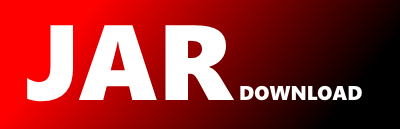
com.zuora.model.CreateAccountRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.AccountEInvoiceProfile;
import com.zuora.model.AccountObjectNSFieldsCustomerTypeNS;
import com.zuora.model.AccountObjectNSFieldsSynctoNetSuiteNS;
import com.zuora.model.CreateAccountContact;
import com.zuora.model.CreateAccountCreditCard;
import com.zuora.model.CreateAccountPaymentMethod;
import com.zuora.model.CreateAccountSubscription;
import com.zuora.model.TaxInfo;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateAccountRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateAccountRequest {
public static final String SERIALIZED_NAME_CLASS_N_S = "Class__NS";
@SerializedName(SERIALIZED_NAME_CLASS_N_S)
private String classNS;
public static final String SERIALIZED_NAME_CUSTOMER_TYPE_N_S = "CustomerType__NS";
@SerializedName(SERIALIZED_NAME_CUSTOMER_TYPE_N_S)
private AccountObjectNSFieldsCustomerTypeNS customerTypeNS;
public static final String SERIALIZED_NAME_DEPARTMENT_N_S = "Department__NS";
@SerializedName(SERIALIZED_NAME_DEPARTMENT_N_S)
private String departmentNS;
public static final String SERIALIZED_NAME_INTEGRATION_ID_N_S = "IntegrationId__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_ID_N_S)
private String integrationIdNS;
public static final String SERIALIZED_NAME_INTEGRATION_STATUS_N_S = "IntegrationStatus__NS";
@SerializedName(SERIALIZED_NAME_INTEGRATION_STATUS_N_S)
private String integrationStatusNS;
public static final String SERIALIZED_NAME_LOCATION_N_S = "Location__NS";
@SerializedName(SERIALIZED_NAME_LOCATION_N_S)
private String locationNS;
public static final String SERIALIZED_NAME_SUBSIDIARY_N_S = "Subsidiary__NS";
@SerializedName(SERIALIZED_NAME_SUBSIDIARY_N_S)
private String subsidiaryNS;
public static final String SERIALIZED_NAME_SYNC_DATE_N_S = "SyncDate__NS";
@SerializedName(SERIALIZED_NAME_SYNC_DATE_N_S)
private String syncDateNS;
public static final String SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S = "SynctoNetSuite__NS";
@SerializedName(SERIALIZED_NAME_SYNCTO_NET_SUITE_N_S)
private AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES = "additionalEmailAddresses";
@SerializedName(SERIALIZED_NAME_ADDITIONAL_EMAIL_ADDRESSES)
private List additionalEmailAddresses;
public static final String SERIALIZED_NAME_APPLICATION_ORDER = "applicationOrder";
@SerializedName(SERIALIZED_NAME_APPLICATION_ORDER)
private List applicationOrder;
public static final String SERIALIZED_NAME_APPLY_CREDIT = "applyCredit";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT)
private Boolean applyCredit;
public static final String SERIALIZED_NAME_APPLY_CREDIT_BALANCE = "applyCreditBalance";
@SerializedName(SERIALIZED_NAME_APPLY_CREDIT_BALANCE)
private Boolean applyCreditBalance;
public static final String SERIALIZED_NAME_AUTO_PAY = "autoPay";
@SerializedName(SERIALIZED_NAME_AUTO_PAY)
private Boolean autoPay;
public static final String SERIALIZED_NAME_BATCH = "batch";
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BILL_CYCLE_DAY = "billCycleDay";
@SerializedName(SERIALIZED_NAME_BILL_CYCLE_DAY)
private Integer billCycleDay;
public static final String SERIALIZED_NAME_BILL_TO_CONTACT = "billToContact";
@SerializedName(SERIALIZED_NAME_BILL_TO_CONTACT)
private CreateAccountContact billToContact;
public static final String SERIALIZED_NAME_COLLECT = "collect";
@SerializedName(SERIALIZED_NAME_COLLECT)
private Boolean collect;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_ID = "communicationProfileId";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_ID)
private String communicationProfileId;
public static final String SERIALIZED_NAME_CREDIT_CARD = "creditCard";
@SerializedName(SERIALIZED_NAME_CREDIT_CARD)
private CreateAccountCreditCard creditCard;
public static final String SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE = "creditMemoReasonCode";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_REASON_CODE)
private String creditMemoReasonCode;
public static final String SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID = "creditMemoTemplateId";
@SerializedName(SERIALIZED_NAME_CREDIT_MEMO_TEMPLATE_ID)
private String creditMemoTemplateId;
public static final String SERIALIZED_NAME_CRM_ID = "crmId";
@SerializedName(SERIALIZED_NAME_CRM_ID)
private String crmId;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID = "debitMemoTemplateId";
@SerializedName(SERIALIZED_NAME_DEBIT_MEMO_TEMPLATE_ID)
private String debitMemoTemplateId;
public static final String SERIALIZED_NAME_DOCUMENT_DATE = "documentDate";
@SerializedName(SERIALIZED_NAME_DOCUMENT_DATE)
private LocalDate documentDate;
public static final String SERIALIZED_NAME_HPM_CREDIT_CARD_PAYMENT_METHOD_ID = "hpmCreditCardPaymentMethodId";
@SerializedName(SERIALIZED_NAME_HPM_CREDIT_CARD_PAYMENT_METHOD_ID)
private String hpmCreditCardPaymentMethodId;
public static final String SERIALIZED_NAME_INVOICE = "invoice";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE)
private Boolean invoice;
public static final String SERIALIZED_NAME_INVOICE_COLLECT = "invoiceCollect";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE_COLLECT)
private Boolean invoiceCollect;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL = "invoiceDeliveryPrefsEmail";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_EMAIL)
private Boolean invoiceDeliveryPrefsEmail = false;
public static final String SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT = "invoiceDeliveryPrefsPrint";
@SerializedName(SERIALIZED_NAME_INVOICE_DELIVERY_PREFS_PRINT)
private Boolean invoiceDeliveryPrefsPrint = false;
public static final String SERIALIZED_NAME_INVOICE_TARGET_DATE = "invoiceTargetDate";
@Deprecated
@SerializedName(SERIALIZED_NAME_INVOICE_TARGET_DATE)
private LocalDate invoiceTargetDate;
public static final String SERIALIZED_NAME_INVOICE_TEMPLATE_ID = "invoiceTemplateId";
@SerializedName(SERIALIZED_NAME_INVOICE_TEMPLATE_ID)
private String invoiceTemplateId;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_NOTES = "notes";
@SerializedName(SERIALIZED_NAME_NOTES)
private String notes;
public static final String SERIALIZED_NAME_PARENT_ID = "parentId";
@SerializedName(SERIALIZED_NAME_PARENT_ID)
private String parentId;
public static final String SERIALIZED_NAME_PARTNER_ACCOUNT = "partnerAccount";
@SerializedName(SERIALIZED_NAME_PARTNER_ACCOUNT)
private Boolean partnerAccount;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY = "paymentGateway";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY)
private String paymentGateway;
public static final String SERIALIZED_NAME_PAYMENT_METHOD = "paymentMethod";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD)
private CreateAccountPaymentMethod paymentMethod;
public static final String SERIALIZED_NAME_PAYMENT_TERM = "paymentTerm";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERM)
private String paymentTerm;
public static final String SERIALIZED_NAME_PROFILE_NUMBER = "profileNumber";
@SerializedName(SERIALIZED_NAME_PROFILE_NUMBER)
private String profileNumber;
public static final String SERIALIZED_NAME_ROLL_UP_USAGE = "rollUpUsage";
@SerializedName(SERIALIZED_NAME_ROLL_UP_USAGE)
private Boolean rollUpUsage;
public static final String SERIALIZED_NAME_RUN_BILLING = "runBilling";
@SerializedName(SERIALIZED_NAME_RUN_BILLING)
private Boolean runBilling;
public static final String SERIALIZED_NAME_SALES_REP = "salesRep";
@SerializedName(SERIALIZED_NAME_SALES_REP)
private String salesRep;
public static final String SERIALIZED_NAME_SEQUENCE_SET_ID = "sequenceSetId";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_ID)
private String sequenceSetId;
public static final String SERIALIZED_NAME_SHIP_TO_CONTACT = "shipToContact";
@SerializedName(SERIALIZED_NAME_SHIP_TO_CONTACT)
private CreateAccountContact shipToContact;
public static final String SERIALIZED_NAME_SHIP_TO_SAME_AS_BILL_TO = "shipToSameAsBillTo";
@SerializedName(SERIALIZED_NAME_SHIP_TO_SAME_AS_BILL_TO)
private Boolean shipToSameAsBillTo;
public static final String SERIALIZED_NAME_SOLD_TO_CONTACT = "soldToContact";
@SerializedName(SERIALIZED_NAME_SOLD_TO_CONTACT)
private CreateAccountContact soldToContact;
public static final String SERIALIZED_NAME_SOLD_TO_SAME_AS_BILL_TO = "soldToSameAsBillTo";
@SerializedName(SERIALIZED_NAME_SOLD_TO_SAME_AS_BILL_TO)
private Boolean soldToSameAsBillTo;
public static final String SERIALIZED_NAME_SUBSCRIPTION = "subscription";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION)
private CreateAccountSubscription subscription;
public static final String SERIALIZED_NAME_TAGGING = "tagging";
@SerializedName(SERIALIZED_NAME_TAGGING)
private String tagging;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_TAX_INFO = "taxInfo";
@SerializedName(SERIALIZED_NAME_TAX_INFO)
private TaxInfo taxInfo;
public static final String SERIALIZED_NAME_COMMUNICATION_PROFILE_NAME = "communicationProfileName";
@SerializedName(SERIALIZED_NAME_COMMUNICATION_PROFILE_NAME)
private String communicationProfileName;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER = "paymentGatewayNumber";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_NUMBER)
private String paymentGatewayNumber;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_NAME = "paymentGatewayName";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_NAME)
private String paymentGatewayName;
public static final String SERIALIZED_NAME_ORGANIZATION_ID = "organizationId";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_ID)
private String organizationId;
public static final String SERIALIZED_NAME_ORGANIZATION_NAME = "organizationName";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_NAME)
private String organizationName;
public static final String SERIALIZED_NAME_GATEWAY_ID = "gatewayId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ID)
private String gatewayId;
public static final String SERIALIZED_NAME_PAYMENT_METHOD_ID = "paymentMethodId";
@SerializedName(SERIALIZED_NAME_PAYMENT_METHOD_ID)
private String paymentMethodId;
public static final String SERIALIZED_NAME_PARENT_ACCOUNT_NUMBER = "parentAccountNumber";
@SerializedName(SERIALIZED_NAME_PARENT_ACCOUNT_NUMBER)
private String parentAccountNumber;
public static final String SERIALIZED_NAME_SUMMARY_STATEMENT_TEMPLATE_ID = "summaryStatementTemplateId";
@SerializedName(SERIALIZED_NAME_SUMMARY_STATEMENT_TEMPLATE_ID)
private String summaryStatementTemplateId;
public static final String SERIALIZED_NAME_SEQUENCE_SET_NAME = "sequenceSetName";
@SerializedName(SERIALIZED_NAME_SEQUENCE_SET_NAME)
private String sequenceSetName;
public static final String SERIALIZED_NAME_EINVOICE_PROFILE = "einvoiceProfile";
@SerializedName(SERIALIZED_NAME_EINVOICE_PROFILE)
private AccountEInvoiceProfile einvoiceProfile;
public static final String SERIALIZED_NAME_ORGANIZATION_LABEL = "organizationLabel";
@SerializedName(SERIALIZED_NAME_ORGANIZATION_LABEL)
private String organizationLabel;
public CreateAccountRequest() {
}
public CreateAccountRequest classNS(String classNS) {
this.classNS = classNS;
return this;
}
/**
* Value of the Class field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return classNS
*/
@javax.annotation.Nullable
public String getClassNS() {
return classNS;
}
public void setClassNS(String classNS) {
this.classNS = classNS;
}
public CreateAccountRequest customerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
return this;
}
/**
* Get customerTypeNS
* @return customerTypeNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsCustomerTypeNS getCustomerTypeNS() {
return customerTypeNS;
}
public void setCustomerTypeNS(AccountObjectNSFieldsCustomerTypeNS customerTypeNS) {
this.customerTypeNS = customerTypeNS;
}
public CreateAccountRequest departmentNS(String departmentNS) {
this.departmentNS = departmentNS;
return this;
}
/**
* Value of the Department field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return departmentNS
*/
@javax.annotation.Nullable
public String getDepartmentNS() {
return departmentNS;
}
public void setDepartmentNS(String departmentNS) {
this.departmentNS = departmentNS;
}
public CreateAccountRequest integrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
return this;
}
/**
* ID of the corresponding object in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationIdNS
*/
@javax.annotation.Nullable
public String getIntegrationIdNS() {
return integrationIdNS;
}
public void setIntegrationIdNS(String integrationIdNS) {
this.integrationIdNS = integrationIdNS;
}
public CreateAccountRequest integrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
return this;
}
/**
* Status of the account's synchronization with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return integrationStatusNS
*/
@javax.annotation.Nullable
public String getIntegrationStatusNS() {
return integrationStatusNS;
}
public void setIntegrationStatusNS(String integrationStatusNS) {
this.integrationStatusNS = integrationStatusNS;
}
public CreateAccountRequest locationNS(String locationNS) {
this.locationNS = locationNS;
return this;
}
/**
* Value of the Location field for the corresponding customer account in NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return locationNS
*/
@javax.annotation.Nullable
public String getLocationNS() {
return locationNS;
}
public void setLocationNS(String locationNS) {
this.locationNS = locationNS;
}
public CreateAccountRequest subsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
return this;
}
/**
* Value of the Subsidiary field for the corresponding customer account in NetSuite. The Subsidiary field is required if you use NetSuite OneWorld. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return subsidiaryNS
*/
@javax.annotation.Nullable
public String getSubsidiaryNS() {
return subsidiaryNS;
}
public void setSubsidiaryNS(String subsidiaryNS) {
this.subsidiaryNS = subsidiaryNS;
}
public CreateAccountRequest syncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
return this;
}
/**
* Date when the account was sychronized with NetSuite. Only available if you have installed the [Zuora Connector for NetSuite](https://www.zuora.com/connect/app/?appId=265).
* @return syncDateNS
*/
@javax.annotation.Nullable
public String getSyncDateNS() {
return syncDateNS;
}
public void setSyncDateNS(String syncDateNS) {
this.syncDateNS = syncDateNS;
}
public CreateAccountRequest synctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
return this;
}
/**
* Get synctoNetSuiteNS
* @return synctoNetSuiteNS
*/
@javax.annotation.Nullable
public AccountObjectNSFieldsSynctoNetSuiteNS getSynctoNetSuiteNS() {
return synctoNetSuiteNS;
}
public void setSynctoNetSuiteNS(AccountObjectNSFieldsSynctoNetSuiteNS synctoNetSuiteNS) {
this.synctoNetSuiteNS = synctoNetSuiteNS;
}
public CreateAccountRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* A unique account number, up to 50 characters that do not begin with the default account number prefix. If no account number is specified, one is generated.
* @return accountNumber
*/
@javax.annotation.Nullable
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public CreateAccountRequest additionalEmailAddresses(List additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
return this;
}
public CreateAccountRequest addAdditionalEmailAddressesItem(String additionalEmailAddressesItem) {
if (this.additionalEmailAddresses == null) {
this.additionalEmailAddresses = new ArrayList<>();
}
this.additionalEmailAddresses.add(additionalEmailAddressesItem);
return this;
}
/**
* A list of additional email addresses to receive email notifications. Use commas to separate email addresses.
* @return additionalEmailAddresses
*/
@javax.annotation.Nullable
public List getAdditionalEmailAddresses() {
return additionalEmailAddresses;
}
public void setAdditionalEmailAddresses(List additionalEmailAddresses) {
this.additionalEmailAddresses = additionalEmailAddresses;
}
public CreateAccountRequest applicationOrder(List applicationOrder) {
this.applicationOrder = applicationOrder;
return this;
}
public CreateAccountRequest addApplicationOrderItem(String applicationOrderItem) {
if (this.applicationOrder == null) {
this.applicationOrder = new ArrayList<>();
}
this.applicationOrder.add(applicationOrderItem);
return this;
}
/**
* The priority order to apply credit memos and/or unapplied payments to an invoice. Possible item values are: `CreditMemo`, `UnappliedPayment`. **Note:** - This field is valid only if the `applyCredit` field is set to `true`. - If no value is specified for this field, the default priority order is used, [\"CreditMemo\", \"UnappliedPayment\"], to apply credit memos first and then apply unapplied payments. - If only one item is specified, only the items of the spedified type are applied to invoices. For example, if the value is `[\"CreditMemo\"]`, only credit memos are used to apply to invoices.
* @return applicationOrder
*/
@javax.annotation.Nullable
public List getApplicationOrder() {
return applicationOrder;
}
public void setApplicationOrder(List applicationOrder) {
this.applicationOrder = applicationOrder;
}
public CreateAccountRequest applyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
return this;
}
/**
* Whether to automatically apply credit memos or unapplied payments, or both to an invoice. If the value is `true`, the credit memo or unapplied payment, or both will be automatically applied to the invoice. If no value is specified or the value is `false`, no action is taken. **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return applyCredit
*/
@javax.annotation.Nullable
public Boolean getApplyCredit() {
return applyCredit;
}
public void setApplyCredit(Boolean applyCredit) {
this.applyCredit = applyCredit;
}
public CreateAccountRequest applyCreditBalance(Boolean applyCreditBalance) {
this.applyCreditBalance = applyCreditBalance;
return this;
}
/**
* Applies a credit balance to an invoice. If the value is `true`, the credit balance is applied to the invoice. If the value is `false`, no action is taken. Prerequisite: `invoice` must be `true`. To view the credit balance adjustment, retrieve the details of the invoice using the Get Invoices method. **Note:** - If you are using the field `invoiceCollect` rather than the field `invoice`, the `invoiceCollect` value must be `true`. - This field is deprecated if you have the Invoice Settlement feature enabled.
* @return applyCreditBalance
*/
@javax.annotation.Nullable
public Boolean getApplyCreditBalance() {
return applyCreditBalance;
}
public void setApplyCreditBalance(Boolean applyCreditBalance) {
this.applyCreditBalance = applyCreditBalance;
}
public CreateAccountRequest autoPay(Boolean autoPay) {
this.autoPay = autoPay;
return this;
}
/**
* Whether future payments are to be automatically billed when they are due. - If this field is set to `true`, you must specify either the `creditCard` field or the `hpmCreditCardPaymentMethodId` field, but not both. - If this field is set to `false`, you can specify neither the `creditCard` field nor the `hpmCreditCardPaymentMethodId` field.
* @return autoPay
*/
@javax.annotation.Nullable
public Boolean getAutoPay() {
return autoPay;
}
public void setAutoPay(Boolean autoPay) {
this.autoPay = autoPay;
}
public CreateAccountRequest batch(String batch) {
this.batch = batch;
return this;
}
/**
* The alias name given to a batch. A string of 50 characters or less.
* @return batch
*/
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
public void setBatch(String batch) {
this.batch = batch;
}
public CreateAccountRequest billCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
return this;
}
/**
* The account's bill cycle day (BCD), when bill runs generate invoices for the account. Specify any day of the month (1-31, where 31 = end-of-month), or 0 for auto-set. Required if no subscription will be created. Optional if a subscription is created and defaults to the day-of-the-month of the subscription's `contractEffectiveDate`.
* @return billCycleDay
*/
@javax.annotation.Nullable
public Integer getBillCycleDay() {
return billCycleDay;
}
public void setBillCycleDay(Integer billCycleDay) {
this.billCycleDay = billCycleDay;
}
public CreateAccountRequest billToContact(CreateAccountContact billToContact) {
this.billToContact = billToContact;
return this;
}
/**
* Get billToContact
* @return billToContact
*/
@javax.annotation.Nonnull
public CreateAccountContact getBillToContact() {
return billToContact;
}
public void setBillToContact(CreateAccountContact billToContact) {
this.billToContact = billToContact;
}
public CreateAccountRequest collect(Boolean collect) {
this.collect = collect;
return this;
}
/**
* Collects an automatic payment for a subscription. The collection generated in this operation is only for this subscription, not for the entire customer account. If the value is `true`, the automatic payment is collected. If the value is `false`, no action is taken. Prerequisite: The `invoice` or `runBilling` field must be `true`. **Note**: This field is only available if you set the `zuora-version` request header to `196.0` or later.
* @return collect
*/
@javax.annotation.Nullable
public Boolean getCollect() {
return collect;
}
public void setCollect(Boolean collect) {
this.collect = collect;
}
public CreateAccountRequest communicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
return this;
}
/**
* The ID of the communication profile that this account is linked to. You can provide either or both of the `communicationProfileId` and `profileNumber` fields. If both are provided, the request will fail if they do not refer to the same communication profile.
* @return communicationProfileId
*/
@javax.annotation.Nullable
public String getCommunicationProfileId() {
return communicationProfileId;
}
public void setCommunicationProfileId(String communicationProfileId) {
this.communicationProfileId = communicationProfileId;
}
public CreateAccountRequest creditCard(CreateAccountCreditCard creditCard) {
this.creditCard = creditCard;
return this;
}
/**
* Get creditCard
* @return creditCard
*/
@javax.annotation.Nullable
public CreateAccountCreditCard getCreditCard() {
return creditCard;
}
public void setCreditCard(CreateAccountCreditCard creditCard) {
this.creditCard = creditCard;
}
public CreateAccountRequest creditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
return this;
}
/**
* A code identifying the reason for the credit memo transaction that is generated by the request. The value must be an existing reason code. If you do not pass the field or pass the field with empty value, Zuora uses the default reason code.
* @return creditMemoReasonCode
*/
@javax.annotation.Nullable
public String getCreditMemoReasonCode() {
return creditMemoReasonCode;
}
public void setCreditMemoReasonCode(String creditMemoReasonCode) {
this.creditMemoReasonCode = creditMemoReasonCode;
}
public CreateAccountRequest creditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
return this;
}
/**
* **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information. The unique ID of the credit memo template, configured in **Billing Settings** > **Manage Billing Document Configuration** through the Zuora UI. For example, 2c92c08a6246fdf101626b1b3fe0144b.
* @return creditMemoTemplateId
*/
@javax.annotation.Nullable
public String getCreditMemoTemplateId() {
return creditMemoTemplateId;
}
public void setCreditMemoTemplateId(String creditMemoTemplateId) {
this.creditMemoTemplateId = creditMemoTemplateId;
}
public CreateAccountRequest crmId(String crmId) {
this.crmId = crmId;
return this;
}
/**
* CRM account ID for the account, up to 100 characters.
* @return crmId
*/
@javax.annotation.Nullable
public String getCrmId() {
return crmId;
}
public void setCrmId(String crmId) {
this.crmId = crmId;
}
public CreateAccountRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* A currency as defined in Billing Settings in the Zuora UI. For payment method authorization, if the `paymentMethod` > `currencyCode` field is specified, `currencyCode` is used. Otherwise, this `currency` field is used for payment method authorization. If no currency is specified for the account, the default currency of the account is then used.
* @return currency
*/
@javax.annotation.Nonnull
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CreateAccountRequest debitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
return this;
}
/**
* **Note:** This field is only available if you have [Invoice Settlement](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement) enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information. The unique ID of the debit memo template, configured in **Billing Settings** > **Manage Billing Document Configuration** through the Zuora UI. For example, 2c92c08d62470a8501626b19d24f19e2.
* @return debitMemoTemplateId
*/
@javax.annotation.Nullable
public String getDebitMemoTemplateId() {
return debitMemoTemplateId;
}
public void setDebitMemoTemplateId(String debitMemoTemplateId) {
this.debitMemoTemplateId = debitMemoTemplateId;
}
public CreateAccountRequest documentDate(LocalDate documentDate) {
this.documentDate = documentDate;
return this;
}
/**
* The date of the billing document, in `yyyy-mm-dd` format. It represents the invoice date for invoices, credit memo date for credit memos, and debit memo date for debit memos. - If this field is specified, the specified date is used as the billing document date. - If this field is not specified, the date specified in the `targetDate` is used as the billing document date.
* @return documentDate
*/
@javax.annotation.Nullable
public LocalDate getDocumentDate() {
return documentDate;
}
public void setDocumentDate(LocalDate documentDate) {
this.documentDate = documentDate;
}
public CreateAccountRequest hpmCreditCardPaymentMethodId(String hpmCreditCardPaymentMethodId) {
this.hpmCreditCardPaymentMethodId = hpmCreditCardPaymentMethodId;
return this;
}
/**
* The ID of the payment method associated with this account. The payment method specified for this field will be set as the default payment method of the account. If the `autoPay` field is set to `true`, you must provide the credit card payment method ID for either this field or the `creditCard` field, but not both. For the Credit Card Reference Transaction payment method, you can specify the payment method ID in this field or use the `paymentMethod` field to create a CC Reference Transaction payment method for an account.
* @return hpmCreditCardPaymentMethodId
*/
@javax.annotation.Nullable
public String getHpmCreditCardPaymentMethodId() {
return hpmCreditCardPaymentMethodId;
}
public void setHpmCreditCardPaymentMethodId(String hpmCreditCardPaymentMethodId) {
this.hpmCreditCardPaymentMethodId = hpmCreditCardPaymentMethodId;
}
@Deprecated
public CreateAccountRequest invoice(Boolean invoice) {
this.invoice = invoice;
return this;
}
/**
* **Note:** This field has been replaced by the `runBilling` field. The `invoice` field is only available for backward compatibility. Creates an invoice for a subscription. The invoice generated in this operation is only for this subscription, not for the entire customer account. If the value is `true`, an invoice is created. If the value is `false`, no action is taken. **Note**: This field is only available if you set the `zuora-version` request header to `196.0` or `207.0`.
* @return invoice
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public Boolean getInvoice() {
return invoice;
}
@Deprecated
public void setInvoice(Boolean invoice) {
this.invoice = invoice;
}
@Deprecated
public CreateAccountRequest invoiceCollect(Boolean invoiceCollect) {
this.invoiceCollect = invoiceCollect;
return this;
}
/**
* This field has been replaced by the `invoice` field and the `collect` field. `invoiceCollect` is available only for backward compatibility. If this field is set to `true`, and a subscription is created, an invoice is generated at account creation time and payment is immediately collected using the account's default payment method. This field is only available if you set the `zuora-version` request header to `186.0`, `187.0`, `188.0`, or `189.0`. The default field value is `true`.
* @return invoiceCollect
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public Boolean getInvoiceCollect() {
return invoiceCollect;
}
@Deprecated
public void setInvoiceCollect(Boolean invoiceCollect) {
this.invoiceCollect = invoiceCollect;
}
public CreateAccountRequest invoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
return this;
}
/**
* Whether the customer wants to receive invoices through email.
* @return invoiceDeliveryPrefsEmail
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsEmail() {
return invoiceDeliveryPrefsEmail;
}
public void setInvoiceDeliveryPrefsEmail(Boolean invoiceDeliveryPrefsEmail) {
this.invoiceDeliveryPrefsEmail = invoiceDeliveryPrefsEmail;
}
public CreateAccountRequest invoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
return this;
}
/**
* Whether the customer wants to receive printed invoices, such as through postal mail.
* @return invoiceDeliveryPrefsPrint
*/
@javax.annotation.Nullable
public Boolean getInvoiceDeliveryPrefsPrint() {
return invoiceDeliveryPrefsPrint;
}
public void setInvoiceDeliveryPrefsPrint(Boolean invoiceDeliveryPrefsPrint) {
this.invoiceDeliveryPrefsPrint = invoiceDeliveryPrefsPrint;
}
@Deprecated
public CreateAccountRequest invoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
return this;
}
/**
* **Note:** This field has been replaced by the `targetDate` field. The `invoiceTargetDate` field is only available for backward compatibility. Date through which to calculate charges if an invoice is generated, as yyyy-mm-dd. Default is current date. This field is in REST API minor version control. To use this field in the method, you can set the `zuora-version` parameter to the minor version number in the request header. Supported minor versions are `207.0` and earlier.
* @return invoiceTargetDate
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public LocalDate getInvoiceTargetDate() {
return invoiceTargetDate;
}
@Deprecated
public void setInvoiceTargetDate(LocalDate invoiceTargetDate) {
this.invoiceTargetDate = invoiceTargetDate;
}
public CreateAccountRequest invoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
return this;
}
/**
* Invoice template ID, configured in Billing Settings in the Zuora UI.
* @return invoiceTemplateId
*/
@javax.annotation.Nullable
public String getInvoiceTemplateId() {
return invoiceTemplateId;
}
public void setInvoiceTemplateId(String invoiceTemplateId) {
this.invoiceTemplateId = invoiceTemplateId;
}
public CreateAccountRequest name(String name) {
this.name = name;
return this;
}
/**
* Account name, up to 255 characters.
* @return name
*/
@javax.annotation.Nonnull
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public CreateAccountRequest notes(String notes) {
this.notes = notes;
return this;
}
/**
* A string of up to 65,535 characters.
* @return notes
*/
@javax.annotation.Nullable
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public CreateAccountRequest parentId(String parentId) {
this.parentId = parentId;
return this;
}
/**
* Identifier of the parent customer account for this Account object. The length is 32 characters. Use this field if you have <a href=\"https://knowledgecenter.zuora.com/Billing/Subscriptions/Customer_Accounts/A_Customer_Account_Introduction#Customer_Hierarchy\" target=\"_blank\">Customer Hierarchy</a> enabled.
* @return parentId
*/
@javax.annotation.Nullable
public String getParentId() {
return parentId;
}
public void setParentId(String parentId) {
this.parentId = parentId;
}
public CreateAccountRequest partnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
return this;
}
/**
* Whether the customer account is a partner, distributor, or reseller. You can set this field to `true` if you have business with distributors or resellers, or operating in B2B model to manage numerous subscriptions through concurrent API requests. After this field is set to `true`, the calculation of account metrics is performed asynchronously during operations such as subscription creation, order changes, invoice generation, and payments. **Note**: This field is available only if you have the <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Manage_customer_accounts/AAA_Overview_of_customer_accounts/Reseller_Account\" target=\"_blank\">Reseller Account</a> feature enabled.
* @return partnerAccount
*/
@javax.annotation.Nullable
public Boolean getPartnerAccount() {
return partnerAccount;
}
public void setPartnerAccount(Boolean partnerAccount) {
this.partnerAccount = partnerAccount;
}
public CreateAccountRequest paymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
return this;
}
/**
* The name of the payment gateway instance. If null or left unassigned, the Account will use the Default Gateway.
* @return paymentGateway
*/
@javax.annotation.Nullable
public String getPaymentGateway() {
return paymentGateway;
}
public void setPaymentGateway(String paymentGateway) {
this.paymentGateway = paymentGateway;
}
public CreateAccountRequest paymentMethod(CreateAccountPaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* Get paymentMethod
* @return paymentMethod
*/
@javax.annotation.Nullable
public CreateAccountPaymentMethod getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(CreateAccountPaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public CreateAccountRequest paymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Payment terms for this account. Possible values are: `Due Upon Receipt`, `Net 30`, `Net 60`, `Net 90`. **Note**: If you want to specify a payment term when creating a new account, you must set a value in this field. If you do not set a value in this field, Zuora will use `Due Upon Receipt` as the value instead of the default value set in **Billing Settings** > **Payment Terms** from Zuora UI.
* @return paymentTerm
*/
@javax.annotation.Nullable
public String getPaymentTerm() {
return paymentTerm;
}
public void setPaymentTerm(String paymentTerm) {
this.paymentTerm = paymentTerm;
}
public CreateAccountRequest profileNumber(String profileNumber) {
this.profileNumber = profileNumber;
return this;
}
/**
* The number of the communication profile that this account is linked to. You can provide either or both of the `communicationProfileId` and `profileNumber` fields. If both are provided, the request will fail if they do not refer to the same communication profile.
* @return profileNumber
*/
@javax.annotation.Nullable
public String getProfileNumber() {
return profileNumber;
}
public void setProfileNumber(String profileNumber) {
this.profileNumber = profileNumber;
}
public CreateAccountRequest rollUpUsage(Boolean rollUpUsage) {
this.rollUpUsage = rollUpUsage;
return this;
}
/**
* Whether roll up the usage of the account to its parent account
* @return rollUpUsage
*/
@javax.annotation.Nullable
public Boolean getRollUpUsage() {
return rollUpUsage;
}
public void setRollUpUsage(Boolean rollUpUsage) {
this.rollUpUsage = rollUpUsage;
}
public CreateAccountRequest runBilling(Boolean runBilling) {
this.runBilling = runBilling;
return this;
}
/**
* Creates an invoice for a subscription. If you have the Invoice Settlement feature enabled, a credit memo might also be created based on the [invoice and credit memo generation rule](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/B_Credit_and_Debit_Memos/Rules_for_generating_invoices_and_credit_memos). The billing documents generated in this operation is only for this subscription, not for the entire customer account. Possible values: - `true`: An invoice is created. If you have the Invoice Settlement feature enabled, a credit memo might also be created. - `false`: No invoice is created. **Note:** This field is in Zuora REST API version control. Supported minor versions are `211.0` or later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return runBilling
*/
@javax.annotation.Nullable
public Boolean getRunBilling() {
return runBilling;
}
public void setRunBilling(Boolean runBilling) {
this.runBilling = runBilling;
}
public CreateAccountRequest salesRep(String salesRep) {
this.salesRep = salesRep;
return this;
}
/**
* The name of the sales representative associated with this account, if applicable. Maximum of 50 characters.
* @return salesRep
*/
@javax.annotation.Nullable
public String getSalesRep() {
return salesRep;
}
public void setSalesRep(String salesRep) {
this.salesRep = salesRep;
}
public CreateAccountRequest sequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
return this;
}
/**
* The ID of the billing document sequence set to assign to the customer account. The billing documents to generate for this account will adopt the prefix and starting document number configured in the sequence set. If a customer account has no assigned billing document sequence set, billing documents generated for this account adopt the prefix and starting document number from the default sequence set.
* @return sequenceSetId
*/
@javax.annotation.Nullable
public String getSequenceSetId() {
return sequenceSetId;
}
public void setSequenceSetId(String sequenceSetId) {
this.sequenceSetId = sequenceSetId;
}
public CreateAccountRequest shipToContact(CreateAccountContact shipToContact) {
this.shipToContact = shipToContact;
return this;
}
/**
* Get shipToContact
* @return shipToContact
*/
@javax.annotation.Nullable
public CreateAccountContact getShipToContact() {
return shipToContact;
}
public void setShipToContact(CreateAccountContact shipToContact) {
this.shipToContact = shipToContact;
}
public CreateAccountRequest shipToSameAsBillTo(Boolean shipToSameAsBillTo) {
this.shipToSameAsBillTo = shipToSameAsBillTo;
return this;
}
/**
* Whether the ship-to contact and bill-to contact are the same entity. The created account has the same bill-to contact and ship-to contact entity only when all the following conditions are met in the request body: - This field is set to `true`. - A bill-to contact is specified. - No ship-to contact is specified.
* @return shipToSameAsBillTo
*/
@javax.annotation.Nullable
public Boolean getShipToSameAsBillTo() {
return shipToSameAsBillTo;
}
public void setShipToSameAsBillTo(Boolean shipToSameAsBillTo) {
this.shipToSameAsBillTo = shipToSameAsBillTo;
}
public CreateAccountRequest soldToContact(CreateAccountContact soldToContact) {
this.soldToContact = soldToContact;
return this;
}
/**
* Get soldToContact
* @return soldToContact
*/
@javax.annotation.Nullable
public CreateAccountContact getSoldToContact() {
return soldToContact;
}
public void setSoldToContact(CreateAccountContact soldToContact) {
this.soldToContact = soldToContact;
}
public CreateAccountRequest soldToSameAsBillTo(Boolean soldToSameAsBillTo) {
this.soldToSameAsBillTo = soldToSameAsBillTo;
return this;
}
/**
* Whether the sold-to contact and bill-to contact are the same entity. The created account has the same bill-to contact and sold-to contact entity only when all the following conditions are met in the request body: - This field is set to `true`. - A bill-to contact is specified. - No sold-to contact is specified.
* @return soldToSameAsBillTo
*/
@javax.annotation.Nullable
public Boolean getSoldToSameAsBillTo() {
return soldToSameAsBillTo;
}
public void setSoldToSameAsBillTo(Boolean soldToSameAsBillTo) {
this.soldToSameAsBillTo = soldToSameAsBillTo;
}
public CreateAccountRequest subscription(CreateAccountSubscription subscription) {
this.subscription = subscription;
return this;
}
/**
* Get subscription
* @return subscription
*/
@javax.annotation.Nullable
public CreateAccountSubscription getSubscription() {
return subscription;
}
public void setSubscription(CreateAccountSubscription subscription) {
this.subscription = subscription;
}
public CreateAccountRequest tagging(String tagging) {
this.tagging = tagging;
return this;
}
/**
*
* @return tagging
*/
@javax.annotation.Nullable
public String getTagging() {
return tagging;
}
public void setTagging(String tagging) {
this.tagging = tagging;
}
public CreateAccountRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* Date through which to calculate charges if an invoice or a credit memo is generated, as yyyy-mm-dd. Default is current date. **Note:** The credit memo is only available only if you have the Invoice Settlement feature enabled. This field is in Zuora REST API version control. Supported minor versions are `211.0` and later. To use this field in the method, you must set the `zuora-version` parameter to the minor version number in the request header.
* @return targetDate
*/
@javax.annotation.Nullable
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public CreateAccountRequest taxInfo(TaxInfo taxInfo) {
this.taxInfo = taxInfo;
return this;
}
/**
* Get taxInfo
* @return taxInfo
*/
@javax.annotation.Nullable
public TaxInfo getTaxInfo() {
return taxInfo;
}
public void setTaxInfo(TaxInfo taxInfo) {
this.taxInfo = taxInfo;
}
public CreateAccountRequest communicationProfileName(String communicationProfileName) {
this.communicationProfileName = communicationProfileName;
return this;
}
/**
* communicationProfileName
* @return communicationProfileName
*/
@javax.annotation.Nullable
public String getCommunicationProfileName() {
return communicationProfileName;
}
public void setCommunicationProfileName(String communicationProfileName) {
this.communicationProfileName = communicationProfileName;
}
public CreateAccountRequest paymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
return this;
}
/**
* paymentGatewayNumber
* @return paymentGatewayNumber
*/
@javax.annotation.Nullable
public String getPaymentGatewayNumber() {
return paymentGatewayNumber;
}
public void setPaymentGatewayNumber(String paymentGatewayNumber) {
this.paymentGatewayNumber = paymentGatewayNumber;
}
public CreateAccountRequest paymentGatewayName(String paymentGatewayName) {
this.paymentGatewayName = paymentGatewayName;
return this;
}
/**
* paymentGatewayName
* @return paymentGatewayName
*/
@javax.annotation.Nullable
public String getPaymentGatewayName() {
return paymentGatewayName;
}
public void setPaymentGatewayName(String paymentGatewayName) {
this.paymentGatewayName = paymentGatewayName;
}
public CreateAccountRequest organizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
/**
* organizationId
* @return organizationId
*/
@javax.annotation.Nullable
public String getOrganizationId() {
return organizationId;
}
public void setOrganizationId(String organizationId) {
this.organizationId = organizationId;
}
public CreateAccountRequest organizationName(String organizationName) {
this.organizationName = organizationName;
return this;
}
/**
* organizationName
* @return organizationName
*/
@javax.annotation.Nullable
public String getOrganizationName() {
return organizationName;
}
public void setOrganizationName(String organizationName) {
this.organizationName = organizationName;
}
public CreateAccountRequest gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* gatewayId
* @return gatewayId
*/
@javax.annotation.Nullable
public String getGatewayId() {
return gatewayId;
}
public void setGatewayId(String gatewayId) {
this.gatewayId = gatewayId;
}
public CreateAccountRequest paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
return this;
}
/**
* paymentMethodId
* @return paymentMethodId
*/
@javax.annotation.Nullable
public String getPaymentMethodId() {
return paymentMethodId;
}
public void setPaymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
}
public CreateAccountRequest parentAccountNumber(String parentAccountNumber) {
this.parentAccountNumber = parentAccountNumber;
return this;
}
/**
* parentAccountNumber
* @return parentAccountNumber
*/
@javax.annotation.Nullable
public String getParentAccountNumber() {
return parentAccountNumber;
}
public void setParentAccountNumber(String parentAccountNumber) {
this.parentAccountNumber = parentAccountNumber;
}
public CreateAccountRequest summaryStatementTemplateId(String summaryStatementTemplateId) {
this.summaryStatementTemplateId = summaryStatementTemplateId;
return this;
}
/**
* summaryStatementTemplateId
* @return summaryStatementTemplateId
*/
@javax.annotation.Nullable
public String getSummaryStatementTemplateId() {
return summaryStatementTemplateId;
}
public void setSummaryStatementTemplateId(String summaryStatementTemplateId) {
this.summaryStatementTemplateId = summaryStatementTemplateId;
}
public CreateAccountRequest sequenceSetName(String sequenceSetName) {
this.sequenceSetName = sequenceSetName;
return this;
}
/**
* sequenceSetName
* @return sequenceSetName
*/
@javax.annotation.Nullable
public String getSequenceSetName() {
return sequenceSetName;
}
public void setSequenceSetName(String sequenceSetName) {
this.sequenceSetName = sequenceSetName;
}
public CreateAccountRequest einvoiceProfile(AccountEInvoiceProfile einvoiceProfile) {
this.einvoiceProfile = einvoiceProfile;
return this;
}
/**
* Get einvoiceProfile
* @return einvoiceProfile
*/
@javax.annotation.Nullable
public AccountEInvoiceProfile getEinvoiceProfile() {
return einvoiceProfile;
}
public void setEinvoiceProfile(AccountEInvoiceProfile einvoiceProfile) {
this.einvoiceProfile = einvoiceProfile;
}
public CreateAccountRequest organizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
return this;
}
/**
* Get organizationLabel
* @return organizationLabel
*/
@javax.annotation.Nullable
public String getOrganizationLabel() {
return organizationLabel;
}
public void setOrganizationLabel(String organizationLabel) {
this.organizationLabel = organizationLabel;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateAccountRequest instance itself
*/
public CreateAccountRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateAccountRequest createAccountRequest = (CreateAccountRequest) o;
return Objects.equals(this.classNS, createAccountRequest.classNS) &&
Objects.equals(this.customerTypeNS, createAccountRequest.customerTypeNS) &&
Objects.equals(this.departmentNS, createAccountRequest.departmentNS) &&
Objects.equals(this.integrationIdNS, createAccountRequest.integrationIdNS) &&
Objects.equals(this.integrationStatusNS, createAccountRequest.integrationStatusNS) &&
Objects.equals(this.locationNS, createAccountRequest.locationNS) &&
Objects.equals(this.subsidiaryNS, createAccountRequest.subsidiaryNS) &&
Objects.equals(this.syncDateNS, createAccountRequest.syncDateNS) &&
Objects.equals(this.synctoNetSuiteNS, createAccountRequest.synctoNetSuiteNS) &&
Objects.equals(this.accountNumber, createAccountRequest.accountNumber) &&
Objects.equals(this.additionalEmailAddresses, createAccountRequest.additionalEmailAddresses) &&
Objects.equals(this.applicationOrder, createAccountRequest.applicationOrder) &&
Objects.equals(this.applyCredit, createAccountRequest.applyCredit) &&
Objects.equals(this.applyCreditBalance, createAccountRequest.applyCreditBalance) &&
Objects.equals(this.autoPay, createAccountRequest.autoPay) &&
Objects.equals(this.batch, createAccountRequest.batch) &&
Objects.equals(this.billCycleDay, createAccountRequest.billCycleDay) &&
Objects.equals(this.billToContact, createAccountRequest.billToContact) &&
Objects.equals(this.collect, createAccountRequest.collect) &&
Objects.equals(this.communicationProfileId, createAccountRequest.communicationProfileId) &&
Objects.equals(this.creditCard, createAccountRequest.creditCard) &&
Objects.equals(this.creditMemoReasonCode, createAccountRequest.creditMemoReasonCode) &&
Objects.equals(this.creditMemoTemplateId, createAccountRequest.creditMemoTemplateId) &&
Objects.equals(this.crmId, createAccountRequest.crmId) &&
Objects.equals(this.currency, createAccountRequest.currency) &&
Objects.equals(this.debitMemoTemplateId, createAccountRequest.debitMemoTemplateId) &&
Objects.equals(this.documentDate, createAccountRequest.documentDate) &&
Objects.equals(this.hpmCreditCardPaymentMethodId, createAccountRequest.hpmCreditCardPaymentMethodId) &&
Objects.equals(this.invoice, createAccountRequest.invoice) &&
Objects.equals(this.invoiceCollect, createAccountRequest.invoiceCollect) &&
Objects.equals(this.invoiceDeliveryPrefsEmail, createAccountRequest.invoiceDeliveryPrefsEmail) &&
Objects.equals(this.invoiceDeliveryPrefsPrint, createAccountRequest.invoiceDeliveryPrefsPrint) &&
Objects.equals(this.invoiceTargetDate, createAccountRequest.invoiceTargetDate) &&
Objects.equals(this.invoiceTemplateId, createAccountRequest.invoiceTemplateId) &&
Objects.equals(this.name, createAccountRequest.name) &&
Objects.equals(this.notes, createAccountRequest.notes) &&
Objects.equals(this.parentId, createAccountRequest.parentId) &&
Objects.equals(this.partnerAccount, createAccountRequest.partnerAccount) &&
Objects.equals(this.paymentGateway, createAccountRequest.paymentGateway) &&
Objects.equals(this.paymentMethod, createAccountRequest.paymentMethod) &&
Objects.equals(this.paymentTerm, createAccountRequest.paymentTerm) &&
Objects.equals(this.profileNumber, createAccountRequest.profileNumber) &&
Objects.equals(this.rollUpUsage, createAccountRequest.rollUpUsage) &&
Objects.equals(this.runBilling, createAccountRequest.runBilling) &&
Objects.equals(this.salesRep, createAccountRequest.salesRep) &&
Objects.equals(this.sequenceSetId, createAccountRequest.sequenceSetId) &&
Objects.equals(this.shipToContact, createAccountRequest.shipToContact) &&
Objects.equals(this.shipToSameAsBillTo, createAccountRequest.shipToSameAsBillTo) &&
Objects.equals(this.soldToContact, createAccountRequest.soldToContact) &&
Objects.equals(this.soldToSameAsBillTo, createAccountRequest.soldToSameAsBillTo) &&
Objects.equals(this.subscription, createAccountRequest.subscription) &&
Objects.equals(this.tagging, createAccountRequest.tagging) &&
Objects.equals(this.targetDate, createAccountRequest.targetDate) &&
Objects.equals(this.taxInfo, createAccountRequest.taxInfo) &&
Objects.equals(this.communicationProfileName, createAccountRequest.communicationProfileName) &&
Objects.equals(this.paymentGatewayNumber, createAccountRequest.paymentGatewayNumber) &&
Objects.equals(this.paymentGatewayName, createAccountRequest.paymentGatewayName) &&
Objects.equals(this.organizationId, createAccountRequest.organizationId) &&
Objects.equals(this.organizationName, createAccountRequest.organizationName) &&
Objects.equals(this.gatewayId, createAccountRequest.gatewayId) &&
Objects.equals(this.paymentMethodId, createAccountRequest.paymentMethodId) &&
Objects.equals(this.parentAccountNumber, createAccountRequest.parentAccountNumber) &&
Objects.equals(this.summaryStatementTemplateId, createAccountRequest.summaryStatementTemplateId) &&
Objects.equals(this.sequenceSetName, createAccountRequest.sequenceSetName) &&
Objects.equals(this.einvoiceProfile, createAccountRequest.einvoiceProfile) &&
Objects.equals(this.organizationLabel, createAccountRequest.organizationLabel)&&
Objects.equals(this.additionalProperties, createAccountRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(classNS, customerTypeNS, departmentNS, integrationIdNS, integrationStatusNS, locationNS, subsidiaryNS, syncDateNS, synctoNetSuiteNS, accountNumber, additionalEmailAddresses, applicationOrder, applyCredit, applyCreditBalance, autoPay, batch, billCycleDay, billToContact, collect, communicationProfileId, creditCard, creditMemoReasonCode, creditMemoTemplateId, crmId, currency, debitMemoTemplateId, documentDate, hpmCreditCardPaymentMethodId, invoice, invoiceCollect, invoiceDeliveryPrefsEmail, invoiceDeliveryPrefsPrint, invoiceTargetDate, invoiceTemplateId, name, notes, parentId, partnerAccount, paymentGateway, paymentMethod, paymentTerm, profileNumber, rollUpUsage, runBilling, salesRep, sequenceSetId, shipToContact, shipToSameAsBillTo, soldToContact, soldToSameAsBillTo, subscription, tagging, targetDate, taxInfo, communicationProfileName, paymentGatewayNumber, paymentGatewayName, organizationId, organizationName, gatewayId, paymentMethodId, parentAccountNumber, summaryStatementTemplateId, sequenceSetName, einvoiceProfile, organizationLabel, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateAccountRequest {\n");
sb.append(" classNS: ").append(toIndentedString(classNS)).append("\n");
sb.append(" customerTypeNS: ").append(toIndentedString(customerTypeNS)).append("\n");
sb.append(" departmentNS: ").append(toIndentedString(departmentNS)).append("\n");
sb.append(" integrationIdNS: ").append(toIndentedString(integrationIdNS)).append("\n");
sb.append(" integrationStatusNS: ").append(toIndentedString(integrationStatusNS)).append("\n");
sb.append(" locationNS: ").append(toIndentedString(locationNS)).append("\n");
sb.append(" subsidiaryNS: ").append(toIndentedString(subsidiaryNS)).append("\n");
sb.append(" syncDateNS: ").append(toIndentedString(syncDateNS)).append("\n");
sb.append(" synctoNetSuiteNS: ").append(toIndentedString(synctoNetSuiteNS)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" additionalEmailAddresses: ").append(toIndentedString(additionalEmailAddresses)).append("\n");
sb.append(" applicationOrder: ").append(toIndentedString(applicationOrder)).append("\n");
sb.append(" applyCredit: ").append(toIndentedString(applyCredit)).append("\n");
sb.append(" applyCreditBalance: ").append(toIndentedString(applyCreditBalance)).append("\n");
sb.append(" autoPay: ").append(toIndentedString(autoPay)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" billCycleDay: ").append(toIndentedString(billCycleDay)).append("\n");
sb.append(" billToContact: ").append(toIndentedString(billToContact)).append("\n");
sb.append(" collect: ").append(toIndentedString(collect)).append("\n");
sb.append(" communicationProfileId: ").append(toIndentedString(communicationProfileId)).append("\n");
sb.append(" creditCard: ").append(toIndentedString(creditCard)).append("\n");
sb.append(" creditMemoReasonCode: ").append(toIndentedString(creditMemoReasonCode)).append("\n");
sb.append(" creditMemoTemplateId: ").append(toIndentedString(creditMemoTemplateId)).append("\n");
sb.append(" crmId: ").append(toIndentedString(crmId)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" debitMemoTemplateId: ").append(toIndentedString(debitMemoTemplateId)).append("\n");
sb.append(" documentDate: ").append(toIndentedString(documentDate)).append("\n");
sb.append(" hpmCreditCardPaymentMethodId: ").append(toIndentedString(hpmCreditCardPaymentMethodId)).append("\n");
sb.append(" invoice: ").append(toIndentedString(invoice)).append("\n");
sb.append(" invoiceCollect: ").append(toIndentedString(invoiceCollect)).append("\n");
sb.append(" invoiceDeliveryPrefsEmail: ").append(toIndentedString(invoiceDeliveryPrefsEmail)).append("\n");
sb.append(" invoiceDeliveryPrefsPrint: ").append(toIndentedString(invoiceDeliveryPrefsPrint)).append("\n");
sb.append(" invoiceTargetDate: ").append(toIndentedString(invoiceTargetDate)).append("\n");
sb.append(" invoiceTemplateId: ").append(toIndentedString(invoiceTemplateId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" parentId: ").append(toIndentedString(parentId)).append("\n");
sb.append(" partnerAccount: ").append(toIndentedString(partnerAccount)).append("\n");
sb.append(" paymentGateway: ").append(toIndentedString(paymentGateway)).append("\n");
sb.append(" paymentMethod: ").append(toIndentedString(paymentMethod)).append("\n");
sb.append(" paymentTerm: ").append(toIndentedString(paymentTerm)).append("\n");
sb.append(" profileNumber: ").append(toIndentedString(profileNumber)).append("\n");
sb.append(" rollUpUsage: ").append(toIndentedString(rollUpUsage)).append("\n");
sb.append(" runBilling: ").append(toIndentedString(runBilling)).append("\n");
sb.append(" salesRep: ").append(toIndentedString(salesRep)).append("\n");
sb.append(" sequenceSetId: ").append(toIndentedString(sequenceSetId)).append("\n");
sb.append(" shipToContact: ").append(toIndentedString(shipToContact)).append("\n");
sb.append(" shipToSameAsBillTo: ").append(toIndentedString(shipToSameAsBillTo)).append("\n");
sb.append(" soldToContact: ").append(toIndentedString(soldToContact)).append("\n");
sb.append(" soldToSameAsBillTo: ").append(toIndentedString(soldToSameAsBillTo)).append("\n");
sb.append(" subscription: ").append(toIndentedString(subscription)).append("\n");
sb.append(" tagging: ").append(toIndentedString(tagging)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" taxInfo: ").append(toIndentedString(taxInfo)).append("\n");
sb.append(" communicationProfileName: ").append(toIndentedString(communicationProfileName)).append("\n");
sb.append(" paymentGatewayNumber: ").append(toIndentedString(paymentGatewayNumber)).append("\n");
sb.append(" paymentGatewayName: ").append(toIndentedString(paymentGatewayName)).append("\n");
sb.append(" organizationId: ").append(toIndentedString(organizationId)).append("\n");
sb.append(" organizationName: ").append(toIndentedString(organizationName)).append("\n");
sb.append(" gatewayId: ").append(toIndentedString(gatewayId)).append("\n");
sb.append(" paymentMethodId: ").append(toIndentedString(paymentMethodId)).append("\n");
sb.append(" parentAccountNumber: ").append(toIndentedString(parentAccountNumber)).append("\n");
sb.append(" summaryStatementTemplateId: ").append(toIndentedString(summaryStatementTemplateId)).append("\n");
sb.append(" sequenceSetName: ").append(toIndentedString(sequenceSetName)).append("\n");
sb.append(" einvoiceProfile: ").append(toIndentedString(einvoiceProfile)).append("\n");
sb.append(" organizationLabel: ").append(toIndentedString(organizationLabel)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("Class__NS");
openapiFields.add("CustomerType__NS");
openapiFields.add("Department__NS");
openapiFields.add("IntegrationId__NS");
openapiFields.add("IntegrationStatus__NS");
openapiFields.add("Location__NS");
openapiFields.add("Subsidiary__NS");
openapiFields.add("SyncDate__NS");
openapiFields.add("SynctoNetSuite__NS");
openapiFields.add("accountNumber");
openapiFields.add("additionalEmailAddresses");
openapiFields.add("applicationOrder");
openapiFields.add("applyCredit");
openapiFields.add("applyCreditBalance");
openapiFields.add("autoPay");
openapiFields.add("batch");
openapiFields.add("billCycleDay");
openapiFields.add("billToContact");
openapiFields.add("collect");
openapiFields.add("communicationProfileId");
openapiFields.add("creditCard");
openapiFields.add("creditMemoReasonCode");
openapiFields.add("creditMemoTemplateId");
openapiFields.add("crmId");
openapiFields.add("currency");
openapiFields.add("debitMemoTemplateId");
openapiFields.add("documentDate");
openapiFields.add("hpmCreditCardPaymentMethodId");
openapiFields.add("invoice");
openapiFields.add("invoiceCollect");
openapiFields.add("invoiceDeliveryPrefsEmail");
openapiFields.add("invoiceDeliveryPrefsPrint");
openapiFields.add("invoiceTargetDate");
openapiFields.add("invoiceTemplateId");
openapiFields.add("name");
openapiFields.add("notes");
openapiFields.add("parentId");
openapiFields.add("partnerAccount");
openapiFields.add("paymentGateway");
openapiFields.add("paymentMethod");
openapiFields.add("paymentTerm");
openapiFields.add("profileNumber");
openapiFields.add("rollUpUsage");
openapiFields.add("runBilling");
openapiFields.add("salesRep");
openapiFields.add("sequenceSetId");
openapiFields.add("shipToContact");
openapiFields.add("shipToSameAsBillTo");
openapiFields.add("soldToContact");
openapiFields.add("soldToSameAsBillTo");
openapiFields.add("subscription");
openapiFields.add("tagging");
openapiFields.add("targetDate");
openapiFields.add("taxInfo");
openapiFields.add("communicationProfileName");
openapiFields.add("paymentGatewayNumber");
openapiFields.add("paymentGatewayName");
openapiFields.add("organizationId");
openapiFields.add("organizationName");
openapiFields.add("gatewayId");
openapiFields.add("paymentMethodId");
openapiFields.add("parentAccountNumber");
openapiFields.add("summaryStatementTemplateId");
openapiFields.add("sequenceSetName");
openapiFields.add("einvoiceProfile");
openapiFields.add("organizationLabel");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("billToContact");
openapiRequiredFields.add("currency");
openapiRequiredFields.add("name");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateAccountRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateAccountRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateAccountRequest is not found in the empty JSON string", CreateAccountRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateAccountRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("Class__NS") != null && !jsonObj.get("Class__NS").isJsonNull()) && !jsonObj.get("Class__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Class__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Class__NS").toString()));
}
if ((jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) && !jsonObj.get("CustomerType__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `CustomerType__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("CustomerType__NS").toString()));
}
// validate the optional field `CustomerType__NS`
if (jsonObj.get("CustomerType__NS") != null && !jsonObj.get("CustomerType__NS").isJsonNull()) {
AccountObjectNSFieldsCustomerTypeNS.validateJsonElement(jsonObj.get("CustomerType__NS"));
}
if ((jsonObj.get("Department__NS") != null && !jsonObj.get("Department__NS").isJsonNull()) && !jsonObj.get("Department__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Department__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Department__NS").toString()));
}
if ((jsonObj.get("IntegrationId__NS") != null && !jsonObj.get("IntegrationId__NS").isJsonNull()) && !jsonObj.get("IntegrationId__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationId__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationId__NS").toString()));
}
if ((jsonObj.get("IntegrationStatus__NS") != null && !jsonObj.get("IntegrationStatus__NS").isJsonNull()) && !jsonObj.get("IntegrationStatus__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `IntegrationStatus__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("IntegrationStatus__NS").toString()));
}
if ((jsonObj.get("Location__NS") != null && !jsonObj.get("Location__NS").isJsonNull()) && !jsonObj.get("Location__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Location__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Location__NS").toString()));
}
if ((jsonObj.get("Subsidiary__NS") != null && !jsonObj.get("Subsidiary__NS").isJsonNull()) && !jsonObj.get("Subsidiary__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `Subsidiary__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("Subsidiary__NS").toString()));
}
if ((jsonObj.get("SyncDate__NS") != null && !jsonObj.get("SyncDate__NS").isJsonNull()) && !jsonObj.get("SyncDate__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SyncDate__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SyncDate__NS").toString()));
}
if ((jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) && !jsonObj.get("SynctoNetSuite__NS").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `SynctoNetSuite__NS` to be a primitive type in the JSON string but got `%s`", jsonObj.get("SynctoNetSuite__NS").toString()));
}
// validate the optional field `SynctoNetSuite__NS`
if (jsonObj.get("SynctoNetSuite__NS") != null && !jsonObj.get("SynctoNetSuite__NS").isJsonNull()) {
AccountObjectNSFieldsSynctoNetSuiteNS.validateJsonElement(jsonObj.get("SynctoNetSuite__NS"));
}
if ((jsonObj.get("accountNumber") != null && !jsonObj.get("accountNumber").isJsonNull()) && !jsonObj.get("accountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `accountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("accountNumber").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("additionalEmailAddresses") != null && !jsonObj.get("additionalEmailAddresses").isJsonNull() && !jsonObj.get("additionalEmailAddresses").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `additionalEmailAddresses` to be an array in the JSON string but got `%s`", jsonObj.get("additionalEmailAddresses").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("applicationOrder") != null && !jsonObj.get("applicationOrder").isJsonNull() && !jsonObj.get("applicationOrder").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `applicationOrder` to be an array in the JSON string but got `%s`", jsonObj.get("applicationOrder").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
// validate the required field `billToContact`
CreateAccountContact.validateJsonElement(jsonObj.get("billToContact"));
if ((jsonObj.get("communicationProfileId") != null && !jsonObj.get("communicationProfileId").isJsonNull()) && !jsonObj.get("communicationProfileId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `communicationProfileId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("communicationProfileId").toString()));
}
// validate the optional field `creditCard`
if (jsonObj.get("creditCard") != null && !jsonObj.get("creditCard").isJsonNull()) {
CreateAccountCreditCard.validateJsonElement(jsonObj.get("creditCard"));
}
if ((jsonObj.get("creditMemoReasonCode") != null && !jsonObj.get("creditMemoReasonCode").isJsonNull()) && !jsonObj.get("creditMemoReasonCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoReasonCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoReasonCode").toString()));
}
if ((jsonObj.get("creditMemoTemplateId") != null && !jsonObj.get("creditMemoTemplateId").isJsonNull()) && !jsonObj.get("creditMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `creditMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("creditMemoTemplateId").toString()));
}
if ((jsonObj.get("crmId") != null && !jsonObj.get("crmId").isJsonNull()) && !jsonObj.get("crmId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `crmId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("crmId").toString()));
}
if (!jsonObj.get("currency").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `currency` to be a primitive type in the JSON string but got `%s`", jsonObj.get("currency").toString()));
}
if ((jsonObj.get("debitMemoTemplateId") != null && !jsonObj.get("debitMemoTemplateId").isJsonNull()) && !jsonObj.get("debitMemoTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `debitMemoTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("debitMemoTemplateId").toString()));
}
if ((jsonObj.get("hpmCreditCardPaymentMethodId") != null && !jsonObj.get("hpmCreditCardPaymentMethodId").isJsonNull()) && !jsonObj.get("hpmCreditCardPaymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `hpmCreditCardPaymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("hpmCreditCardPaymentMethodId").toString()));
}
if ((jsonObj.get("invoiceTemplateId") != null && !jsonObj.get("invoiceTemplateId").isJsonNull()) && !jsonObj.get("invoiceTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `invoiceTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("invoiceTemplateId").toString()));
}
if (!jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("notes") != null && !jsonObj.get("notes").isJsonNull()) && !jsonObj.get("notes").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `notes` to be a primitive type in the JSON string but got `%s`", jsonObj.get("notes").toString()));
}
if ((jsonObj.get("parentId") != null && !jsonObj.get("parentId").isJsonNull()) && !jsonObj.get("parentId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parentId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parentId").toString()));
}
if ((jsonObj.get("paymentGateway") != null && !jsonObj.get("paymentGateway").isJsonNull()) && !jsonObj.get("paymentGateway").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGateway` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGateway").toString()));
}
// validate the optional field `paymentMethod`
if (jsonObj.get("paymentMethod") != null && !jsonObj.get("paymentMethod").isJsonNull()) {
CreateAccountPaymentMethod.validateJsonElement(jsonObj.get("paymentMethod"));
}
if ((jsonObj.get("paymentTerm") != null && !jsonObj.get("paymentTerm").isJsonNull()) && !jsonObj.get("paymentTerm").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentTerm` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentTerm").toString()));
}
if ((jsonObj.get("profileNumber") != null && !jsonObj.get("profileNumber").isJsonNull()) && !jsonObj.get("profileNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `profileNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("profileNumber").toString()));
}
if ((jsonObj.get("salesRep") != null && !jsonObj.get("salesRep").isJsonNull()) && !jsonObj.get("salesRep").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `salesRep` to be a primitive type in the JSON string but got `%s`", jsonObj.get("salesRep").toString()));
}
if ((jsonObj.get("sequenceSetId") != null && !jsonObj.get("sequenceSetId").isJsonNull()) && !jsonObj.get("sequenceSetId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetId").toString()));
}
// validate the optional field `shipToContact`
if (jsonObj.get("shipToContact") != null && !jsonObj.get("shipToContact").isJsonNull()) {
CreateAccountContact.validateJsonElement(jsonObj.get("shipToContact"));
}
// validate the optional field `soldToContact`
if (jsonObj.get("soldToContact") != null && !jsonObj.get("soldToContact").isJsonNull()) {
CreateAccountContact.validateJsonElement(jsonObj.get("soldToContact"));
}
// validate the optional field `subscription`
if (jsonObj.get("subscription") != null && !jsonObj.get("subscription").isJsonNull()) {
CreateAccountSubscription.validateJsonElement(jsonObj.get("subscription"));
}
if ((jsonObj.get("tagging") != null && !jsonObj.get("tagging").isJsonNull()) && !jsonObj.get("tagging").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tagging` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tagging").toString()));
}
// validate the optional field `taxInfo`
if (jsonObj.get("taxInfo") != null && !jsonObj.get("taxInfo").isJsonNull()) {
TaxInfo.validateJsonElement(jsonObj.get("taxInfo"));
}
if ((jsonObj.get("communicationProfileName") != null && !jsonObj.get("communicationProfileName").isJsonNull()) && !jsonObj.get("communicationProfileName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `communicationProfileName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("communicationProfileName").toString()));
}
if ((jsonObj.get("paymentGatewayNumber") != null && !jsonObj.get("paymentGatewayNumber").isJsonNull()) && !jsonObj.get("paymentGatewayNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGatewayNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGatewayNumber").toString()));
}
if ((jsonObj.get("paymentGatewayName") != null && !jsonObj.get("paymentGatewayName").isJsonNull()) && !jsonObj.get("paymentGatewayName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentGatewayName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentGatewayName").toString()));
}
if ((jsonObj.get("organizationId") != null && !jsonObj.get("organizationId").isJsonNull()) && !jsonObj.get("organizationId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationId").toString()));
}
if ((jsonObj.get("organizationName") != null && !jsonObj.get("organizationName").isJsonNull()) && !jsonObj.get("organizationName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationName").toString()));
}
if ((jsonObj.get("gatewayId") != null && !jsonObj.get("gatewayId").isJsonNull()) && !jsonObj.get("gatewayId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayId").toString()));
}
if ((jsonObj.get("paymentMethodId") != null && !jsonObj.get("paymentMethodId").isJsonNull()) && !jsonObj.get("paymentMethodId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `paymentMethodId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("paymentMethodId").toString()));
}
if ((jsonObj.get("parentAccountNumber") != null && !jsonObj.get("parentAccountNumber").isJsonNull()) && !jsonObj.get("parentAccountNumber").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parentAccountNumber` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parentAccountNumber").toString()));
}
if ((jsonObj.get("summaryStatementTemplateId") != null && !jsonObj.get("summaryStatementTemplateId").isJsonNull()) && !jsonObj.get("summaryStatementTemplateId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `summaryStatementTemplateId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("summaryStatementTemplateId").toString()));
}
if ((jsonObj.get("sequenceSetName") != null && !jsonObj.get("sequenceSetName").isJsonNull()) && !jsonObj.get("sequenceSetName").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sequenceSetName` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sequenceSetName").toString()));
}
// validate the optional field `einvoiceProfile`
if (jsonObj.get("einvoiceProfile") != null && !jsonObj.get("einvoiceProfile").isJsonNull()) {
AccountEInvoiceProfile.validateJsonElement(jsonObj.get("einvoiceProfile"));
}
if ((jsonObj.get("organizationLabel") != null && !jsonObj.get("organizationLabel").isJsonNull()) && !jsonObj.get("organizationLabel").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `organizationLabel` to be a primitive type in the JSON string but got `%s`", jsonObj.get("organizationLabel").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateAccountRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateAccountRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateAccountRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateAccountRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateAccountRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateAccountRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateAccountRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateAccountRequest
* @throws IOException if the JSON string is invalid with respect to CreateAccountRequest
*/
public static CreateAccountRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateAccountRequest.class);
}
/**
* Convert an instance of CreateAccountRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy