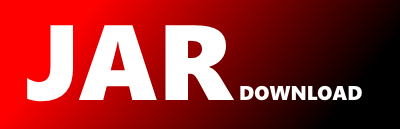
com.zuora.model.CreateAuthorizationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.CreateAuthorizationResponsePaymentGateway;
import com.zuora.model.CreateAuthorizationResponseReasons;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateAuthorizationResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateAuthorizationResponse {
public static final String SERIALIZED_NAME_GATEWAY_ORDER_ID = "gatewayOrderId";
@SerializedName(SERIALIZED_NAME_GATEWAY_ORDER_ID)
private String gatewayOrderId;
public static final String SERIALIZED_NAME_PAYMENT_GATEWAY_RESPONSE = "paymentGatewayResponse";
@SerializedName(SERIALIZED_NAME_PAYMENT_GATEWAY_RESPONSE)
private CreateAuthorizationResponsePaymentGateway paymentGatewayResponse;
public static final String SERIALIZED_NAME_PROCESS_ID = "processId";
@SerializedName(SERIALIZED_NAME_PROCESS_ID)
private String processId;
public static final String SERIALIZED_NAME_REASONS = "reasons";
@SerializedName(SERIALIZED_NAME_REASONS)
private List reasons;
public static final String SERIALIZED_NAME_REQUEST_ID = "requestId";
@SerializedName(SERIALIZED_NAME_REQUEST_ID)
private String requestId;
public static final String SERIALIZED_NAME_RESULT_CODE = "resultCode";
@SerializedName(SERIALIZED_NAME_RESULT_CODE)
private String resultCode;
public static final String SERIALIZED_NAME_RESULT_MESSAGE = "resultMessage";
@SerializedName(SERIALIZED_NAME_RESULT_MESSAGE)
private String resultMessage;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_TRANSACTION_ID = "transactionId";
@SerializedName(SERIALIZED_NAME_TRANSACTION_ID)
private String transactionId;
public CreateAuthorizationResponse() {
}
public CreateAuthorizationResponse gatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
return this;
}
/**
* The order ID for the specific gateway. The specified order ID will be used in transaction authorization. If you specify an empty value for this field, Zuora will generate an ID and you will have to associate this ID with your order ID by yourself if needed. It is recommended to specify an ID for this field.
* @return gatewayOrderId
*/
@javax.annotation.Nullable
public String getGatewayOrderId() {
return gatewayOrderId;
}
public void setGatewayOrderId(String gatewayOrderId) {
this.gatewayOrderId = gatewayOrderId;
}
public CreateAuthorizationResponse paymentGatewayResponse(CreateAuthorizationResponsePaymentGateway paymentGatewayResponse) {
this.paymentGatewayResponse = paymentGatewayResponse;
return this;
}
/**
* Get paymentGatewayResponse
* @return paymentGatewayResponse
*/
@javax.annotation.Nullable
public CreateAuthorizationResponsePaymentGateway getPaymentGatewayResponse() {
return paymentGatewayResponse;
}
public void setPaymentGatewayResponse(CreateAuthorizationResponsePaymentGateway paymentGatewayResponse) {
this.paymentGatewayResponse = paymentGatewayResponse;
}
public CreateAuthorizationResponse processId(String processId) {
this.processId = processId;
return this;
}
/**
* The ID of the running process when the exception occurs. This field is available only if the `success` field is `false`.
* @return processId
*/
@javax.annotation.Nullable
public String getProcessId() {
return processId;
}
public void setProcessId(String processId) {
this.processId = processId;
}
public CreateAuthorizationResponse reasons(List reasons) {
this.reasons = reasons;
return this;
}
public CreateAuthorizationResponse addReasonsItem(CreateAuthorizationResponseReasons reasonsItem) {
if (this.reasons == null) {
this.reasons = new ArrayList<>();
}
this.reasons.add(reasonsItem);
return this;
}
/**
* The container of the error code and message. This field is available only if the `success` field is `false`.
* @return reasons
*/
@javax.annotation.Nullable
public List getReasons() {
return reasons;
}
public void setReasons(List reasons) {
this.reasons = reasons;
}
public CreateAuthorizationResponse requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* The ID of the request. This field is available only if the `success` field is `false`
* @return requestId
*/
@javax.annotation.Nullable
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public CreateAuthorizationResponse resultCode(String resultCode) {
this.resultCode = resultCode;
return this;
}
/**
* The result code of the request. 0 indicates that the request succeeded, and the following values indicate that the request failed: - 1: The request is declined. - 7: The field format is not correct. - 10: Client connection has timed out. - 11: Host connection has timed out. - 12: Processor connection has timed out. - 13: Gateway server is busy. - 20: The card type is not supported. - 21: The merchant account information is invalid. - 22: A generic error occurred on the processor. - 40: The card type has not been set up yet. - 41: The limit for a single transaction is exceeded. - 42: Address checking failed. - 43: Card security code checking failed. - 44: Failed due to the gateway security setting. - 45: Fraud protection is declined. - 46: Address checking or card security code checking failed (for Authorize.net gateway only). - 47: The maximum amount is exceeded (for Authorize.net gateway only). - 48: The IP address is blocked by the gateway (for Authorize.net gateway only). - 49: Card security code checking failed (for Authorize.net gateway only). - 60: User authentication failed. - 61: The currency code is invalid. - 62: The transaction ID is invalid. - 63: The credit card number is invalid. - 64: The card expiration date is invalid. - 65: The transaction is duplicated. - 66: Credit transaction error. - 67: Void transaction error. - 90: A valid amount is required. - 91: The BA code is invalid. - 92: The account number is invalid. - 93: The ACH transaction is not accepted by the merchant. - 94: An error occurred for the ACH transaction. - 95: The version parameter is invalid. - 96: The transaction type is invalid. - 97: The transaction method is invalid. - 98: The bank account type is invalid. - 99: The authorization code is invalid. - 200: General transaction error. - 500: The transaction is queued for submission. - 999: Unknown error. - -1: An error occurred in gateway communication. - -2: Idempotency is not supported. - -3: Inquiry call is not supported.
* @return resultCode
*/
@javax.annotation.Nullable
public String getResultCode() {
return resultCode;
}
public void setResultCode(String resultCode) {
this.resultCode = resultCode;
}
public CreateAuthorizationResponse resultMessage(String resultMessage) {
this.resultMessage = resultMessage;
return this;
}
/**
* The corresponding request ID.
* @return resultMessage
*/
@javax.annotation.Nullable
public String getResultMessage() {
return resultMessage;
}
public void setResultMessage(String resultMessage) {
this.resultMessage = resultMessage;
}
public CreateAuthorizationResponse success(Boolean success) {
this.success = success;
return this;
}
/**
* Indicates whether the call succeeded.
* @return success
*/
@javax.annotation.Nullable
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public CreateAuthorizationResponse transactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* The ID of the transaction.
* @return transactionId
*/
@javax.annotation.Nullable
public String getTransactionId() {
return transactionId;
}
public void setTransactionId(String transactionId) {
this.transactionId = transactionId;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateAuthorizationResponse instance itself
*/
public CreateAuthorizationResponse putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateAuthorizationResponse createAuthorizationResponse = (CreateAuthorizationResponse) o;
return Objects.equals(this.gatewayOrderId, createAuthorizationResponse.gatewayOrderId) &&
Objects.equals(this.paymentGatewayResponse, createAuthorizationResponse.paymentGatewayResponse) &&
Objects.equals(this.processId, createAuthorizationResponse.processId) &&
Objects.equals(this.reasons, createAuthorizationResponse.reasons) &&
Objects.equals(this.requestId, createAuthorizationResponse.requestId) &&
Objects.equals(this.resultCode, createAuthorizationResponse.resultCode) &&
Objects.equals(this.resultMessage, createAuthorizationResponse.resultMessage) &&
Objects.equals(this.success, createAuthorizationResponse.success) &&
Objects.equals(this.transactionId, createAuthorizationResponse.transactionId)&&
Objects.equals(this.additionalProperties, createAuthorizationResponse.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(gatewayOrderId, paymentGatewayResponse, processId, reasons, requestId, resultCode, resultMessage, success, transactionId, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateAuthorizationResponse {\n");
sb.append(" gatewayOrderId: ").append(toIndentedString(gatewayOrderId)).append("\n");
sb.append(" paymentGatewayResponse: ").append(toIndentedString(paymentGatewayResponse)).append("\n");
sb.append(" processId: ").append(toIndentedString(processId)).append("\n");
sb.append(" reasons: ").append(toIndentedString(reasons)).append("\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append(" resultCode: ").append(toIndentedString(resultCode)).append("\n");
sb.append(" resultMessage: ").append(toIndentedString(resultMessage)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" transactionId: ").append(toIndentedString(transactionId)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("gatewayOrderId");
openapiFields.add("paymentGatewayResponse");
openapiFields.add("processId");
openapiFields.add("reasons");
openapiFields.add("requestId");
openapiFields.add("resultCode");
openapiFields.add("resultMessage");
openapiFields.add("success");
openapiFields.add("transactionId");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateAuthorizationResponse
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateAuthorizationResponse.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateAuthorizationResponse is not found in the empty JSON string", CreateAuthorizationResponse.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("gatewayOrderId") != null && !jsonObj.get("gatewayOrderId").isJsonNull()) && !jsonObj.get("gatewayOrderId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gatewayOrderId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gatewayOrderId").toString()));
}
// validate the optional field `paymentGatewayResponse`
if (jsonObj.get("paymentGatewayResponse") != null && !jsonObj.get("paymentGatewayResponse").isJsonNull()) {
CreateAuthorizationResponsePaymentGateway.validateJsonElement(jsonObj.get("paymentGatewayResponse"));
}
if ((jsonObj.get("processId") != null && !jsonObj.get("processId").isJsonNull()) && !jsonObj.get("processId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `processId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("processId").toString()));
}
if (jsonObj.get("reasons") != null && !jsonObj.get("reasons").isJsonNull()) {
JsonArray jsonArrayreasons = jsonObj.getAsJsonArray("reasons");
if (jsonArrayreasons != null) {
// ensure the json data is an array
if (!jsonObj.get("reasons").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `reasons` to be an array in the JSON string but got `%s`", jsonObj.get("reasons").toString()));
}
// validate the optional field `reasons` (array)
for (int i = 0; i < jsonArrayreasons.size(); i++) {
CreateAuthorizationResponseReasons.validateJsonElement(jsonArrayreasons.get(i));
};
}
}
if ((jsonObj.get("requestId") != null && !jsonObj.get("requestId").isJsonNull()) && !jsonObj.get("requestId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `requestId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("requestId").toString()));
}
if ((jsonObj.get("resultCode") != null && !jsonObj.get("resultCode").isJsonNull()) && !jsonObj.get("resultCode").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resultCode` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resultCode").toString()));
}
if ((jsonObj.get("resultMessage") != null && !jsonObj.get("resultMessage").isJsonNull()) && !jsonObj.get("resultMessage").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `resultMessage` to be a primitive type in the JSON string but got `%s`", jsonObj.get("resultMessage").toString()));
}
if ((jsonObj.get("transactionId") != null && !jsonObj.get("transactionId").isJsonNull()) && !jsonObj.get("transactionId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `transactionId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("transactionId").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateAuthorizationResponse.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateAuthorizationResponse' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateAuthorizationResponse.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateAuthorizationResponse value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateAuthorizationResponse read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateAuthorizationResponse instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateAuthorizationResponse given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateAuthorizationResponse
* @throws IOException if the JSON string is invalid with respect to CreateAuthorizationResponse
*/
public static CreateAuthorizationResponse fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateAuthorizationResponse.class);
}
/**
* Convert an instance of CreateAuthorizationResponse to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy