
}
public CreateAccountPaymentMethod businessIdentificationCode(String businessIdentificationCode) {
this.businessIdentificationCode = businessIdentificationCode;
return this;
}
/**
* The BIC code used for SEPA.
* @return businessIdentificationCode
*/
@javax.annotation.Nullable
public String getBusinessIdentificationCode() {
return businessIdentificationCode;
}
public void setBusinessIdentificationCode(String businessIdentificationCode) {
this.businessIdentificationCode = businessIdentificationCode;
}
public CreateAccountPaymentMethod currencyCode(String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/**
* The currency used for payment method authorization. If this field is not specified, `currency` specified for the account is used for payment method authorization. If no currency is specified for the account, the default currency of the account is then used.
* @return currencyCode
*/
@javax.annotation.Nullable
public String getCurrencyCode() {
return currencyCode;
}
public void setCurrencyCode(String currencyCode) {
this.currencyCode = currencyCode;
}
public CreateAccountPaymentMethod identityNumber(String identityNumber) {
this.identityNumber = identityNumber;
return this;
}
/**
* The identity number of the customer. This field is required for the following bank transfer payment methods: - Denmark Direct Debit (`Betalingsservice`) - Sweden Direct Debit (`Autogiro`)
* @return identityNumber
*/
@javax.annotation.Nullable
public String getIdentityNumber() {
return identityNumber;
}
public void setIdentityNumber(String identityNumber) {
this.identityNumber = identityNumber;
}
public CreateAccountPaymentMethod cardHolderInfo(CreatePaymentMethodCardholderInfo cardHolderInfo) {
this.cardHolderInfo = cardHolderInfo;
return this;
}
/**
* Get cardHolderInfo
* @return cardHolderInfo
*/
@javax.annotation.Nullable
public CreatePaymentMethodCardholderInfo getCardHolderInfo() {
return cardHolderInfo;
}
public void setCardHolderInfo(CreatePaymentMethodCardholderInfo cardHolderInfo) {
this.cardHolderInfo = cardHolderInfo;
}
public CreateAccountPaymentMethod cardNumber(String cardNumber) {
this.cardNumber = cardNumber;
return this;
}
/**
* Credit card number. This field is required if `type` is set to `CreditCard`. However, for creating tokenized credit card payment methods, this field is optional if the `tokens` and `cardMaskNumber` fields are specified.
* @return cardNumber
*/
@javax.annotation.Nullable
public String getCardNumber() {
return cardNumber;
}
public void setCardNumber(String cardNumber) {
this.cardNumber = cardNumber;
}
public CreateAccountPaymentMethod cardMaskNumber(String cardMaskNumber) {
this.cardMaskNumber = cardMaskNumber;
return this;
}
/**
* The masked card number associated with the credit card payment. This field is only required if the credit card payment method is created using tokens.
* @return cardMaskNumber
*/
@javax.annotation.Nullable
public String getCardMaskNumber() {
return cardMaskNumber;
}
public void setCardMaskNumber(String cardMaskNumber) {
this.cardMaskNumber = cardMaskNumber;
}
public CreateAccountPaymentMethod cardType(String cardType) {
this.cardType = cardType;
return this;
}
/**
* The type of the credit card. This field is required if `type` is set to `CreditCard`. Possible values include `Visa`, `MasterCard`, `AmericanExpress`, `Discover`, `JCB`, and `Diners`. For more information about credit card types supported by different payment gateways, see [Supported Payment Gateways](https://knowledgecenter.zuora.com/CB_Billing/M_Payment_Gateways/Supported_Payment_Gateways).
* @return cardType
*/
@javax.annotation.Nullable
public String getCardType() {
return cardType;
}
public void setCardType(String cardType) {
this.cardType = cardType;
}
public CreateAccountPaymentMethod checkDuplicated(Boolean checkDuplicated) {
this.checkDuplicated = checkDuplicated;
return this;
}
/**
* Indicates whether the duplication check is performed when you create a new credit card payment method. The default value is `false`. With this field set to `true`, Zuora will check all active payment methods associated with the same billing account to ensure that no duplicate credit card payment methods are created. An error is returned if a duplicate payment method is found. The following fields are used for the duplication check: - `cardHolderName` - `expirationMonth` - `expirationYear` - `creditCardMaskNumber`. It is the masked credit card number generated by Zuora. For example: ``` ************1234 ```
* @return checkDuplicated
*/
@javax.annotation.Nullable
public Boolean getCheckDuplicated() {
return checkDuplicated;
}
public void setCheckDuplicated(Boolean checkDuplicated) {
this.checkDuplicated = checkDuplicated;
}
public CreateAccountPaymentMethod expirationMonth(Integer expirationMonth) {
this.expirationMonth = expirationMonth;
return this;
}
/**
* One or two digit expiration month (1-12) of the credit card. This field is required if `type` is set to `CreditCard`. However, for creating tokenized credit card payment methods, this field is optional if the `tokens` and `cardMaskNumber` fields are specified.
* @return expirationMonth
*/
@javax.annotation.Nullable
public Integer getExpirationMonth() {
return expirationMonth;
}
public void setExpirationMonth(Integer expirationMonth) {
this.expirationMonth = expirationMonth;
}
public CreateAccountPaymentMethod expirationYear(Integer expirationYear) {
this.expirationYear = expirationYear;
return this;
}
/**
* Four-digit expiration year of the credit card. This field is required if `type` is set to `CreditCard`. However, for creating tokenized credit card payment methods, this field is optional if the `tokens` and `cardMaskNumber` fields are specified.
* @return expirationYear
*/
@javax.annotation.Nullable
public Integer getExpirationYear() {
return expirationYear;
}
public void setExpirationYear(Integer expirationYear) {
this.expirationYear = expirationYear;
}
public CreateAccountPaymentMethod mitConsentAgreementRef(String mitConsentAgreementRef) {
this.mitConsentAgreementRef = mitConsentAgreementRef;
return this;
}
/**
* Specifies your reference for the stored credential consent agreement that you have established with the customer. Only applicable if you set the `mitProfileAction` field.
* @return mitConsentAgreementRef
*/
@javax.annotation.Nullable
public String getMitConsentAgreementRef() {
return mitConsentAgreementRef;
}
public void setMitConsentAgreementRef(String mitConsentAgreementRef) {
this.mitConsentAgreementRef = mitConsentAgreementRef;
}
public CreateAccountPaymentMethod mitConsentAgreementSrc(StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc) {
this.mitConsentAgreementSrc = mitConsentAgreementSrc;
return this;
}
/**
* Get mitConsentAgreementSrc
* @return mitConsentAgreementSrc
*/
@javax.annotation.Nullable
public StoredCredentialProfileConsentAgreementSrc getMitConsentAgreementSrc() {
return mitConsentAgreementSrc;
}
public void setMitConsentAgreementSrc(StoredCredentialProfileConsentAgreementSrc mitConsentAgreementSrc) {
this.mitConsentAgreementSrc = mitConsentAgreementSrc;
}
public CreateAccountPaymentMethod mitNetworkTransactionId(String mitNetworkTransactionId) {
this.mitNetworkTransactionId = mitNetworkTransactionId;
return this;
}
/**
* Specifies the ID of a network transaction. Only applicable if you set the `mitProfileAction` field to `Persist`.
* @return mitNetworkTransactionId
*/
@javax.annotation.Nullable
public String getMitNetworkTransactionId() {
return mitNetworkTransactionId;
}
public void setMitNetworkTransactionId(String mitNetworkTransactionId) {
this.mitNetworkTransactionId = mitNetworkTransactionId;
}
public CreateAccountPaymentMethod mitProfileAction(StoredCredentialProfileAction mitProfileAction) {
this.mitProfileAction = mitProfileAction;
return this;
}
/**
* Get mitProfileAction
* @return mitProfileAction
*/
@javax.annotation.Nullable
public StoredCredentialProfileAction getMitProfileAction() {
return mitProfileAction;
}
public void setMitProfileAction(StoredCredentialProfileAction mitProfileAction) {
this.mitProfileAction = mitProfileAction;
}
public CreateAccountPaymentMethod mitProfileAgreedOn(LocalDate mitProfileAgreedOn) {
this.mitProfileAgreedOn = mitProfileAgreedOn;
return this;
}
/**
* The date on which the profile is agreed. The date format is `yyyy-mm-dd`.
* @return mitProfileAgreedOn
*/
@javax.annotation.Nullable
public LocalDate getMitProfileAgreedOn() {
return mitProfileAgreedOn;
}
public void setMitProfileAgreedOn(LocalDate mitProfileAgreedOn) {
this.mitProfileAgreedOn = mitProfileAgreedOn;
}
public CreateAccountPaymentMethod mitProfileType(StoredCredentialProfileType mitProfileType) {
this.mitProfileType = mitProfileType;
return this;
}
/**
* Get mitProfileType
* @return mitProfileType
*/
@javax.annotation.Nullable
public StoredCredentialProfileType getMitProfileType() {
return mitProfileType;
}
public void setMitProfileType(StoredCredentialProfileType mitProfileType) {
this.mitProfileType = mitProfileType;
}
public CreateAccountPaymentMethod securityCode(String securityCode) {
this.securityCode = securityCode;
return this;
}
/**
* CVV or CVV2 security code of the credit card. To ensure PCI compliance, this value is not stored and cannot be queried.
* @return securityCode
*/
@javax.annotation.Nullable
public String getSecurityCode() {
return securityCode;
}
public void setSecurityCode(String securityCode) {
this.securityCode = securityCode;
}
public CreateAccountPaymentMethod creditCardMaskNumber(String creditCardMaskNumber) {
this.creditCardMaskNumber = creditCardMaskNumber;
return this;
}
/**
* The masked credit card number, such as: ``` *********1112 ``` This field is specific for the CC Reference Transaction payment method. It is an optional field that you can use to distinguish different CC Reference Transaction payment methods. Though there are no special restrictions on the input string, it is highly recommended to specify a card number that is masked.
* @return creditCardMaskNumber
*/
@javax.annotation.Nullable
public String getCreditCardMaskNumber() {
return creditCardMaskNumber;
}
public void setCreditCardMaskNumber(String creditCardMaskNumber) {
this.creditCardMaskNumber = creditCardMaskNumber;
}
public CreateAccountPaymentMethod secondTokenId(String secondTokenId) {
this.secondTokenId = secondTokenId;
return this;
}
/**
* A gateway unique identifier that replaces sensitive payment method data. `secondTokenId` is conditionally required only when `tokenId` is being used to represent a gateway customer profile. `secondTokenId` is used in the CC Reference Transaction payment method.
* @return secondTokenId
*/
@javax.annotation.Nullable
public String getSecondTokenId() {
return secondTokenId;
}
public void setSecondTokenId(String secondTokenId) {
this.secondTokenId = secondTokenId;
}
public CreateAccountPaymentMethod tokenId(String tokenId) {
this.tokenId = tokenId;
return this;
}
/**
* A gateway unique identifier that replaces sensitive payment method data or represents a gateway's unique customer profile. `tokenId` is required for the CC Reference Transaction payment method. When `tokenId` is used to represent a customer profile, `secondTokenId` is conditionally required for representing the underlying tokenized payment method. The values for the `tokenId` and `secondTokenId` fields differ for gateways. For more information, see the Knowledge Center article specific to each gateway that supports the CC Reference Transaction payment method. **Note:** When creating an ACH payment method, if you need to pass in tokenized information, use the `mandateId` instead of `tokenId` field.
* @return tokenId
*/
@javax.annotation.Nullable
public String getTokenId() {
return tokenId;
}
public void setTokenId(String tokenId) {
this.tokenId = tokenId;
}
public CreateAccountPaymentMethod BAID(String BAID) {
this.BAID = BAID;
return this;
}
/**
* ID of a PayPal billing agreement. For example, I-1TJ3GAGG82Y9.
* @return BAID
*/
@javax.annotation.Nullable
public String getBAID() {
return BAID;
}
public void setBAID(String BAID) {
this.BAID = BAID;
}
public CreateAccountPaymentMethod email(String email) {
this.email = email;
return this;
}
/**
* Email address associated with the payment method. This field is supported for the following payment methods: - PayPal payment methods. This field is required for creating any of the following PayPal payment methods. - PayPal Express Checkout - PayPal Adaptive - PayPal Commerce Platform - Apple Pay and Google Pay payment methods on Adyen v2.0. This field will be passed to Adyen as `shopperEmail`.
* @return email
*/
@javax.annotation.Nullable
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public CreateAccountPaymentMethod preapprovalKey(String preapprovalKey) {
this.preapprovalKey = preapprovalKey;
return this;
}
/**
* The PayPal preapproval key.
* @return preapprovalKey
*/
@javax.annotation.Nullable
public String getPreapprovalKey() {
return preapprovalKey;
}
public void setPreapprovalKey(String preapprovalKey) {
this.preapprovalKey = preapprovalKey;
}
public CreateAccountPaymentMethod applePaymentData(String applePaymentData) {
this.applePaymentData = applePaymentData;
return this;
}
/**
* This field is specific for setting up Apple Pay for Adyen to include payload with Apple Pay token or Apple payment data. This information should be stringified. For more information, see [Set up Adyen Apple Pay](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/Payment_Method_Types/Apple_Pay_on_Web/Set_up_Adyen_Apple_Pay).
* @return applePaymentData
*/
@javax.annotation.Nullable
public String getApplePaymentData() {
return applePaymentData;
}
public void setApplePaymentData(String applePaymentData) {
this.applePaymentData = applePaymentData;
}
public CreateAccountPaymentMethod googlePaymentToken(String googlePaymentToken) {
this.googlePaymentToken = googlePaymentToken;
return this;
}
/**
* This field is specific for setting up Google Pay for Adyen and Chase gateway integrations to specify the stringified Google Pay token. For more information, see [Set up Adyen Google Pay](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/Payment_Method_Types/Set_up_Adyen_Google_Pay) and [Set up Google Pay on Chase](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/Payment_Method_Types/Set_up_Google_Pay_on_Chase).
* @return googlePaymentToken
*/
@javax.annotation.Nullable
public String getGooglePaymentToken() {
return googlePaymentToken;
}
public void setGooglePaymentToken(String googlePaymentToken) {
this.googlePaymentToken = googlePaymentToken;
}
public CreateAccountPaymentMethod type(String type) {
this.type = type;
return this;
}
/**
* Type of payment method. Possible values include: * `CreditCard` - Credit card payment method. * `CreditCardReferenceTransaction` - Credit Card Reference Transaction. See [Supported payment methods](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/Supported_Payment_Methods) for payment gateways that support this type of payment method. * `ACH` - ACH payment method. * `SEPA` - Single Euro Payments Area. * `Betalingsservice` - Direct Debit DK. * `Autogiro` - Direct Debit SE. * `Bacs` - Direct Debit UK. * `Becs` - Direct Entry AU. * `Becsnz` - Direct Debit NZ. * `PAD` - Pre-Authorized Debit. * `PayPalCP` - PayPal Commerce Platform payment method. Use this type if you are using a [PayPal Commerce Platform Gateway](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/M_Payment_Gateways/Supported_Payment_Gateways/PayPal_Commerce_Platform_Gateway) instance. * `PayPalEC` - PayPal Express Checkout payment method. Use this type if you are using a [PayPal Payflow Pro Gateway](https://knowledgecenter.zuora.com/CB_Billing/M_Payment_Gateways/Supported_Payment_Gateways/PayPal_Payflow_Pro%2C_Website_Payments_Payflow_Edition%2C_Website_Pro_Payment_Gateway) instance. * `PayPalNativeEC` - PayPal Native Express Checkout payment method. Use this type if you are using a [PayPal Express Checkout Gateway](https://knowledgecenter.zuora.com/CB_Billing/M_Payment_Gateways/Supported_Payment_Gateways/PayPal_Express_Checkout_Gateway) instance. * `PayPalAdaptive` - PayPal Adaptive payment method. Use this type if you are using a [PayPal Adaptive Payment Gateway](https://knowledgecenter.zuora.com/CB_Billing/M_Payment_Gateways/Supported_Payment_Gateways/PayPal_Adaptive_Payments_Gateway) instance. * `AdyenApplePay` - Apple Pay on Adyen Integration v2.0. See [Set up Adyen Apple Pay](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/Payment_Method_Types/Apple_Pay_on_Web/Set_up_Adyen_Apple_Pay) for details. * `AdyenGooglePay` - Google Pay on Adyen Integration v2.0. See [Set up Adyen Google Pay](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/Payment_Method_Types/Set_up_Adyen_Google_Pay) for details. * `GooglePay` - Google Pay on Chase Paymentech Orbital gateway integration. See [Set up Google Pay on Chase](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/L_Payment_Methods/Payment_Method_Types/Set_up_Google_Pay_on_Chase) for details. * You can also specify a custom payment method type. See [Set up custom payment gateways and payment methods](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/MB_Set_up_custom_payment_gateways_and_payment_methods) for details.
* @return type
*/
@javax.annotation.Nonnull
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateAccountPaymentMethod instance itself
*/
public CreateAccountPaymentMethod putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateAccountPaymentMethod createAccountPaymentMethod = (CreateAccountPaymentMethod) o;
return Objects.equals(this.accountKey, createAccountPaymentMethod.accountKey) &&
Objects.equals(this.authGateway, createAccountPaymentMethod.authGateway) &&
Objects.equals(this.ipAddress, createAccountPaymentMethod.ipAddress) &&
Objects.equals(this.makeDefault, createAccountPaymentMethod.makeDefault) &&
Objects.equals(this.addressLine1, createAccountPaymentMethod.addressLine1) &&
Objects.equals(this.addressLine2, createAccountPaymentMethod.addressLine2) &&
Objects.equals(this.bankABACode, createAccountPaymentMethod.bankABACode) &&
Objects.equals(this.bankAccountName, createAccountPaymentMethod.bankAccountName) &&
Objects.equals(this.bankAccountNumber, createAccountPaymentMethod.bankAccountNumber) &&
Objects.equals(this.bankAccountMaskNumber, createAccountPaymentMethod.bankAccountMaskNumber) &&
Objects.equals(this.bankAccountType, createAccountPaymentMethod.bankAccountType) &&
Objects.equals(this.bankName, createAccountPaymentMethod.bankName) &&
Objects.equals(this.city, createAccountPaymentMethod.city) &&
Objects.equals(this.country, createAccountPaymentMethod.country) &&
Objects.equals(this.phone, createAccountPaymentMethod.phone) &&
Objects.equals(this.state, createAccountPaymentMethod.state) &&
Objects.equals(this.zipCode, createAccountPaymentMethod.zipCode) &&
Objects.equals(this.IBAN, createAccountPaymentMethod.IBAN) &&
Objects.equals(this.accountHolderInfo, createAccountPaymentMethod.accountHolderInfo) &&
Objects.equals(this.accountNumber, createAccountPaymentMethod.accountNumber) &&
Objects.equals(this.bankCode, createAccountPaymentMethod.bankCode) &&
Objects.equals(this.branchCode, createAccountPaymentMethod.branchCode) &&
Objects.equals(this.businessIdentificationCode, createAccountPaymentMethod.businessIdentificationCode) &&
Objects.equals(this.currencyCode, createAccountPaymentMethod.currencyCode) &&
Objects.equals(this.identityNumber, createAccountPaymentMethod.identityNumber) &&
Objects.equals(this.cardHolderInfo, createAccountPaymentMethod.cardHolderInfo) &&
Objects.equals(this.cardNumber, createAccountPaymentMethod.cardNumber) &&
Objects.equals(this.cardMaskNumber, createAccountPaymentMethod.cardMaskNumber) &&
Objects.equals(this.cardType, createAccountPaymentMethod.cardType) &&
Objects.equals(this.checkDuplicated, createAccountPaymentMethod.checkDuplicated) &&
Objects.equals(this.expirationMonth, createAccountPaymentMethod.expirationMonth) &&
Objects.equals(this.expirationYear, createAccountPaymentMethod.expirationYear) &&
Objects.equals(this.mitConsentAgreementRef, createAccountPaymentMethod.mitConsentAgreementRef) &&
Objects.equals(this.mitConsentAgreementSrc, createAccountPaymentMethod.mitConsentAgreementSrc) &&
Objects.equals(this.mitNetworkTransactionId, createAccountPaymentMethod.mitNetworkTransactionId) &&
Objects.equals(this.mitProfileAction, createAccountPaymentMethod.mitProfileAction) &&
Objects.equals(this.mitProfileAgreedOn, createAccountPaymentMethod.mitProfileAgreedOn) &&
Objects.equals(this.mitProfileType, createAccountPaymentMethod.mitProfileType) &&
Objects.equals(this.securityCode, createAccountPaymentMethod.securityCode) &&
Objects.equals(this.creditCardMaskNumber, createAccountPaymentMethod.creditCardMaskNumber) &&
Objects.equals(this.secondTokenId, createAccountPaymentMethod.secondTokenId) &&
Objects.equals(this.tokenId, createAccountPaymentMethod.tokenId) &&
Objects.equals(this.BAID, createAccountPaymentMethod.BAID) &&
Objects.equals(this.email, createAccountPaymentMethod.email) &&
Objects.equals(this.preapprovalKey, createAccountPaymentMethod.preapprovalKey) &&
Objects.equals(this.applePaymentData, createAccountPaymentMethod.applePaymentData) &&
Objects.equals(this.googlePaymentToken, createAccountPaymentMethod.googlePaymentToken) &&
Objects.equals(this.type, createAccountPaymentMethod.type)&&
Objects.equals(this.additionalProperties, createAccountPaymentMethod.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(accountKey, authGateway, ipAddress, makeDefault, addressLine1, addressLine2, bankABACode, bankAccountName, bankAccountNumber, bankAccountMaskNumber, bankAccountType, bankName, city, country, phone, state, zipCode, IBAN, accountHolderInfo, accountNumber, bankCode, branchCode, businessIdentificationCode, currencyCode, identityNumber, cardHolderInfo, cardNumber, cardMaskNumber, cardType, checkDuplicated, expirationMonth, expirationYear, mitConsentAgreementRef, mitConsentAgreementSrc, mitNetworkTransactionId, mitProfileAction, mitProfileAgreedOn, mitProfileType, securityCode, creditCardMaskNumber, secondTokenId, tokenId, BAID, email, preapprovalKey, applePaymentData, googlePaymentToken, type, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateAccountPaymentMethod {\n");
sb.append(" accountKey: ").append(toIndentedString(accountKey)).append("\n");
sb.append(" authGateway: ").append(toIndentedString(authGateway)).append("\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append(" makeDefault: ").append(toIndentedString(makeDefault)).append("\n");
sb.append(" addressLine1: ").append(toIndentedString(addressLine1)).append("\n");
sb.append(" addressLine2: ").append(toIndentedString(addressLine2)).append("\n");
sb.append(" bankABACode: ").append(toIndentedString(bankABACode)).append("\n");
sb.append(" bankAccountName: ").append(toIndentedString(bankAccountName)).append("\n");
sb.append(" bankAccountNumber: ").append(toIndentedString(bankAccountNumber)).append("\n");
sb.append(" bankAccountMaskNumber: ").append(toIndentedString(bankAccountMaskNumber)).append("\n");
sb.append(" bankAccountType: ").append(toIndentedString(bankAccountType)).append("\n");
sb.append(" bankName: ").append(toIndentedString(bankName)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" zipCode: ").append(toIndentedString(zipCode)).append("\n");
sb.append(" IBAN: ").append(toIndentedString(IBAN)).append("\n");
sb.append(" accountHolderInfo: ").append(toIndentedString(accountHolderInfo)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" bankCode: ").append(toIndentedString(bankCode)).append("\n");
sb.append(" branchCode: ").append(toIndentedString(branchCode)).append("\n");
sb.append(" businessIdentificationCode: ").append(toIndentedString(businessIdentificationCode)).append("\n");
sb.append(" currencyCode: ").append(toIndentedString(currencyCode)).append("\n");
sb.append(" identityNumber: ").append(toIndentedString(identityNumber)).append("\n");
sb.append(" cardHolderInfo: ").append(toIndentedString(cardHolderInfo)).append("\n");
sb.append(" cardNumber: ").append(toIndentedString(cardNumber)).append("\n");
sb.append(" cardMaskNumber: ").append(toIndentedString(cardMaskNumber)).append("\n");
sb.append(" cardType: ").append(toIndentedString(cardType)).append("\n");
sb.append(" checkDuplicated: ").append(toIndentedString(checkDuplicated)).append("\n");
sb.append(" expirationMonth: ").append(toIndentedString(expirationMonth)).append("\n");
sb.append(" expirationYear: ").append(toIndentedString(expirationYear)).append("\n");
sb.append(" mitConsentAgreementRef: ").append(toIndentedString(mitConsentAgreementRef)).append("\n");
sb.append(" mitConsentAgreementSrc: ").append(toIndentedString(mitConsentAgreementSrc)).append("\n");
sb.append(" mitNetworkTransactionId: ").append(toIndentedString(mitNetworkTransactionId)).append("\n");
sb.append(" mitProfileAction: ").append(toIndentedString(mitProfileAction)).append("\n");
sb.append(" mitProfileAgreedOn: ").append(toIndentedString(mitProfileAgreedOn)).append("\n");
sb.append(" mitProfileType: ").append(toIndentedString(mitProfileType)).append("\n");
sb.append(" securityCode: ").append(toIndentedString(securityCode)).append("\n");
sb.append(" creditCardMaskNumber: ").append(toIndentedString(creditCardMaskNumber)).append("\n");
sb.append(" secondTokenId: ").append(toIndentedString(secondTokenId)).append("\n");
sb.append(" tokenId: ").append(toIndentedString(tokenId)).append("\n");
sb.append(" BAID: ").append(toIndentedString(BAID)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" preapprovalKey: ").append(toIndentedString(preapprovalKey)).append("\n");
sb.append(" applePaymentData: ").append(toIndentedString(applePaymentData)).append("\n");
sb.append(" googlePaymentToken: ").append(toIndentedString(googlePaymentToken)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("accountKey");
openapiFields.add("authGateway");
openapiFields.add("ipAddress");
openapiFields.add("makeDefault");
openapiFields.add("addressLine1");
openapiFields.add("addressLine2");
openapiFields.add("bankABACode");
openapiFields.add("bankAccountName");
openapiFields.add("bankAccountNumber");
openapiFields.add("bankAccountMaskNumber");
openapiFields.add("bankAccountType");
openapiFields.add("bankName");
openapiFields.add("city");
openapiFields.add("country");
openapiFields.add("phone");
openapiFields.add("state");
openapiFields.add("zipCode");
openapiFields.add("IBAN");
openapiFields.add("accountHolderInfo");
openapiFields.add("accountNumber");
openapiFields.add("bankCode");
openapiFields.add("branchCode");
openapiFields.add("businessIdentificationCode");
openapiFields.add("currencyCode");
openapiFields.add("identityNumber");
openapiFields.add("cardHolderInfo");
openapiFields.add("cardNumber");
openapiFields.add("cardMaskNumber");
openapiFields.add("cardType");
openapiFields.add("checkDuplicated");
openapiFields.add("expirationMonth");
openapiFields.add("expirationYear");
openapiFields.add("mitConsentAgreementRef");
openapiFields.add("mitConsentAgreementSrc");
openapiFields.add("mitNetworkTransactionId");
openapiFields.add("mitProfileAction");
openapiFields.add("mitProfileAgreedOn");
openapiFields.add("mitProfileType");
openapiFields.add("securityCode");
openapiFields.add("creditCardMaskNumber");
openapiFields.add("secondTokenId");
openapiFields.add("tokenId");
openapiFields.add("BAID");
openapiFields.add("email");
openapiFields.add("preapprovalKey");
openapiFields.add("applePaymentData");
openapiFields.add("googlePaymentToken");
openapiFields.add("type");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("type");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateAccountPaymentMethod
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateAccountPaymentMethod.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateAccountPaymentMethod is not found in the empty JSON string", CreateAccountPaymentMethod.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateAccountPaymentMethod.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("accountKey") != null && !jsonObj.get("accountKey").isJsonNull()) && !jsonObj.get("accountKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountKey").toString()));
}
if ((jsonObj.get("authGateway") != null && !jsonObj.get("authGateway").isJsonNull()) && !jsonObj.get("authGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `authGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("authGateway").toString()));
}
if ((jsonObj.get("ipAddress") != null && !jsonObj.get("ipAddress").isJsonNull()) && !jsonObj.get("ipAddress").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ipAddress` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ipAddress").toString()));
}
if ((jsonObj.get("addressLine1") != null && !jsonObj.get("addressLine1").isJsonNull()) && !jsonObj.get("addressLine1").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `addressLine1` to be a primitive type in the JSON string but got `%s`", jsonObj.get("addressLine1").toString()));
}
if ((jsonObj.get("addressLine2") != null && !jsonObj.get("addressLine2").isJsonNull()) && !jsonObj.get("addressLine2").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `addressLine2` to be a primitive type in the JSON string but got `%s`", jsonObj.get("addressLine2").toString()));
}
if ((jsonObj.get("bankABACode") != null && !jsonObj.get("bankABACode").isJsonNull()) && !jsonObj.get("bankABACode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankABACode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankABACode").toString()));
}
if ((jsonObj.get("bankAccountName") != null && !jsonObj.get("bankAccountName").isJsonNull()) && !jsonObj.get("bankAccountName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankAccountName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankAccountName").toString()));
}
if ((jsonObj.get("bankAccountNumber") != null && !jsonObj.get("bankAccountNumber").isJsonNull()) && !jsonObj.get("bankAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankAccountNumber").toString()));
}
if ((jsonObj.get("bankAccountMaskNumber") != null && !jsonObj.get("bankAccountMaskNumber").isJsonNull()) && !jsonObj.get("bankAccountMaskNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankAccountMaskNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankAccountMaskNumber").toString()));
}
if ((jsonObj.get("bankAccountType") != null && !jsonObj.get("bankAccountType").isJsonNull()) && !jsonObj.get("bankAccountType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankAccountType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankAccountType").toString()));
}
// validate the optional field `bankAccountType`
if (jsonObj.get("bankAccountType") != null && !jsonObj.get("bankAccountType").isJsonNull()) {
PaymentMethodACHBankAccountType.validateJsonElement(jsonObj.get("bankAccountType"));
}
if ((jsonObj.get("bankName") != null && !jsonObj.get("bankName").isJsonNull()) && !jsonObj.get("bankName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankName").toString()));
}
if ((jsonObj.get("city") != null && !jsonObj.get("city").isJsonNull()) && !jsonObj.get("city").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `city` to be a primitive type in the JSON string but got `%s`", jsonObj.get("city").toString()));
}
if ((jsonObj.get("country") != null && !jsonObj.get("country").isJsonNull()) && !jsonObj.get("country").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `country` to be a primitive type in the JSON string but got `%s`", jsonObj.get("country").toString()));
}
if ((jsonObj.get("phone") != null && !jsonObj.get("phone").isJsonNull()) && !jsonObj.get("phone").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `phone` to be a primitive type in the JSON string but got `%s`", jsonObj.get("phone").toString()));
}
if ((jsonObj.get("state") != null && !jsonObj.get("state").isJsonNull()) && !jsonObj.get("state").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `state` to be a primitive type in the JSON string but got `%s`", jsonObj.get("state").toString()));
}
if ((jsonObj.get("zipCode") != null && !jsonObj.get("zipCode").isJsonNull()) && !jsonObj.get("zipCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `zipCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("zipCode").toString()));
}
if ((jsonObj.get("IBAN") != null && !jsonObj.get("IBAN").isJsonNull()) && !jsonObj.get("IBAN").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IBAN` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IBAN").toString()));
}
// validate the optional field `accountHolderInfo`
if (jsonObj.get("accountHolderInfo") != null && !jsonObj.get("accountHolderInfo").isJsonNull()) {
CreatePaymentMethodBankTransferAccountHolderInfo.validateJsonElement(jsonObj.get("accountHolderInfo"));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
if ((jsonObj.get("bankCode") != null && !jsonObj.get("bankCode").isJsonNull()) && !jsonObj.get("bankCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `bankCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("bankCode").toString()));
}
if ((jsonObj.get("branchCode") != null && !jsonObj.get("branchCode").isJsonNull()) && !jsonObj.get("branchCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `branchCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("branchCode").toString()));
}
if ((jsonObj.get("businessIdentificationCode") != null && !jsonObj.get("businessIdentificationCode").isJsonNull()) && !jsonObj.get("businessIdentificationCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `businessIdentificationCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("businessIdentificationCode").toString()));
}
if ((jsonObj.get("currencyCode") != null && !jsonObj.get("currencyCode").isJsonNull()) && !jsonObj.get("currencyCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currencyCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currencyCode").toString()));
}
if ((jsonObj.get("identityNumber") != null && !jsonObj.get("identityNumber").isJsonNull()) && !jsonObj.get("identityNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `identityNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("identityNumber").toString()));
}
// validate the optional field `cardHolderInfo`
if (jsonObj.get("cardHolderInfo") != null && !jsonObj.get("cardHolderInfo").isJsonNull()) {
CreatePaymentMethodCardholderInfo.validateJsonElement(jsonObj.get("cardHolderInfo"));
}
if ((jsonObj.get("cardNumber") != null && !jsonObj.get("cardNumber").isJsonNull()) && !jsonObj.get("cardNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cardNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cardNumber").toString()));
}
if ((jsonObj.get("cardMaskNumber") != null && !jsonObj.get("cardMaskNumber").isJsonNull()) && !jsonObj.get("cardMaskNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cardMaskNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cardMaskNumber").toString()));
}
if ((jsonObj.get("cardType") != null && !jsonObj.get("cardType").isJsonNull()) && !jsonObj.get("cardType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `cardType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("cardType").toString()));
}
if ((jsonObj.get("mitConsentAgreementRef") != null && !jsonObj.get("mitConsentAgreementRef").isJsonNull()) && !jsonObj.get("mitConsentAgreementRef").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitConsentAgreementRef` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitConsentAgreementRef").toString()));
}
if ((jsonObj.get("mitConsentAgreementSrc") != null && !jsonObj.get("mitConsentAgreementSrc").isJsonNull()) && !jsonObj.get("mitConsentAgreementSrc").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitConsentAgreementSrc` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitConsentAgreementSrc").toString()));
}
// validate the optional field `mitConsentAgreementSrc`
if (jsonObj.get("mitConsentAgreementSrc") != null && !jsonObj.get("mitConsentAgreementSrc").isJsonNull()) {
StoredCredentialProfileConsentAgreementSrc.validateJsonElement(jsonObj.get("mitConsentAgreementSrc"));
}
if ((jsonObj.get("mitNetworkTransactionId") != null && !jsonObj.get("mitNetworkTransactionId").isJsonNull()) && !jsonObj.get("mitNetworkTransactionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitNetworkTransactionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitNetworkTransactionId").toString()));
}
if ((jsonObj.get("mitProfileAction") != null && !jsonObj.get("mitProfileAction").isJsonNull()) && !jsonObj.get("mitProfileAction").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitProfileAction` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitProfileAction").toString()));
}
// validate the optional field `mitProfileAction`
if (jsonObj.get("mitProfileAction") != null && !jsonObj.get("mitProfileAction").isJsonNull()) {
StoredCredentialProfileAction.validateJsonElement(jsonObj.get("mitProfileAction"));
}
if ((jsonObj.get("mitProfileType") != null && !jsonObj.get("mitProfileType").isJsonNull()) && !jsonObj.get("mitProfileType").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mitProfileType` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mitProfileType").toString()));
}
// validate the optional field `mitProfileType`
if (jsonObj.get("mitProfileType") != null && !jsonObj.get("mitProfileType").isJsonNull()) {
StoredCredentialProfileType.validateJsonElement(jsonObj.get("mitProfileType"));
}
if ((jsonObj.get("securityCode") != null && !jsonObj.get("securityCode").isJsonNull()) && !jsonObj.get("securityCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `securityCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("securityCode").toString()));
}
if ((jsonObj.get("creditCardMaskNumber") != null && !jsonObj.get("creditCardMaskNumber").isJsonNull()) && !jsonObj.get("creditCardMaskNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditCardMaskNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditCardMaskNumber").toString()));
}
if ((jsonObj.get("secondTokenId") != null && !jsonObj.get("secondTokenId").isJsonNull()) && !jsonObj.get("secondTokenId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `secondTokenId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("secondTokenId").toString()));
}
if ((jsonObj.get("tokenId") != null && !jsonObj.get("tokenId").isJsonNull()) && !jsonObj.get("tokenId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tokenId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tokenId").toString()));
}
if ((jsonObj.get("BAID") != null && !jsonObj.get("BAID").isJsonNull()) && !jsonObj.get("BAID").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `BAID` to be a primitive type in the JSON string but got `%s`", jsonObj.get("BAID").toString()));
}
if ((jsonObj.get("email") != null && !jsonObj.get("email").isJsonNull()) && !jsonObj.get("email").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `email` to be a primitive type in the JSON string but got `%s`", jsonObj.get("email").toString()));
}
if ((jsonObj.get("preapprovalKey") != null && !jsonObj.get("preapprovalKey").isJsonNull()) && !jsonObj.get("preapprovalKey").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `preapprovalKey` to be a primitive type in the JSON string but got `%s`", jsonObj.get("preapprovalKey").toString()));
}
if ((jsonObj.get("applePaymentData") != null && !jsonObj.get("applePaymentData").isJsonNull()) && !jsonObj.get("applePaymentData").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `applePaymentData` to be a primitive type in the JSON string but got `%s`", jsonObj.get("applePaymentData").toString()));
}
if ((jsonObj.get("googlePaymentToken") != null && !jsonObj.get("googlePaymentToken").isJsonNull()) && !jsonObj.get("googlePaymentToken").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `googlePaymentToken` to be a primitive type in the JSON string but got `%s`", jsonObj.get("googlePaymentToken").toString()));
}
if (!jsonObj.get("type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateAccountPaymentMethod.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateAccountPaymentMethod' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateAccountPaymentMethod.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateAccountPaymentMethod value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateAccountPaymentMethod read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateAccountPaymentMethod instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateAccountPaymentMethod given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateAccountPaymentMethod
* @throws IOException if the JSON string is invalid with respect to CreateAccountPaymentMethod
*/
public static CreateAccountPaymentMethod fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateAccountPaymentMethod.class);
}
/**
* Convert an instance of CreateAccountPaymentMethod to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy