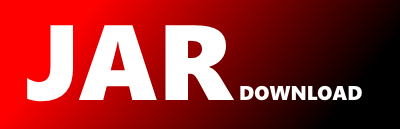
com.zuora.model.CreateBillingPreviewRunRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Zuora API Reference
* REST API reference for the Zuora Billing, Payments, and Central Platform! Check out the [REST API Overview](https://www.zuora.com/developer/api-references/api/overview/).
*
* The version of the OpenAPI document: 2024-05-20
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.zuora.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.zuora.model.PostBillingPreviewRunParamStorageOption;
import java.io.IOException;
import java.time.LocalDate;
import java.util.Arrays;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.zuora.JSON;
/**
* CreateBillingPreviewRunRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.6.0")
public class CreateBillingPreviewRunRequest {
public static final String SERIALIZED_NAME_ASSUME_RENEWAL = "assumeRenewal";
@SerializedName(SERIALIZED_NAME_ASSUME_RENEWAL)
private String assumeRenewal;
public static final String SERIALIZED_NAME_BATCH = "batch";
@Deprecated
@SerializedName(SERIALIZED_NAME_BATCH)
private String batch;
public static final String SERIALIZED_NAME_BATCHES = "batches";
@SerializedName(SERIALIZED_NAME_BATCHES)
private String batches;
public static final String SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE = "chargeTypeToExclude";
@SerializedName(SERIALIZED_NAME_CHARGE_TYPE_TO_EXCLUDE)
private String chargeTypeToExclude;
public static final String SERIALIZED_NAME_INCLUDING_DRAFT_ITEMS = "includingDraftItems";
@SerializedName(SERIALIZED_NAME_INCLUDING_DRAFT_ITEMS)
private Boolean includingDraftItems;
public static final String SERIALIZED_NAME_INCLUDING_EVERGREEN_SUBSCRIPTION = "includingEvergreenSubscription";
@SerializedName(SERIALIZED_NAME_INCLUDING_EVERGREEN_SUBSCRIPTION)
private Boolean includingEvergreenSubscription;
public static final String SERIALIZED_NAME_STORAGE_OPTION = "storageOption";
@SerializedName(SERIALIZED_NAME_STORAGE_OPTION)
private PostBillingPreviewRunParamStorageOption storageOption;
public static final String SERIALIZED_NAME_TARGET_DATE = "targetDate";
@SerializedName(SERIALIZED_NAME_TARGET_DATE)
private LocalDate targetDate;
public static final String SERIALIZED_NAME_COMPARED_BILLING_PREVIEW_RUN_ID = "comparedBillingPreviewRunId";
@SerializedName(SERIALIZED_NAME_COMPARED_BILLING_PREVIEW_RUN_ID)
private String comparedBillingPreviewRunId;
public static final String SERIALIZED_NAME_STORE_DIFFERENCE = "storeDifference";
@SerializedName(SERIALIZED_NAME_STORE_DIFFERENCE)
private Boolean storeDifference;
public CreateBillingPreviewRunRequest() {
}
public CreateBillingPreviewRunRequest assumeRenewal(String assumeRenewal) {
this.assumeRenewal = assumeRenewal;
return this;
}
/**
* Indicates whether to generate a preview of future invoice items and credit memo items with the assumption that the subscriptions are renewed. Set one of the following values in this field to decide how the assumption is applied in the billing preview. * **All:** The assumption is applied to all the subscriptions. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date. * **None:** (Default) The assumption is not applied to the subscriptions. Zuora generates preview invoice item data and credit memo item data based on the current term end date and the target date. * If the target date is later than the current term end date, Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the current term end date. * If the target date is earlier than the current term end date, Zuora generates preview invoice item data and credit memeo item data from the first day of the customer's next billing period to the target date. * **Autorenew:** The assumption is applied to the subscriptions that have auto-renew enabled. Zuora generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date. **Note:** - This field can only be used if the subscription renewal term is not set to 0. - The credit memo item data is only available if you have Invoice Settlement feature enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return assumeRenewal
*/
@javax.annotation.Nullable
public String getAssumeRenewal() {
return assumeRenewal;
}
public void setAssumeRenewal(String assumeRenewal) {
this.assumeRenewal = assumeRenewal;
}
@Deprecated
public CreateBillingPreviewRunRequest batch(String batch) {
this.batch = batch;
return this;
}
/**
* The customer batch to include in the billing preview run. If not specified, all customer batches are included. **Note**: This field is not available if you set the `zuora-version` request header to `314.0` or later.
* @return batch
* @deprecated
*/
@Deprecated
@javax.annotation.Nullable
public String getBatch() {
return batch;
}
@Deprecated
public void setBatch(String batch) {
this.batch = batch;
}
public CreateBillingPreviewRunRequest batches(String batches) {
this.batches = batches;
return this;
}
/**
* The customer batches to include in the billing preview run. You can specify multiple batches separated by comma. If not specified, all customer batches are included. **Note**: This field is only available if you set the `zuora-version` request header to `314.0` or later.
* @return batches
*/
@javax.annotation.Nullable
public String getBatches() {
return batches;
}
public void setBatches(String batches) {
this.batches = batches;
}
public CreateBillingPreviewRunRequest chargeTypeToExclude(String chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
return this;
}
/**
* The charge types to exclude from the forecast run. **Possible values:** OneTime, Recurring, Usage, and any comma-separated combination of these values.
* @return chargeTypeToExclude
*/
@javax.annotation.Nullable
public String getChargeTypeToExclude() {
return chargeTypeToExclude;
}
public void setChargeTypeToExclude(String chargeTypeToExclude) {
this.chargeTypeToExclude = chargeTypeToExclude;
}
public CreateBillingPreviewRunRequest includingDraftItems(Boolean includingDraftItems) {
this.includingDraftItems = includingDraftItems;
return this;
}
/**
* Whether draft document items are included in the billing preview run. By default, draft document items are not included. This field loads draft invoice items and credit memo items. The `chargeTypeToExclude`, `targetDate`, `includingEvergreenSubscription`, and `assumeRenewal` fields do not affect the behavior of the `includingDraftItems` field.
* @return includingDraftItems
*/
@javax.annotation.Nullable
public Boolean getIncludingDraftItems() {
return includingDraftItems;
}
public void setIncludingDraftItems(Boolean includingDraftItems) {
this.includingDraftItems = includingDraftItems;
}
public CreateBillingPreviewRunRequest includingEvergreenSubscription(Boolean includingEvergreenSubscription) {
this.includingEvergreenSubscription = includingEvergreenSubscription;
return this;
}
/**
* Whether evergreen subscriptions are included in the billing preview run. By default, evergreen subscriptions are not included.
* @return includingEvergreenSubscription
*/
@javax.annotation.Nullable
public Boolean getIncludingEvergreenSubscription() {
return includingEvergreenSubscription;
}
public void setIncludingEvergreenSubscription(Boolean includingEvergreenSubscription) {
this.includingEvergreenSubscription = includingEvergreenSubscription;
}
public CreateBillingPreviewRunRequest storageOption(PostBillingPreviewRunParamStorageOption storageOption) {
this.storageOption = storageOption;
return this;
}
/**
* Get storageOption
* @return storageOption
*/
@javax.annotation.Nullable
public PostBillingPreviewRunParamStorageOption getStorageOption() {
return storageOption;
}
public void setStorageOption(PostBillingPreviewRunParamStorageOption storageOption) {
this.storageOption = storageOption;
}
public CreateBillingPreviewRunRequest targetDate(LocalDate targetDate) {
this.targetDate = targetDate;
return this;
}
/**
* The target date for the billing preview run. The billing preview run generates preview invoice item data and credit memo item data from the first day of the customer's next billing period to the target date. The value for the `targetDate` field must be in _`YYYY-MM-DD`_ format. If the target date is later than the subscription current term end date, the preview invoice item data and credit memo item data is generated from the first day of the customer's next billing period to the current term end date. If you want to generate preview invoice item data and credit memo item data past the end of the subscription current term, specify the AssumeRenewal field in the request. **Note:** The credit memo item data is only available if you have Invoice Settlement feature enabled. The Invoice Settlement feature is generally available as of Zuora Billing Release 296 (March 2021). This feature includes Unapplied Payments, Credit and Debit Memo, and Invoice Item Settlement. If you want to enable Invoice Settlement, see [Invoice Settlement Enablement and Checklist Guide](https://knowledgecenter.zuora.com/Billing/Billing_and_Payments/Invoice_Settlement/Invoice_Settlement_Migration_Checklist_and_Guide) for more information.
* @return targetDate
*/
@javax.annotation.Nonnull
public LocalDate getTargetDate() {
return targetDate;
}
public void setTargetDate(LocalDate targetDate) {
this.targetDate = targetDate;
}
public CreateBillingPreviewRunRequest comparedBillingPreviewRunId(String comparedBillingPreviewRunId) {
this.comparedBillingPreviewRunId = comparedBillingPreviewRunId;
return this;
}
/**
* The ID of the billing preview run to compare with the current billing preview run.
* @return comparedBillingPreviewRunId
*/
@javax.annotation.Nullable
public String getComparedBillingPreviewRunId() {
return comparedBillingPreviewRunId;
}
public void setComparedBillingPreviewRunId(String comparedBillingPreviewRunId) {
this.comparedBillingPreviewRunId = comparedBillingPreviewRunId;
}
public CreateBillingPreviewRunRequest storeDifference(Boolean storeDifference) {
this.storeDifference = storeDifference;
return this;
}
/**
* Whether to store the difference between the current billing preview run and the compared billing preview run. The default value is `false`.
* @return storeDifference
*/
@javax.annotation.Nullable
public Boolean getStoreDifference() {
return storeDifference;
}
public void setStoreDifference(Boolean storeDifference) {
this.storeDifference = storeDifference;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the CreateBillingPreviewRunRequest instance itself
*/
public CreateBillingPreviewRunRequest putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateBillingPreviewRunRequest createBillingPreviewRunRequest = (CreateBillingPreviewRunRequest) o;
return Objects.equals(this.assumeRenewal, createBillingPreviewRunRequest.assumeRenewal) &&
Objects.equals(this.batch, createBillingPreviewRunRequest.batch) &&
Objects.equals(this.batches, createBillingPreviewRunRequest.batches) &&
Objects.equals(this.chargeTypeToExclude, createBillingPreviewRunRequest.chargeTypeToExclude) &&
Objects.equals(this.includingDraftItems, createBillingPreviewRunRequest.includingDraftItems) &&
Objects.equals(this.includingEvergreenSubscription, createBillingPreviewRunRequest.includingEvergreenSubscription) &&
Objects.equals(this.storageOption, createBillingPreviewRunRequest.storageOption) &&
Objects.equals(this.targetDate, createBillingPreviewRunRequest.targetDate) &&
Objects.equals(this.comparedBillingPreviewRunId, createBillingPreviewRunRequest.comparedBillingPreviewRunId) &&
Objects.equals(this.storeDifference, createBillingPreviewRunRequest.storeDifference)&&
Objects.equals(this.additionalProperties, createBillingPreviewRunRequest.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(assumeRenewal, batch, batches, chargeTypeToExclude, includingDraftItems, includingEvergreenSubscription, storageOption, targetDate, comparedBillingPreviewRunId, storeDifference, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateBillingPreviewRunRequest {\n");
sb.append(" assumeRenewal: ").append(toIndentedString(assumeRenewal)).append("\n");
sb.append(" batch: ").append(toIndentedString(batch)).append("\n");
sb.append(" batches: ").append(toIndentedString(batches)).append("\n");
sb.append(" chargeTypeToExclude: ").append(toIndentedString(chargeTypeToExclude)).append("\n");
sb.append(" includingDraftItems: ").append(toIndentedString(includingDraftItems)).append("\n");
sb.append(" includingEvergreenSubscription: ").append(toIndentedString(includingEvergreenSubscription)).append("\n");
sb.append(" storageOption: ").append(toIndentedString(storageOption)).append("\n");
sb.append(" targetDate: ").append(toIndentedString(targetDate)).append("\n");
sb.append(" comparedBillingPreviewRunId: ").append(toIndentedString(comparedBillingPreviewRunId)).append("\n");
sb.append(" storeDifference: ").append(toIndentedString(storeDifference)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("assumeRenewal");
openapiFields.add("batch");
openapiFields.add("batches");
openapiFields.add("chargeTypeToExclude");
openapiFields.add("includingDraftItems");
openapiFields.add("includingEvergreenSubscription");
openapiFields.add("storageOption");
openapiFields.add("targetDate");
openapiFields.add("comparedBillingPreviewRunId");
openapiFields.add("storeDifference");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("targetDate");
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to CreateBillingPreviewRunRequest
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!CreateBillingPreviewRunRequest.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in CreateBillingPreviewRunRequest is not found in the empty JSON string", CreateBillingPreviewRunRequest.openapiRequiredFields.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : CreateBillingPreviewRunRequest.openapiRequiredFields) {
if (jsonElement.getAsJsonObject().get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonElement.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("assumeRenewal") != null && !jsonObj.get("assumeRenewal").isJsonNull()) && !jsonObj.get("assumeRenewal").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `assumeRenewal` to be a primitive type in the JSON string but got `%s`", jsonObj.get("assumeRenewal").toString()));
}
if ((jsonObj.get("batch") != null && !jsonObj.get("batch").isJsonNull()) && !jsonObj.get("batch").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batch` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batch").toString()));
}
if ((jsonObj.get("batches") != null && !jsonObj.get("batches").isJsonNull()) && !jsonObj.get("batches").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `batches` to be a primitive type in the JSON string but got `%s`", jsonObj.get("batches").toString()));
}
if ((jsonObj.get("chargeTypeToExclude") != null && !jsonObj.get("chargeTypeToExclude").isJsonNull()) && !jsonObj.get("chargeTypeToExclude").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `chargeTypeToExclude` to be a primitive type in the JSON string but got `%s`", jsonObj.get("chargeTypeToExclude").toString()));
}
if ((jsonObj.get("storageOption") != null && !jsonObj.get("storageOption").isJsonNull()) && !jsonObj.get("storageOption").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `storageOption` to be a primitive type in the JSON string but got `%s`", jsonObj.get("storageOption").toString()));
}
// validate the optional field `storageOption`
if (jsonObj.get("storageOption") != null && !jsonObj.get("storageOption").isJsonNull()) {
PostBillingPreviewRunParamStorageOption.validateJsonElement(jsonObj.get("storageOption"));
}
if ((jsonObj.get("comparedBillingPreviewRunId") != null && !jsonObj.get("comparedBillingPreviewRunId").isJsonNull()) && !jsonObj.get("comparedBillingPreviewRunId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `comparedBillingPreviewRunId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("comparedBillingPreviewRunId").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CreateBillingPreviewRunRequest.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreateBillingPreviewRunRequest' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CreateBillingPreviewRunRequest.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CreateBillingPreviewRunRequest value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// support null values
out.beginObject();
Iterator iterator = obj.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry e = (Map.Entry) iterator.next();
out.name((String)e.getKey());
elementAdapter.write(out, e.getValue());
}
// end
// serialize additional properties
if (value.getAdditionalProperties() != null) {
// support null values
boolean oldSerializeNulls = out.getSerializeNulls();
out.setSerializeNulls(true); //force serialize
// end
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else if (entry.getValue() == null)
obj.add(entry.getKey(), null);
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
out.name((String)entry.getKey());
elementAdapter.write(out, obj.get(entry.getKey()));
}
out.setSerializeNulls(oldSerializeNulls); //restore
}
out.endObject();
}
@Override
public CreateBillingPreviewRunRequest read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
CreateBillingPreviewRunRequest instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of CreateBillingPreviewRunRequest given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreateBillingPreviewRunRequest
* @throws IOException if the JSON string is invalid with respect to CreateBillingPreviewRunRequest
*/
public static CreateBillingPreviewRunRequest fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CreateBillingPreviewRunRequest.class);
}
/**
* Convert an instance of CreateBillingPreviewRunRequest to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy